Python os.listdir() Function | Directory Listing Guide
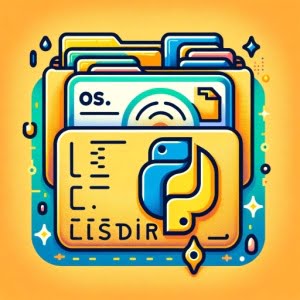
Are you finding it challenging to list files and directories in Python? You’re not alone. Many developers find themselves in a maze when it comes to navigating through directories in Python, but there’s a function that can make this process a breeze.
Just like a seasoned librarian, Python’s os.listdir() function can help you navigate through your directories. This function is a powerful tool that can list all files and directories in a specific directory, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using os.listdir() in Python, from its basic usage to more advanced techniques. We’ll cover everything from the fundamentals of Python’s os module to handling common issues and their solutions.
So, let’s dive in and start mastering os.listdir() in Python!
TL;DR: How Do I List Files and Directories in Python?
To list files and directories in Python, you can use the
os.listdir()
function in Python’s os module, likeprint(os.listdir('.'))
. This function returns a list containing the names of the entries in the directory given by path.
Here’s a simple example:
import os
print(os.listdir('.'))
# Output:
# ['file1.txt', 'file2.txt', 'dir1', 'dir2']
In this example, we import the os module and use the os.listdir()
function to list all files and directories in the current directory, denoted by ‘.’. The output is a list of file and directory names.
This is a basic way to use
os.listdir()
in Python, but there’s much more to learn about listing files and directories in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of os.listdir()
The os.listdir()
function in Python is a straightforward and effective way to list files and directories in a specific directory. It is part of the os module, which provides a portable way of using operating system dependent functionality.
To use os.listdir()
, you first need to import the os module. Here’s how you can do it:
import os
Now that the os module is imported, you can use os.listdir()
. The function takes a single argument: the path of the directory you want to list files and directories from. If no argument is given, it defaults to the current directory (‘.’).
Here’s an example of how to use os.listdir()
to list files and directories in the current directory:
import os
files_and_directories = os.listdir()
print(files_and_directories)
# Output:
# ['file1.txt', 'file2.txt', 'dir1', 'dir2']
In this example, os.listdir()
returns a list of all files and directories in the current directory. The output is a list of file and directory names.
The os.listdir()
function is a simple yet powerful tool for listing files and directories in Python. It’s easy to use and works across all platforms, making it a versatile tool for any Python developer.
However, os.listdir()
does not differentiate between files and directories. It simply returns all entries in the specified directory. If you need to distinguish between files and directories, you’ll need to use additional functions from the os module, which we’ll cover in the advanced use section.
One potential pitfall when using os.listdir()
is that it does not handle exceptions by default. If you try to list files and directories from a non-existent directory or a directory you do not have permission to access, os.listdir()
will raise an exception. We’ll discuss how to handle these exceptions in the troubleshooting and considerations section.
Advanced Usage of os.listdir()
While the basic use of os.listdir()
is quite straightforward, you can use it in conjunction with other functions from the os module for more complex tasks. In this section, we’ll discuss how to use os.listdir()
to not only list files and directories, but also distinguish between them and manipulate them.
One common use case is to separate files from directories. To do this, you can use the os.path.isdir()
function, which checks if a given path is a directory. Here’s an example:
import os
all_entries = os.listdir()
files = [entry for entry in all_entries if not os.path.isdir(entry)]
directories = [entry for entry in all_entries if os.path.isdir(entry)]
print('Files:', files)
print('Directories:', directories)
# Output:
# Files: ['file1.txt', 'file2.txt']
# Directories: ['dir1', 'dir2']
In this example, we use list comprehensions to separate files and directories. We use os.path.isdir()
to check if each entry returned by os.listdir()
is a directory. If it is, we add it to the directories list. If it’s not, we add it to the files list.
Another advanced use of os.listdir()
is to manipulate files and directories. For instance, you can rename all files in a directory with a specific extension. To do this, you can use the os.rename()
function. Here’s an example:
import os
for filename in os.listdir():
if filename.endswith('.txt'):
os.rename(filename, filename[:-4] + '.bak')
print(os.listdir())
# Output:
# ['file1.bak', 'file2.bak', 'dir1', 'dir2']
In this example, we iterate over each file in the current directory. If a file ends with ‘.txt’, we rename it to end with ‘.bak’ instead. We use os.rename()
to rename the files.
These are just a few examples of how you can use os.listdir()
in more complex ways. By combining it with other functions from the os module, you can perform a wide range of tasks related to file and directory manipulation.
Exploring Alternative Methods to List Files
While os.listdir()
is a powerful and commonly used function to list files and directories in Python, it’s not the only way to do it. There are other modules in Python that provide similar functionality. In this section, we’ll introduce two alternative methods: the glob
module and the pathlib
module.
Listing Files with the glob
Module
The glob
module is used to find all pathnames matching a specified pattern. Here’s an example of how to use it to list all ‘.txt’ files in the current directory:
import glob
txt_files = glob.glob('*.txt')
print(txt_files)
# Output:
# ['file1.txt', 'file2.txt']
In this example, glob.glob('*.txt')
returns a list of all ‘.txt’ files in the current directory. The output is a list of file names.
One advantage of using glob
over os.listdir()
is that glob
can search for files with specific patterns, making it a powerful tool for more complex file searching tasks. However, glob
does not differentiate between files and directories by default, just like os.listdir()
.
Listing Files with the pathlib
Module
The pathlib
module offers an object-oriented approach to file and directory manipulation. It provides a more high-level and intuitive interface compared to os.listdir()
. Here’s an example of how to use it to list all files in the current directory:
from pathlib import Path
files = [entry.name for entry in Path('.').iterdir() if entry.is_file()]
directories = [entry.name for entry in Path('.').iterdir() if entry.is_dir()]
print('Files:', files)
print('Directories:', directories)
# Output:
# Files: ['file1.txt', 'file2.txt']
# Directories: ['dir1', 'dir2']
In this example, Path('.').iterdir()
returns an iterator that produces Path
objects for all files and directories in the current directory. We use list comprehensions and the is_file()
and is_dir()
methods to separate files and directories.
One advantage of using pathlib
over os.listdir()
is that pathlib
provides a more high-level and intuitive interface for file and directory manipulation. However, pathlib
is only available in Python 3.4 and later, so it’s not suitable for codebases that need to support older versions of Python.
In conclusion, while os.listdir()
is a simple and effective way to list files and directories in Python, there are alternative methods that offer different advantages. Depending on your specific needs and the complexity of your tasks, you might find the glob
module or the pathlib
module more suitable. We recommend trying out all three methods and choosing the one that fits your needs the best.
Troubleshooting os.listdir()
While os.listdir()
is a powerful tool, it’s not without its potential pitfalls. In this section, we’ll discuss some common issues you might encounter when using os.listdir()
, such as handling FileNotFoundError
and PermissionError
, and provide solutions and workarounds for each issue.
Handling FileNotFoundError
os.listdir()
raises a FileNotFoundError
if the directory you’re trying to list files and directories from does not exist. Here’s an example:
import os
try:
os.listdir('/non/existent/directory')
except FileNotFoundError:
print('Directory does not exist.')
# Output:
# Directory does not exist.
In this example, we use a try/except block to catch the FileNotFoundError
and print a custom error message. This is a simple way to handle non-existent directories.
Handling PermissionError
os.listdir()
raises a PermissionError
if you don’t have permission to access the directory you’re trying to list files and directories from. Here’s an example:
import os
try:
os.listdir('/root')
except PermissionError:
print('Permission denied.')
# Output:
# Permission denied.
In this example, we use a try/except block to catch the PermissionError
and print a custom error message. This is a simple way to handle directories you don’t have permission to access.
Other Considerations
It’s important to note that os.listdir()
does not return hidden files (files starting with a dot) by default. If you need to list hidden files, you’ll need to use other functions or modules, such as os.scandir()
or the glob
module with the glob.glob('*.*')
pattern.
In conclusion, while os.listdir()
is a powerful tool for listing files and directories in Python, it’s not without its potential pitfalls. By understanding these pitfalls and how to handle them, you can use os.listdir()
more effectively and avoid common issues.
Understanding Python’s os Module
The os
module in Python provides a way of using operating system dependent functionality. It includes functions for interacting with the file system, such as creating, removing, and changing directories, and listing files and directories.
The os.listdir()
function is part of the os
module. It uses an underlying system call to read the directory entries. On Unix and Linux, it uses the readdir()
system call. On Windows, it uses the FindFirstFile()
and FindNextFile()
functions.
Here’s an example of how to use os.listdir()
to list files and directories in the current directory:
import os
print(os.listdir())
# Output:
# ['file1.txt', 'file2.txt', 'dir1', 'dir2']
In this example, os.listdir()
returns a list of all files and directories in the current directory. The output is a list of file and directory names.
The os.listdir()
function is a simple yet powerful tool for listing files and directories in Python. It’s easy to use and works across all platforms, making it a versatile tool for any Python developer.
However, os.listdir()
does not handle exceptions by default. If you try to list files and directories from a non-existent directory or a directory you do not have permission to access, os.listdir()
will raise an exception. We’ll discuss how to handle these exceptions in the troubleshooting and considerations section.
In conclusion, the os
module, and in particular the os.listdir()
function, is a fundamental part of file and directory manipulation in Python. Understanding how it works and how to use it effectively is crucial for any Python developer.
Extending the Use of os.listdir()
The os.listdir()
function is not just for small scripts or one-off tasks. It can also play a crucial role in larger scripts or projects. For instance, in a file management system or a web server, you might need to list files and directories to perform operations like file upload, download, or deletion.
In such cases, os.listdir()
can be used in combination with other related functions from the os module, such as os.path.join()
, os.path.isfile()
, and os.path.isdir()
, to build more complex and robust file handling mechanisms.
Here’s an example of how to use os.listdir()
and os.path.join()
to list all files in a directory and its subdirectories recursively:
import os
def list_files_recursive(path):
for root, dirs, files in os.walk(path):
for file in files:
print(os.path.join(root, file))
list_files_recursive('.')
# Output:
# ./file1.txt
# ./file2.txt
# ./dir1/file3.txt
# ./dir2/file4.txt
In this example, os.walk()
is used to iterate over all files in a directory and its subdirectories. os.path.join()
is used to join the root directory and the file name to get the full path of each file.
Further Resources for Mastering Python’s os Module
To further your understanding and mastery of Python’s os module and the os.listdir()
function, here are some additional resources:
- Python OS Essentials: Quick Guide – Master the art of checking file existence, creating directories, and more with “os.”
Navigating Pathlib in Python – Learn how “pathlib” simplifies file and directory operations in Python.
Python File Renaming Techniques – Dive into the world of file renaming and understand renaming strategies.
Python’s os module documentation provides a comprehensive overview of Python’s OS module.
Python’s official file and directory access tutorial details the usage of the OS module for file and directory access.
Real Python’s Python files guide can help you manage your files, with examples and tips.
These resources are a great starting point for anyone looking to master file and directory manipulation in Python.
Wrapping Up: Mastering os.listdir() in Python
In this comprehensive guide, we’ve unlocked the power of Python’s os.listdir()
function, a versatile tool for listing files and directories in Python.
We started with the basics, learning how to use os.listdir()
in its simplest form to list files and directories in a specific directory. We then delved deeper, exploring more advanced uses of os.listdir()
, such as distinguishing between files and directories and manipulating them.
We also tackled common challenges you might face when using os.listdir()
, such as handling FileNotFoundError
and PermissionError
, providing solutions and workarounds for each issue.
We didn’t stop there. We also explored alternative approaches to listing files and directories in Python, comparing os.listdir()
with other methods like the glob
module and the pathlib
module.
Here’s a quick comparison of these methods:
Method | Versatility | Ease of Use | Python Version Compatibility |
---|---|---|---|
os.listdir() | High | High | All |
glob module | High (with pattern matching) | Moderate | All |
pathlib module | High (with object-oriented interface) | High | Python 3.4+ |
Whether you’re just starting out with os.listdir()
or you’re looking to enhance your file and directory manipulation skills in Python, we hope this guide has given you a deeper understanding of os.listdir()
and its capabilities.
With its simplicity, versatility, and wide compatibility, os.listdir()
is a powerful tool for listing files and directories in Python. Now, you’re well equipped to navigate the file system with Python. Happy coding!