Python Environment Variables Guide
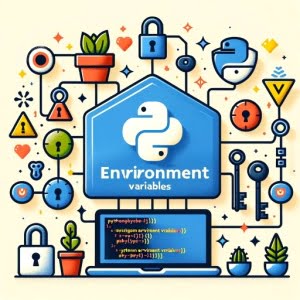
Ever found yourself puzzled about managing environment variables in Python? You’re not alone. Many developers find themselves in a maze when it comes to handling environment variables in Python. But don’t worry, we’re here to help.
Think of Python’s environment variables as a secret agent. They can handle sensitive data securely, keeping your secrets safe from prying eyes. They are a powerful tool that can help you manage configuration settings and other information that you don’t want to hard-code into your Python scripts.
In this guide, we’ll walk you through the process of managing environment variables in Python, from the basics to more advanced techniques. We’ll cover everything from setting and getting environment variables using the os.environ
object, to using environment variables in different contexts (e.g., development vs. production), and even alternative approaches for managing environment variables.
So, let’s dive in and start mastering Python environment variables!
TL;DR: How Do I Set and Get Environment Variables in Python?
To set and get environment variables in Python, you can use the
os
module’senviron
object. Here’s a simple example:
import os
os.environ['TEST'] = 'Hello World'
print(os.environ['TEST'])
# Output:
# 'Hello World'
In this example, we first import the os
module. Then, we use os.environ
to set an environment variable named ‘TEST’ with the value ‘Hello World’. Finally, we print the value of the ‘TEST’ environment variable, which outputs ‘Hello World’.
This is a basic way to set and get environment variables in Python, but there’s much more to learn about managing environment variables in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Python Environment Variables: Basic Usage
- Python Environment Variables: Advanced Usage
- Alternative Methods for Managing Python Environment Variables
- Troubleshooting Python Environment Variables
- Unraveling Python Environment Variables
- Python Environment Variables: The Bigger Picture
- Wrapping Up: Mastering Python Environment Variables
Python Environment Variables: Basic Usage
Python offers a straightforward way to set and get environment variables using the os.environ
object. It’s a mapping object representing the string environment. Here’s how it works:
import os
os.environ['TEST'] = 'Hello Python'
print(os.environ['TEST'])
# Output:
# 'Hello Python'
In the code block above, we first import the os
module. Then, we use os.environ
to set an environment variable named ‘TEST’ with the value ‘Hello Python’. Finally, we print the value of the ‘TEST’ environment variable, which outputs ‘Hello Python’.
Advantages of Using os.environ
The primary advantage of using os.environ
is its simplicity. With just a couple of lines of code, you can set and retrieve environment variables. This approach is particularly useful for managing configuration settings and other sensitive information that you don’t want to hard-code into your Python scripts.
Potential Pitfalls of Using os.environ
While os.environ
is a powerful tool, it’s not without its potential pitfalls. One key thing to remember is that os.environ
only affects the current process where it’s used. If you set an environment variable using os.environ
in a Python script, that environment variable will not be available in other processes or in your system’s environment variables.
Another potential pitfall is that os.environ
does not provide any mechanism for persisting environment variables. When the Python process ends, any environment variables set using os.environ
will be lost.
Despite these potential pitfalls, os.environ
remains a valuable tool for managing environment variables in Python. In the following sections, we’ll explore more advanced usage scenarios and alternative approaches for managing environment variables.
Python Environment Variables: Advanced Usage
As you become more comfortable with Python environment variables, you may find yourself needing to use them in different contexts, such as development versus production environments, or needing to handle sensitive data. Let’s delve into these advanced scenarios.
Environment Variables in Different Contexts
In a real-world application, you’ll often have different environments like development, testing, and production. Each of these environments may require different settings. Python environment variables can be a powerful tool in managing these different contexts.
For example, consider a scenario where you have a different database for development and production. You can use environment variables to manage the database URL.
import os
db_url = os.environ.get('DB_URL', 'default_db_url')
print(db_url)
# Output (assuming DB_URL is not set):
# 'default_db_url'
In this example, we’re using os.environ.get
to retrieve the value of the ‘DB_URL’ environment variable. If ‘DB_URL’ is not set, it will return ‘default_db_url’. This way, you can set ‘DB_URL’ to the appropriate database URL in each environment.
Handling Sensitive Data
Python environment variables can also be useful for handling sensitive data, such as API keys or passwords. By storing these values as environment variables, you can keep them out of your code and avoid accidentally committing them to version control.
import os
api_key = os.environ['API_KEY']
print('API key retrieved successfully')
# Output:
# 'API key retrieved successfully'
In this example, we’re using os.environ
to retrieve the value of the ‘API_KEY’ environment variable. Note that we’re not printing the actual API key to avoid exposing it.
Advantages and Pitfalls
Using environment variables for different contexts and handling sensitive data has several advantages. It improves the security of your application, keeps your code clean, and makes it easier to manage configuration settings. However, it also comes with potential pitfalls.
One potential pitfall is that you need to ensure that the environment variables are properly set in each environment. If an environment variable is not set, it could cause your application to behave unexpectedly or even crash.
Another potential pitfall is that environment variables can be harder to debug compared to hard-coded values. If there’s an issue with an environment variable, it might not be immediately obvious since the value is not visible in the code.
Despite these potential pitfalls, using environment variables for different contexts and handling sensitive data is a powerful technique that can greatly improve the quality of your Python applications.
Alternative Methods for Managing Python Environment Variables
As you delve deeper into Python programming, you might find situations where the built-in os.environ
object might not suffice. Fear not, Python offers additional methods to manage environment variables, including using third-party libraries and configuration files.
Using Third-Party Libraries
There are several third-party libraries available that provide enhanced functionality for managing environment variables in Python. One of the most popular is python-dotenv. This library allows you to specify your environment variables in a .env file, which python-dotenv can then load into os.environ
.
Here’s a simple example of how to use python-dotenv:
from dotenv import load_dotenv
load_dotenv()
api_key = os.getenv('API_KEY')
print('API key retrieved successfully')
# Output:
# 'API key retrieved successfully'
In this example, load_dotenv()
loads the environment variables from the .env file. os.getenv('API_KEY')
then retrieves the value of the ‘API_KEY’ environment variable.
Using Configuration Files
Another approach is to use configuration files to manage your environment variables. This can be particularly useful if you have a large number of environment variables or if you want to organize your environment variables into sections.
Python’s built-in configparser module can be used to read and write data from and to configuration files. Here’s an example of how to use configparser to manage environment variables:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
db_url = config['DATABASE']['DB_URL']
print('Database URL retrieved successfully')
# Output:
# 'Database URL retrieved successfully'
In this example, configparser.ConfigParser()
creates a new ConfigParser object. config.read('config.ini')
reads the configuration file ‘config.ini’. config['DATABASE']['DB_URL']
then retrieves the value of the ‘DB_URL’ environment variable under the ‘DATABASE’ section.
Advantages and Disadvantages of Alternative Methods
These alternative methods for managing Python environment variables come with their own advantages and disadvantages. Third-party libraries like python-dotenv can provide enhanced functionality and ease of use. However, they also introduce additional dependencies to your project.
Configuration files can provide a high level of organization and flexibility, especially for large projects. However, they can also be harder to manage compared to environment variables, particularly if you have multiple configuration files or if your configuration files are complex.
Despite these potential disadvantages, these alternative methods can be powerful tools for managing Python environment variables, particularly for complex or large-scale projects.
Troubleshooting Python Environment Variables
As you work with Python environment variables, you might encounter some common issues or challenges. Here, we’ll discuss some of these potential problems and provide solutions to help you navigate them.
Environment Variable Not Set
One common issue is trying to access an environment variable that has not been set. This will raise a KeyError
.
import os
try:
print(os.environ['UNSET_VARIABLE'])
except KeyError:
print('Environment variable not set')
# Output:
# 'Environment variable not set'
In this example, we’re trying to access an environment variable ‘UNSET_VARIABLE’ that has not been set, which raises a KeyError
. We catch this error and print a message indicating that the environment variable is not set.
To avoid this issue, you can use os.environ.get()
, which will return None
if the environment variable is not set. Alternatively, you can set a default value to be returned if the environment variable is not set.
import os
print(os.environ.get('UNSET_VARIABLE', 'Default Value'))
# Output:
# 'Default Value'
Environment Variable Persistence
Another common issue is expecting environment variables set with os.environ
to persist across sessions or to be available in other scripts. As we discussed earlier, environment variables set with os.environ
are only available in the current process and will not persist once the process ends.
If you need to set environment variables that will be available in other scripts or that will persist across sessions, you’ll need to set them in your system’s environment variables or use a tool like python-dotenv.
Best Practices for Managing Python Environment Variables
When managing Python environment variables, there are a few best practices to keep in mind:
- Avoid hard-coding sensitive information: Use environment variables to manage sensitive information like API keys or passwords. This keeps this information out of your code and makes it easier to manage.
Use descriptive names for your environment variables: This makes it easier to understand what each environment variable is used for.
Use
os.environ.get()
to avoidKeyError
: This allows you to specify a default value to be returned if an environment variable is not set.
By keeping these common issues and best practices in mind, you can effectively manage Python environment variables in your projects.
Unraveling Python Environment Variables
To truly master Python environment variables, we need to start from the ground up. Let’s take a deep dive into the concept of environment variables, why they’re used, and how they operate within a Python application.
What are Environment Variables?
Environment variables are dynamic-named values that can affect the way running processes behave on a computer. They exist in every operating system, and Python provides methods to read them and set them.
import os
print(os.environ)
# Output:
# Environ({...})
In the code block above, os.environ
gives us a dictionary-like object representing our current environment variables. The output will be a list of all your system’s environment variables and their values.
Why Use Environment Variables?
Environment variables are particularly useful for the following reasons:
- Configuration: They can be used to set configuration options for your application, such as the location of your database or the port your server should run on.
Security: They allow sensitive data, like API keys or passwords, to be stored securely outside your application’s code.
Portability: They make your applications more portable, allowing them to be run on any machine without modification.
Python and Environment Variables
In Python, the os
module provides a way of using operating system dependent functionality, including accessing environment variables. The os.environ
object is the way to access environment variables. It’s a dictionary-like object that allows you to interact with the underlying operating system’s environment variables.
By understanding the concept of environment variables and how they work in the context of a Python application, we can leverage them to make our applications more secure, configurable, and portable.
Python Environment Variables: The Bigger Picture
As your Python projects grow in size and complexity, the importance of environment variables becomes even more pronounced. They can play a crucial role in managing configuration settings, ensuring the security of sensitive data, and enhancing the portability of your applications.
Environment Variables in Larger Python Projects
In larger Python projects, environment variables can be used to manage a wide range of configuration settings. This might include database settings, API keys, secret keys for encryption, and much more. They can help to keep your codebase clean and maintainable, by separating configuration from code.
Moreover, environment variables can be critical for security. By storing sensitive data in environment variables, you can keep it out of your codebase and prevent it from being exposed in logs or version control systems.
Further Resources for Python Environment Variable Mastery
To continue your journey in mastering Python environment variables, consider exploring these resources:
- IOFlood’s Python Command Line Arguments Guide – Validate and process user inputs from the command line.
Using kwargs for Flexible Function Definitions in Python – Master Python’s **kwargs for dynamic parameter handling.
Simplifying Environment Variable Access in Python – Explore methods for safely handling missing environment variables.
Python’s official documentation on the os module is a comprehensive resource on all functionalities of the os module.
The Twelve-Factor App’s config section – While not Python-specific, it provides a deep-dive into app configuration and using environment variables.
Python Args Tutorial on YouTube – Enhance your skills in handling command line arguments in Python with this video tutorial.
Remember, mastering Python environment variables is not just about knowing the syntax. It’s about understanding how to use them effectively to make your applications more secure, maintainable, and portable.
Wrapping Up: Mastering Python Environment Variables
In this comprehensive guide, we’ve explored the intricacies of managing Python environment variables. We’ve delved into the basics, advanced usage scenarios, and even alternative methods, equipping you with the knowledge to effectively handle environment variables in your Python projects.
We began with the basics, understanding how to set and get environment variables using the os.environ
object. We then delved into more advanced usage, exploring how to use environment variables in different contexts and how to handle sensitive data. We also explored alternative methods for managing Python environment variables, including third-party libraries and configuration files.
Along the way, we tackled common challenges that you may face when working with Python environment variables, providing solutions and best practices to help you navigate these potential pitfalls. We also discussed the importance of environment variables in larger Python projects and provided resources for further learning.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
os.environ | Simple, direct access to environment variables | Does not persist across sessions |
Third-party libraries (e.g., python-dotenv) | Enhanced functionality, ease of use | Additional dependencies |
Configuration files | High level of organization for large projects | Can be harder to manage |
Whether you’re just starting out with Python environment variables, or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Python environment variables and their importance in Python programming.
The ability to effectively manage Python environment variables is a powerful tool in your Python programming arsenal. Now, you’re well equipped to handle any environment variable challenges that come your way. Happy coding!