Python Command Line Arguments: Complete Guide
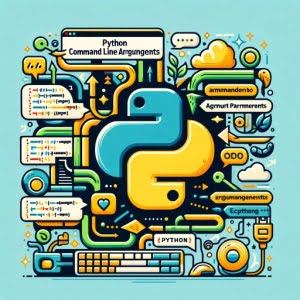
Ever felt the need to make your Python scripts more adaptable and dynamic? You’re not alone. Many developers seek ways to give their scripts a wider range of capabilities. Think of Python’s command line arguments as a Swiss army knife – versatile and handy for various tasks.
Whether you’re automating tasks, parsing data, or developing complex applications, understanding how to use command line arguments in Python can significantly enhance your coding process.
This guide will walk you through the process of using command line arguments in Python, from the basics to more advanced techniques. We’ll cover everything from the sys.argv
list, argument parsing with the argparse
module, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use Command Line Arguments in Python?
Python’s built-in
sys
module allows you to use command line arguments in your scripts via thesys.argv
list. Here’s a simple example:
import sys
print(sys.argv)
# Run your script with some arguments, and they will be printed out.
In this example, we import the sys
module and print sys.argv
, which is a list in Python that contains the command-line arguments passed to the script. With the help of sys.argv
, you can write scripts that can be customized each time they are run, without needing to change the script itself.
But that’s just the tip of the iceberg. There’s much more to learn about using command line arguments in Python. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding sys.argv in Python
- Leveling Up with argparse
- Exploring Alternatives: getopt, click, and fire
- Navigating Common Challenges with Python Command Line Arguments
- The Command Line: Python’s Powerful Ally
- Expanding Horizons: The Power of Python Command Line Arguments
- Wrapping Up: Mastering Python Command Line Arguments
Understanding sys.argv
in Python
At the heart of using command line arguments in Python is the sys.argv
list. This built-in Python list automatically captures all command-line inputs in your script.
The sys.argv
list works in a straightforward way. It’s indexed from zero, and each command line argument is a string in this list. The first item, sys.argv[0]
, is always the script name. Any arguments that follow are added to the list in the order they are provided.
Let’s take a look at a simple code example:
import sys
print('Script Name:', sys.argv[0])
for i in range(1, len(sys.argv)):
print(f'Argument {i}:', sys.argv[i])
# Run the script with command line arguments:
# python my_script.py arg1 arg2
# Output:
# Script Name: my_script.py
# Argument 1: arg1
# Argument 2: arg2
In this example, we first print the script name, which is always sys.argv[0]
. We then loop through the rest of the sys.argv
list to print out each argument. The output shows the script name and the arguments we passed in.
The sys.argv
list is a powerful tool, but it’s also basic. It doesn’t handle complex scenarios like optional arguments or flags. It also doesn’t provide any built-in error checking. If you’re not careful, this can lead to errors when your script is expecting a certain number of arguments, and it doesn’t get them.
But don’t worry! As you advance in your understanding of Python command line arguments, you’ll learn about more sophisticated tools like the argparse
module, which can handle these more complex scenarios.
Leveling Up with argparse
As you start working with more complex scripts, you’ll need more advanced ways to handle Python command line arguments. That’s where the argparse
module comes in. It’s part of Python’s standard library and provides a more sophisticated way to handle command line arguments.
Dealing with Multiple Arguments
The argparse
module makes it easy to specify the type of command-line arguments your script accepts. It automatically generates help and usage messages and throws errors when users provide invalid arguments.
Here’s an example of how to use argparse
to accept multiple arguments:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('numbers', type=int, nargs='+')
args = parser.parse_args()
print(sum(args.numbers))
# Run the script with multiple arguments:
# python my_script.py 1 2 3 4 5
# Output:
# 15
In this example, we create an argparse.ArgumentParser
instance, which will hold all the information necessary to parse the command line. We then call add_argument()
to specify which command-line options the program is expecting. In this case, it’s expecting one or more integers (nargs='+'
). The args
object returned by parse_args()
will hold the parsed arguments, and we can access the list of numbers with args.numbers
.
Optional Arguments and Flags
argparse
also allows you to specify optional arguments and flags. Here’s an example:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-v', '--verbose', action='store_true', help='increase output verbosity')
args = parser.parse_args()
if args.verbose:
print('verbosity turned on')
# Run the script with the verbose flag:
# python my_script.py -v
# Output:
# verbosity turned on
In this example, -v
or --verbose
is an optional argument. If the script is run with this flag, args.verbose
will be True
, and the message ‘verbosity turned on’ will be printed.
The argparse
module is a powerful tool for handling command line arguments in Python. It allows you to define the type of arguments your script accepts, provides helpful error messages, and even generates usage help messages.
Exploring Alternatives: getopt
, click
, and fire
While argparse
is a powerful tool for handling command line arguments in Python, it’s not the only one. There are other methods and libraries available that offer different approaches. Let’s take a look at a few of them.
The Old School: getopt
Before argparse
, there was getopt
. It’s a more traditional tool for parsing command line options and arguments, and it’s still available in Python. Here’s an example of how to use it:
import getopt
import sys
short_options = 'ho:v'
long_options = ['help', 'output=', 'verbose']
try:
arguments, values = getopt.getopt(sys.argv[1:], short_options, long_options)
except getopt.error as err:
print(str(err))
sys.exit(2)
for current_argument, current_value in arguments:
if current_argument in ('-v', '--verbose'):
print('enabling verbose mode')
elif current_argument in ('-h', '--help'):
print('displaying help')
elif current_argument in ('-o', '--output'):
print(f'enabling output mode with value: {current_value}')
# Run the script with command line arguments:
# python my_script.py -o output_value --verbose
# Output:
# enabling output mode with value: output_value
# enabling verbose mode
In this example, we define our short and long options and then use getopt.getopt()
to parse the command line arguments. getopt
also supports error handling, which we demonstrate with a try/except
block.
While getopt
is a bit more low-level than argparse
, it might be a good fit for certain use cases. However, it doesn’t automatically generate help or usage messages, and it can be a bit more complex to set up.
The Modern Choices: click
and fire
If you’re looking for something a bit more modern and flexible, you might want to check out the click
and fire
libraries. Both libraries offer a lot of functionality and can be a good choice for more complex scripts.
click
is a Python package that creates beautiful command line interfaces in a composable way. It’s highly configurable but also easy to use.
fire
is a library for automatically generating command line interfaces. With fire
, you can generate a CLI directly from any Python object, including classes, methods, and functions.
Both click
and fire
come with a lot of features and can handle complex scenarios. However, they also come with a steeper learning curve compared to argparse
or getopt
.
In conclusion, while argparse
is a great starting point for handling command line arguments in Python, it’s not the only option. Depending on your needs, getopt
, click
, or fire
might be a better fit. As always, the best tool depends on your specific use case.
As with any coding concept, there are potential pitfalls and common issues to be aware of when using Python command line arguments. In this section, we’ll discuss some of these challenges and provide solutions and tips to handle them effectively.
Handling Errors and Exceptions
One common issue is handling errors and exceptions. For instance, what happens when your script expects a command line argument but doesn’t receive one? In such cases, your script might throw an IndexError
.
Consider this example:
import sys
try:
print(sys.argv[1])
except IndexError:
print('No argument provided!')
# Run the script without any argument:
# python my_script.py
# Output:
# No argument provided!
Here, we’re trying to print sys.argv[1]
, the first command line argument. But if we run the script without any arguments, there’s no sys.argv[1]
, and an IndexError
is raised. We handle this with a try/except
block, providing a helpful message to the user instead of letting the script crash.
Type Checking and Conversion
Another common issue is dealing with the type of command line arguments. All command line arguments are passed as strings, so if your script expects an integer or a float, you’ll need to convert the argument to the correct type. If the conversion fails, you can handle the ValueError
exception.
import sys
try:
number = int(sys.argv[1])
print(number * 2)
except ValueError:
print('Please provide an integer!')
# Run the script with a non-integer argument:
# python my_script.py hello
# Output:
# Please provide an integer!
In this example, we’re trying to convert sys.argv[1]
to an integer. If the argument can’t be converted to an integer (like when we pass in the string ‘hello’), a ValueError
is raised. Again, we handle this with a try/except
block, providing a helpful message to the user.
These are just a few examples of the issues you might encounter when working with Python command line arguments. By understanding these challenges and knowing how to handle them, you can create more robust and user-friendly scripts.
The Command Line: Python’s Powerful Ally
The command line, also known as the terminal or console, is a powerful tool for developers. It allows for direct interaction with your computer’s operating system, executing commands, running scripts, and even managing files and directories.
Python’s interaction with the command line is facilitated through various built-in modules. Two of the most significant ones are sys
and argparse
. Let’s dig a little deeper into these two key players.
The sys
Module: Python’s Bridge to the Command Line
The sys
module is part of Python’s standard library and provides access to some variables used or maintained by the Python interpreter. One of its most powerful features is sys.argv
, a list in Python, which contains the command-line arguments passed to the script.
Here’s a simple example of sys.argv
in action:
import sys
for i in range(len(sys.argv)):
print(f'Argument {i}:', sys.argv[i])
# Run the script with command line arguments:
# python my_script.py arg1 arg2
# Output:
# Argument 0: my_script.py
# Argument 1: arg1
# Argument 2: arg2
In this example, we loop through the sys.argv
list and print out each argument. The output shows the script name and the arguments we passed in. This is a fundamental way of how Python interacts with the command line.
The argparse
Module: Handling Command Line Arguments Like a Pro
While sys.argv
is powerful, it’s quite basic. For more advanced handling of command line arguments, Python provides the argparse
module. It’s part of Python’s standard library and provides a more sophisticated way to handle command line arguments.
With argparse
, you can specify the type of command-line arguments your script accepts, create custom error messages, and even generate help and usage messages. It’s a significant upgrade from sys.argv
and is an excellent tool for any Python developer’s toolkit.
Understanding the command line, as well as the sys
and argparse
modules, is crucial when diving into Python command line arguments. They form the foundation upon which we can build more complex and flexible scripts.
Expanding Horizons: The Power of Python Command Line Arguments
Mastering Python command line arguments is more than just a coding exercise. It’s a stepping stone to building more robust and flexible applications. Let’s explore some of these possibilities.
Building Command Line Interfaces (CLI)
Command line arguments are the backbone of any Command Line Interface (CLI) application. They allow users to specify options and arguments to customize the behavior of your program. With Python’s argparse
module, you can build powerful and user-friendly CLIs that can compete with any native shell command.
Automating Tasks and Scripts
Python command line arguments are also a great tool for automation. Whether it’s a script that needs to run with different parameters, a task that needs to be scheduled, or a complex workflow, command line arguments can make your scripts more versatile and easier to use.
Diving Deeper: Subprocesses and Shell Scripting
If you’re interested in going even deeper, you can explore related concepts like subprocesses and shell scripting. Python’s subprocess
module allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. This can be incredibly powerful when combined with command line arguments.
Further Resources for Python Command Line Arguments Mastery
To continue your journey in mastering Python command line arguments, here are some additional resources:
- Python kwargs: Simplifying Function Arguments – Explore kwargs in Python for flexible function arguments.
Python’s official documentation on
argparse
provides a comprehensive overview of theargparse
module.Python Command Line Arguments by Real Python is a great tutorial on command line arguments.
Python Command Line Arguments Guide by GeeksforGeeks gives info on using command line arguments in Python.
Mastering Python command line arguments can open up a whole new world of possibilities for your Python scripts. So keep exploring, keep learning, and most importantly, keep coding!
Wrapping Up: Mastering Python Command Line Arguments
In this comprehensive guide, we’ve delved deep into the world of Python command line arguments, exploring their usage, benefits, and potential pitfalls.
We began with the basics, learning how to use Python’s built-in sys
module and the sys.argv
list to handle command line arguments. We then ventured into more advanced territory, exploring the argparse
module and its capabilities in handling multiple arguments, optional arguments, and flags.
Along the way, we tackled common challenges you might face when working with Python command line arguments, such as handling errors and exceptions, type checking, and conversion. We provided solutions and tips to help you navigate these issues effectively.
We also looked at alternative approaches to handling command line arguments, comparing Python’s argparse
with other methods and libraries like getopt
, click
, and fire
. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | Learning Curve |
---|---|---|---|
argparse | High | Moderate | Moderate |
getopt | Moderate | High | High |
click and fire | High | Low | Low |
Whether you’re a beginner just starting out with Python command line arguments or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python command line arguments and their capabilities.
With their balance of flexibility and power, Python command line arguments are a valuable tool for any Python developer. Happy coding!