Mypy | Guide To Python Static Type Checking
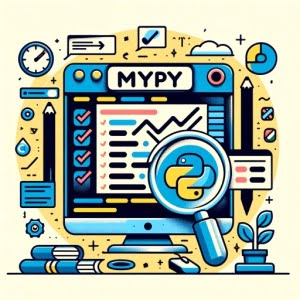
Are you tired of encountering unexpected errors in your Python code? You’re not alone. Many Python developers find themselves in a constant battle with bugs that could have been avoided with a more proactive approach.
Think of Mypy as your personal code guardian, a static type checker for Python that can catch common errors even before you run your code. It’s like having a vigilant guard, always on the lookout for potential issues that could derail your program.
In this guide, we’ll provide a comprehensive overview of Mypy, covering everything from basic use to advanced techniques. We’ll explore how Mypy can help you write more robust and error-free Python code, and delve into its advanced features that can further enhance your coding process.
Let’s dive in and start mastering Mypy!
TL;DR: What is Mypy and How Do I Use It?
Mypy is a static type checker for Python, which helps to catch common errors before running your code. You use it by running the
mypy
command followed by your Python script.
Here’s a simple example:
def greet(name: str) -> str:
return 'Hello, ' + name
print(greet(123))
# Running `mypy script.py` will output:
# script.py:5: error: Argument 1 to 'greet' has incompatible type 'int'; expected 'str'
In this example, we’ve defined a function greet
that expects a string argument. However, we’re trying to call greet
with an integer, which is a type mismatch. Mypy catches this error before we even run the script, saving us from a potential runtime error.
This is just a glimpse of what Mypy can do. Keep reading for a comprehensive guide on how to use Mypy, from basic usage to advanced techniques.
Table of Contents
- Getting Started with Mypy
- The Pros and Cons of Mypy
- Mypy and Python Versions
- Mypy and Third-Party Libraries
- Exploring Alternatives to Mypy
- Troubleshooting Common Mypy Errors
- Understanding Static Type Checking
- The Theory Behind Mypy
- Integrating Mypy into Larger Projects
- CI Systems and Mypy Automation
- Recap: Mypy Type Checking Guide
Getting Started with Mypy
Before you can start catching errors with Mypy, you’ll need to install it. This can be done easily using pip, Python’s package installer.
pip install mypy
With Mypy installed, you can now start using it to check your Python scripts. Here’s a basic example:
def greet(name: str) -> str:
return 'Hello, ' + name
print(greet('Alice'))
# Running `mypy script.py` will output:
# Success: no issues found in 1 source file
In this example, the greet
function is correctly called with a string argument, so Mypy reports no issues.
The Pros and Cons of Mypy
Using Mypy comes with several benefits. It can catch common errors before runtime, saving you from potential bugs and crashes. It also encourages you to think more about your code’s design, leading to more robust and maintainable code.
However, using Mypy also has potential pitfalls. It requires you to spend extra time on type annotations, which can slow down development. It also can’t catch all types of errors, so it’s not a replacement for good testing practices.
Despite these potential drawbacks, the benefits of using Mypy often outweigh the costs, especially for larger projects where catching errors early can save significant time and effort.
Mypy and Python Versions
Mypy is versatile and supports a wide range of Python versions, from Python 2.7 all the way up to Python 3.8. This means you can use Mypy to check your code, no matter which version of Python you’re using.
To specify a Python version for Mypy to check against, you can use the --python-version
flag followed by the version number. Here’s an example:
mypy --python-version 3.6 script.py
# Output:
# Success: no issues found in 1 source file
In this example, Mypy checks the script as if it were running under Python 3.6.
Mypy and Third-Party Libraries
Mypy can also check code that uses third-party libraries. However, it requires type information for these libraries to do so. Some libraries come with type annotations or stubs that provide this information, while others do not.
If a library does not have type annotations or stubs, Mypy won’t be able to check the types of the library’s functions, methods, and classes. In this case, you can use # type: ignore
to tell Mypy to ignore a particular line.
Here’s an example:
import some_library
some_library.some_function() # type: ignore
# Running `mypy script.py` will output:
# Success: no issues found in 1 source file
In this example, Mypy ignores the line where some_function
from some_library
is called, and thus does not report any potential type errors in that line.
Exploring Alternatives to Mypy
While Mypy is a powerful tool for static type checking in Python, it isn’t the only one. Other tools like Pyright and Pytype also offer static type checking capabilities and might be more suitable depending on your specific needs.
Pyright: A Faster, Configurable Type Checker
Pyright, developed by Microsoft, is known for its speed and configurability. It’s written in TypeScript and runs on Node.js, making it faster than Mypy in some cases.
npm install -g pyright
pyright script.py
# Output:
# Loading configuration file at /path/to/pyrightconfig.json
# No configuration file found.
# Assuming Python platform Linux
# Searching for source files
# Found 1 source file
# 0 errors, 0 warnings, 0 infos
# Completed in 1.688sec
In this example, Pyright is used to check script.py
, and it completes the check in under two seconds.
Pytype: Google’s Static Type Analyzer
Pytype, developed by Google, can infer types even when no type annotations are present. It can also generate type stubs for a Python file.
pip install pytype
pytype script.py
# Output:
# Computing dependencies
# Analyzing 1 sources with 0 local dependencies
# n block(s), 0 equivalence(s)
# No errors found
In this example, Pytype is used to check script.py
, and it reports no errors.
While Mypy is a great tool for static type checking, Pyright and Pytype are worthy alternatives to consider. Depending on your project’s needs, one may prove more beneficial than the others.
Troubleshooting Common Mypy Errors
While Mypy is a powerful tool for catching errors in your Python code, it’s not without its own quirks. Here, we’ll discuss some common issues you might encounter when using Mypy and how to solve them.
Dealing with Missing Imports
One common issue with Mypy is dealing with missing imports. When Mypy can’t find an imported module, it will raise an error. Here’s an example:
import non_existent_module
# Running `mypy script.py` will output:
# script.py:1: error: Cannot find implementation or library stub for module named 'non_existent_module'
In this example, Mypy raises an error because it can’t find non_existent_module
. To fix this, ensure that the module is installed and that Mypy is running in the correct environment.
Handling Incompatible Types
Another common issue is dealing with incompatible types. This occurs when the type of a variable doesn’t match the expected type. Here’s an example:
def greet(name: str) -> str:
return 'Hello, ' + name
print(greet(123))
# Running `mypy script.py` will output:
# script.py:5: error: Argument 1 to 'greet' has incompatible type 'int'; expected 'str'
In this example, Mypy raises an error because we’re trying to use an integer as an argument to greet
, which expects a string. To fix this, ensure that the types of your variables match their expected types.
Remember, while Mypy is a helpful tool, it’s not a silver bullet. It’s still important to test your code and use good programming practices.
Understanding Static Type Checking
Static type checking is a method where the type of a variable is checked at compile-time, rather than at runtime. The main advantage of this approach is that many type errors can be caught early in the development process, preventing bugs from creeping into the final product.
Consider the following Python code:
def add_numbers(a: int, b: int) -> int:
return a + b
result = add_numbers('one', 'two')
# Running `mypy script.py` will output:
# script.py:5: error: Argument 1 to 'add_numbers' has incompatible type 'str'; expected 'int'
# script.py:5: error: Argument 2 to 'add_numbers' has incompatible type 'str'; expected 'int'
In this example, Mypy catches the error before the code is even run. This is the power of static type checking.
The Theory Behind Mypy
Mypy is built on the concept of gradual typing, a type system in which variables may be typed either at compile-time (which is static typing) or at runtime (which is dynamic typing). This gives you the flexibility of Python’s dynamic typing, along with the safety of static type checking.
Mypy checks types during the compile time, and if it finds inconsistencies in the data types that are not supposed to be compatible, it raises errors, thus helping in debugging the code.
Remember, while Mypy can catch many type errors, it’s not a substitute for good programming practices or thorough testing. Always test your code, even if it passes Mypy’s checks.
Integrating Mypy into Larger Projects
Mypy isn’t just for small scripts or individual modules. It can also be integrated into larger projects, providing type checking across multiple modules and even entire packages.
To do this, you simply run Mypy on the root directory of your project. Mypy will recursively traverse the directory, checking all Python files it encounters.
mypy my_project/
# Output:
# Success: no issues found in 10 source files
In this example, Mypy checks all Python files in the my_project
directory and its subdirectories, reporting no issues.
CI Systems and Mypy Automation
Mypy can also be integrated into continuous integration (CI) systems. This allows Mypy to automatically check your code every time you make a commit, helping to catch errors as early as possible.
Here’s an example of a GitLab CI job that runs Mypy on your code:
mypy:
script:
- pip install mypy
- mypy my_project/
In this example, the CI job installs Mypy and then runs it on the my_project
directory.
Further Resources for Mypy and Testing
If you’re interested in learning more about Mypy, here are some resources you might find helpful:
- Pytest Guide | Elevating Your Python Testing Game – Learn how Pytest can improve your testing workflow and simplify test maintenance.
Using the Built-in Python Debugger – Master the art of using Python’s debugger to identify and fix issues in your programs.
Python unittest Best Practices – Enhance code quality by creating test cases, fixtures, and test suites with Python’s unittest.
Mypy Documentation – The official documentation for Mypy.
Python Typing by Real Python – An article about type checking in Python, including a section on Mypy.
Introduction to Mypy – A YouTube tutorial by PyCon 2019 explaining the basics of using Mypy.
Recap: Mypy Type Checking Guide
In this comprehensive guide, we’ve delved deep into the world of Mypy, a static type checker for Python. We’ve explored its basic usage, advanced features, and how it can be integrated into larger projects and continuous integration systems.
We started our journey with the basics, learning how to install Mypy and use it to check our Python scripts. We then advanced to more complex usage, such as using Mypy with different Python versions and third-party libraries. Along the way, we tackled common issues you might encounter when using Mypy and provided solutions to these problems.
We also looked at alternative static type checkers for Python, such as Pyright and Pytype, giving you a sense of the broader landscape of tools available for static type checking. Here’s a quick comparison of these tools:
Tool | Pros | Cons |
---|---|---|
Mypy | Versatile, supports many Python versions | May require additional time for type annotations |
Pyright | Fast, configurable | Requires Node.js |
Pytype | Can infer types, generates type stubs | May be less accurate with complex code |
Whether you’re a beginner just starting out with Mypy or an experienced Python developer looking to level up your static type checking skills, we hope this guide has given you a deeper understanding of Mypy and its capabilities. Happy coding!