Python: Get Current Directory | Easy Guide
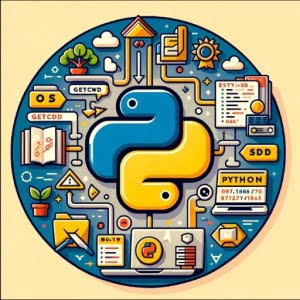
Are you trying to figure out where your Python script is running from? This can be a common challenge for many developers. Python, however, offers a simple way to get the current directory, acting as a GPS for your code.
This guide will walk you through the process of getting the current directory in Python, explaining why it’s useful and how you can implement it in your projects. We’ll start from the basics and gradually move towards more advanced techniques.
Let’s dive in and start mastering the art of getting the current directory in Python!
TL;DR: How Do I Get the Current Directory in Python?
To get the current directory in Python, you can use the
os.getcwd()
function. This function returns the path of the current working directory where your Python script is executing.
Here’s a simple example:
import os
print(os.getcwd())
# Output:
# '/Users/username/Desktop'
In this example, we import the os
module and use the os.getcwd()
function to print the current directory. The output will be the path of the directory where your Python script is running.
This is a basic way to get the current directory in Python, but there’s much more to learn about file and directory manipulation in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Use of Python’s os.getcwd() Function
- Advanced Use of os.getcwd()
- Exploring Alternative Approaches to Get Current Directory
- Troubleshooting Common Issues with Python Get Current Directory
- Understanding Python’s File and Directory Handling
- Beyond the Basics: The Power of Python Directory Handling
- Wrapping Up: Mastering Python’s Current Directory Handling
Basic Use of Python’s os.getcwd() Function
Python’s built-in os
module provides a function os.getcwd()
to get the current directory. The os.getcwd()
function is a simple, yet powerful command that can help you keep track of your file and directory locations.
Here’s a step-by-step guide on how to use it:
- Import the
os
module. This module provides a portable way of using operating system dependent functionality like reading or writing to the file system.
import os
- Use the
os.getcwd()
function to get the current directory.
current_directory = os.getcwd()
print(current_directory)
# Output:
# '/Users/username/Desktop'
In this example, we first import the os
module. Then, we use os.getcwd()
to get the current directory and store it in the current_directory
variable. Finally, we print the current_directory
which outputs the path of the directory where your Python script is running.
Advantages of Using os.getcwd()
Using os.getcwd()
has several advantages:
- It’s a quick and easy way to get the current directory.
- It’s part of the built-in
os
module, so no additional installations are necessary. - It’s cross-platform and works on any operating system that Python supports.
Potential Pitfalls of Using os.getcwd()
While os.getcwd()
is handy, there are a few things to be aware of:
- If the current working directory is deleted,
os.getcwd()
will raise an error. The result of
os.getcwd()
can change if another part of your code changes the current working directory.The output is an absolute path, which can be a problem if you want to distribute your code and need relative paths.
Despite these potential pitfalls, os.getcwd()
is a reliable and straightforward way to get the current directory in Python, making it a valuable tool for any Python programmer.
Advanced Use of os.getcwd()
As you become more comfortable with Python, you’ll find that os.getcwd()
can be combined with other file and directory manipulation functions for more complex operations.
Using os.getcwd() with os.chdir()
One such function is os.chdir()
, which changes the current working directory. Here’s an example of how you might use these two functions together:
import os
# Print the initial working directory
print('Initial Directory:', os.getcwd())
# Change the current working directory
os.chdir('/Users/username/Documents')
# Print the current working directory after change
print('Current Directory:', os.getcwd())
# Output:
# Initial Directory: /Users/username/Desktop
# Current Directory: /Users/username/Documents
In this example, we first print the initial working directory. Then, we change the current working directory to ‘/Users/username/Documents’ using os.chdir()
. Finally, we print the current working directory again, which now shows the updated directory.
Using os.getcwd() with os.path.join()
Another useful function to use in tandem with os.getcwd()
is os.path.join()
. This function can be used to join one or more path components intelligently.
import os
# Get the current directory
current_directory = os.getcwd()
# Create a new file path
new_file_path = os.path.join(current_directory, 'new_file.txt')
print('New File Path:', new_file_path)
# Output:
# New File Path: /Users/username/Desktop/new_file.txt
In this code block, we first get the current directory. Then, we create a new file path by joining the current directory path with a new file name ‘new_file.txt’ using os.path.join()
. The output will be the complete path of the new file in the current directory.
These examples demonstrate that os.getcwd()
is not just a standalone function, but a part of Python’s comprehensive toolkit for file and directory manipulation.
Exploring Alternative Approaches to Get Current Directory
While os.getcwd()
is a straightforward way to get the current directory in Python, it’s not the only way. Python’s os.path
module offers alternative methods that can be more suitable in certain situations.
Using os.path.dirname(os.path.realpath(file))
One such alternative method involves using os.path.dirname(os.path.realpath(__file__))
. This command returns the directory of the script being run. Here’s how you can use it:
import os
# Get the directory of the script
directory = os.path.dirname(os.path.realpath(__file__))
print('Directory:', directory)
# Output:
# Directory: /Users/username/Desktop
In this example, os.path.realpath(__file__)
returns the absolute path of the script file, and os.path.dirname()
returns the directory of this path. This is particularly useful when you want to get the directory of the script file, even if it’s not the current working directory.
Benefits and Drawbacks
This alternative approach has its advantages and drawbacks:
- Benefits:
- It returns the directory of the script file, not the current working directory, which can be useful in certain situations.
- Like
os.getcwd()
, it’s part of the built-inos
module, so no additional installations are necessary.
- Drawbacks:
- It can be a bit more complex to understand and use than
os.getcwd()
. - It returns the directory of the script file, not the current working directory. This can be a drawback if the script changes the current working directory.
- It can be a bit more complex to understand and use than
When deciding which method to use, consider the needs of your script. If you need the current working directory, os.getcwd()
is the best choice. If you need the directory of the script file, consider using os.path.dirname(os.path.realpath(__file__))
.
Troubleshooting Common Issues with Python Get Current Directory
While Python’s os.getcwd()
function is generally reliable, there are a few common issues that you might encounter when trying to get the current directory. In this section, we’ll discuss these issues and provide solutions.
Issue: Current Working Directory is Deleted
If the current working directory is deleted while your script is running, os.getcwd()
will raise an error.
import os
# Delete the current working directory manually before running this
try:
print(os.getcwd())
except FileNotFoundError:
print('Directory not found')
# Output:
# Directory not found
In this example, if the current working directory is deleted before running the script, Python raises a FileNotFoundError
. To handle this error, we use a try/except
block that prints ‘Directory not found’ when the error occurs.
Issue: Current Working Directory is Changed
Another common issue occurs when the current working directory is changed by another part of your code. This can cause os.getcwd()
to return an unexpected directory.
import os
# Change the current working directory
current_directory = '/Users/username/Documents'
os.chdir(current_directory)
# Get the current working directory
print('Current Directory:', os.getcwd())
# Output:
# Current Directory: /Users/username/Documents
In this example, we first change the current working directory using os.chdir()
. Then, when we get the current directory using os.getcwd()
, it returns the new directory, not the original one.
To avoid this issue, be mindful of where and when you’re changing the current working directory in your code. If necessary, you can always store the original directory in a variable before changing it, so you can refer back to it later.
Understanding Python’s File and Directory Handling
Python’s approach to file and directory handling is one of the reasons behind its popularity. It provides a set of built-in modules and functions that make it easy to read, write, and manipulate files and directories. Understanding these fundamentals is crucial when working with Python, particularly when dealing with tasks like getting the current directory.
File Paths in Python
A file path in Python is a string that states the location of a file or a directory on your system. Python supports both absolute and relative file paths.
- An absolute file path starts with the root folder, like
/Users/username/Documents/my_script.py
on Unix-based systems orC:\Users\username\Documents\my_script.py
on Windows. A relative file path, on the other hand, is relative to the current working directory. For instance, if the current directory is
/Users/username/Documents
, the relative path formy_script.py
would be simplymy_script.py
.
Python’s os.path
module provides several functions to work with file paths, like os.path.join()
to join multiple paths, os.path.dirname()
to get the directory name, os.path.basename()
to get the file name, etc.
The os Module and Current Directory
The os
module is a part of Python’s standard library that provides functions to interact with the operating system, including file and directory manipulation. One of the most commonly used functions from this module is os.getcwd()
, which returns the current directory. Knowing the current directory is essential, as many file operations are performed relative to it.
In the context of our search query ‘python get current directory’, understanding these fundamentals is key. As you’ve seen in the previous sections, getting the current directory in Python is not just about calling os.getcwd()
. It’s about understanding the file system, knowing how Python interacts with it, and using this knowledge to write effective and reliable code.
Beyond the Basics: The Power of Python Directory Handling
Understanding how to get the current directory in Python is a fundamental skill, but it’s just the tip of the iceberg. The real power comes when you start combining this knowledge with other file and directory manipulation techniques in Python.
Leveraging Python for Larger Projects
In larger scripts or projects, knowing the current directory can be crucial. It allows you to create, read, update, and delete files in the correct locations. It also helps you navigate through directories, making your code more flexible and robust.
For instance, if you’re developing a web scraping tool in Python, you might need to save the scraped data into a CSV file in the same directory as your script. By getting the current directory, you can ensure the CSV file is created in the right place, regardless of where you run the script from.
File Manipulation and Directory Navigation
Getting the current directory often goes hand-in-hand with other tasks like file manipulation and directory navigation. Python’s os
module provides a wealth of functions for these tasks, such as os.listdir()
to list the files in a directory, os.rename()
to rename a file, os.remove()
to delete a file, and many more.
Here’s a simple example of how you might use these functions together:
import os
# Get the current directory
current_directory = os.getcwd()
# List the files in the current directory
files = os.listdir(current_directory)
print('Files:', files)
# Output:
# Files: ['script.py', 'data.csv', 'report.txt']
In this example, we first get the current directory. Then, we list the files in the current directory using os.listdir()
. The output is a list of file names in the current directory.
Further Resources for Python File and Directory Handling
To delve deeper into Python’s file and directory handling capabilities, here are some valuable resources:
- IOFlood’s Python OS Article explores real-world examples of using “os” for file management and system interaction.
Python File Renaming Guide – Master the art of renaming files for data organization and maintenance.
Path Manipulation with Python Pathlib explains the details of path handling, joining, and validation using “pathlib.”
Python’s official os module documentation is acomprehensive guide to all the functions provided by the
os
module.Real Python’s tutorial on reading and writing Python files explains file I/O operations in Python, with plenty of examples.
Python Course’s guide on file management in Python covers various aspects of file management and manipulation.
Wrapping Up: Mastering Python’s Current Directory Handling
In this comprehensive guide, we’ve navigated the ins and outs of working with the current directory in Python, using the os.getcwd()
function and other related techniques.
We began with the basics, learning how to use os.getcwd()
to retrieve the current directory. We further delved into more advanced usage, exploring how to use os.getcwd()
in conjunction with other file and directory manipulation functions like os.chdir()
and os.path.join()
. We also highlighted alternative approaches using os.path.dirname(os.path.realpath(__file__))
to get the directory of the script file.
Along the way, we tackled common issues that you might encounter when trying to get the current directory in Python, such as the current working directory being deleted or changed, and provided solutions to help you overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Complexity |
---|---|---|
os.getcwd() | Get the current working directory | Low |
os.getcwd() with os.chdir() | Change and get the current working directory | Medium |
os.getcwd() with os.path.join() | Create a new file path in the current directory | Medium |
os.path.dirname(os.path.realpath(file)) | Get the directory of the script file | High |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of how to get the current directory in Python and the importance of this operation in file and directory manipulation.
With its balance of simplicity and versatility, Python’s approach to getting the current directory is a powerful tool for any developer. Happy coding!