Python String Concatenation | Guide (With Examples)
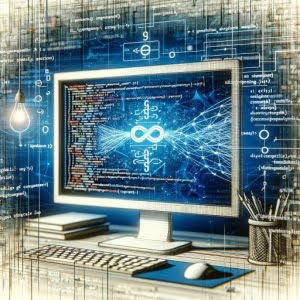
In this article, we will guide you through various methods of string concatenation in Python. From the +
operator to the join
method, to % operator and the format() method, there are many ways to concatenante strings in Python.
We’ll also provide you with practical examples for each method, so you can understand better and start applying these techniques in your own Python programs.
TL;DR: How Do I Concatenate Strings in Python?
The simplest way to concatenate, or join, strings in Python is by using the
'+'
operator and the syntax,str3 = str1 + ' ' + str2
.
Here’s a quick example:
str1 = 'Hello'
str2 = 'World'
result = str1 + ' ' + str2
print(result)
# Output:
# 'Hello World'
In this example, we’ve taken two strings, ‘Hello’ and ‘World’, and joined them together with a space in between using the ‘+’ operator. The result is a new string ‘Hello World’.
Intrigued? Keep reading to learn more about the various methods and best practices for string concatenation in Python, from basic to advanced techniques.
Table of Contents
Basic String Concatenation
For beginners in Python, the simplest and most straightforward method to concatenate strings is by using the ‘+’ operator. This operator takes two strings and combines them into one. Let’s take a look at a basic example:
str1 = 'Python'
str2 = 'Programming'
result = str1 + ' ' + str2
print(result)
# Output:
# 'Python Programming'
In the above code block, we have two strings ‘Python’ and ‘Programming’. We use the ‘+’ operator to join these strings with a space in between. The result is a new string ‘Python Programming’.
While the ‘+’ operator is a simple and intuitive way to concatenate strings, it has its limitations. It can only concatenate strings, not other data types. If you try to concatenate a string with a non-string data type, Python will throw a TypeError. Also, when concatenating a large number of strings, the ‘+’ operator can be less efficient than some other methods.
Advanced String Concatenation
As you learn more about Python, you’ll find that the .join()
method offers a more powerful and flexible way to concatenate strings. This method is particularly useful when you have a list of strings that you want to join into a single string. Here’s how it works:
str_list = ['Python', 'is', 'fun']
result = ' '.join(str_list)
print(result)
# Output:
# 'Python is fun'
In the above example, we have a list of strings ['Python', 'is', 'fun']
. We use the ”.join() method to combine these strings into a single string ‘Python is fun’. The space ‘ ‘ that we pass to the ”.join() method is used as the separator between the strings.
The ”.join() method is more efficient than the ‘+’ operator when concatenating a large number of strings. However, like the ‘+’ operator, it can only concatenate strings, not other data types. If your list contains non-string elements, you’ll need to convert them to strings before using the ”.join() method.
In conclusion, the ”.join() method is an advanced technique for string concatenation in Python that offers greater efficiency and flexibility than the ‘+’ operator.
Exploring Alternative Methods
While the ‘+’ operator and .join()
method are commonly used for string concatenation in Python, there are other methods that offer additional flexibility and functionality. Let’s delve into two of these methods: the %
operator and the format()
method.
Using the ‘%’ Operator for String Concatenation
The ‘%’ operator allows you to embed variables in a string. This method is similar to printf-style formatting in C. Here’s an example:
name = 'John'
age = 30
result = 'My name is %s and I am %d years old.' % (name, age)
print(result)
# Output:
# 'My name is John and I am 30 years old.'
In the above example, we use the ‘%’ operator to replace the placeholders ‘%s’ and ‘%d’ with the variables ‘name’ and ‘age’. The ‘%s’ is a placeholder for a string, and ‘%d’ is a placeholder for a decimal (integer).
String Concatenation Using the format() Method
The format() method is a more modern way to format strings in Python. It’s more readable and versatile than the ‘%’ operator. Here’s how it works:
name = 'John'
age = 30
result = 'My name is {} and I am {} years old.'.format(name, age)
print(result)
# Output:
# 'My name is John and I am 30 years old.'
In the above example, we use the format() method to replace the placeholders ‘{}’ with the variables ‘name’ and ‘age’. The placeholders ‘{}’ are replaced by the arguments in the order they are passed to the format() method.
Both the ‘%’ operator and the format() method offer more flexibility than the ‘+’ operator and ”.join() method, especially when you need to embed variables in a string. However, they can be a bit more complex and may not be necessary for simple string concatenation tasks.
Solving Issues within Concatenation
While concatenating strings in Python is generally straightforward, you may encounter some common issues. Understanding these issues and knowing how to resolve them can save you a lot of time and frustration.
Handling Type Errors
One common issue when concatenating strings in Python is the TypeError. This error occurs when you try to concatenate a string with a non-string type. For example:
str1 = 'Hello'
num = 123
result = str1 + num # This will raise a TypeError
# Output:
# TypeError: can only concatenate str (not "int") to str
In the above example, we try to concatenate a string ‘Hello’ with an integer 123, which raises a TypeError. To resolve this issue, you need to convert the non-string type to a string using the str() function:
str1 = 'Hello'
num = 123
result = str1 + str(num) # Convert the integer to a string
print(result)
# Output:
# 'Hello123'
Now, the integer 123 is converted to a string before concatenation, and the result is a new string ‘Hello123’.
Efficiency Considerations
Another consideration when concatenating strings in Python is efficiency. If you’re concatenating a large number of strings, using the ‘+’ operator can be slow and inefficient. This is because each ‘+’ operation creates a new string and copies the old strings into it, which takes time and memory.
In such cases, the ”.join() method is a more efficient alternative, as it only creates a new string once. However, if you’re only concatenating a few strings, the difference in efficiency between the ‘+’ operator and ”.join() method is negligible.
In conclusion, understanding the potential issues and considerations when concatenating strings in Python can help you write more robust and efficient code.
Concepts of Strings and Concatenation
Before we dive deeper into the intricacies of string concatenation in Python, it’s essential to understand what strings are and why concatenation is a crucial operation.
What are Strings in Python?
In Python, a string is a sequence of characters enclosed in single quotes (”), double quotes (“”), or triple quotes (”’ ”’ or “”” “””). For example:
str1 = 'Hello'
str2 = "World"
str3 = '''Hello World'''
print(str1, str2, str3)
# Output:
# Hello World Hello World
In the above example, str1, str2, and str3 are all strings. They can contain any characters: letters, numbers, symbols, and even spaces.
Why is Concatenation Useful?
Concatenation is the operation of joining two or more strings into one. It’s a fundamental operation in Python and many other programming languages. Concatenation is useful in a wide range of scenarios, from building sentences and paragraphs in text processing to generating dynamic strings in web development.
For instance, if you’re writing a program that greets the user, you might want to concatenate the greeting ‘Hello’ with the user’s name. Here’s a simple example:
greeting = 'Hello'
name = 'John'
message = greeting + ', ' + name + '!' # Concatenate the strings
print(message)
# Output:
# Hello, John!
In the above example, we concatenate the strings ‘Hello’, ‘, ‘, ‘John’, and ‘!’ to create a personalized greeting message for the user.
In conclusion, understanding strings and the operation of concatenation is fundamental to programming in Python. These concepts form the basis for many more advanced topics and techniques in Python, such as string formatting and text processing.
Use Cases of String Concatenation
String concatenation in Python isn’t just about joining a couple of words or sentences. It plays a significant role in larger scripts or projects, especially in areas like data analysis, web scraping, and automation.
For instance, in web scraping, you often need to build dynamic URLs by concatenating a base URL with different paths or parameters. Similarly, in data analysis, you might need to concatenate strings to create labels or titles for your data.
Let’s consider an example where we’re building a dynamic URL for a web scraping task:
base_url = 'https://example.com'
path = 'products'
product_id = '123'
url = base_url + '/' + path + '/' + product_id
print(url)
# Output:
# 'https://example.com/products/123'
In this example, we concatenate the base_url, path, and product_id to create a complete URL for a specific product. This technique can be used to scrape data for different products by just changing the product_id.
Further Resources for Python Strings
To deepen your understanding of string concatenation and its applications in Python, you may want to explore the following topics:
- String formatting: Learn more about the ‘%’ operator and the format() method, and how they can be used to format strings in more complex ways.
Text processing: Discover how string concatenation and other string operations are used in text processing, such as parsing text files or cleaning data.
Regular expressions: Regular expressions are a powerful tool for manipulating strings. They can be used to search, replace, and extract information from strings in complex ways.
Here are some additional resources from our blog:
- Simplifying Text Processing with Python Strings: Learn how to simplify text processing in your Python projects by effectively utilizing string manipulation techniques.
Compare Strings in Python: Methods and Tips: IOFlood’s guide on comparing strings in Python, covering case-insensitive comparison, sorting, and string comparison methods with examples.
Convert String to Float in Python: IOFlood’s tutorial on converting strings to floats in Python, demonstrating techniques using
float()
,ast.literal_eval()
, and custom conversion methods with examples.Python String Concatenation: A Step-By-Step Guide: A comprehensive guide on Real Python explaining different methods of string concatenation in Python.
Python String Concatenation: A brief explanation on w3schools.com about string concatenation in Python using the
+
operator and+=
assignment operator.Python String Concatenation: An Introduction: A tutorial on DigitalOcean introducing string concatenation in Python with examples.
Recap: String Concatenation in Python
In this comprehensive guide, we’ve explored various methods to concatenate strings in Python, a fundamental operation that comes in handy in a wide range of programming scenarios.
We began with the simplest method, the ‘+’ operator, which is intuitive and great for beginners. However, we also discussed its limitations, such as the inability to concatenate non-string types and its inefficiency when dealing with a large number of strings.
Next, we delved into the ”.join() method, a more advanced technique that offers greater efficiency and flexibility, especially when concatenating a list of strings.
We also explored alternative methods like the ‘%’ operator and the format() method, which provide more flexibility and functionality, especially when you need to embed variables in a string.
Finally, we addressed common issues such as TypeErrors and efficiency considerations, providing you with the tools to troubleshoot and write more robust, efficient code.
In conclusion, mastering the art of string concatenation in Python can significantly enhance your coding skills, whether you’re building simple scripts or complex applications. Keep practicing and exploring, and you’ll be a Python string concatenation expert in no time!