Python Debugger: Comprehensive Guide
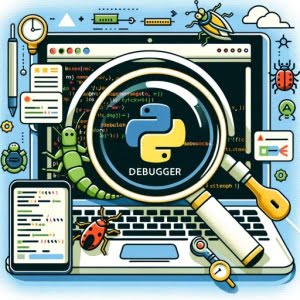
Ever felt like you’re lost in a maze while debugging your Python code? You’re not alone. Many developers find themselves in a similar predicament, but there’s a tool that can help you navigate this labyrinth.
Think of Python’s built-in debugger (pdb) as your guiding compass. It can help you trace the path of your code, inspect variables, and even change the course of your program on the fly.
This guide will walk you through the basics to advanced usage of the Python debugger. We’ll cover everything from setting breakpoints, stepping through the code, to using more advanced features like conditional breakpoints and post-mortem debugging.
Let’s dive in and start mastering the Python debugger!
TL;DR: How Do I Use the Python Debugger?
To use the Python debugger, you can use the
pdb
module. This module allows you to pause your program, inspect variables, execute commands, and step through the code.
Here’s a simple example:
import pdb
# Some Python code
x = 10
y = 20
# Set a breakpoint here
pdb.set_trace()
# More Python code
result = x + y
print(result)
# Output:
# > <stdin>(7)<module>()->None
# (Pdb) p x
# 10
# (Pdb) p y
# 20
# (Pdb) c
# 30
In this example, we import the pdb
module and set a breakpoint using pdb.set_trace()
. When the Python interpreter reaches this line, it will pause the program. At this point, you can inspect variables (p x
, p y
), execute commands, and step through the code. The c
command continues the execution of the program.
This is just a basic introduction to using the Python debugger. There’s a lot more to learn about
pdb
, including advanced features like conditional breakpoints and post-mortem debugging. Continue reading for a comprehensive guide on mastering the Python debugger.
Table of Contents
Getting Started with Python Debugger
The Python debugger, also known as pdb
, is a built-in module that allows you to interactively examine your code. Let’s take a closer look at some of its basic features including setting breakpoints, stepping through the code, and inspecting variables.
Setting Breakpoints
Breakpoints are specific points in your code where you instruct the debugger to pause the execution of your program. This allows you to inspect the current state of your program, including the values of variables.
Here’s how to set a breakpoint using pdb
:
import pdb
x = 10
y = 20
# Set a breakpoint here
pdb.set_trace()
result = x + y
print(result)
# Output:
# > <stdin>(7)<module>()->None
# (Pdb) c
# 30
In this code, we’ve set a breakpoint at the line pdb.set_trace()
. When the Python interpreter reaches this line, it’ll pause the program and open the debugger.
Stepping Through the Code
Once the debugger is open, you can use various commands to control the execution of your program. The n
(next) command executes the current line and moves to the next one. The s
(step) command steps into functions or methods. Here’s an example:
import pdb
def add(x, y):
return x + y
x = 10
y = 20
# Set a breakpoint here
pdb.set_trace()
result = add(x, y)
print(result)
# Output:
# > <stdin>(9)<module>()->None
# (Pdb) s
# --Call--
# > <stdin>(4)add()
# (Pdb) n
# > <stdin>(5)add()->30
# (Pdb) c
# 30
In this example, we used the s
command to step into the add
function. We then used the n
command to execute the return statement, and the c
command to continue the execution of the program.
Inspecting Variables
The p
(print) command allows you to print the value of variables. Here’s how you can use it:
import pdb
x = 10
y = 20
# Set a breakpoint here
pdb.set_trace()
result = x + y
print(result)
# Output:
# > <stdin>(7)<module>()->None
# (Pdb) p x
# 10
# (Pdb) p y
# 20
# (Pdb) c
# 30
In this example, we used the p
command to print the values of x
and y
. This is particularly useful when you want to inspect the values of variables at a specific point in your code.
These are just the basics of using the Python debugger, but they’re enough to get you started on your journey. As you become more comfortable with these features, you can start exploring more advanced features of pdb
.
Advanced Features: Python Debugger
Now that we’ve covered the basics of the Python debugger, let’s dive into some of its more advanced features. We’ll explore conditional breakpoints, post-mortem debugging, and using the debugger within an Integrated Development Environment (IDE).
Conditional Breakpoints
Sometimes, you might want your program to pause only when a certain condition is met. This is where conditional breakpoints come in handy. Here’s how you can set a conditional breakpoint in pdb
:
import pdb
x = 10
y = 20
# Set a conditional breakpoint here
if y > x:
pdb.set_trace()
result = x + y
print(result)
# Output:
# > <stdin>(8)<module>()->None
# (Pdb) p x
# 10
# (Pdb) p y
# 20
# (Pdb) c
# 30
In this example, the debugger will only pause if y
is greater than x
. This can be incredibly useful when debugging complex conditions in your code.
Post-Mortem Debugging
Post-mortem debugging allows you to inspect the state of your program at the point of an exception. Here’s an example of how you can use it:
import pdb
def divide(x, y):
return x / y
try:
result = divide(10, 0)
except Exception:
pdb.post_mortem()
# Output:
# > <stdin>(4)divide()->None
# (Pdb) p x
# 10
# (Pdb) p y
# 0
In this example, an exception occurs when we try to divide by zero. The pdb.post_mortem()
function is called within the except
block, which opens the debugger at the point of the exception.
Using the Debugger in an IDE
Most IDEs, such as PyCharm or Visual Studio Code, have built-in support for pdb
. This means you can set breakpoints, step through the code, and inspect variables right within your IDE. This can greatly enhance your debugging experience.
These advanced features of the Python debugger can help you debug more effectively and efficiently. So don’t be afraid to explore them and see how they can improve your debugging skills.
Alternative Python Debugging Tools
While pdb
is a powerful debugger, Python offers other debugging tools that you might find useful. Let’s take a look at some of these alternatives, including PyCharm’s debugger, ipdb
, and pdb++
, and discuss their advantages and disadvantages compared to the built-in Python debugger.
PyCharm’s Debugger
PyCharm, a popular Python IDE, comes with its own debugger. It provides a user-friendly interface that allows you to easily set breakpoints, inspect variables, and control the execution of your program.
PyCharm’s debugger can be used by clicking on the gutter next to the line number and then running your program in debug mode.
One advantage of PyCharm’s debugger is its integration with the IDE, which can make the debugging process smoother. However, it might be overkill if you’re working on a small script or if you prefer working in a text editor.
ipdb
ipdb
is another debugging tool that you can use. It provides the same functionality as pdb
, but with added features like syntax highlighting and tab completion.
import ipdb
x = 10
y = 20
# Set a breakpoint here
ipdb.set_trace()
result = x + y
print(result)
# Output:
# > <stdin>(6)<module>()->None
# ipdb> p x
# 10
# ipdb> p y
# 20
# ipdb> c
# 30
The main advantage of ipdb
is its enhanced user interface, which can make your debugging experience more pleasant. However, it’s not built-in and requires an additional installation.
pdb++
pdb++
is an extension of pdb
that provides additional features like sticky mode, syntax highlighting, and tab completion.
# pdb++ is used in the same way as pdb, but with additional features. Here's how you can use it:
# First, install pdb++
# pip install pdbpp
import pdb
x = 10
y = 20
# Set a breakpoint here
pdb.set_trace()
result = x + y
print(result)
# Output:
# > <stdin>(8)<module>()->None
# (Pdb++) p x
# 10
# (Pdb++) p y
# 20
# (Pdb++) c
# 30
The advantage of pdb++
is that it enhances the functionality of pdb
without changing the way you use it. However, like ipdb
, it requires an additional installation.
While the built-in Python debugger is a powerful tool, these alternatives offer enhanced features that can improve your debugging experience. It’s worth exploring these tools to see which one fits your needs best.
Fixing Issues: Python Debugger
Like any tool, the Python debugger isn’t without its quirks. You might run into issues like not being able to inspect variables or the debugger not stopping at breakpoints. Let’s tackle these common problems and provide some solutions.
Debugger Not Inspecting Variables
Sometimes, you might find that the debugger isn’t inspecting variables as expected. This could be due to a variety of reasons, such as the variable not being defined at the point of the breakpoint.
import pdb
# Set a breakpoint here
pdb.set_trace()
x = 10
# Output:
# > <stdin>(4)<module>()->None
# (Pdb) p x
# *** NameError: name 'x' is not defined
In this example, we tried to print the value of x
before it was defined, resulting in a NameError
. To fix this, ensure that the variable is defined before the breakpoint.
Debugger Not Stopping at Breakpoints
Another common issue is the debugger not stopping at breakpoints. This could be because the line with the breakpoint isn’t being executed.
import pdb
x = 10
if x > 20:
# Set a breakpoint here
pdb.set_trace()
result = x * 2
print(result)
# Output:
# 20
In this example, the breakpoint is inside an if
statement that isn’t true, so the line with the breakpoint isn’t executed. To fix this, ensure that the line with the breakpoint is being executed.
These are just a couple of common issues you might face when using the Python debugger. Remember, every problem has a solution, so don’t let these minor hiccups discourage you from using this powerful debugging tool!
The Art of Debugging Explained
Before we delve deeper into the specifics of the Python debugger, it’s important to understand the concept of debugging and its significance in the realm of software development.
What is Debugging?
Debugging is a methodical process of finding and fixing bugs or issues in a computer program. It’s an integral part of the software development cycle, often taking up a significant portion of the development time.
# A simple Python program with a bug
def add(x, y):
return x - y # Oops! This should be x + y
result = add(10, 20)
print(result)
# Output:
# -10
In this example, the add
function is supposed to add x
and y
, but due to a bug, it subtracts y
from x
instead. Debugging is the process of identifying and fixing this bug.
Why is Debugging Necessary?
Bugs can lead to unexpected behavior, incorrect results, and even system crashes. Debugging helps ensure that your program works as expected and provides correct results.
# The corrected Python program
def add(x, y):
return x + y # Fixed the bug
result = add(10, 20)
print(result)
# Output:
# 30
After debugging and fixing the bug, the add
function now works correctly, providing the correct result.
The Role of a Debugger in the Development Process
A debugger is a tool that aids in the debugging process. It allows you to control the execution of your program, inspect the program’s state at any point, and even change the program’s state on the fly.
Using a debugger like pdb
can greatly enhance your productivity and make the process of finding and fixing bugs much more efficient. It’s a crucial tool in any developer’s toolkit, and mastering it is well worth the effort.
Larger Projects and the Debugger
While we’ve covered the basics and advanced usage of the Python debugger, there’s still a lot more to explore. Let’s discuss how you can use pdb
in larger projects and how it integrates with testing frameworks and continuous integration systems. We’ll also suggest some related topics for you to explore further.
Python Debugger in Large-Scale Projects
When working on larger projects, the Python debugger can be an invaluable tool. You can use it to debug complex systems, inspect interactions between different parts of your code, and even debug code running on remote servers.
# In a large project, you might have multiple modules. Here's how you can use pdb to debug them.
# module1.py
import pdb
import module2
x = 10
y = 20
# Set a breakpoint here
pdb.set_trace()
result = module2.add(x, y)
print(result)
# module2.py
def add(x, y):
return x + y
# Output:
# > <stdin>(8)<module>()->None
# (Pdb) s
# --Call--
# > <stdin>(4)add()
# (Pdb) n
# > <stdin>(5)add()->30
# (Pdb) c
# 30
In this example, we’re using pdb
to debug a function in a different module. This can be incredibly useful when debugging large projects with multiple modules.
Integrating Python Debugger with Testing Frameworks
The Python debugger can also be integrated with testing frameworks like pytest. This allows you to debug your tests, making it easier to identify and fix issues.
# test_example.py
import pytest
import pdb
def add(x, y):
return x + y
def test_add():
# Set a breakpoint here
pdb.set_trace()
assert add(10, 20) == 30
# Run the test with pytest
# pytest test_example.py
# Output:
# > <stdin>(9)test_add()->None
# (Pdb) s
# --Return--
# > <stdin>(9)test_add()->None
# (Pdb) c
# .
# 1 passed in 0.01s
In this example, we’re using pdb
to debug a test case. When the breakpoint is hit, you can inspect variables, step through the code, and even change the state of the program.
Further Resources for Mastering Python Debugger
If you’re interested in delving deeper into the Python debugger, here are some resources you might find useful:
- This blog post explains the ins and outs of Pytest’s command-line options for Python testing.
Exploring the Benefits of mypy – Master the art of Static Typing in Python with mypy to enhance code quality and maintainability in Python.
Resolving “No Module Named” Errors in Python – Learn how to troubleshoot and resolve the “No module named” error in Python.
Python’s Official pdb: The Python Debugger Documentation – This is the official Python documentation for their built-in debugger pdb.
Python Debugging With Pdb – This tutorial provides a comprehensive overview of the Python debugger, including its basic and advanced features.
Python Debugging Using PyCharm – JetBrains provides a useful guide to debugging Python programs using the PyCharm IDE.
Remember, mastering a tool like the Python debugger takes time and practice. So don’t be discouraged if you don’t get it right away. Keep practicing, and before you know it, you’ll be a pro at debugging Python code.
Summary: Python Debugger Tutorial
In this comprehensive guide, we’ve journeyed through the world of Python debugging, exploring the built-in Python debugger, pdb, from its basic to advanced features.
We began with the basics, learning how to set breakpoints, step through the code, and inspect variables. We then ventured into more advanced territory, exploring conditional breakpoints, post-mortem debugging, and how to use pdb within an Integrated Development Environment (IDE).
Along the way, we tackled common challenges you might face when using pdb, such as not being able to inspect variables or the debugger not stopping at breakpoints, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to debugging in Python, comparing pdb with other debugging tools like PyCharm’s debugger, ipdb, and pdb++. Here’s a quick comparison of these tools:
Debugger | Pros | Cons |
---|---|---|
pdb | Built-in, no additional installation needed | User interface can be improved |
PyCharm’s Debugger | User-friendly interface, integrated with the IDE | Might be overkill for small scripts |
ipdb | Enhanced user interface, syntax highlighting, tab completion | Requires additional installation |
pdb++ | Enhances pdb’s functionality, syntax highlighting, tab completion | Requires additional installation |
Whether you’re a beginner just starting out with Python debugging or an experienced developer looking to level up your debugging skills, we hope this guide has given you a deeper understanding of the Python debugger and its capabilities. Happy debugging!