Python: Get Environment Variables Step-by-Step
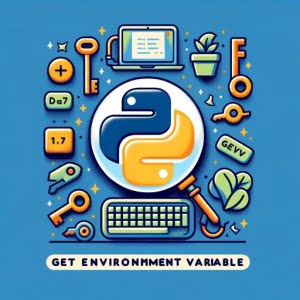
Are you finding it difficult to access environment variables in Python? You’re not alone. Many developers find themselves puzzled when it comes to retrieving these hidden pieces of information from their system.
Think of Python’s ability to get environment variables as a secret agent’s capability to extract classified data. It’s a powerful tool that can provide your Python scripts with valuable information about the system they’re running on.
In this guide, we’ll explore how to get environment variables in Python, starting from the basics and moving on to more advanced techniques. We’ll cover everything from using the os.environ
object, handling non-existent variables, to discussing alternative approaches.
So, let’s dive in and start mastering how to retrieve environment variables in Python!
TL;DR: How Do I Get an Environment Variable in Python?
To get an environment variable in Python, you use the
os.environ
object. This object is a dictionary-like object that allows you to interact with your system’s environment variables.
Here’s a simple example:
import os
var = os.environ['MY_VARIABLE']
print(var)
# Output:
# 'Value of MY_VARIABLE'
In this example, we import the os
module and use the os.environ
object to access the value of the environment variable ‘MY_VARIABLE’. The value of ‘MY_VARIABLE’ is then printed to the console.
This is just a basic way to get environment variables in Python. There’s much more to learn about accessing and manipulating environment variables in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Understanding the os.environ
Object
Python provides the os
module, which includes a variety of functions to interact with the operating system, including the os.environ
object. This object is a dictionary-like object that allows you to interact with your system’s environment variables.
Accessing Environment Variables with os.environ
To access an environment variable in Python, you can use the os.environ
object like a dictionary. Here’s an example:
import os
# Access an environment variable
var = os.environ['MY_VARIABLE']
print(var)
# Output:
# 'Value of MY_VARIABLE'
In this example, we’re importing the os
module, accessing the environment variable ‘MY_VARIABLE’ using os.environ
, and printing its value.
Advantages and Potential Pitfalls
Using os.environ
is straightforward and easy, making it a great option for beginners. However, there are potential pitfalls. If you try to access a non-existent environment variable using os.environ
, Python will raise a KeyError
. This is something you’ll need to handle in your code, especially if there’s a chance the environment variable might not exist.
In the next section, we’ll discuss how to handle non-existent environment variables and introduce the os.getenv()
function.
Handling Non-Existent Environment Variables
When dealing with environment variables, it’s crucial to handle the case where an environment variable might not exist. As we saw in the previous section, trying to access a non-existent variable using os.environ
results in a KeyError
. But there’s a safer way to access environment variables: the os.getenv()
function.
Using the os.getenv()
Function
The os.getenv()
function is a more forgiving method for accessing environment variables. Instead of raising a KeyError
when a variable doesn’t exist, os.getenv()
simply returns None
. Here’s how you use it:
import os
# Use os.getenv() to access an environment variable
var = os.getenv('MY_VARIABLE')
print(var)
# Output:
# 'Value of MY_VARIABLE' or None if 'MY_VARIABLE' does not exist
In this example, if ‘MY_VARIABLE’ exists, its value is printed. If ‘MY_VARIABLE’ doesn’t exist, None
is printed instead.
os.environ
vs os.getenv()
: Which to Use?
Both os.environ
and os.getenv()
allow you to access environment variables, but they handle non-existent variables differently. If your code assumes that an environment variable will always exist, you might prefer os.environ
because it will raise an error if the variable doesn’t exist. On the other hand, if your code can handle an environment variable being None
, os.getenv()
is a safer option.
In the next section, we’ll explore additional methods for getting environment variables in Python.
Exploring Alternative Approaches
While os.environ
and os.getenv()
are commonly used to access environment variables in Python, there are other methods worth considering. One such method is the os.environ.get()
function.
The os.environ.get()
Method
The os.environ.get()
method is similar to os.getenv()
. It returns the value of the specified environment variable if it exists. If it doesn’t, it returns None
or a default value if you provide one. Here’s how you can use it:
import os
# Use os.environ.get() to access an environment variable
var = os.environ.get('MY_VARIABLE', 'default_value')
print(var)
# Output:
# 'Value of MY_VARIABLE' or 'default_value' if 'MY_VARIABLE' does not exist
In this example, if ‘MY_VARIABLE’ exists, its value is printed. If ‘MY_VARIABLE’ doesn’t exist, ‘default_value’ is printed instead.
Comparing the Methods
All three methods (os.environ
, os.getenv()
, os.environ.get()
) allow you to access environment variables, but they have different behaviors when the variable doesn’t exist:
os.environ
: Raises aKeyError
os.getenv()
: ReturnsNone
os.environ.get()
: ReturnsNone
or a default value
Choosing the right method depends on your specific needs. If you want to ensure an environment variable exists, os.environ
might be the best choice. If you want to avoid exceptions and can handle a None
value, os.getenv()
or os.environ.get()
would be more suitable.
Troubleshooting Common Issues
While working with environment variables in Python, you may encounter some common issues. Let’s discuss these and provide solutions and workarounds.
Handling ‘KeyError’
As mentioned earlier, trying to access a non-existent environment variable using os.environ
raises a KeyError
. Here’s an example of what this looks like:
import os
try:
var = os.environ['NON_EXISTENT_VARIABLE']
except KeyError:
print('Variable does not exist')
# Output:
# 'Variable does not exist'
In this example, we’re trying to access a variable that doesn’t exist (‘NON_EXISTENT_VARIABLE’). Python raises a KeyError
, which we catch and handle by printing a message.
To avoid this error, you can use the os.getenv()
function or the os.environ.get()
method. Both of these return None
instead of raising an error when the variable doesn’t exist.
Tips for Working with Environment Variables
Here are some additional tips for working with environment variables in Python:
- Always check if an environment variable exists before trying to access it.
- Use
os.getenv()
oros.environ.get()
to safely access environment variables. - Remember that environment variables are case-sensitive.
- Be aware that environment variables can change between different runs of your program, especially if your program is being run on different systems or under different user accounts.
Unveiling Environment Variables
Before we delve further into the world of Python and environment variables, let’s take a step back and understand what environment variables are and why they are important.
What are Environment Variables?
Environment variables are dynamic, named values stored in the operating system. They can affect the way running processes behave on a computer. For example, they can contain information about the file system, system locale, and operating system details.
Here’s an example of accessing an environment variable in Python:
import os
# Access the 'PATH' environment variable
path = os.getenv('PATH')
print(path)
# Output:
# '/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin'
In this example, we’re accessing the ‘PATH’ environment variable, which is a common environment variable that lists directories the operating system should look in when it needs to run a command.
Why Use Environment Variables?
Environment variables serve several purposes. They can:
- Provide a way to influence the behavior of software on the system.
- Store system-wide settings, like the paths to system folders.
- Store application settings or user preferences.
In Python, environment variables can be accessed using the os
module, as we’ve seen in the examples above. They are essential for developing applications that are platform-independent and can be configured without changing the application’s code.
In the following section, we will explore the relevance of environment variables in configuration management, security, and other applications.
Python Environment Variables: Beyond the Basics
Environment variables in Python are not just about accessing system information. They carry a significant role in configuration management, security, and many other aspects of application development.
Configuration Management with Environment Variables
Environment variables are commonly used in configuration management. They can store configuration values that your application can access. This way, you can change the behavior of your application without modifying the code. For example, you might use an environment variable to store the path to a database or the hostname of a server.
Security Considerations
Environment variables can also play a role in security. They can store sensitive information, like API keys or database passwords, keeping this information out of your code. However, it’s important to manage these variables securely to avoid exposing sensitive information.
Setting Environment Variables in Python
In addition to getting environment variables, Python can also set environment variables. This is done using the os.environ
object. Here’s an example:
import os
# Set an environment variable
os.environ['MY_VARIABLE'] = 'my value'
# Verify that the variable was set
print(os.getenv('MY_VARIABLE'))
# Output:
# 'my value'
In this example, we’re setting the environment variable ‘MY_VARIABLE’ to ‘my value’, then verifying that the variable was set correctly.
Further Resources for Python Environment Variables Mastery
If you’re interested in learning more about environment variables in Python, here are some resources to explore:
- Beginner’s Guide to Python Command Line Arguments – Get hands-on with Python CLI interfaces and sys.argv.
Configuration Management with Python Environment Variables – Learn how environment variables influence Python programs.
Python Command-Line Argument Parsing with argparse – Learn how argparse simplifies building CLI applications.
Official Python Documentation on the os Module – Dive into Python’s native operating system interface.
Environment Variables in Python – Understand the function and implementation of environment variables in Python.
Python and Environment Variables – Learn from video instructions about tackling environment variables in Python.
Setting and Getting Environment Variables in Python – Learn how to effectively use environment variables in Python.
Wrapping Up: Mastering Python Environment Variables
In this comprehensive guide, we’ve covered the ins and outs of how to get environment variables in Python. We’ve explored the role of environment variables, their significance in configuration management, and how they impact security.
We began with the basics, learning how to use the os.environ
object to access environment variables. We then moved on to handle non-existent variables, introducing the os.getenv()
function and illustrating its usage.
Along the way, we addressed common issues you might encounter when working with environment variables in Python, such as handling ‘KeyError’. We provided solutions and workarounds for each issue to help you tackle these challenges effectively.
To equip you with more tools, we also discussed alternative approaches, such as using the os.environ.get()
method.
Here’s a quick comparison of the methods we’ve discussed:
Method | Behavior when variable doesn’t exist |
---|---|
os.environ | Raises a KeyError |
os.getenv() | Returns None |
os.environ.get() | Returns None or a default value |
Whether you’re just starting out with Python or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to get environment variables in Python.
Understanding how to work with environment variables is a valuable skill in Python programming, allowing you to write more flexible and robust code. Now, you’re well equipped to handle environment variables in your Python projects. Happy coding!