Python kwargs: Guide to Keyword Arguments
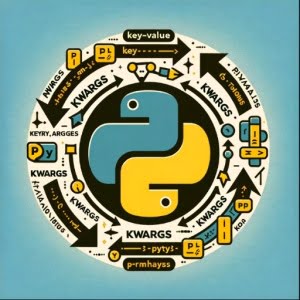
Are you finding it difficult to understand kwargs in Python? You’re not alone. Many Python developers find kwargs to be a complex concept. Think of kwargs as a Swiss army knife – versatile and powerful, providing your Python functions with a level of flexibility that might seem daunting at first.
In this guide, we’ll walk you through the process of using kwargs in Python, from the basics to more advanced techniques. We’ll cover everything from defining a function that accepts kwargs, passing kwargs to a function, accessing the values of kwargs within the function, and even discuss common issues and their solutions.
Let’s dive in and start mastering kwargs in Python!
TL;DR: What are kwargs in Python and how do I use them?
kwargs, short for keyword arguments, allow you to pass a variable number of arguments to a function, where each argument is a key-value pair with the **kwargs operator. This provides your Python functions with a level of flexibility and power.
Here’s a simple example:
def greet(**kwargs):
for key, value in kwargs.items():
print(f'{key}: {value}')
greet(name='John', age=25)
# Output:
# name: John
# age: 25
In this example, we define a function greet()
that accepts any number of keyword arguments. We then call this function with two keyword arguments: name
and age
. Inside the function, we loop over the kwargs dictionary and print out each key-value pair.
This is just the tip of the iceberg when it comes to using kwargs in Python. Keep reading for a more detailed explanation and advanced usage examples.
Table of Contents
- Defining and Using kwargs in Python Functions
- Enhancing Function Flexibility with kwargs
- Exploring Alternative Concepts: *args, Argument Unpacking, and Function Decorators
- Overcoming Common Issues with Python kwargs
- Delving Deeper: Understanding Argument Passing in Python
- Expanding kwargs Usage in Object-Oriented Programming
- Wrapping Up: Mastering kwargs in Python
Defining and Using kwargs in Python Functions
In Python, kwargs (keyword arguments) allow you to pass a variable number of arguments to a function. This is especially useful when you don’t know in advance how many arguments will be passed to your function.
Defining a Function that Accepts kwargs
To define a function that accepts kwargs, you use the double asterisk **
before the parameter name. This tells Python that the function can accept any number of keyword arguments. Here’s an example:
def greet(**kwargs):
for key, value in kwargs.items():
print(f'{key}: {value}')
In this code, greet()
is a function that accepts any number of keyword arguments. Inside the function, we loop over the kwargs dictionary and print out each key-value pair.
Passing kwargs to a Function
To pass kwargs to a function, you simply specify the keyword arguments when calling the function. Here’s how you can call the greet()
function with two keyword arguments:
greet(name='John', age=25)
# Output:
# name: John
# age: 25
In this code, we’re calling the greet()
function and passing two keyword arguments: name
and age
. The function then prints out each key-value pair.
Accessing kwargs within a Function
Inside the function, kwargs are treated as a dictionary. You can access the values of kwargs by using the keys. In the greet()
function, we’re using a for loop to iterate over the kwargs dictionary and print out each key-value pair.
This basic usage of kwargs in Python gives your functions a high level of flexibility, allowing them to handle a variable number of arguments. As you’ll see in the next sections, kwargs can be used in more advanced ways to further enhance your Python programming.
Enhancing Function Flexibility with kwargs
As we delve deeper into the power of kwargs in Python, we’ll discover how they can be used with positional arguments and default arguments. We’ll also explore how to pass a dictionary as kwargs and how to use kwargs in function calls.
Using kwargs with Positional Arguments
In Python, you can use kwargs together with positional arguments. Positional arguments are arguments that can be called by their position in the function definition. Here’s an example:
def greet(greeting, **kwargs):
for key, value in kwargs.items():
print(f'{greeting}, {key}: {value}')
greet('Hello', name='John', age=25)
# Output:
# Hello, name: John
# Hello, age: 25
In this code, the greet()
function accepts a positional argument greeting
and any number of kwargs. When we call the function, we pass a string ‘Hello’ as the positional argument and two keyword arguments name
and age
.
Using kwargs with Default Arguments
Similarly, kwargs can be used with default arguments. Default arguments are arguments that take a default value if no argument value is passed during the function call. Here’s an example:
def greet(greeting='Hello', **kwargs):
for key, value in kwargs.items():
print(f'{greeting}, {key}: {value}')
greet(name='John', age=25)
# Output:
# Hello, name: John
# Hello, age: 25
In this code, the greet()
function has a default argument greeting
with a default value ‘Hello’, and any number of kwargs. If we don’t pass a value for greeting
when calling the function, it will use the default value ‘Hello’.
Passing a Dictionary as kwargs
You can also pass a dictionary as kwargs. This is useful when you have a dictionary of data that you want to pass to a function as keyword arguments. Here’s how you can do this:
def greet(**kwargs):
for key, value in kwargs.items():
print(f'{key}: {value}')
person = {'name': 'John', 'age': 25}
greet(**person)
# Output:
# name: John
# age: 25
In this code, we have a dictionary person
. When we call the greet()
function, we pass the person
dictionary as kwargs by using the double asterisk **
before the dictionary name. The function then prints out each key-value pair in the dictionary.
Using kwargs in Function Calls
Finally, kwargs can be used in function calls to pass keyword arguments to the function. This is especially useful when you’re working with functions that accept a variable number of keyword arguments. Here’s an example:
def greet(**kwargs):
for key, value in kwargs.items():
print(f'{key}: {value}')
greet(name='John', age=25)
# Output:
# name: John
# age: 25
In this code, we’re calling the greet()
function and passing two keyword arguments: name
and age
. The function then prints out each key-value pair.
As you can see, kwargs provide a powerful way to make your Python functions more flexible and dynamic. By understanding how to use kwargs in different scenarios, you can write more efficient and versatile Python code.
Exploring Alternative Concepts: *args, Argument Unpacking, and Function Decorators
While kwargs provide a powerful tool for enhancing the flexibility of your Python functions, they’re not the only game in town. By understanding related concepts like *args, argument unpacking, and function decorators, you can further increase your Python prowess.
The Power of *args
In Python, *args allow you to pass a variable number of non-keyword arguments to a function. Here’s an example:
def sum_numbers(*args):
return sum(args)
print(sum_numbers(1, 2, 3, 4, 5))
# Output:
# 15
In this code, sum_numbers()
is a function that accepts any number of non-keyword arguments. It sums these arguments and returns the result. This approach is beneficial when you don’t know in advance how many arguments will be passed to your function.
Understanding Argument Unpacking
Argument unpacking is a technique in Python that allows you to unpack the values from a list or dictionary and pass them to a function as separate arguments. Here’s how you can do this:
def greet(name, age):
print(f'Name: {name}, Age: {age}')
person = ['John', 25]
greet(*person)
# Output:
# Name: John, Age: 25
In this code, we have a list person
. When we call the greet()
function, we pass the person
list as separate arguments by using the single asterisk *
before the list name. The function then prints out each argument.
Leveraging Function Decorators
Function decorators allow you to wrap a function with another function, enhancing its functionality. This is useful when you want to add a common behavior to multiple functions. Here’s an example:
def decorator(func):
def wrapper(**kwargs):
print('Before function call')
func(**kwargs)
print('After function call')
return wrapper
@decorator
def greet(name):
print(f'Hello, {name}')
greet(name='John')
# Output:
# Before function call
# Hello, John
# After function call
In this code, decorator()
is a function that accepts another function and returns a new function that wraps the original function. When we call the greet()
function, which is decorated by decorator()
, it first prints ‘Before function call’, then calls the original function, and finally prints ‘After function call’.
These alternative approaches provide additional tools for enhancing the flexibility and functionality of your Python functions. By understanding their benefits and drawbacks, you can make better decisions about when to use each approach.
Overcoming Common Issues with Python kwargs
As with any programming concept, using kwargs in Python may present some challenges. However, understanding these common issues and their solutions can help you write more robust Python code.
TypeError: Passing a Non-Dictionary as kwargs
One common error is trying to pass a non-dictionary as kwargs. This will result in a TypeError. For example:
def greet(**kwargs):
for key, value in kwargs.items():
print(f'{key}: {value}')
person = ['John', 25]
greet(**person)
# Output:
# TypeError: greet() argument after ** must be a mapping, not list
In this code, we’re trying to pass a list person
as kwargs, which results in a TypeError because kwargs expects a dictionary. The solution is to ensure that you’re passing a dictionary as kwargs.
SyntaxError: Using a Keyword Argument Before a Positional Argument
Another common issue is using a keyword argument before a positional argument. This will result in a SyntaxError. For example:
def greet(name, **kwargs):
print(f'Name: {name}')
for key, value in kwargs.items():
print(f'{key}: {value}')
greet(name='John', 'Hello')
# Output:
# SyntaxError: positional argument follows keyword argument
In this code, we’re trying to pass a positional argument ‘Hello’ after a keyword argument name
, which results in a SyntaxError. The solution is to ensure that positional arguments are specified before keyword arguments.
Understanding these common issues and their solutions can help you avoid pitfalls when using kwargs in Python. By being aware of these potential obstacles, you can write more effective and error-free Python code.
Delving Deeper: Understanding Argument Passing in Python
In Python, arguments are the values we pass to a function when calling it. There are several types of arguments you can use, and understanding each is crucial to mastering Python programming.
Positional Arguments
Positional arguments are arguments that need to be passed in the same order as they are defined in the function. For example:
def greet(name, age):
print(f'Name: {name}, Age: {age}')
greet('John', 25)
# Output:
# Name: John, Age: 25
In this code, name
and age
are positional arguments. When calling the greet()
function, we need to pass the arguments in the same order: first name
, then age
.
Default Arguments
Default arguments are arguments that take a default value if no argument value is passed during the function call. For example:
def greet(name='John'):
print(f'Name: {name}')
greet()
# Output:
# Name: John
In this code, name
is a default argument with a default value ‘John’. If we don’t pass a value for name
when calling the greet()
function, it will use the default value ‘John’.
Variable-Length Arguments
Variable-length arguments are arguments that allow you to pass a variable number of arguments to a function. These include *args for non-keyword arguments and **kwargs for keyword arguments. We’ve already seen examples of these in previous sections.
Keyword Arguments
Keyword arguments are arguments that are identified by the keyword used when passing them to a function. This is where **kwargs come into play, as we’ve discussed throughout this guide.
Understanding the differences between these types of arguments and knowing when to use each can greatly enhance your Python programming skills. Remember, the key is to choose the right type of argument for the right situation.
Expanding kwargs Usage in Object-Oriented Programming
While we’ve explored the use of kwargs in Python functions, their utility extends beyond functions and into the realm of object-oriented programming. Exploring their application in class constructors, method overriding, and related concepts like function overloading and polymorphism can provide a deeper understanding of kwargs.
Leveraging kwargs in Class Constructors
In object-oriented programming, kwargs can be used in class constructors to initialize object attributes. Here’s an example:
class Person:
def __init__(self, **kwargs):
for key, value in kwargs.items():
setattr(self, key, value)
person = Person(name='John', age=25)
print(person.name)
print(person.age)
# Output:
# John
# 25
In this code, we define a Person
class with a constructor that accepts kwargs. We then create a Person
object and pass two keyword arguments: name
and age
. The constructor sets these as attributes of the object.
Overriding Methods with kwargs
kwargs can also be used to override methods in subclasses. This can be useful when you want to change the behavior of a method in a subclass without changing its interface.
Exploring Related Concepts: Function Overloading and Polymorphism
Function overloading, a concept where two or more functions can have the same name but different parameters, isn’t directly supported in Python. However, kwargs can provide similar functionality by allowing a function to accept a variable number of arguments.
Polymorphism, a concept where an object can take on many forms, can also be achieved with kwargs. By passing different keyword arguments, you can change the behavior of a function or method.
Further Resources for Python kwargs Mastery
To continue your journey towards mastering kwargs in Python, check out these resources:
- IOFlood’s Python Command Line Arguments Article – Learn Python argparse for advanced argument parsing.
Environment Variable Configuration with Python dotenv – Learn how to securely store sensitive information in .env files.
Python Environment Variables: Managing Configuration – Dive into managing environment variables in Python.
Python’s official documentation on defining functions provides an explanation of function definitions as well as **kwargs.
Real Python’s guide on Python’s functions offers a practical approach to using Python’s functions, including **kwargs.
Python Tips’ article on *args and **kwargs provides a clear and concise explanation of these special symbols in Python.
Wrapping Up: Mastering kwargs in Python
In this comprehensive guide, we’ve journeyed through the world of kwargs in Python, a powerful tool that gives your functions a high level of flexibility.
We began with the basics, learning how to define a function that accepts kwargs, how to pass kwargs to a function, and how to access the values of kwargs within the function. We then ventured into more advanced territory, exploring how to use kwargs with positional and default arguments, how to pass a dictionary as kwargs, and how to use kwargs in function calls.
Along the way, we tackled common issues you might face when using kwargs, such as TypeError when passing a non-dictionary as kwargs, or SyntaxError when using a keyword argument before a positional argument, providing you with solutions for each issue.
We also looked at alternative approaches to using kwargs, exploring related concepts like *args, argument unpacking, and function decorators. Here’s a quick comparison of these concepts:
Concept | Usage | Flexibility |
---|---|---|
**kwargs | Pass variable number of keyword arguments to a function | High |
*args | Pass variable number of non-keyword arguments to a function | Moderate |
Argument Unpacking | Unpack values from a list or dictionary and pass them to a function as separate arguments | High |
Function Decorators | Wrap a function with another function to enhance its functionality | High |
Whether you’re a beginner just starting out with kwargs or an experienced Python developer looking to level up your skills, we hope this guide has given you a deeper understanding of kwargs and its capabilities.
With its balance of flexibility and power, kwargs is a crucial tool for Python programming. Happy coding!