Python Rename File: 7 Easy Methods with Examples
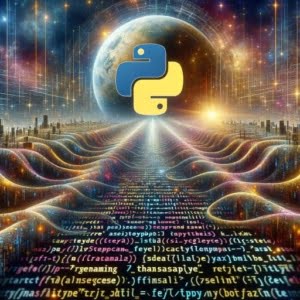
File renaming is a frequent task in system management, and it can often be quite tedious. But what if there was a tool to make this task less daunting? This is where Python comes in. Python is a robust and versatile programming language that can significantly streamline the process of file renaming.
In this comprehensive guide, we will journey into the realm of file renaming with Python. We will explore various methods of renaming files, from the simplest to the more advanced ones, and even learn how to handle potential errors.
Whether you’re a Python novice or a seasoned developer seeking more efficient ways to manage files, this guide is for you. Let’s discover how Python can make your file renaming tasks less of a chore and more of a breeze.
TL;DR: How can Python help with file renaming?
Python provides various functions and modules, such as
os.rename()
,shutil.move()
, andglob.glob()
, which can be used to rename files efficiently. For instance, the simplest way to rename a file in Python is by using theos.rename()
function as shown below:
import os
os.rename('old_filename.txt', 'new_filename.txt')
This command will rename the file ‘old_filename.txt’ to ‘new_filename.txt’. For more advanced methods, tips, and tricks on file renaming with Python, continue reading the rest of the article.
Table of Contents
Renaming a Single File in Python
Python offers an array of methods to rename a single file, each with its unique advantages. In this section, we’ll explore three of these methods in detail.
1: The os.rename() Function
One of the simplest ways to rename a single file in Python is by using the rename() function provided by Python’s os module. Here’s an example of how you can use it:
import os
os.rename('old_filename.txt', 'new_filename.txt')
In the above code, ‘old_filename.txt’ is the current name of the file, and ‘new_filename.txt’ is the name you want to change it to. This method is straightforward and efficient.
2: The shutil Module
Another effective way to rename a file in Python is by using the shutil module. This module offers a function called move(), which can also be used for renaming files. Here’s how you can use it:
import shutil
shutil.move('old_filename.txt', 'new_filename.txt')
In this instance, move() works similarly to os.rename(). The advantage of using shutil.move() is its added flexibility, such as renaming files across different file systems.
3: Incorporating a Timestamp
Python also enables you to rename a file by incorporating a timestamp, adding a unique chronological aspect to your file organization. This can be particularly handy when dealing with a large number of files and needing to maintain a chronological order. Here’s an example:
import os
import time
timestamp = time.strftime('%Y%m%d%H%M%S')
new_filename = f'{timestamp}_filename.txt'
os.rename('old_filename.txt', new_filename)
In this example, we’re using Python’s time module to get the current timestamp, and then using an f-string to create a new file name that includes the timestamp. This way, every time you rename a file, it will have a unique name based on the exact time it was renamed.
Summary of methods to rename a single file in Python:
Method | Syntax | Advantage |
---|---|---|
os.rename() | os.rename('old_filename.txt', 'new_filename.txt') | Straightforward and efficient |
shutil.move() | shutil.move('old_filename.txt', 'new_filename.txt') | Flexibility in renaming files across different file systems |
Incorporating a Timestamp | os.rename('old_filename.txt', f'{timestamp}_filename.txt') | Adds a unique chronological aspect to file organization |
Renaming Multiple Files with Python
Renaming multiple files with Python can be done using several effective methods. In this section, we will dive into three of these approaches and see how they can enhance your file renaming efficiency.
1: The os.listdir() Function with a For Loop
One of the simplest ways to rename multiple files in Python is by using the os.listdir() function in conjunction with a for loop. The os.listdir() function provides a list of all files and directories in the specified directory, which can then be iterated over using a for loop to rename each file. Here’s an example:
import os
for filename in os.listdir('directory_path'):
if filename.endswith('.txt'):
os.rename(filename, 'new_' + filename)
In this example, we’re renaming all .txt files in the specified directory by adding a ‘new_’ prefix to each file name.
2: Basic use of the glob Module
Another method to rename multiple files in Python is by using the glob module. This module is especially handy when you want to rename a specific set of files that match a certain pattern. Here’s how you can use it:
import glob
import os
for filename in glob.glob('*.txt'):
os.rename(filename, 'new_' + filename)
In this example, we’re using glob.glob() to get a list of all .txt files, and then renaming each file by adding a ‘new_’ prefix to the file name.
3: Pattern-Based File Renaming with the glob Module
The glob module can also be employed for pattern-based file renaming, offering a more targeted approach to file management in Python.
This can be especially beneficial when dealing with a large number of files and needing to rename only a specific subset of them. Here’s an example:
import glob
import os
for filename in glob.glob('draft*.txt'):
os.rename(filename, filename.replace('draft', 'final'))
This command changes all filenames starting with ‘draft’ and ending with ‘.txt’ in your current directory.
Here’s the output you can expect before and after running the Python command.
# Before
$ ls
draft1.txt draft2.txt draft3.txt
# After
$ ls
final1.txt final2.txt final3.txt
The ‘draft’ prefix has been replaced with ‘final’ for all text files.
Whether you’re dealing with a large number of files or need to rename a specific subset of them, Python’s os and glob modules provide the flexibility and efficiency you need.
Summary of approaches to rename multiple files in Python:
Approach | Syntax | Use Case |
---|---|---|
os.listdir() with a For Loop | os.rename(filename, 'new_' + filename) | Renames all .txt files in a directory by adding a ‘new_’ prefix |
glob Module | os.rename(filename, 'new_' + filename) | Renames all .txt files by adding a ‘new_’ prefix |
Pattern-Based with glob Module | os.rename(filename, filename.replace('old_pattern', 'new_pattern')) | Renames all .txt files that start with ‘old_pattern’ by replacing ‘old_pattern’ with ‘new_pattern’ |
Handling Errors and Exceptions in File Renaming
While working with file operations in Python, encountering errors is not a rare occurrence.
One of the most common errors you might stumble upon when renaming files is OSError. This error often pops up when the file you’re trying to rename either doesn’t exist or you lack the necessary permissions to modify it.
Fortunately, Python equips you with mechanisms to handle such errors and exceptions, enabling you to write robust and error-resistant code.
Understanding OSError
In Python, OSError is a built-in exception triggered for system-related errors, encompassing many file-related operations including renaming.
This error houses several attributes that can provide more insight into the error. The two most crucial ones are errno
(providing the error number) and strerror
(offering a string representation of the error).
Here’s an example of how you might encounter an OSError when trying to rename a file:
import os
try:
os.rename('non_existent_file.txt', 'new_filename.txt')
except OSError as e:
print(f'Error number: {e.errno}, Error text: {e.strerror}')
In this example, we’re trying to rename a file that doesn’t exist, which triggers an OSError. The error number and error text are then printed out to provide more information about the error.
Utilizing Python’s Exception Handling Mechanism
The try and except blocks in Python offer a robust mechanism for handling exceptions like OSError.
The code that could potentially trigger an exception is placed inside the try block. If an exception is raised, the code inside the except block gets executed.
Here’s how you can use try and except blocks to handle errors when renaming a file:
import os
try:
os.rename('old_filename.txt', 'new_filename.txt')
except OSError as e:
print(f'Error: {e.strerror}')
In this example, if the file renaming operation raises an OSError, the error message is printed out, helping you understand and tackle the issue.
Advanced File Renaming
Now that we’ve covered the basics, let’s learn about some more advanced topics. These include dynamic file naming, renaming directories, renaming files with pathlib, and renaming file extentions.
Using Python’s f-strings for Dynamic File Naming
Python’s extensive capabilities include the ability to dynamically generate and assign new names to files. This feature is especially beneficial when dealing with a large number of files that need renaming based on specific conditions or patterns
Python’s f-strings, a unique feature allowing you to embed expressions inside string literals using curly braces {}
, can be used with the os.rename()
function to dynamically generate new file names. Here’s an example:
import os
file_number = 1
for filename in os.listdir('directory_path'):
if filename.endswith('.txt'):
new_filename = f'new_file_{file_number}.txt'
os.rename(filename, new_filename)
file_number += 1
In this example, we’re renaming all .txt files in the specified directory by assigning a new name that includes a dynamically generated file number. The file number increments for each file, producing unique file names like ‘new_file_1.txt’, ‘new_file_2.txt’, and so on.
How To Rename a Directory in Python
Renaming a directory in Python is as straightforward as renaming a file. The os.rename()
function can be used for this purpose. Here’s an example:
import os
os.rename('old_directory_name', 'new_directory_name')
In this example, ‘old_directory_name’ is the current name of the directory, and ‘new_directory_name’ is the name you want to change it to.
Using pathlib to Rename a File
The pathlib module in Python provides an object-oriented interface for handling file paths, making it a user-friendly approach to renaming files. Here’s how you can utilize pathlib to rename a file:
from pathlib import Path
old_file = Path('old_filename.txt')
old_file.rename('new_filename.txt')
In this example, we’re creating a Path object for the file we want to rename and then using the rename() method to modify its name.
How To Change File Extensions
If you wish to change the extension of a file without modifying its name, you can utilize the os
and os.path
modules in Python. Here’s how:
import os
filename, file_extension = os.path.splitext('filename.txt')
os.rename('filename.txt', filename + '.docx')
In this example, we’re using os.path.splitext()
to separate the file name and its extension, and then employing os.rename()
to change the extension to ‘.docx’.
Further Resources for Python File I/O Operations
To further enhance your skills in Python file handling, as well as Python File classes and methods, consider exploring the following resources:
- Python OS Module Best Practices – Explore the role of “os” in creating temporary files and directories securely.
Python os.listdir() Usage – Explore Python’s “os” module for listing directory contents and files.
Directory Navigation with Python GetCurrentDirectory() – Discover the importance of knowing your current directory for file operations in Python.
Guide on files I/O in Python provides a detailed understanding about how to read, write and manipulate files in Python.
Reading and writing files in Python guide teaches you about file operations in Python, including reading, writing, and appending.
Guide on exception and file handling in Python covers the basics of exception handling, relating to file operations in Python.
Invest time in investigating these resources and weave them into your skillset. Remember, each new skill you acquire strengthens your programming prowess.
Final Thoughts
In this comprehensive guide, we’ve explored the power of Python in renaming files. We’ve seen how Python offers a variety of methods for renaming a single file, from the direct os.rename()
function to the flexible shutil.move()
method, and the unique approach of integrating a timestamp, much like a librarian systematically updating book titles.
We’ve also delved into the practical approaches for renaming multiple files, using the os.listdir()
function with a for loop, the glob module for pattern matching, and the dynamic generation of new file names with Python’s f-strings.
Furthermore, we’ve discussed the importance of error handling in file operations and how Python’s exception handling mechanism can help manage errors effectively.
Beyond what we’ve covered, there’s so much more to discover in Python. See for yourself!
Whether you’re a beginner just starting out with Python or a seasoned developer, these methods can greatly simplify and streamline your file renaming tasks. Give it a try, and you’ll find Python’s power in file renaming as intuitive and efficient as managing a well-organized library.