Python Read File Quick Guide with Examples
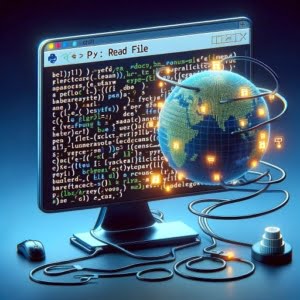
File handling is a cornerstone of Python programming, with file reading being a critical segment. Whether your task involves text files, CSVs, or JSON data, Python offers an impressively simple method to open, read, and process files.
The best part? Mastering file reading in Python equips you with a potent tool for data analysis, web development, and much more.
Our goal is clear-cut: to arm you with the expertise to proficiently read files in Python. By the conclusion of this guide, you’ll have a firm grasp on Python’s file reading principles, be able to manage various file types, and troubleshoot common issues.
So, are you prepared to explore the might of Python’s file reading prowess? Let’s dive in!
TL;DR: How does Python read files?
Python reads files using the built-in
open()
function in ‘read’ mode ('r'
), followed by theread()
method. The content of the file is then stored in a variable for further processing. It’s important to close the file after reading to free up system resources. See the rest of the article for more advanced methods, background, tips and tricks.
# Open the file
file = open('example.txt', 'r')
# Read the file
content = file.read()
# Close the file
file.close()
Table of Contents
Python File Reading Basics
At its core, file reading in Python is about accessing and interpreting the data stored in a file. Here’s the fundamental principle: Python reads files as a stream of bytes, which it can then interpret as text, numbers, or any other type of data.
To read a file in Python, we employ the built-in open()
function, followed by the read()
method.
Consider the following example:
# Open the file
file = open('example.txt', 'r')
# Read the file
content = file.read()
# Print the content
print(content)
# Close the file
file.close()
In this instance, 'example.txt'
is the name of the file we wish to read, and 'r'
is the mode in which we open the file. The 'r'
mode signifies ‘read’, indicating that we’re opening the file intending to read its content.
The read()
method then reads the entire content of the file, which we store in the content
variable. Lastly, we print the content to the console and close the file using file.close()
.
The output of the
read()
method is a string encapsulating the entire content of the file. If ‘example.txt’ contained the text ‘Hello, World!’, our code would output the same text.
Python’s file handling capabilities are revered for their simplicity and versatility. With a few lines of code, you can open, read, and process files in various formats.
Closing Files Automatically using “with”
It’s crucial to remember to close the file once you’re finished reading it. Leaving files open can result in memory leaks and other problems.
The with
statement assists in this, automatically closing the file when the block of code is exited. Here’s a code example:
# Instead of doing this...
file = open('example.txt', 'r')
print(file.read())
file.close() # Don't forget this!
# You can do this...
with open('example.txt', 'r') as file:
print(file.read())
# The file is automatically closed at this point
In the second example, the with
statement automatically closes the file after it’s no longer needed, even if exceptions were raised within the with
block. This makes your code safer and easier to read.
Advanced File Reading in Python
Python presents an array of methods for reading files, each suited to different use cases and offering unique advantages. Let’s delve into some of these advanced techniques and gain an understanding of their application.
Modes and Options
Python allows you to specify different modes when opening a file. We’ve already encountered the ‘r’ mode for reading, but there exist others like ‘w’ for writing, ‘a’ for appending, and ‘b’ for binary mode. You can even combine these modes, for instance, ‘rb’ opens the file in binary mode for reading.
Here is a table summarizing the different modes:
Mode | Description |
---|---|
‘r’ | Read mode |
‘w’ | Write mode (creates a new file or truncates an existing file) |
‘a’ | Append mode (appends to an existing file or creates a new file if it doesn’t exist) |
‘b’ | Binary mode |
‘rb’ | Binary mode for reading |
‘wb’ | Binary mode for writing |
‘ab’ | Binary mode for appending |
Consider the following example of opening a file in binary mode for reading:
# Open the file in binary mode for reading
file = open('example.bin', 'rb')
# Read the file
content = file.read()
# Close the file
file.close()
Reading Various File Types
Python can manage a range of file types. Let’s examine how to read a CSV file and a JSON file.
CSV Module
Reading a CSV file is straightforward with the csv
module:
Assume we have a CSV file ‘example.csv’ with the following content:
Name,Age,Occupation
John,30,Engineer
Jane,28,Doctor
And our Python code for reading it:
import csv
# Open the CSV file
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# ['Name', 'Age', 'Occupation']
# ['John', '30', 'Engineer']
# ['Jane', '28', 'Doctor']
JSON Module
For JSON files, the json
module can be employed:
Assume we have a JSON file ‘example.json’ with the following content:
{
'Name': 'John',
'Age': 30,
'Occupation': 'Engineer'
}
And our Python code for reading it:
import json
# Open the JSON file
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
# Output:
# {'Name': 'John', 'Age': 30, 'Occupation': 'Engineer'}
Handling Errors
There’s always a risk of encountering errors when reading files. The file might not exist, or there might be a reading error.
File Not Found Error
The FileNotFoundError
is one of the most frequent errors encountered when reading files in Python. This arises when Python is unable to locate the file you’re attempting to open. To prevent this, always verify your file paths and ensure the file’s existence prior to opening it.
Python’s try/except
structure enables you to gracefully handle these errors:
try:
# Attempt to open and read the file
with open('example.txt', 'r') as file:
print(file.read())
except FileNotFoundError:
# Handle the error
print('File not found')
PermissionError
The PermissionError
is triggered when you try to open a file for which you don’t have the required permissions. This often happens when you try to read a file that is write-protected or attempt to open a system file that requires administrative rights. Here’s an example on how to handle this error:
try:
# Attempt to open and read the file
with open('example.txt', 'r') as file:
print(file.read())
except PermissionError:
# Handle the permission error
print('Permission denied')
IOError
An IOError
signifies that there’s a problem with the input or output. This could be due to the file being in use by another program or the disk being full. The following code shows how this error can be managed:
try:
# Attempt to open and read the file
with open('example.txt', 'r') as file:
print(file.read())
except IOError:
# Handle the IO error
print('An I/O error occurred')
Handling All Errors
If you want to catch all types of errors in one fell swoop (perhaps to print a general error message or perform some cleanup), use the base Exception
class in your except
clause.
The base
Exception
class will handle any exception that is an instance of theException
class (almost all exceptions are derived from this class)
Here’s an example where we first catch the FileNotFoundError
specifically, and then catch all other exceptions using the general Exception
class:
try:
# Attempt to open and read the file
with open('example.txt', 'r') as file:
print(file.read())
except FileNotFoundError:
# Handle the file not found error
print('File not found')
except Exception:
# Handle all other types of errors
print('An error occurred')
This way, if the error is a FileNotFoundError
, it will be caught and handled by the first except
clause. If the error is of any other type, it will be caught and handled by the second except
clause.
File Handling in Python: A Broader Perspective
While our primary focus in this guide is on reading files in Python, it’s crucial to understand that Python’s file handling capabilities extend far beyond this.
File handling in Python is a comprehensive skill set that includes reading, writing, and updating files, all of which are vital for many real-world applications.
Beyond Reading: Writing and Updating Files
Python doesn’t limit you to just reading files; it also facilitates writing and updating files. The write()
method enables you to write data to a file. Furthermore, the open()
function’s w
and a
modes allow you to overwrite or append data to a file, respectively.
Here’s a basic example of writing to a file in Python:
# Open the file in write mode
with open('example.txt', 'w') as file:
# Write to the file
file.write('Hello, World!')
And here’s an example of appending to a file in Python:
# Open the file in append mode
with open('example.txt', 'a') as file:
# Append to the file
file.write('Hello, again!')
OS Module for File Operations
The os
library offers a way to utilize operating system-dependent functionality, like reading or writing to the file system.
Here’s an example of using the os
library to list all files in the current directory:
import os
# List all files in the current directory
files = os.listdir('.')
print(files)
Advanced Tools and Libraries
Python’s extensive ecosystem boasts libraries such as pandas
for data analysis, capable of reading files in formats like CSV and Excel, and pickle
for serializing and deserializing Python object structures.
Here’s an example of using pandas and pickle:
import pandas as pd
# Load a CSV file into a pandas DataFrame
df = pd.read_csv('example.csv')
import pickle
# Serialize a Python object
with open('example.pkl', 'wb') as file:
pickle.dump(my_object, file)
# Deserialize a Python object
with open('example.pkl', 'rb') as file:
my_object = pickle.load(file)
Further Resources for Python File Management
To deepen your understanding of Python file classes, and methods, here are some resources that you might find helpful:
- Python OS Module Syntax – Dive into the world of file and directory renaming, moving, and copying with “os.”
Removing Files with Python “os.remove()” – Discover how to clean up files and folders in Python programs effectively.
Reading Files Line by Line in Python – Learn how to read files line by line in Python for efficient data handling.
Python’s official documentation on input and output outlines the ways to display outputs effectively and to read inputs in various types.
Python’s official filesys library documentation details the functions and methods available in Python’s file system manipulation library.
Python file methods introduces all the built-in methods used for file handling in Python.
Wrapping Up:
Through this comprehensive guide, we’ve journeyed through the various aspects of reading files in Python. From the simplicity of the open()
and read()
functions to the more advanced techniques and error handling mechanisms, we’ve seen how Python simplifies the process of reading files, making it accessible and straightforward.
However, reading files is merely one aspect of Python’s file handling capabilities. File handling in Python is a broad domain, encompassing not just reading, but also writing and updating files. These capabilities, in combination with Python’s robust libraries and tools, make Python an excellent choice for working with a plethora of data types and formats.
In conclusion, Python’s file reading capabilities are not only simple and powerful but also versatile. Whether you’re a novice venturing into Python or an experienced programmer, mastering file reading in Python is an invaluable skill that will undoubtedly enhance your programming journey.