Bash Multi-Line Strings: Methods and Best Practices
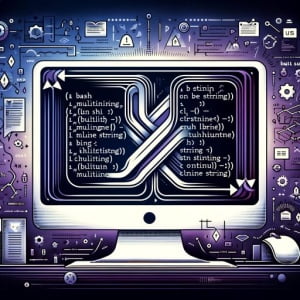
Are you finding it challenging to work with multiline strings in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling multiline strings in Bash. But think of Bash as a skilled poet, capable of handling verses that span multiple lines, making it a versatile and handy tool for various tasks.
This guide will walk you through the ins and outs of working with multiline strings in Bash, from the basics to more advanced techniques. We’ll cover everything from creating multiline strings using Here Documents and Here Strings, to dealing with common issues and even troubleshooting them.
So, let’s dive in and start mastering multiline strings in Bash!
TL;DR: How Do I Create a Multiline String in Bash?
In Bash, you can create a multiline string using a
Here Document
orHere String
. These are special types of redirections that allow you to create multiline strings easily and efficiently.
Here’s a simple example using a Here Document:
cat << EOF
This is a
multiline string
EOF
# Output:
# This is a
# multiline string
In this example, we use the cat
command in conjunction with a Here Document (cat << EOF
). This is just a basic way to create multiline strings in Bash. But there’s much more to learn about handling multiline strings in Bash, including advanced techniques and alternative approaches. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Bash Multiline String Basics: Here Documents and Here Strings
- Advanced Bash Multiline String Techniques
- Exploring Alternative Methods for Bash Multiline Strings
- Troubleshooting Bash Multiline Strings: Common Issues and Solutions
- Understanding Bash’s String Handling Capabilities
- Beyond Multiline Strings: Expanding Your Bash Knowledge
- Wrapping Up: Mastering Bash Multiline Strings
Bash Multiline String Basics: Here Documents and Here Strings
Bash provides two powerful features for creating multiline strings: Here Documents and Here Strings. Let’s dive into each of these and understand how they work, their advantages, and potential pitfalls.
Here Documents
A Here Document is a type of redirection that allows you to create multiline strings. It uses a form of I/O redirection to feed a command list to an interactive program or command, such as ftp, cat, or the ex text editor.
Here’s an example of how to create a multiline string using a Here Document:
cat << EndOfText
This is line 1.
This is line 2.
This is line 3.
EndOfText
# Output:
# This is line 1.
# This is line 2.
# This is line 3.
In this example, EndOfText
is a delimiter that marks the beginning and end of the text block. The cat
command then processes this text block as its standard input.
Here Strings
Here Strings are another way to create multiline strings in Bash. They are similar to Here Documents but are used with a single line of input. They are useful when you want to read multiline input into a variable or pass multiline input to a command.
Here’s an example of a Here String:
read -d '\n' multilinestring << EndOfText
Line 1
Line 2
Line 3
EndOfText
echo "$multilinestring"
# Output:
# Line 1
# Line 2
# Line 3
In this example, we use the read
command with the -d
option (which changes the delimiter from a newline to a null character) and a Here String to read multiple lines of input into a variable. The echo
command then prints the multiline string.
While Here Documents and Here Strings are powerful, they have some potential pitfalls. For example, if you don’t quote the delimiter (EndOfText
in our examples), Bash will perform parameter expansion, command substitution, and arithmetic expansion. To avoid this, you can quote the delimiter as shown:
cat << 'EndOfText'
$HOME
$(date)
EndOfText
# Output:
# $HOME
# $(date)
In this example, $HOME
and $(date)
are not expanded and are treated as literal strings. This can be useful when you want to preserve special characters in your multiline strings.
Advanced Bash Multiline String Techniques
As you become more comfortable with Bash, you’ll find that multiline strings can do much more than just store text. They can also incorporate variables and command substitutions, making them a powerful tool for generating complex strings and scripts.
Incorporating Variables
You can easily include variables in your multiline strings. When the shell encounters a dollar-sign ($), it interprets the following text as a variable name and replaces it with the variable’s value.
Here’s an example of how to incorporate a variable in a multiline string:
name="Alice"
cat << EOF
Hello, $name
Welcome to our Bash tutorial!
EOF
# Output:
# Hello, Alice
# Welcome to our Bash tutorial!
In this example, we first define a variable name
with the value Alice
. We then use this variable in our multiline string. The $name
in the multiline string is replaced by Alice
, which is the value of the name
variable.
Command Substitution
Command substitution allows you to execute a command and substitute its output in place. This is done by wrapping the command with $( )
.
Here’s an example of command substitution in a multiline string:
cat << EOF
Today's date is: $(date)
EOF
# Output:
# Today's date is: Mon Sep 27 12:34:56 PDT 2021
In this example, the $(date)
command is replaced by the current date and time. This demonstrates how you can dynamically generate parts of your multiline string.
Using variables and command substitutions in your multiline strings allows you to create more complex and dynamic strings. However, remember to be mindful of potential issues with whitespace and special characters, which we’ll cover in the next section.
Exploring Alternative Methods for Bash Multiline Strings
While Here Documents and Here Strings are commonly used to handle multiline strings in Bash, there are alternative methods that can be just as effective, depending on your specific needs. Let’s explore some of these alternatives, their advantages, and potential drawbacks.
Using Printf Function
The printf
function is a powerful tool that can also be used to create multiline strings. It provides more control over the formatting of the output than the echo
command.
Here’s an example of how to create a multiline string using printf
:
printf "Line 1 \n
Line 2 \n
Line 3 \n"
# Output:
# Line 1
# Line 2
# Line 3
In this example, we use printf
and newline characters (\n
) to create a multiline string. The advantage of printf
is its flexibility in controlling the output format, but it can be more complex to use than Here Documents or Here Strings.
Combining Echo Statements
Another alternative to create multiline strings in Bash is by combining multiple echo
statements. This can be a simpler method when dealing with shorter strings.
Here’s an example of how to create a multiline string by combining echo
statements:
echo -e "Line 1 \n
Line 2 \n
Line 3"
# Output:
# Line 1
# Line 2
# Line 3
In this example, we use the -e
option with echo
to interpret backslash escapes, allowing us to include newline characters (\n
) in the string. This method is simple and straightforward, but can become unwieldy with larger strings.
Choosing the right method to handle multiline strings in Bash depends on your specific requirements and the complexity of the strings. Here Documents and Here Strings are powerful and flexible, but printf
and combined echo
statements can also be effective in certain scenarios. It’s important to understand the advantages and potential pitfalls of each method to make an informed choice.
Troubleshooting Bash Multiline Strings: Common Issues and Solutions
Working with multiline strings in Bash can sometimes lead to unexpected results. Issues can arise due to whitespace, special characters, or variable substitution. Let’s discuss these common problems and how to solve them.
Dealing with Whitespace
In Bash, leading whitespace in a Here Document or Here String can cause unexpected results. Bash does not strip leading tabs or spaces, which means they become part of the string.
Consider the following example:
cat << EOF
This is a
multiline string
EOF
# Output:
# This is a
# multiline string
In this example, the leading spaces are preserved in the output. If this is not the desired behavior, you can use the -
option with the Here Document to strip leading tabs (but not spaces):
cat <<- EOF
This is a
multiline string
EOF
# Output:
# This is a
# multiline string
Handling Special Characters
Special characters, such as $
, \
, and ““ , can cause issues in multiline strings because Bash treats them as part of its syntax. To prevent Bash from interpreting these characters, you can quote the Here Document delimiter:
cat << 'EOF'
This is a $string with special characters: \ `command`
EOF
# Output:
# This is a $string with special characters: \ `command`
In this example, the special characters are preserved in the output because we quoted the EOF
delimiter.
Variable Substitution Pitfalls
When using variables in multiline strings, remember that Bash performs variable substitution when it sees a dollar-sign ($). If you want to include a literal dollar-sign, you need to escape it with a backslash (\$
).
Here’s an example:
price=20
cat << EOF
The price is $${price}.
EOF
# Output:
# The price is $20.
In this example, we escape the dollar-sign that we want to include in the output, and Bash substitutes ${price}
with its value.
Understanding these common issues and how to handle them can help you avoid unexpected results when working with multiline strings in Bash.
Understanding Bash’s String Handling Capabilities
Bash, or the Bourne Again Shell, is a powerful command-line interface used in many Linux distributions. It’s renowned for its ability to handle strings, or sequences of characters, which are a fundamental data type in Bash scripting.
Bash and Multiline Strings: The Basics
A basic string in Bash is typically defined using double or single quotes, like so:
string="This is a string"
echo $string
# Output:
# This is a string
However, when it comes to multiline strings, the process is a bit more involved. Multiline strings are strings that span across multiple lines. Bash does not inherently support multiline strings like some other programming languages, but it provides features such as Here Documents and Here Strings to handle multiline strings effectively.
Here Documents: A Closer Look
A Here Document is a type of redirection that allows the creation of multiline strings. It uses a form of I/O redirection to feed a command list to an interactive program or command. In essence, it’s a way of embedding a block of input text within a script.
Here’s a different example of a Here Document:
read -r -d '' var << EndOfText
Hello,
This is a multiline string.
EndOfText
echo "$var"
# Output:
# Hello,
# This is a multiline string.
In this example, we use the read
command with the -r
option (to prevent backslash escapes from being interpreted) and the -d
option (to change the delimiter from a newline to a null character) to read the Here Document into a variable.
Here Strings: A Deeper Dive
Here Strings, on the other hand, are a type of redirection that allow you to pass a word or string directly to a command’s standard input, similar to a Here Document, but they are used with a single line of input.
Here’s a unique example of a Here String:
cut -d ' ' -f 1 <<< "This is a Here String"
# Output:
# This
In this example, we use a Here String to pass a string to the cut
command, which then extracts the first word of the string.
Understanding the fundamentals of Bash’s string handling capabilities, and the concepts underlying Here Documents and Here Strings, is crucial when working with multiline strings in Bash.
Beyond Multiline Strings: Expanding Your Bash Knowledge
Multiline strings in Bash are not just a standalone concept. They play a significant role in larger Bash scripts and projects. Understanding and mastering them can significantly enhance your Bash scripting skills.
Relevance in Larger Bash Scripts
In more extensive Bash projects, multiline strings can be utilized to create complex scripts, store large amounts of data, or even generate other scripts. They can be used to create SQL queries, generate HTML or XML files, or create configuration files, among other uses. The ability to include variables and command substitutions within these strings adds another layer of dynamic functionality.
Exploring Related Concepts
Once you’re comfortable with multiline strings, there are related concepts in Bash that are worth exploring. String manipulation, for example, allows you to modify strings in various ways, such as extracting substrings, replacing substrings, or changing the case of strings.
Regular expressions, another powerful feature in Bash, can be used to match and manipulate strings based on patterns. They can be particularly useful when working with large amounts of text or when you need to parse complex data.
Further Resources for Bash Mastery
To deepen your understanding of Bash and its features, here are some resources that provide in-depth tutorials and guides:
- GNU Bash Manual – This is the official manual for Bash, providing a comprehensive overview of the shell’s features.
Advanced Bash-Scripting Guide – This guide covers Bash scripting in detail, including advanced topics like string manipulation and regular expressions.
Bash Academy – Bash Academy offers interactive lessons on various Bash concepts, including scripting, string handling, and regular expressions.
By understanding multiline strings and related concepts in Bash, you’ll be well-equipped to write efficient, robust Bash scripts for a wide variety of tasks.
Wrapping Up: Mastering Bash Multiline Strings
In this comprehensive guide, we’ve delved into the intricacies of handling multiline strings in Bash, a fundamental yet powerful aspect of Bash scripting.
We began with the basics, learning how to create multiline strings using Here Documents and Here Strings. We then ventured into more advanced territory, exploring how to incorporate variables and command substitutions within these strings, thereby adding a layer of dynamic functionality to our scripts.
We also tackled common challenges you might face when working with multiline strings in Bash, such as issues with whitespace, special characters, and variable substitution. For each challenge, we provided solutions and workarounds to help you overcome these hurdles and continue scripting efficiently.
Moreover, we didn’t limit ourselves to just Here Documents and Here Strings. We also looked at alternative approaches for handling multiline strings, such as the printf
function and combining echo
statements. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Here Documents | Powerful, flexible | Can be complex for beginners |
Here Strings | Simple for single lines of input | Limited to single lines |
Printf Function | More control over output format | More complex to use |
Combining Echo Statements | Simple for short strings | Can be unwieldy for larger strings |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of Bash multiline strings and how to handle them effectively.
With the knowledge you’ve gained, you’re now well-equipped to tackle any challenges that come your way when dealing with multiline strings in Bash. Happy scripting!