Understanding Parameters in Java: A Detailed Guide
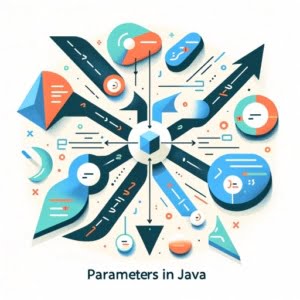
Ever felt like you’re wrestling with understanding parameters in Java? You’re not alone. Many developers find parameters in Java a bit puzzling. Think of parameters as the ingredients in a recipe – they’re the data that methods and constructors need to perform their tasks.
Parameters in Java are a powerful way to extend the functionality of your methods and constructors, making them extremely popular for creating dynamic and reusable code.
In this guide, we’ll walk you through the process of using parameters in Java, from the basics to more advanced techniques. We’ll cover everything from declaring and using parameters, handling different types of parameters (formal, actual, optional), to dealing with common issues and even troubleshooting.
Let’s kick things off and learn to use parameters in Java!
TL;DR: What Are Parameters in Java?
Parameters in Java are variables that are passed into methods or constructors, such as the
name
parameter in,public void greet(String name)
. They are the data that methods and constructors need to perform their tasks. Here’s a simple example:
public void greet(String name) {
System.out.println('Hello, ' + name);
}
// Output:
// 'Hello, John'
In this example, ‘name’ is a parameter. The method ‘greet’ takes one parameter of type String. When we call this method and pass it the string ‘John’, it prints out ‘Hello, John’.
This is a basic way to use parameters in Java, but there’s much more to learn about declaring, using, and understanding different types of parameters in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Grasping Parameters in Java: A Beginner’s Guide
- Diving Deeper: Types of Parameters in Java
- Exploring Overloading: A Powerful Use of Parameters in Java
- Java Parameters: Common Issues and Solutions
- Unraveling Java Fundamentals: Methods, Constructors, and Parameters
- Expanding Horizons: Parameters in the Context of Java Classes and Objects
- Comparing Java Parameters with Other Programming Languages
- Wrapping Up: Parameters in Java
Grasping Parameters in Java: A Beginner’s Guide
Parameters in Java, also known as arguments, are the variables that are passed into methods or constructors. They provide a way for methods and constructors to accept input, making them more flexible and reusable.
Declaring Parameters in Java
In Java, parameters are declared within the parentheses of a method or constructor definition. Each parameter declaration includes a type and a name. For instance, in the following method definition, the parameter is of type String
and named name
:
public void greet(String name) {
}
Using Parameters in Java Methods
Once a parameter is declared, it can be used within the method or constructor. Here’s an example of a simple method that uses a parameter:
public void greet(String name) {
System.out.println('Hello, ' + name);
}
greet('Alice');
// Output:
// 'Hello, Alice'
In this example, the greet
method accepts one parameter: a String
named name
. When we call greet('Alice')
, the string ‘Alice’ is passed as the name
parameter. The method then prints out ‘Hello, Alice’.
This is a basic use of parameters in Java. By declaring and using parameters, we can make our methods and constructors more dynamic and powerful.
Diving Deeper: Types of Parameters in Java
As you continue your journey with Java, you’ll encounter different types of parameters. Let’s explore each type in detail.
Formal Parameters
Formal parameters are the parameters that are listed in the method or constructor definition. They act as placeholders for the values that will be passed when the method or constructor is called. Here’s an example:
public void greet(String name) { // 'name' is a formal parameter
System.out.println('Hello, ' + name);
}
Actual Parameters
Actual parameters, also known as arguments, are the actual values that are passed in when the method or constructor is called. Here’s an example:
public void greet(String name) {
System.out.println('Hello, ' + name);
}
greet('Alice'); // 'Alice' is an actual parameter
// Output:
// 'Hello, Alice'
In the above example, ‘Alice’ is the actual parameter that is passed to the greet
method.
Optional Parameters
Java doesn’t directly support optional parameters like some other programming languages do. However, you can achieve similar functionality by overloading methods or using varargs (variable arguments). Here’s an example of method overloading to create an optional parameter:
public void greet() {
System.out.println('Hello, User');
}
public void greet(String name) {
System.out.println('Hello, ' + name);
}
greet();
greet('Alice');
// Output:
// 'Hello, User'
// 'Hello, Alice'
In this example, there are two greet
methods. The first greet
method takes no parameters and is used when no name is provided. The second greet
method takes a String
parameter and is used when a name is provided. This is how we can simulate optional parameters in Java.
Exploring Overloading: A Powerful Use of Parameters in Java
Java provides the capability to overload methods and constructors, which allows us to define multiple methods or constructors with the same name but different parameters. This is a powerful technique that often involves the use of different parameters.
Method Overloading in Java
Method overloading is when we have multiple methods with the same name but different parameters (either different types or a different number of parameters). Here’s an example:
public void greet() {
System.out.println('Hello, User');
}
public void greet(String name) {
System.out.println('Hello, ' + name);
}
greet();
greet('Alice');
// Output:
// 'Hello, User'
// 'Hello, Alice'
In this example, we have two greet
methods. The first greet
method takes no parameters and prints out ‘Hello, User’. The second greet
method takes a String
parameter and prints out a personalized greeting. When we call greet()
with no parameters, the first greet
method is called. When we call greet('Alice')
with a String
parameter, the second greet
method is called.
Constructor Overloading in Java
Constructor overloading is similar to method overloading, but it involves constructors instead of methods. Here’s an example:
public class Greeter {
private String name;
public Greeter() {
this.name = 'User';
}
public Greeter(String name) {
this.name = name;
}
public void greet() {
System.out.println('Hello, ' + this.name);
}
}
Greeter greeter1 = new Greeter();
Greeter greeter2 = new Greeter('Alice');
greeter1.greet();
greeter2.greet();
// Output:
// 'Hello, User'
// 'Hello, Alice'
In this example, we have a Greeter
class with two constructors. The first constructor takes no parameters and sets the name
field to ‘User’. The second constructor takes a String
parameter and sets the name
field to the provided name. When we create a Greeter
object with no parameters, the first constructor is called. When we create a Greeter
object with a String
parameter, the second constructor is called.
Method overloading and constructor overloading are powerful techniques in Java that provide flexibility in how we use parameters.
Java Parameters: Common Issues and Solutions
Working with parameters in Java is not always a smooth ride. You might encounter some common issues, such as type mismatch, missing parameters, and null values. Let’s explore these issues and their solutions.
Type Mismatch
A type mismatch occurs when the type of the actual parameter doesn’t match the type of the formal parameter. Here’s an example:
public void greet(String name) {
System.out.println('Hello, ' + name);
}
greet(123);
// Output:
// Error: incompatible types: int cannot be converted to String
In this example, the greet
method expects a String
parameter, but we’re trying to pass an int
parameter. This results in a type mismatch error. The solution is to ensure that the actual parameter matches the type of the formal parameter.
Missing Parameters
If a method or constructor is called without the required number of parameters, a compile-time error occurs. Here’s an example:
public void greet(String name) {
System.out.println('Hello, ' + name);
}
greet();
// Output:
// Error: method greet in class Main cannot be applied to given types;
In this example, the greet
method requires one parameter, but we’re calling it with no parameters. This results in a missing parameter error. The solution is to provide the required number of parameters when calling a method or constructor.
Null Values
A null value is a value that has no associated instance. If a method or constructor is called with a null parameter, a NullPointerException
may occur. Here’s an example:
public void greet(String name) {
System.out.println('Hello, ' + name.toUpperCase());
}
greet(null);
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this example, the greet
method is called with a null parameter. When the method tries to call the toUpperCase
method on the null parameter, a NullPointerException
occurs. The solution is to check for null values before calling methods on parameters.
Unraveling Java Fundamentals: Methods, Constructors, and Parameters
To fully grasp the concept of parameters in Java, it’s essential to understand the basics of methods and constructors, and how parameters play a pivotal role in these elements.
Java Methods: A Quick Refresher
In Java, a method is a block of code that performs a specific task. It’s like a little machine – you feed it some input (in the form of parameters), it does something useful, and then it might give you back some output (in the form of a return value).
Here’s an example of a simple Java method that takes a String
parameter:
public void greet(String name) {
System.out.println('Hello, ' + name);
}
In this example, greet
is a method that takes one parameter: name
. The name
parameter is used in the method body to print a greeting.
Java Constructors: Laying the Foundation
A constructor in Java is a special type of method that’s used to initialize objects. Like methods, constructors can also take parameters. These parameters are used to initialize the object’s state.
Here’s an example of a Java constructor that takes a String
parameter:
public class Greeter {
private String name;
public Greeter(String name) {
this.name = name;
}
public void greet() {
System.out.println('Hello, ' + this.name);
}
}
In this example, Greeter
is a class that has a constructor. The constructor takes one parameter: name
. The name
parameter is used to initialize the name
field of the Greeter
object.
Variable Scope and Data Types: The Building Blocks
In Java, every variable has a scope, which is the part of the code where the variable can be accessed. Parameters in Java have local scope – they can only be accessed within the method or constructor where they’re declared.
Java is a statically typed language, which means that every variable must have a declared type. When declaring parameters, you must specify the type of the parameter. This type determines what kind of values the parameter can hold and what operations can be performed on it.
By understanding these fundamentals, you’ll be able to better understand the role of parameters in Java and how to use them effectively.
Expanding Horizons: Parameters in the Context of Java Classes and Objects
Parameters in Java play a crucial role beyond just methods and constructors. They are instrumental in the realm of object-oriented programming (OOP), particularly when dealing with Java classes and objects.
When you create a class in Java, you define a blueprint for a data type. This blueprint includes methods, and these methods often use parameters. When you create an object of that class, you can call these methods, passing in parameters as needed. This allows each object to have unique behavior based on the parameters passed.
Here’s an example:
public class Greeter {
private String name;
public Greeter(String name) {
this.name = name;
}
public void greet() {
System.out.println('Hello, ' + this.name);
}
}
Greeter aliceGreeter = new Greeter('Alice');
aliceGreeter.greet();
// Output:
// 'Hello, Alice'
In this example, the Greeter
class has a constructor that takes a String
parameter. When we create a Greeter
object, we pass in a String
parameter to give that object a unique name
.
Comparing Java Parameters with Other Programming Languages
Parameters in Java function similarly to parameters in many other programming languages. Most languages that support procedures or functions also support parameters in some form. However, the syntax and specific features can vary.
For example, in Python, parameters are defined in the function definition, similar to Java. However, Python supports optional parameters with default values directly, which Java does not.
In JavaScript, parameters are also defined in the function definition. JavaScript is more lenient with parameters, allowing you to call a function with more or fewer parameters than declared.
Despite these differences, the core concept of parameters—providing input to procedures, functions, methods, or constructors—remains the same across different languages.
Further Resources for Mastering Java Parameters
To expand your knowledge on parameters in Java and related concepts, you can explore the following resources:
- Optimizing Code Structure with Java Methods – Enhance your development workflow with Java methods.
Method Overload Usage in Java – Learn how to use method overloading to create versatile and flexible Java classes.
Mastering Varargs Parameter Usage in Java – Simplify method calls with a variable number of arguments with varargs in.
Oracle’s Java Documentation is a good resource that covers all aspects of Java parameters.
Java Methods with Parameters by W3Schools explains the concept of Java methods with parameters and how to use them.
Java Programming Masterclass for Software Developers covers using parameters in methods and constructors in Java programming.
Wrapping Up: Parameters in Java
In this comprehensive guide, we’ve delved into the world of parameters in Java, uncovering their significance from basic to advanced applications. We’ve seen how parameters serve as the lifeblood of methods and constructors, empowering them to perform their tasks dynamically and efficiently.
We began with the basics, understanding what parameters are, how to declare them, and how to use them in methods and constructors. We then ventured into more advanced territory, exploring different types of parameters such as formal parameters, actual parameters, and optional parameters. We also discussed how to overcome common issues with parameters in Java, such as type mismatch, missing parameters, and null values.
Our journey didn’t stop there. We examined alternative approaches like method overloading and constructor overloading, which often involve the use of different parameters. We also delved into the background fundamentals of methods and constructors in Java, to provide a better understanding of the role of parameters.
Here’s a quick comparison of the topics we’ve discussed:
Topic | Description |
---|---|
Basic Use | Understanding what parameters are, how to declare and use them |
Advanced Use | Exploring different types of parameters, such as formal, actual, optional parameters |
Alternative Approaches | Discussing method and constructor overloading in Java |
Troubleshooting | Addressing common issues with parameters, such as type mismatch, missing parameters, null values |
Whether you’re just starting out with Java or you’re looking to deepen your understanding, we hope this guide has given you a solid foundation in working with parameters in Java. The ability to effectively use parameters is a powerful tool in your Java programming arsenal. Happy coding!