Python Try / Except: How to Catch Errors (With Examples)
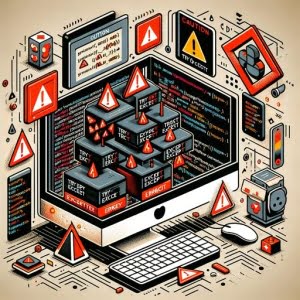
Ever found yourself stuck with error handling in Python? You’re not alone. Python’s try
and except
blocks function like a safety net, catching errors and ensuring your program runs without hiccups.
This comprehensive guide will walk you through the intricacies of using try
and except
in Python. Whether you’re a beginner or an advanced Python programmer, we’ve got you covered.
We’ll start from the basics and gradually move to more advanced techniques, complete with code examples and their explanations. So, buckle up and let’s dive into the world of Python exception handling with try
and except
.
TL;DR: How Do I Use Try and Except in Python?
Try and except blocks in Python are used to catch and handle exceptions. Here’s a simple illustration:
try:
x = 1 / 0
except ZeroDivisionError:
x = 0
print(x)
# Output:
# 0
In this example, we’re attempting to divide by zero, which would normally throw a ZeroDivisionError
. However, our try
and except
blocks catch this exception and handle it by setting x
to 0. Therefore, instead of our program crashing, it prints out ‘0’.
For a more detailed explanation and advanced usage scenarios, keep reading!
Table of Contents
Python Try and Except: The Basics
In Python, try
and except
blocks are used to catch and handle exceptions. Exceptions are errors that occur during the execution of a program. When an exception is encountered in the try
block, the flow of control is immediately transferred to the except
block where the exception is handled.
Here’s a simple example:
try:
x = 1 / 0
except ZeroDivisionError:
x = 0
print(x)
# Output:
# 0
In this code, we attempt to divide 1 by 0 within the try
block. Since division by zero is mathematically undefined, Python throws a ZeroDivisionError
exception. However, because this operation is inside a try
block, the exception is caught and control is passed to the except
block. Here, we handle the ZeroDivisionError
by assigning x
the value 0, thus preventing the program from crashing.
Catching Specific Exceptions
One of the key advantages of using try
and except
is the ability to catch specific exceptions. Python has numerous built-in exceptions (like ZeroDivisionError
, TypeError
, IndexError
, etc.) that you can catch and handle. By specifying the exception type in the except
block, you can tailor your error handling to the specific issue.
Potential Pitfalls
While try
and except
blocks are powerful tools, they should be used judiciously. Catching too many exceptions or catching exceptions broadly can mask errors and make debugging more difficult. It’s generally best to catch and handle only those exceptions that you expect and know how to handle.
Advanced Python Exception Handling
As you delve deeper into Python, you’ll encounter situations that require more complex exception handling. Python’s try
and except
blocks offer several advanced features to handle these scenarios.
Catching Multiple Exceptions
Python allows you to catch multiple exceptions in a single except
block. This is particularly useful when different exceptions can be handled in the same way. Here’s an example:
try:
# some code here
except (TypeError, ZeroDivisionError) as e:
print(f'Caught an exception: {e}')
In this code, both TypeError
and ZeroDivisionError
are caught by the same except
block and handled similarly.
The Else Clause
Python’s try
and except
blocks also support an else
clause. The else
clause is executed if the try
block doesn’t throw any exceptions. Here’s how you can use it:
try:
x = 1 / 2
except ZeroDivisionError:
print('Division by zero!')
else:
print('No exceptions were thrown.')
# Output:
# No exceptions were thrown.
In this example, since no exceptions are thrown in the try
block, the else
clause is executed.
The Finally Clause
The finally
clause is executed no matter what, making it ideal for cleanup operations. Whether an exception is thrown or not, the finally
clause always runs. Here’s an example:
try:
x = 1 / 0
except ZeroDivisionError:
print('Division by zero!')
finally:
print('This always executes.')
# Output:
# Division by zero!
# This always executes.
In this code, despite the ZeroDivisionError
exception, the finally
clause is executed.
Alternatives to Try and Except
While try
and except
are widely used for exception handling in Python, there are other methods you can use to manage errors. These include using the assert
statement and raising custom exceptions.
Python’s Assert Statement
The assert
statement is used for debugging purposes. It checks if a certain condition is true. If the condition is false, the program will stop and give an AssertionError
.
x = 5
assert x < 4, 'x is too high'
# Output:
# AssertionError: x is too high
In this example, the assert
statement checks whether x
is less than 4. Since x
is 5, the condition is false and an AssertionError
is raised with the message ‘x is too high’.
Raising Custom Exceptions
Python also allows you to raise your own exceptions using the raise
keyword. This can be useful for making your code more readable and easier to debug.
x = 5
if x > 4:
raise Exception('x should not exceed 4.')
# Output:
# Exception: x should not exceed 4.
In this code, we raise an Exception
if x
is greater than 4. Since x
is 5, our custom exception is raised with the message ‘x should not exceed 4.’
While these methods offer more control and can help improve your code’s readability, they should be used judiciously. Overuse of assert
statements or custom exceptions can make your code harder to understand and maintain. As always, it’s important to strike a balance between robust error handling and clean, readable code.
Troubleshooting Tips: Try and Except
While try
and except
blocks are powerful tools for handling exceptions in Python, they can also present certain challenges. Here, we’ll discuss some of the common issues you may encounter when using try
and except
, along with solutions and workarounds.
Catching Too Many Exceptions
One common pitfall is catching too many exceptions. This can mask errors and make debugging more difficult. For instance, consider the following code:
try:
x = 1 / 0
except:
x = 0
print(x)
# Output:
# 0
Here, the except
block without any specified exception type catches all exceptions, not just ZeroDivisionError
. This can be problematic because it may catch and ignore exceptions you didn’t anticipate, making it harder to debug your code. A better approach is to catch and handle only the exceptions you expect.
Not Handling Exceptions Properly
Another issue is not handling exceptions properly. When an exception is caught, it’s crucial to handle it in a way that doesn’t disrupt the flow of the program or leaves resources in an uncertain state. For instance, if an exception occurs while a file is open, it’s important to ensure the file is closed before the program continues. This can be achieved using the finally
clause.
try:
f = open('file.txt', 'r')
# some code here
except IOError:
print('An error occurred.')
finally:
f.close()
In this code, regardless of whether an exception is thrown, the finally
clause ensures that the file f
is closed.
Remember, the goal of using try
and except
is not just to prevent your program from crashing, but also to handle exceptions in a way that allows your program to continue running correctly.
Fundamentals of Exception Handling
Python’s exception handling mechanism is built around try
and except
blocks. But to fully appreciate its power and flexibility, it’s important to understand the fundamentals of how Python raises and handles exceptions.
Hierarchy of Exceptions
In Python, all exceptions are instances of classes that derive from the built-in BaseException
class. The Exception
class is a direct child of BaseException
and serves as the base class for most built-in exceptions. This hierarchy allows you to catch multiple related exceptions by catching their common ancestor. For example, catching Exception
will catch all built-in, non-system-exiting exceptions, whether they are standard exceptions, warnings, or your own custom exceptions.
Raising Exceptions
Python raises an exception whenever it encounters an error that it cannot handle. This can be a built-in exception (like ZeroDivisionError
or TypeError
) or a custom exception that you define. Exceptions can be raised using the raise
statement.
raise ValueError('A value error occurred.')
# Output:
# ValueError: A value error occurred.
In this example, we raise a ValueError
with a custom error message.
Handling Exceptions
When an exception is raised, Python looks for an except
block that can handle it. If it finds one, control is passed to that block and the exception is handled. If it doesn’t find an except
block (either because there isn’t one or because none of them can handle the exception), the program terminates with an error message.
try:
raise ValueError('A value error occurred.')
except ValueError as e:
print(f'Caught an exception: {e}')
# Output:
# Caught an exception: A value error occurred.
In this code, the ValueError
we raise is caught and handled by the except
block.
Large Programs and Exceptions
Exception handling isn’t just about preventing your program from crashing when an error occurs. In larger Python programs, it plays a crucial role in maintaining the program’s overall structure and flow of control.
Consider a Python web application. If an exception occurs while processing a user’s request, you wouldn’t want the entire application to crash. Instead, you’d want to catch the exception, log it, and possibly return an error message to the user. This is where try
and except
come in. By wrapping the request processing code in a try
block, you can catch any exceptions that occur and handle them appropriately.
try:
# process user request
except Exception as e:
# log exception and return error message
log_exception(e)
return 'An error occurred. Please try again later.'
In this code, if an exception occurs while processing the user’s request, it’s caught and logged, and a friendly error message is returned to the user. This ensures that even when an error occurs, the application can continue running and serving other requests.
Exploring Related Concepts
If you’re interested in deepening your understanding of Python exception handling, there are several related concepts you can explore. These include logging exceptions and writing unit tests.
Logging exceptions is a good practice as it helps you understand the errors that occurred while your program was running. Python’s built-in logging
module makes it easy to log exceptions.
Writing unit tests is another important aspect of robust Python programming. By writing tests that intentionally cause exceptions, you can ensure that your try
and except
blocks are working as expected.
More Resources for Exception and Error Handling
To further deepen your understanding and refine your skills in Exception and Error Handling in Python, the following resources are worth considering:
- Pytest and Test-Driven Development in Python – Explore real-world use cases of Pytest and its advantages in Python software testing.
Best Practices for Debugging and Monitoring – Dive into the world of logging in Python and explore various log levels and configurations.
Dealing with KeyError Exceptions in Python – Best Practices for handling KeyError exceptions in Python for reliable dictionary operations.
FreeCodeCamp: Exception Handling in Python – A comprehensive guide by FreeCodeCamp discussing various aspects of exception handling in Python.
Automated Software Testing with Python – This tutorial from Geeks For Geeks provides an overview of automated software testing in Python.
Real Python: Python Debugging Course – Real Python presents a course dedicated to Python debugging using pdb, the built-in Python debugger.
Embrace these materials and make your journey in Python exception and error handling an enlightening one.
Wrapping Up: Python Try and Except
In this guide, we’ve explored the fundamentals and advanced usage of try
and except
in Python. We’ve learned how these blocks serve as a safety net, catching and handling exceptions to prevent our program from crashing.
We started with the basics, learning how to use try
and except
to catch specific exceptions and handle them. We saw this in action with a simple code snippet:
try:
x = 1 / 0
except ZeroDivisionError:
x = 0
print(x)
# Output:
# 0
We then delved into more complex uses, such as catching multiple exceptions and using the else
and finally
clauses. We also explored alternative approaches to error handling, including the assert
statement and raising custom exceptions.
Throughout, we’ve emphasized the importance of using try
and except
judiciously. Catching too many exceptions or not handling exceptions properly can lead to hard-to-debug code and potential issues down the line.
Finally, we’ve seen how exception handling fits into the bigger picture of Python programming, particularly in larger programs. We’ve discussed related concepts like logging exceptions and writing unit tests, which can further improve your error handling.
In summary, try
and except
are powerful tools in Python’s error handling arsenal. Used correctly, they can help you write robust, error-resistant code. Happy coding!