“New Line” Python Guide: Uses and Examples
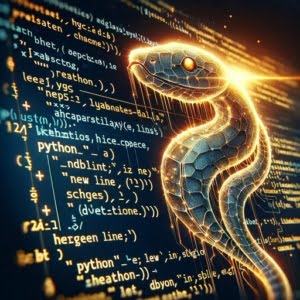
Have you ever found yourself navigating an ocean of code, pondering over the mystique behind certain characters? Among these, the newline character in Python often piques interest. It’s a deceptively simple character, composed of just a backslash and an ‘n’.
But its simplicity belies the power it wields, and it can be a game-changer for any programmer. Think of it as the ‘Enter’ key of the programming world, a tool that helps you break your code into readable chunks.
In this blog post, we’re going to unravel the usage of the newline character in Python. We’ll explore its applications, and delve into the subtleties that make it a versatile tool in a programmer’s arsenal. So, if you’re ready to harness the power of the newline character, let’s get started!
TL;DR: What is the newline character in Python?
The newline character in Python is a special character that helps in formatting your code. Represented as ‘\n’, it’s like the ‘Enter’ key in programming, breaking code into readable chunks.
Newline is a simple tool, but understanding its functionality can have a significant impact on code readability and organization. Consider this simple Python code:
print('Hello\nWorld')
This code will print ‘Hello’ on one line and ‘World’ on the next line.
For more advanced methods, background, tips and tricks on using the newline character effectively, continue reading the article.
Table of Contents
Basics of the Newline Character
In the world of Python programming, the newline character holds a special place. It’s defined as an escape sequence, a combination of characters that carries a unique meaning.
Here are some common escape sequences in Python:
Escape Sequence | Meaning |
---|---|
\\ | Backslash (\) |
\\’ | Single quote (\’) |
\” | Double quote (\”) |
\n | Newline |
\t | Tab |
Represented as ‘\n’, the newline character might appear simple, but its functionality can profoundly impact the readability of your code.
How Does the Newline Character Work?
The newline character operates in a straightforward manner in Python. When the Python interpreter encounters a newline character within a string, it interprets it as an instruction to break the line at that point. Consequently, any text following the newline character is displayed on a new line.
For example, without using the newline character:
print('Hello World')
This will print ‘Hello World’ on one line.
Now, let’s use the newline character:
print('Hello\nWorld')
Output:
# Hello
# World
This will print ‘Hello’ on one line and ‘World’ on the next line.
The Role of the Newline Character
The newline character is instrumental when working with string objects and managing text output in Python. It allows you to neatly format your output, enhancing the readability of your code.
For instance, you can employ the newline character to split a lengthy string into multiple lines or to separate different sections of your output.
Decoding Escape Sequences
The newline character is one among many escape sequences available in Python. Escape sequences are employed to insert special characters into strings. For instance, ‘\t’ is used to insert a tab, and ‘\\’ is used to insert a backslash.
In essence, the newline character, though seemingly simple, is a potent tool that can dramatically improve the readability of your code. Therefore, the next time you’re crafting a Python script, don’t forget the humble ‘\n’ and the significant difference it can make to your code.
Customizing the Print Function
Python’s flexibility is one of its most powerful features. For instance, the print function’s default behavior can be customized to suit your needs.
By default, the print function is like a dutiful scribe that automatically adds a newline character at the end of the output, starting a new line every time you call the print function.
However, you can change this behavior by specifying a different character for the ‘end’ parameter of the print function.
For example, you can use a space instead of a newline character to keep the output on the same line:
print('Hello,', end=' ')
print('world!')
Output:
#Hello world!
When you run this code, Python will print ‘Hello, world!’ on the same line, instead of breaking it into two lines. This gives you even more control over the layout of your output, allowing you to create more complex and flexible output formats.
More Examples of Python Newlines
Let’s delve into some practical examples to understand the newline character’s usage in Python better.
Newline Character in an Iterator
The newline character can also be utilized in an iterator. Here’s an example:
for i in ['Hello', 'World']:
print(i, end='\n')
Running this code will print each item in the list on a new line. The ‘end’ parameter of the print function is set to the newline character ‘\n’, directing Python to start a new line after each item.
Printing a String vs Processing a String in Python
Understanding the distinction between printing a string and processing a string in Python is crucial. When you print a string, Python interprets the escape sequences in the string and formats the output accordingly.
However, when you process a string (for example, when you assign it to a variable or pass it to a function), Python treats the escape sequences as literal characters.
Consider the following code for illustration:
s = 'Hello\nWorld'
print(s)
Executing this code will print ‘Hello’ on one line and ‘World’ on the next line. This is because the print function interprets the newline character in the string and starts a new line.
However, if you were to process the string differently (for example, by counting its characters), Python would count the newline character as two characters (‘\’ and ‘n’), not as a single newline character.
For example:
s = 'Hello\nWorld'
print(len(s))
This will print ’12’, because Python counts the newline character as two characters.
Multiline Strings in Python
In Python, multiline strings can also be used to insert new lines between strings. Multiline strings are denoted by triple quotes ("""
or '''
). When you use triple quotes, Python allows the string to span across multiple lines, automatically inserting a newline character at the end of each line.
Consider the following code for illustration:
print("""Hello
World""")
Executing this code will print ‘Hello’ on one line and ‘World’ on the next line. This is because the triple quotes allow the string to span across multiple lines, and Python automatically inserts a newline character at the end of each line.
Python’s os Package and os.linesep
Python’s os package provides a platform-independent line breaker, os.linesep, which can be used for separating lines.
This becomes especially useful when working with files, as different operating systems use different newline characters.
It’s important to be aware that os.linesep is not recommended for files opened in text mode. This is because Python automatically converts ‘\n’ to the correct newline character for the platform in text mode. Using os.linesep in this context can lead to unexpected results.
Understanding the Nuances of os.linesep in Text Mode
Why is os.linesep not recommended for files opened in text mode? When Python opens a file in text mode, it performs universal newline translation. This means that it automatically converts all newline characters to ‘\n’, regardless of the platform.
While this is usually a helpful feature, it can cause issues if you’re using os.linesep. This is because os.linesep represents the newline character for the current platform, which might not be ‘\n’.
Using os.linesep in text mode can result in double line breaks or other unexpected behavior.
For example:
import os
with open('test.txt', 'w') as f:
f.write('Hello' + os.linesep + 'World')
with open('test.txt', 'r') as f:
print(f.read())
This might print the following on windows:
Hello\nWorld
Because os.linesep is ‘\r\n’ on Windows, and Python automatically converts ‘\r\n’ to ‘\n’ in text mode.
Using REPL and Newline Characters
Python’s REPL (Read-Eval-Print Loop) is an interactive shell that reads a statement, evaluates it, and then prints the result.
When working with the REPL, the newline character can be used to create multi-line statements. This is handy for writing complex code or testing snippets before adding them to your script.
Further Resources for Python Strings
If you’re interested in learning more about Python string handling, here are a few resources that you might find helpful:
- The Power of Python Strings: Unleashing Advanced Capabilities: Unleash the advanced capabilities of Python strings with this guide, exploring deeper functionalities and techniques for professional-level text manipulation.
Python Match Case Statement: New Pattern Matching Syntax: Introduction to the new match case statement in Python, providing examples and explanations of its syntax and applications.
Python String Formatting: A Comprehensive Guide: Comprehensive guide on different methods of formatting strings in Python, including f-strings and format() method.
IOFlood’s Python Syntax Guide: An extensive guide and cheat sheet on Python syntax, covering variables, data types, control flow, functions, and more.
Python New Line: A Comprehensive Guide: A comprehensive guide that explains how to use new lines in Python, including line breaks in strings, printing with and without newline characters, and other related concepts.
Python New Line and How to Print Without a Newline: An article on FreeCodeCamp that covers various ways to handle new lines in Python, including printing with or without newline characters using the print() function.
Print Newline in Python: A blog post that demonstrates different techniques to print newline characters in Python, providing examples and explanations.
Final Thoughts
In conclusion, the newline character is a subtle yet powerful tool when it comes to managing strings and functions in Python. Understanding its nuances and how to use it effectively can greatly enhance your coding skills and productivity.
The newline character is instrumental in managing Python strings, controlling output flow, and enhancing code readability and output formatting. Its omnipresence in Python functions and automatic addition in Python print statements underline its broad applications. We’ve also navigated through practical examples, showcasing how the newline character can be leveraged in various scenarios to augment the structure and readability of your code.
In conclusion, the newline character in Python, though seemingly simple, is a potent tool that every Python programmer should master. So, remember to harness the power of the humble ‘\n’ the next time you’re crafting a Python script. It’s a small addition to your code that can make a big difference.