SLF4J: Your Guide to Simple Logging in Java
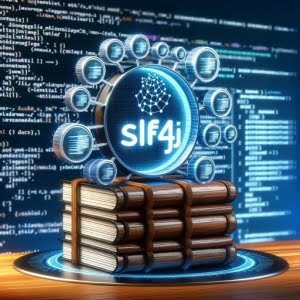
Are you finding it challenging to manage logging in your Java applications? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled interpreter, SLF4J (Simple Logging Facade for Java) can help you translate complex system events into readable logs. These logs can be a lifesaver when debugging or monitoring your application’s behavior.
This guide will walk you through the basics of SLF4J, its advanced usage, and the broader topic of logging in Java. We’ll explore SLF4J’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering SLF4J!
TL;DR: What is SLF4J and How Do I Use It?
SLF4J, or Simple Logging Facade for Java
, is a tool that allows you to use various logging frameworks interchangeably in your Java applications. It must be imported with the statementsimport org.slf4j.Logger;
andimport org.slf4j.LoggerFactory;
, It provides a simple and consistent logging interface that you can use regardless of your actual logging system.
Here’s a basic example of how to use SLF4J:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class HelloWorld {
final static Logger logger = LoggerFactory.getLogger(HelloWorld.class);
public static void main(String[] args) {
logger.info("Hello World");
}
}
# Output:
# 'Hello World'
In this example, we first import the necessary classes from the SLF4J library. We then define a Logger
instance using the LoggerFactory.getLogger()
method, passing in the class that we want to log (in this case, HelloWorld.class
). Finally, we use the logger.info()
method to log a simple message.
This is just a basic introduction to SLF4J. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Getting Started with SLF4J
SLF4J is a powerful tool, but it’s also incredibly easy to start using in your Java projects. Let’s walk through the basics of setting up the logger, logging messages at different levels, and configuring the logging format.
Setting Up the Logger
The first step in using SLF4J is setting up a Logger
instance. This instance will be the primary tool you use to log messages from your application. Here’s how you do it:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
}
In this code block, we import the Logger
and LoggerFactory
classes from the SLF4J library. We then define a Logger
instance using the LoggerFactory.getLogger()
method, passing in the class we want to log (MyApp.class
in this case).
Logging Messages at Different Levels
SLF4J supports logging messages at different levels, allowing you to control the verbosity of your logs. Here’s an example of how to log messages at various levels:
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.error("Error level log");
logger.warn("Warn level log");
logger.info("Info level log");
logger.debug("Debug level log");
logger.trace("Trace level log");
}
}
# Output:
# 'Error level log'
# 'Warn level log'
# 'Info level log'
# Depending on your settings, 'Debug level log' and 'Trace level log' might not be shown
In this example, we log messages at five different levels: error, warn, info, debug, and trace. The level you choose determines the importance of the message, with ‘error’ being the most critical and ‘trace’ being the least.
Configuring the Logging Format
SLF4J allows you to customize the format of your log messages. This is typically done through the configuration file of the logging system you’re using (like Logback or Log4j). Here’s an example of a basic Logback configuration file that sets a custom logging format:
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="debug">
<appender-ref ref="STDOUT" />
</root>
</configuration>
In this configuration file, we define a pattern for our log messages that includes the timestamp, thread name, log level, logger name, and the actual message. We also set the default log level to ‘debug’.
By mastering these fundamental aspects of SLF4J, you’ll be well on your way to effectively logging your Java applications.
Advanced Features of SLF4J
Once you’ve mastered the basics of SLF4J, you can start exploring its more advanced features. These include using markers, parameterized logging, and MDC (Mapped Diagnostic Context). Each of these features can greatly enhance your logging capabilities.
Utilizing Markers
Markers provide a way to add metadata to your log messages, which can be useful for filtering or grouping related messages. Here’s an example of how to use markers:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.Marker;
import org.slf4j.MarkerFactory;
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
final static Marker USER_MARKER = MarkerFactory.getMarker("USER_LOG");
public static void main(String[] args) {
logger.info(USER_MARKER, "This is a user log");
}
}
# Output:
# 'This is a user log'
In this example, we first define a Marker
using the MarkerFactory.getMarker()
method. We then log a message with this marker using the logger.info()
method.
Parameterized Logging
Parameterized logging allows you to embed variables directly into your log messages. This can be a more efficient and cleaner way to log dynamic data. Here’s how it works:
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
String user = "John";
logger.info("User {} has logged in", user);
}
}
# Output:
# 'User John has logged in'
In this example, we log a message with a parameter (user
). The {}
in the log message is a placeholder that gets replaced with the value of user
.
Leveraging Mapped Diagnostic Context (MDC)
MDC is a powerful feature that allows you to add contextual data to your logs. This can be extremely useful for tracking the flow of execution across multiple threads or processes. Here’s an example:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.MDC;
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
MDC.put("user", "John");
logger.info("User has logged in");
MDC.clear();
}
}
# Output:
# 'User has logged in'
In this example, we first put a value (John
) into the MDC under the key user
. We then log a message, and the MDC value is automatically included in the log output. Finally, we clear the MDC to prevent the value from being included in subsequent log messages.
By leveraging these advanced features of SLF4J, you can greatly enhance the richness and usefulness of your logs.
Exploring Other Java Logging Frameworks
While SLF4J is a powerful tool, it’s not the only logging framework available for Java. It’s important to understand your options and choose the right tool for your specific needs. Let’s take a look at two other popular logging frameworks: Log4j and java.util.logging.
Log4j: A Versatile Logging Framework
Log4j is a reliable, flexible, and fast logging framework developed by the Apache Software Foundation. It’s highly configurable through XML, JSON, and YAML files, and it offers a wide range of features, including logging priority levels, output formatting, and more.
Here’s a simple example of logging with Log4j:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class MyApp {
private static final Logger logger = LogManager.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Hello, Log4j");
}
}
# Output:
# 'Hello, Log4j'
In this example, we use the LogManager
class to create a Logger
instance, and then we log a message using the logger.info()
method.
java.util.logging: Java’s Built-In Logger
java.util.logging, also known as JUL, is a logging framework that’s included in the Java Standard Edition (Java SE) platform. It provides basic logging capabilities and is a good choice for simple applications or for getting started with logging in Java.
Here’s a basic example of logging with JUL:
import java.util.logging.Logger;
public class MyApp {
private static final Logger logger = Logger.getLogger(MyApp.class.getName());
public static void main(String[] args) {
logger.info("Hello, JUL");
}
}
# Output:
# 'Hello, JUL'
In this example, we use the Logger
class to create a Logger
instance, and then we log a message using the logger.info()
method.
SLF4J vs. Log4j vs. JUL: A Comparison
Feature | SLF4J | Log4j | JUL |
---|---|---|---|
Ease of Use | High | Medium | High |
Flexibility | High | High | Medium |
Performance | High | High | Medium |
Configuration Options | Medium | High | Low |
While all three frameworks can handle logging in Java applications, they each have their strengths and weaknesses. SLF4J stands out for its simplicity and flexibility, especially when used in conjunction with a powerful logging system like Logback. Log4j offers a wide range of features and high performance, but it can be more complex to set up and configure. JUL is a good choice for simple applications or for getting started with logging, but it may not offer the level of flexibility and configurability that larger applications require.
Ultimately, the best logging framework for your Java application depends on your specific needs and circumstances.
Troubleshooting Common SLF4J Issues
As with any tool, you might encounter some issues when using SLF4J. Let’s discuss some of the common problems that developers face, such as missing log messages, incorrect log levels, and performance considerations. We’ll also provide solutions and best practices to help you avoid these issues.
Missing Log Messages
One common problem you might encounter is missing log messages. This usually happens when the log level is set too high, causing lower-level messages to be ignored.
Here’s an example:
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.debug("Debug level log");
}
}
# Output:
# No output
In this example, the debug
log message doesn’t appear in the output. This could be because the log level is set to info
or higher, causing debug
messages to be ignored.
To fix this issue, you can adjust the log level in your logging system’s configuration file. For example, if you’re using Logback, you can set the log level to debug
or lower in your logback.xml
file.
Incorrect Log Levels
Another common issue is logging messages at the wrong level. This can make your logs harder to read and understand, especially when you’re dealing with a large number of messages.
To avoid this issue, it’s important to understand the different log levels and when to use them. As a general rule, use error
for serious issues that prevent your application from running correctly, warn
for potential problems that don’t immediately affect your application’s operation, info
for important system events, debug
for detailed information that’s useful for debugging, and trace
for fine-grained details about your application’s behavior.
Performance Considerations
Logging can impact your application’s performance, especially if you’re logging a large number of messages or logging at a low level (like debug
or trace
).
To minimize this impact, consider the following best practices:
- Log at the appropriate level: Avoid logging too many
debug
ortrace
messages in a production environment. - Use parameterized logging: This can reduce the overhead of constructing log messages.
- Limit the size of your log files: This can prevent your disk from filling up and slowing down your system.
By keeping these considerations in mind, you can make the most of SLF4J while avoiding common pitfalls.
The Fundamentals of Logging and SLF4J
To understand the role of SLF4J, it’s essential to grasp the importance of logging in software development, the concept of logging facades and frameworks, and how these pieces fit together in the Java logging ecosystem.
The Importance of Logging in Software Development
Logging is a critical aspect of software development. It provides visibility into the behavior of your applications, helping you understand what’s happening under the hood. Whether you’re debugging an issue, monitoring performance, or conducting a post-mortem analysis of a system failure, logs are an invaluable tool.
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Application started");
// Your application code here
logger.info("Application ended");
}
}
# Output:
# 'Application started'
# 'Application ended'
In this example, we’re logging the start and end of an application’s execution. These logs can help us track the application’s lifecycle and identify any unexpected behavior or errors.
Understanding Logging Facades and Frameworks
A logging facade, like SLF4J, provides a simple, unified interface for logging, while a logging framework (like Log4j or java.util.logging) provides the underlying implementation. The facade allows you to switch between different logging frameworks without changing your application code.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Hello, SLF4J");
}
}
# Output:
# 'Hello, SLF4J'
In this example, we’re using SLF4J to log a message. Regardless of the underlying logging framework (Log4j, JUL, etc.), the application code remains the same.
SLF4J’s Role in the Java Logging Ecosystem
SLF4J plays a crucial role in the Java logging ecosystem by serving as a simple, efficient facade for various logging frameworks. It allows developers to use a consistent logging interface, reducing the complexity and enhancing the portability of their code.
By understanding these fundamentals, you’ll be able to fully leverage the power of SLF4J and appreciate its role in the broader context of Java logging.
Expanding Your Logging Horizons with SLF4J
SLF4J’s utility goes beyond just individual applications. In larger software projects, SLF4J becomes an essential tool, working in tandem with other practices like log aggregation and analysis, and application performance monitoring. Let’s delve into how SLF4J fits into these broader contexts.
SLF4J in Large Software Projects
In large-scale software projects, logging becomes a critical part of monitoring and maintaining the system. SLF4J’s ability to work with different logging frameworks makes it a flexible choice for these projects. With SLF4J, you can seamlessly switch between logging frameworks depending on the needs of your project.
SLF4J and Log Aggregation & Analysis
Log aggregation is the process of collecting log data from different sources and consolidating it into one centralized location. Log analysis is the subsequent step of interpreting this data to extract meaningful insights.
SLF4J can play a crucial role in this process. By providing a consistent logging interface across your applications, SLF4J ensures that your log data is uniform and easier to analyze.
SLF4J and Application Performance Monitoring
Application Performance Monitoring (APM) is a practice that involves tracking an application’s performance to ensure it’s running optimally. Logs can provide valuable data for APM, including error reports, execution times, and system events.
With SLF4J, you can customize your logs to include the data most relevant to your performance monitoring needs. This makes SLF4J a valuable tool in any APM strategy.
Further Resources for Mastering SLF4J
To continue your journey in mastering SLF4J and Java logging, consider the following resources:
- Mastering Javadoc: Essential Documentation Techniques – Explore the features and benefits of Javadoc for documenting Java code efficiently.
Javadoc Comments Overview – Learn how to use Javadoc tags to document classes, methods, and fields.
Understanding Log4j in Java – Learn Log4j configuration for different logging needs.
SLF4J User Manual includes a comprehensive overview of its features and usage.
Logback Documentation – Detailed documentation for a popular logging framework that’s often used with SLF4J.
Java Logging Basics – A tutorial on logging in Java, including an introduction to different logging frameworks.
By exploring these resources and understanding the broader implications of logging, you’ll be well-equipped to tackle any logging challenges in your Java projects.
Wrapping Up: Simple Logging Facade for Java
In this comprehensive guide, we’ve delved into the world of SLF4J, a simple yet powerful logging facade for Java. We’ve explored its role in translating complex system events into readable and manageable logs, providing a crucial tool for developers to monitor and debug their Java applications.
We began with the basics of SLF4J, learning how to set up a logger, log messages at different levels, and configure the logging format. We then ventured into more advanced territory, exploring features such as using markers, parameterized logging, and MDC (Mapped Diagnostic Context). These advanced features allow developers to enhance their logs with additional metadata, dynamic data, and contextual information, making the logs richer and more useful.
Along the way, we tackled common challenges you might encounter when using SLF4J, such as missing log messages, incorrect log levels, and performance considerations. We provided solutions and best practices for each issue, helping you to avoid these pitfalls and make the most of SLF4J.
We also looked at alternative approaches to logging in Java, comparing SLF4J with other popular logging frameworks like Log4j and java.util.logging. Here’s a quick comparison of these frameworks:
Framework | Ease of Use | Flexibility | Performance | Configuration Options |
---|---|---|---|---|
SLF4J | High | High | High | Medium |
Log4j | Medium | High | High | High |
java.util.logging | High | Medium | Medium | Low |
Whether you’re just starting out with SLF4J or looking to level up your Java logging skills, we hope this guide has given you a deeper understanding of SLF4J and its capabilities.
With its balance of simplicity, flexibility, and performance, SLF4J is a powerful tool for logging in Java. It provides a consistent logging interface across your applications, reducing complexity and enhancing portability. Happy coding!