Method Overloading in Java: In-Depth Tutorial
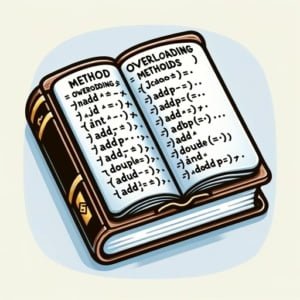
Ever found yourself puzzled about method overloading in Java? You’re not alone. Many developers find themselves in a maze when it comes to understanding this concept. Think of method overloading in Java as a multi-talented actor, capable of playing different roles based on the script it receives.
Method overloading is a powerful feature that allows a single method in Java to perform different tasks based on the parameters it receives. This not only enhances the flexibility of your code but also makes it more readable and maintainable.
In this guide, we’ll walk you through the concept of method overloading in Java, from the basics to more advanced topics. We’ll cover everything from understanding what method overloading is, its uses, to how to effectively implement it in your Java programs.
So, let’s dive in and start mastering method overloading in Java!
TL;DR: What is Method Overloading in Java?
Method overloading in Java is a feature that allows a class to have more than one method having the same name, if their argument lists are different, for example
void demo (int a)
anddouble demo(double a)
. It’s a way to increase the readability of the program.
Here’s a simple example:
void demo (int a) {
System.out.println ("a: " + a);
}
double demo(double a) {
System.out.println("a: " + a);
return a*a;
}
demo(10);
demo(10.10);
# Output:
# a: 10
# a: 10.1
In this example, we have two methods named demo
. The first demo
method takes an integer as a parameter, while the second demo
method takes a double. When we call demo(10)
, the first method is invoked, and when we call demo(10.10)
, the second method is invoked. This is the essence of method overloading in Java.
This is just the tip of the iceberg when it comes to method overloading in Java. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Grasping the Basics of Method Overloading in Java
- Delving Deeper: Advanced Method Overloading in Java
- Exploring Alternatives: Method Overriding in Java
- Troubleshooting Common Issues with Method Overloading
- Polymorphism: The Foundation of Method Overloading
- Method Overloading in Larger Projects
- Related Topics in Java
- Further Resources for Method Overloading Mastery
- Wrapping Up: Method Overloading in Java
Grasping the Basics of Method Overloading in Java
Method overloading in Java allows us to create multiple methods with the same name but different parameters. This not only increases the readability of the program but also provides the flexibility to call the same method with different types of data.
Let’s consider a simple example to understand this better:
public class OverloadDemo {
void demo (int a) {
System.out.println ("a: " + a);
}
void demo (int a, int b) {
System.out.println ("a and b: " + a + "," + b);
}
double demo(double a) {
System.out.println("a: " + a);
return a*a;
}
}
public class MethodOverloadTest {
public static void main (String args []) {
OverloadDemo Obj = new OverloadDemo();
double result;
Obj.demo(10);
Obj.demo(10, 20);
result = Obj.demo(5.5);
System.out.println("O/P : " + result);
}
}
# Output:
# a: 10
# a and b: 10,20
# a: 5.5
# O/P : 30.25
In the above example, we have three methods named demo
. The first demo
method takes one integer as a parameter, the second demo
method takes two integers, and the third demo
method takes a double. When we call demo(10)
, the first method is invoked. When we call demo(10, 20)
, the second method is invoked. When we call demo(5.5)
, the third method is invoked and its return value is printed.
This is the power of method overloading in Java – it allows us to use the same method name for different tasks, making our code cleaner and more readable.
Advantages of Method Overloading
- Increases the readability of the program.
- Makes the code cleaner and well-structured.
- Provides code reusability.
Potential Pitfalls of Method Overloading
While method overloading improves code readability and reusability, it can also lead to confusion if not used properly. For instance, if the methods’ parameters are not distinct enough, it might be challenging to determine which method will be called. Therefore, it’s essential to clearly define the parameters for each overloaded method.
Delving Deeper: Advanced Method Overloading in Java
As we move towards more complex scenarios, method overloading in Java becomes even more versatile. It allows us to overload methods not only with different types of parameters but also with a different number of parameters. This enhances the flexibility of our code, making it adaptable to various data inputs.
Let’s explore this with an example:
public class OverloadDemo {
void demo (int a) {
System.out.println ("a: " + a);
}
void demo (int a, int b) {
System.out.println ("a and b: " + a + "," + b);
}
double demo(double a) {
System.out.println("a: " + a);
return a*a;
}
void demo(int a, double b) {
System.out.println("a and b: " + a + "," + b);
}
void demo(double a, int b) {
System.out.println("a and b: " + a + "," + b);
}
}
public class MethodOverloadTest {
public static void main (String args []) {
OverloadDemo Obj = new OverloadDemo();
double result;
Obj.demo(10);
Obj.demo(10, 20);
result = Obj.demo(5.5);
System.out.println("O/P : " + result);
Obj.demo(10, 5.5);
Obj.demo(5.5, 10);
}
}
# Output:
# a: 10
# a and b: 10,20
# a: 5.5
# O/P : 30.25
# a and b: 10,5.5
# a and b: 5.5,10
In this example, we added two more demo
methods. One takes an integer and a double, and the other takes a double and an integer. When we call demo(10, 5.5)
, the method with an integer and a double parameter is invoked. When we call demo(5.5, 10)
, the method with a double and an integer parameter is invoked.
This shows the flexibility of method overloading in Java – we can use the same method name to perform different tasks based on the types and the order of the parameters, making our code even more adaptable.
Exploring Alternatives: Method Overriding in Java
While method overloading is a powerful feature in Java, it’s not the only way to create flexible and readable code. Another related concept is method overriding, which provides its own set of benefits and considerations.
Understanding Method Overriding
Method overriding in Java occurs when a subclass provides a specific implementation of a method that is already provided by its parent class. The method in the subclass must have the same name, return type, and parameters as the one in its parent class.
Here’s an example to illustrate method overriding:
class Animal {
void eat() {
System.out.println("eating...");
}
}
class Dog extends Animal {
void eat() {
System.out.println("eating bread...");
}
}
class TestOverriding {
public static void main(String args[]) {
Dog d = new Dog();
d.eat();
}
}
# Output:
# eating bread...
In this example, the Dog
class overrides the eat
method from the Animal
class. When we create a Dog
object and call the eat
method, the overridden method in the Dog
class is invoked, not the one in the Animal
class.
Weighing the Pros and Cons
Just like method overloading, method overriding has its own advantages and potential pitfalls. It allows for runtime polymorphism and enables the subclass to provide a different implementation of a method that is already provided by its parent class. However, method overriding can lead to confusion if not used properly, as it requires the method in the subclass to have the exact same name, return type, and parameters as the one in its parent class.
Making the Right Choice
Both method overloading and method overriding have their places in Java programming. Choosing between them depends on your specific needs. If you need to perform different tasks with the same method name but different parameters, method overloading is the way to go. If you want to provide a different implementation of a method that is already provided by a parent class, method overriding is the better choice.
Troubleshooting Common Issues with Method Overloading
While method overloading in Java can greatly enhance your code’s flexibility and readability, it can also present certain challenges. Let’s discuss some common issues and their solutions to help you navigate through these obstacles.
Incorrect Method Signature
One common mistake is having the same method signature for two overloaded methods. Remember, overloaded methods must have different parameter lists. If they don’t, you’ll encounter a compilation error.
public class OverloadDemo {
void demo (int a) {
System.out.println ("a: " + a);
}
void demo (int a) {
System.out.println ("a: " + a);
}
}
# Output:
# Compilation Error: method demo(int) is already defined in class OverloadDemo
In this example, both demo
methods have the same parameter list. As a result, the compiler throws an error. To fix this, ensure each overloaded method has a unique parameter list.
Overloading and Automatic Type Conversions
Java automatically promotes an argument of a lower datatype to a higher one when needed. This can sometimes lead to unexpected results when working with overloaded methods.
public class OverloadDemo {
void demo (int a) {
System.out.println ("a: " + a);
}
void demo (double a) {
System.out.println("a: " + a);
}
}
public class MethodOverloadTest {
public static void main (String args []) {
OverloadDemo Obj = new OverloadDemo();
Obj.demo(10); // calls the method with int parameter
Obj.demo(10.10); // calls the method with double parameter
Obj.demo(10); // calls the method with int parameter, not the one with double parameter
}
}
# Output:
# a: 10
# a: 10.1
# a: 10
In this example, Obj.demo(10)
calls the demo
method with an int parameter, not the one with a double parameter. This is because Java prefers the most specific method that can handle the arguments without a type conversion.
Best Practices and Optimization
- Clarity is key: Ensure that your overloaded methods perform similar tasks. This makes your code easier to read and understand.
- Be specific: Java prefers the most specific method that can handle the arguments without a type conversion. Keep this in mind when creating your overloaded methods.
- Document your code: Always document your code, especially when working with overloaded methods. This makes it easier for others (and future you) to understand what your methods do.
Polymorphism: The Foundation of Method Overloading
To truly understand method overloading in Java, it’s essential to grasp the concept of polymorphism. Polymorphism, a fundamental principle in object-oriented programming, is the characteristic that allows an object to take on many forms.
In Java, polymorphism is the ability of an object to behave in multiple ways. This is achieved through two mechanisms: method overloading (compile-time polymorphism) and method overriding (runtime polymorphism).
Polymorphism and Method Overloading
Method overloading is a form of static or compile-time polymorphism. In method overloading, the method to be invoked is determined at compile time based on the number and type of arguments. This is why we can define multiple methods with the same name but different parameters.
public class OverloadDemo {
void demo (int a) {
System.out.println ("a: " + a);
}
void demo (int a, int b) {
System.out.println ("a and b: " + a + "," + b);
}
double demo(double a) {
System.out.println("a: " + a);
return a*a;
}
}
public class MethodOverloadTest {
public static void main (String args []) {
OverloadDemo Obj = new OverloadDemo();
double result;
Obj.demo(10);
Obj.demo(10, 20);
result = Obj.demo(5.5);
System.out.println("O/P : " + result);
}
}
# Output:
# a: 10
# a and b: 10,20
# a: 5.5
# O/P : 30.25
In this example, demo(10)
, demo(10, 20)
, and demo(5.5)
are all valid calls. The correct method to execute is determined at compile time based on the number and type of arguments.
Understanding polymorphism and its role in method overloading gives us a deeper insight into Java’s flexibility and adaptability. It’s the cornerstone that allows us to use the same method name to perform different tasks, making our code cleaner, more readable, and more efficient.
Method Overloading in Larger Projects
Method overloading in Java isn’t just a concept limited to small programs or basic tasks. It has wide-ranging applications in larger projects, where the complexity of the code increases and the need for flexibility and readability becomes paramount.
In large-scale projects, method overloading can be used to provide different implementations of a method based on different scenarios. This can greatly simplify the code and make it more adaptable to changes.
For example, consider a banking application where you need to calculate interest. The interest calculation might differ based on the type of account (e.g., savings, current, or fixed deposit). In such a case, you can use method overloading to create different calculateInterest
methods for each account type.
Related Topics in Java
While method overloading is a powerful feature in Java, it’s just one part of the bigger picture. There are several related topics that often accompany method overloading in typical use cases. These include:
- Method Overriding: As we discussed earlier, method overriding is another form of polymorphism in Java. It allows a subclass to provide a specific implementation of a method that is already provided by its parent class.
- Inheritance: Inheritance is a mechanism in Java where one class acquires the properties (fields and methods) of another. With the use of inheritance, information is made manageable in a hierarchical order.
- Interfaces and Abstract Classes: These are advanced topics in Java that provide a way to achieve abstraction. They can be used to define a template for a class, including methods without bodies (abstract methods).
Further Resources for Method Overloading Mastery
For a deeper dive into method overloading and related topics in Java, here are some resources that provide more in-depth information:
- Defining and Using Java Methods: Tutorial – Simplify Java programming by mastering essential methods.
Exploring Method Signatures in Java – Master the art of crafting method signatures in Java.
Understanding Method Parameter Passing in Java – Explore Java method parameters for customizing method behavior.
Oracle Java Documentation provides a comprehensive guide on method overloading in obect oriented programming.
GeeksforGeeks’ Java Programming covers all aspects of Java programming, including method overloading.
JavaTpoint’s Java Tutorial covers method overloading and related concepts in Java.
Wrapping Up: Method Overloading in Java
In this comprehensive guide, we’ve taken a deep dive into the world of method overloading in Java, a powerful feature that enhances code flexibility, readability, and maintainability.
We began with the basics, explaining what method overloading is and how it can be used in simple scenarios. We then ventured into more complex uses of method overloading, discussing how it can handle different types and numbers of parameters. We also touched on a related concept, method overriding, and provided a comparison to help you choose the right approach for your needs.
Along the way, we addressed common challenges you might encounter when using method overloading, such as incorrect method signatures and automatic type conversions, and provided solutions to help you navigate these hurdles. We also shared best practices and optimization tips to help you make the most of method overloading.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Readability | Complexity |
---|---|---|---|
Method Overloading | High | High | Moderate |
Method Overriding | Moderate | High | Moderate |
Whether you’re just starting out with method overloading or looking to refine your skills, we hope this guide has given you a deeper understanding of this powerful feature in Java.
With its balance of flexibility, readability, and complexity, method overloading is an essential tool in any Java programmer’s toolkit. Now that you’re equipped with this knowledge, you’re well on your way to writing more efficient and adaptable Java code. Happy coding!