Understanding the Java ‘…’ Parameter: Varargs Explained
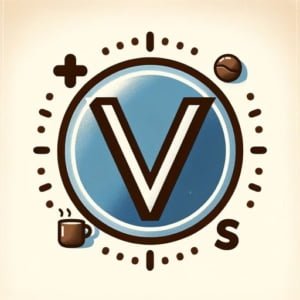
Ever found yourself puzzled by the mysterious ‘…’ parameter in Java? You’re not alone. Many developers find themselves scratching their heads when they first encounter this peculiar syntax.
Think of Java’s ‘…’ parameter, also known as varargs, as a Swiss Army knife – a versatile tool that can handle an arbitrary number of parameters in a method. It’s a powerful feature that can make your code more flexible and easier to read.
In this guide, we’ll demystify the ‘…’ parameter in Java, showing you how to use varargs effectively in your code. We’ll cover everything from the basics of using varargs to more advanced techniques, as well as alternative approaches and common pitfalls to avoid.
So, let’s dive in and start mastering varargs in Java!
TL;DR: What is the ‘…’ Parameter in Java?
The
...
parameter in Java is known as varargs (variable arguments). It allows a method to accept zero or more arguments of the same type, with the syntax:methodName(dataType... inputData){}
. Here’s a simple example:
void printLetters(char... letters) {
for (char letter : letters) {
System.out.println(letter);
}
}
# Output:
# If you call printNumbers('a', 'b', 'c'), the output will be:
# a
# b
# c
In this example, the printLetters
method is declared with varargs of type char
. When we call this method with three characters (a, b, c), it prints each letter on a separate line.
This is just the tip of the iceberg when it comes to understanding and using the ‘…’ parameter, or varargs, in Java. Continue reading for a more detailed explanation, advanced usage scenarios, and potential pitfalls to avoid.
Table of Contents
Varargs in Java: The Basics
Varargs is a shorthand for ‘variable arguments’, and in Java, it’s represented by the three-dot (‘…’) syntax. It allows you to pass an arbitrary number of arguments of the same type to a method.
Declaring a Method with Varargs
Let’s start by declaring a method that uses varargs. In this example, we’ll create a method that can accept any number of int
arguments and print them out:
void printNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
In the printNumbers
method, int... numbers
means that this method can accept zero or more int
arguments. The arguments are treated as an array within the method.
Calling a Method with Varargs
You can call a method with varargs just like any other method. Here’s how you can call the printNumbers
method with three integers:
printNumbers(1, 2, 3);
# Output:
# 1
# 2
# 3
The printNumbers
method prints each number on a separate line. You can pass any number of integers to this method, or even no integers at all.
Advantages and Pitfalls of Using Varargs
Varargs can make your code more flexible and easier to read, as you don’t have to declare an array or a collection to pass multiple arguments of the same type to a method. However, there are some potential pitfalls to be aware of.
One of the main pitfalls is that a method can only have one varargs parameter, and it must be the last parameter. This is because the Java compiler wouldn’t be able to tell where the varargs parameter ends and where the next parameter begins otherwise.
Stay tuned for more advanced uses of varargs and alternative approaches.
Advanced Use of Varargs in Java
Now that we’ve covered the basics, let’s delve deeper into more complex uses of varargs in Java. Specifically, we’ll look at how to use varargs with other method parameters and how to handle varargs of different types.
Varargs with Other Parameters
Although a method can only have one varargs parameter, it can also have other types of parameters. However, the varargs parameter must be the last one. Here’s an example:
void printMessageAndNumbers(String message, int... numbers) {
System.out.println(message);
for (int number : numbers) {
System.out.println(number);
}
}
printMessageAndNumbers("The numbers are:", 1, 2, 3);
# Output:
# The numbers are:
# 1
# 2
# 3
In this example, the printMessageAndNumbers
method has a String
parameter and a varargs parameter of type int
. When we call this method with a string and three integers, it first prints the string, then prints each number on a separate line.
Varargs of Different Types
In Java, you can have varargs of any type. This includes primitive types, classes, and even generics. However, remember that all arguments passed to the varargs parameter must be of the same type or a subtype of the declared type.
Here’s an example of a method that accepts varargs of type Object
, which allows you to pass arguments of different types:
void printObjects(Object... objects) {
for (Object object : objects) {
System.out.println(object);
}
}
printObjects("Hello", 123, true);
# Output:
# Hello
# 123
# true
In this example, the printObjects
method can accept any number of arguments of any type, as all types in Java are subtypes of Object
. When we call this method with a string, an integer, and a boolean, it prints each object on a separate line.
By understanding these advanced uses of varargs, you can harness their full power and flexibility in your Java code.
Alternatives to Varargs: Arrays and Collections
While varargs are a powerful tool in Java, they’re not always the best solution. Depending on your specific needs, using arrays or collections may be more appropriate. Let’s explore these alternatives and their respective advantages and disadvantages.
Using Arrays
Before varargs were introduced in Java 5, developers typically used arrays to pass an arbitrary number of arguments to a method. Here’s how you can rewrite the printNumbers
method using an array:
void printNumbers(int[] numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
int[] numbers = {1, 2, 3};
printNumbers(numbers);
# Output:
# 1
# 2
# 3
In this example, you have to create an array and pass it to the printNumbers
method. This approach is more verbose than using varargs, but it may be preferable if you need to work with the array before or after calling the method.
Using Collections
Another alternative to varargs is using collections, such as List
or Set
. Collections offer more flexibility and functionality than both arrays and varargs, but they also have their own trade-offs.
Here’s how you can rewrite the printNumbers
method using a List
:
void printNumbers(List<Integer> numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
List<Integer> numbers = Arrays.asList(1, 2, 3);
printNumbers(numbers);
# Output:
# 1
# 2
# 3
In this example, you have to create a List
and pass it to the printNumbers
method. This approach is even more verbose than using an array, but it gives you access to the powerful features of the Java Collections Framework.
In conclusion, while varargs are a convenient way to pass an arbitrary number of arguments to a method, they’re not always the best tool for the job. Depending on your specific needs, using arrays or collections may be more appropriate.
Troubleshooting Varargs: Common Issues and Solutions
Like any feature in Java, varargs come with their own set of potential issues and considerations. Let’s discuss some common problems you might encounter when using varargs, along with solutions and workarounds.
Type Errors with Varargs
One common issue when using varargs is type errors. Remember, all arguments passed to the varargs parameter must be of the same type or a subtype of the declared type. If you try to pass arguments of a different type, you’ll get a compile-time error.
For example, the following code will cause a compile-time error because we’re trying to pass a string to a varargs parameter of type int
:
void printNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
printNumbers(1, "two", 3);
# Output:
# Error: incompatible types: String cannot be converted to int
The solution is to ensure that all arguments passed to the varargs parameter are of the correct type.
Performance Considerations with Varargs
Another consideration when using varargs is performance. When you call a method with varargs, an array is created to hold the arguments. This can lead to performance issues if you’re calling the method in a tight loop or with a large number of arguments.
If performance is a concern, you might want to consider using an array or a collection instead of varargs. This allows you to reuse the same array or collection, avoiding the overhead of creating a new one for each method call.
In conclusion, while varargs are a powerful and flexible feature in Java, they come with their own set of potential issues and considerations. By understanding these, you can use varargs effectively and avoid common pitfalls.
Unpacking the Concept of Varargs
Varargs, short for variable arguments, is a feature in Java that allows you to pass an arbitrary number of arguments to a method. It’s represented by the three-dot (‘…’) syntax in the method declaration.
The Rationale Behind Varargs
Before varargs were introduced in Java 5, developers had to use arrays or collections to pass multiple arguments of the same type to a method. This was often cumbersome and made the code harder to read.
Varargs were introduced to provide a more flexible and readable way to pass multiple arguments to a method. With varargs, you can pass any number of arguments (including zero) to a method without having to create an array or a collection.
Varargs and the Java Type System
In Java, varargs are treated as an array of the declared type. This means that inside the method, you can handle the varargs parameter just like any other array.
Here’s a simple example of a method that uses varargs:
void printNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
printNumbers(1, 2, 3);
# Output:
# 1
# 2
# 3
In this example, int... numbers
is a varargs parameter of type int
. Inside the printNumbers
method, numbers
is treated as an array of int
. When we call printNumbers(1, 2, 3)
, it prints each number on a separate line.
In conclusion, varargs are a powerful feature in Java that allow you to write more flexible and readable code. However, like any feature, they should be used judiciously and with an understanding of their potential pitfalls.
Varargs: Beyond the Basics
Varargs, while seemingly simple, play a significant role in larger Java projects and certain design patterns. Their ability to handle an arbitrary number of parameters makes them indispensable in scenarios where flexibility and adaptability are paramount.
Varargs in Larger Java Projects
In large-scale Java projects, varargs prove to be a powerful tool. They allow methods to be more dynamic, able to handle varying numbers of arguments without the need for method overloading or complex array manipulations. This leads to cleaner and more maintainable code.
Varargs and Design Patterns
Varargs are also prevalent in certain design patterns. For instance, in the Builder pattern, which is often used to construct complex objects, varargs can be used to set multiple optional parameters. This makes the code more readable and user-friendly.
Exploring Related Topics
To fully grasp the power of varargs, it’s beneficial to explore related topics such as generics and method overloading in Java. Generics provide type safety for collections, allowing for code reusability. On the other hand, method overloading allows multiple methods to share the same name but with different parameters, increasing code readability.
Further Resources for Varargs
For those interested in diving deeper into varargs and related topics, here are a few resources:
- IOFlood’s article on Java Methods provides insights into Java methods and proficient programming.
Java Method Parameters Basics – Understand the role of parameters in Java methods.
Java Method Calling Guide – Learn the various methods of calling functions in Java.
Oracle’s Oracle’s Java Tutorials cover basic to advanced varargs topics in Java.
Baeldung’s Guide to Java Varargs discusses usage, benefits, and potential pitfalls of varargs.
GeeksforGeeks Java Programming Language offers articles on varargs, generics, and method overloading in Java.
Wrapping Up: Varargs
In this comprehensive guide, we’ve delved into the world of varargs in Java, demystifying the enigmatic ‘…’ parameter.
We started with the basics, learning how to declare and call methods with varargs. We then moved onto more advanced concepts, exploring how to use varargs with other parameters and of different types. Along the way, we tackled common issues you might encounter when using varargs, such as type errors and performance considerations, providing solutions and workarounds for each issue.
We also looked at alternative approaches to handling multiple parameters in Java, comparing varargs with arrays and collections. Here’s a quick comparison of these methods:
Method | Flexibility | Verbosity | Performance |
---|---|---|---|
Varargs | High | Low | Moderate |
Arrays | Moderate | Moderate | High |
Collections | High | High | High |
Whether you’re a beginner just starting out with Java or a seasoned developer looking to deepen your understanding of the language, we hope this guide has shed light on the use of the ‘…’ parameter, or varargs, in Java.
With its balance of flexibility, simplicity, and performance, varargs is a powerful tool in your Java toolkit. Happy coding!