Python
20 Aug 2024
np.where Python Function | Quick Tips for Numpy
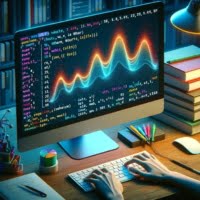
When programming array-based operations into IOFLOOD scripts, the np where function in Python’s NumPy library enables us to perform element-wise comparisons and easily generate boolean masks. This guide will cover exactly how to use np.where and offer code samples to help our customers leverage NumPy in their bare metal hosting projects. This guide will walk
20 Aug 2024
Python Sleep Methods | How to Make Code Pause or Wait
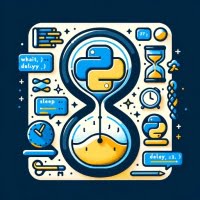
Making Python sleep or wait during execution is a common need that comes up when programming at IOFLOOD. Today’s article will discuss various method, to assist our customers in delaying actions or simulating real-time processes in scripts hosted on their dedicated remote servers. In this guide, we’ll walk you through implementing delays in Python time,
19 Aug 2024
Pip Install Requirements.txt | Python Package Manager
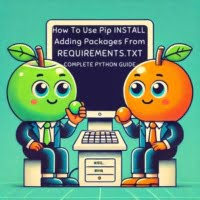
Using pip to install packages from a requirements.txt file is a streamlined approach we follow at IOFLOOD for managing Python dependencies in our projects. To share our process on how to use pip to install packages from a requirements.txt file to assist with simplifying Python dependency management on dedicated cloud hosting platforms. This comprehensive guide
19 Aug 2024
Python String Replace Methods | Using replace() and More
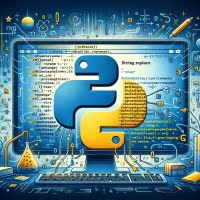
Replacing substrings in Python is a fundamental operation for text manipulation and data cleaning at IOFLOOD. This operation allows for the modification and updating of string content efficiently. Today’s article has been made with to assist our customers running Python scripts on bare metal servers, as well as other developers! We will walk you through
19 Aug 2024
Using train_test_split in Sklearn: A Complete Tutorial
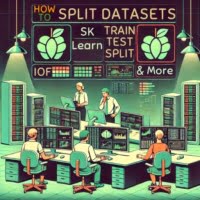
When managing data for machine learning projects on Linux servers at IOFLOOD, correctly splitting datasets is essential for ensuring model performance. We’ve observed that Scikit-Learn’s train_test_split function provides an effective way to create training and testing subsets, allowing us to fine-tune our algorithms. By sharing our best practices, we aim to help our customers optimize
12 Aug 2024
Python Sort Dictionary by Value | Handling Data Structures
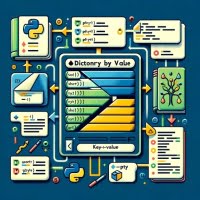
Sorting a list of dictionaries by value in Python is a common task we perform to organize complex data structures at IOFLOOD. Today’s article will explore how to sort dict by value in Python, providing practical examples to assist our bare metal hosting customers in managing their data more effectively. This guide will show you
12 Aug 2024
Python String Comparison Methods | Quick User Guide
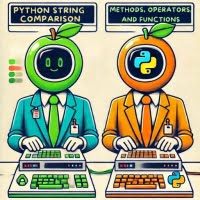
Python String comparison is a fundamental technique we frequently utilize at IOFLOOD to perform operations such as sorting, matching, and validating strings based on their values. Today’s article will explain how to compare strings in Python, providing practical examples to assist our customers utilizing their cloud server hosting services for Python Programming. This guide will
12 Aug 2024
PIP Updates Packages in Python | Practical Commands
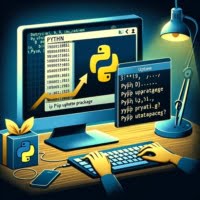
While managing the Python development environments we host at IOFLOOD, updating Python packages is a routine yet crucial task we perform. Today’s article will explain the commands we use to pip update packages and maintain dedicated development server environments for our customers. This comprehensive guide will navigate you through the process of updating a Python
22 Jul 2024
New Line Python | Uses, Printing and Formatting Tutorial
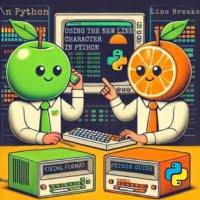
Implementing a Python new line comes up often when formatting text output in our scripts at IOFLOOD. The newline character represented by \n in Python is the most consistent way to code line breaks and ensure our data is displayed clearly and readably. In today’s article, we will explain the concept of the new line
08 Jul 2024
Python Modules | Effective Use of Python Built-in Modules
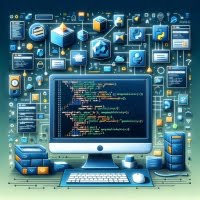
Understanding Python modules is crucial for efficient code organization and reuse at IOFLOOD. Modules enable us to structure our Python scripts into manageable sections, promoting modular programming practices. This article delves into what Python modules are, providing insights and examples to help our dedicated server customers improve their code maintainability. This guide will walk you