Python filter() Function Guide (With Examples)
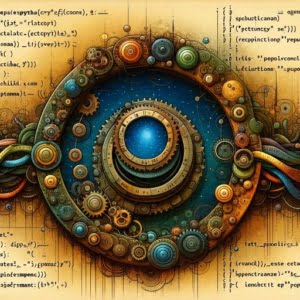
Imagine you’re in a vast desert, and you’re looking for hidden gold nuggets. It’s a daunting task, isn’t it? But what if you could easily separate the gold from the dirt?
In Python, the filter function helps you sift through massive amounts of data, separating the gold nuggets from the dirt and rocks.
This guide aims to provide you with a comprehensive understanding of Python’s filter function, diving deep into its syntax, usage, and various scenarios where it proves to be an invaluable tool. So, let’s get ready to unearth the power of the filter function in Python!
TL;DR: What is the filter function in Python?
The filter function in Python is a built-in function that creates a new list from an existing one, keeping only those elements that satisfy a certain condition. The condition is defined by a function you pass as an argument.
Here’s a simple example:
# list of numbers
numbers = [1, 2, 3, 4, 5, 6]
# function to check if a number is even
def is_even(n):
return n % 2 == 0
# using filter function
even_numbers = filter(is_even, numbers)
# converting the result to a list
print(list(even_numbers)) # Output: [2, 4, 6]
For more advanced methods, background, tips and tricks, continue reading the rest of the article.
Table of Contents
Basics of Python Filter
Simply put, the filter function in Python is an in-built function designed to create a new list from an existing one. This new list contains only those elements that satisfy a specific condition. This condition is established by the function you pass as the first argument.
Syntax of Python Filter Function
The syntax of the filter function is straightforward. It looks like this:
filter(function, iterable)
Here’s a breakdown of the parameters:
Parameter | Description |
---|---|
function | The function that tests if each element of the iterable is true or not. |
iterable | The sequence (like a list, tuple, etc.) which needs to be filtered. |
In this syntax, ‘function’ is the function that tests each element in the iterable to be true or not. The ‘iterable’ refers to the sequence (like a list, tuple, etc.) which needs to be filtered. It can be any sequence that the function will process.
A Simple Example of Python Filter
To understand this better, let’s consider a simple example. Suppose we have a list of numbers, and we want to filter out all the even numbers. Here’s how we can accomplish it:
# our number list
numbers = [1, 2, 3, 4, 5, 6]
# function that tests if a number is even
def is_even(n):
return n % 2 == 0
# using filter function
even_numbers = filter(is_even, numbers)
# converting the result to a list and printing
print(list(even_numbers)) # Output: [2, 4, 6]
In this example, is_even
is the function that tests if a number is even, and numbers
is the list we want to filter.
The Power of Python Filter Function
The beauty of the filter function lies in its efficiency. Instead of writing a loop to filter out data, you can achieve the same result with just a single line of code using the filter function. This not only makes your code cleaner but also significantly faster, especially when dealing with large datasets.
Here’s a comparison of using a loop vs the filter function to filter out even numbers from a list:
# Using a loop
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = []
for n in numbers:
if n % 2 == 0:
even_numbers.append(n)
print(even_numbers) # Output: [2, 4, 6]
# Using filter function
def is_even(n):
return n % 2 == 0
even_numbers = filter(is_even, numbers)
print(list(even_numbers)) # Output: [2, 4, 6]
Advanced Use of Python Filter
With a basic understanding of the filter function under our belt, let’s go a step further and explore its advanced usage. The filter function is a versatile tool that can be employed in a variety of complex scenarios, making it a must-have in every Python developer’s toolkit.
Filtering Based on Multiple Conditions
One such scenario is filtering data based on multiple conditions. For instance, consider a situation where you have a list of numbers and your task is to filter out all the numbers that are either less than 5 or greater than 10. Here’s how you can achieve it:
# our number list
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
# function that tests if a number is less than 5 or greater than 10
def filter_func(n):
return n < 5 or n > 10
# using filter function
filtered_numbers = filter(filter_func, numbers)
# converting the result to a list and printing
print(list(filtered_numbers)) # Output: [1, 2, 3, 4, 11, 12, 13, 14, 15]
In this example, the filter_func
function tests if a number is less than 5 or greater than 10, and the filter function uses this to filter the numbers
list.
Combining Filter with Other Functions
The filter function is powerful in its own right, but its true potential shines through when used in combination with other Python functions.
For example, the filter function can be combined with the 'map()'
function to first filter a list and then perform an operation on each of the filtered elements.
Here’s an example of using the filter function combined with the map function:
# our number list
numbers = [1, 2, 3, 4, 5]
# function that squares a number
def square(n):
return n ** 2
# using filter function to get even numbers
even_numbers = filter(lambda n: n % 2 == 0, numbers)
# using map function to square the even numbers
squared_numbers = map(square, even_numbers)
# converting the result to a list and printing
print(list(squared_numbers)) # Output: [4, 16]
Common Pitfalls
In this section, we will identify and walk through some common pitfalls that one may encounter when using Python’s filter function. Understanding these issues will help prevent confusion in the future and enable you to write cleaner, more efficient code.
Filter Function Returns an Object Not a List
One common misstep involves forgetting that the filter function returns a filter object, rather than a list. To use the filtered data in the same way as a list, we need to convert the filter object to a list using the list()
function.
For instance, consider the following example:
numbers = [1, 2, 3, 4, 5, 6]
evens = filter(lambda x: x % 2 == 0, numbers)
print(evens)
Output:
<filter object at 0x7f7f2c0b9e80>
Above, the filter
function is used to filter out even numbers from the input list. However, as you can see from the output, it returns a filter object, not a list of the filtered numbers.
To get a list, you should convert the filter object to a list as follows:
numbers = [1, 2, 3, 4, 5, 6]
evens = list(filter(lambda x: x % 2 == 0, numbers))
print(evens)
Output:
[2, 4, 6]
Filter Function Creates a New List
Another vital point to remember is that the filter function doesn’t modify the original list. Instead, it creates a new list that contains the filtered elements.
For example:
numbers = [1, 2, 3, 4, 5, 6]
evens = list(filter(lambda x: x % 2 == 0, numbers))
print(numbers)
Output:
[1, 2, 3, 4, 5, 6]
In the code block above, even though using the filter
function found the even numbers, it did not modify the original numbers
list. The numbers
list stays the same even after applying the filter function
, confirming that filter
creates a new list while the initial list remains unmodified.
Filter Function Can Only be Iterated Once
Lastly, it’s crucial to know that a filter object can only be iterated once. If you attempt to iterate over it again, it will not return any data.
For example:
numbers = [1, 2, 3, 4, 5, 6]
evens_filter = filter(lambda x: x % 2 == 0, numbers)
for number in evens_filter:
print(number)
for number in evens_filter:
print(number)
Output:
2
4
6
In the code block above, the first loop over evens_filter
prints out the even numbers as expected. However, attempting to loop over evens_filter
a second time yields no output, demonstrating that filter objects can only be iterated through once.
Filter Function Requires a Boolean-Returning Function
A common misconception about the filter function is that it accepts any kind of function. However, it is necessary for the function passed to filter to return a Boolean value – True
or False
for each element in the iterable. If your function does not return a Boolean, the filter function might give unexpected results.
For example:
Code Block:
numbers = [1, 2, 3, 4, 5, 6]
filtered_numbers = list(filter(lambda x: x + 2, numbers))
print(filtered_numbers)
Output:
[1, 2, 3, 4, 5, 6]
In the code block above, x + 2
does not return a Boolean value. As a result, the filter function does not accurately filter the numbers and returns the original list instead.
To correct this, ensure the function you pass to filter returns True
or False
. For instance, if we wanted to filter out numbers less than 3, we could revise the function to lambda x: x < 3
.
Code Block:
numbers = [1, 2, 3, 4, 5, 6]
filtered_numbers = list(filter(lambda x: x < 3, numbers))
print(filtered_numbers)
Output:
[1, 2]
Above, the filter function correctly identifies and returns a list of elements in numbers
that are less than 3 because the lambda function is correctly returning a Boolean value.
Beyond Filter in Python
While the filter function is a powerful tool in Python’s data manipulation toolkit, it is just one among many. Python is equipped with a plethora of functions and libraries designed specifically to aid you in handling data. Functions such as map()
, reduce()
, and lambda
, along with libraries like pandas and NumPy, all play a pivotal role in data manipulation in Python.
Comparing Filter Function with Map Function
To understand the versatility of Python’s data manipulation functions, let’s draw a comparison between the filter function and the map()
function. While the filter function is employed to filter elements from an iterable based on a condition, the map()
function is used to apply a function to all elements of an iterable. Here’s an example to illustrate this:
Function | Description |
---|---|
filter() | Filters elements from an iterable based on a condition. |
map() | Applies a function to all elements of an iterable. |
# our number list
numbers = [1, 2, 3, 4, 5]
# function that squares a number
def square(n):
return n ** 2
# using map function
squared_numbers = map(square, numbers)
# converting the result to a list and printing
print(list(squared_numbers)) # Output: [1, 4, 9, 16, 25]
In this example, the map()
function applies the square
function to each element in the numbers
list, resulting in a new list of squared numbers.
Reduce() and lambda for Data Analysis
Mastering multiple functions for data manipulation in Python is vital for effective data analysis. Each function has its unique strengths and use cases. Knowing when to use which function can significantly enhance your data manipulation skills.
Here’s an example of using other Python functions like reduce()
and lambda
for data manipulation:
from functools import reduce
# our number list
numbers = [1, 2, 3, 4, 5]
# using reduce function to get the product of numbers
product = reduce(lambda x, y: x * y, numbers)
print(product) # Output: 120
By chaining together multiple functions, you can perform complex data manipulation tasks with just a few lines of code. This makes Python a powerful and flexible tool for data manipulation, and mastering its functions is a valuable skill for any data enthusiast.
Further Resources for Python Functions
To discover useful techniques for working with lists and dictionaries in Python, Click Here for Python Built-In Functions Insights.
To further your understanding of Python functions and their wide array of applications, we have assembled some additional resources:
- Finding Maximum Values in Python with max() – Dive into element selection, custom comparisons, and optional default values.
Python sum() Function: Summing Data Elements – Explore Python’s “sum” function for adding up numeric elements in sequences.
Filtering Lists in Python – Learn how to efficiently filter lists in Python using this FavTutor guide.
Upgrad’s Guide on Filter Function in Python explains how to utilize Python’s filter function.
Python’s Official Documentation on Built-In Functions explores Python’s built-in functions.
Concluding Thoughts
Our exploration of Python’s filter function has revealed its immense power and versatility. From its basic usage of filtering elements from an iterable to its advanced applications in complex data manipulation scenarios, the filter function is a testament to Python’s capabilities.
In conclusion, the filter function, akin to a sieve, helps separate the gold nuggets (wanted elements) from the dirt and rocks (unwanted elements). It’s a tool that not only simplifies your Python journey but also makes it more efficient and enjoyable. So, go ahead, start filtering, and unlock the full potential of Python!