Java Casting Explained: From Basics to Advanced
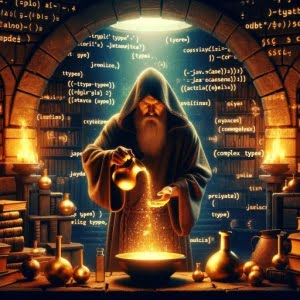
Are you finding it difficult to grasp the concept of Java casting? You’re not alone. Many developers find themselves puzzled when it comes to understanding Java casting, but we’re here to help.
Think of Java casting as a movie director choosing the right actor for a role. It allows us to convert one type of data into another, providing a versatile and handy tool for various tasks.
This guide will walk you through the ins and outs of Java casting, from basic concepts to advanced techniques. We’ll cover everything from the basics of casting to more advanced techniques, as well as alternative approaches.
Let’s get started and master Java casting!
TL;DR: What is Java Casting and How Does it Work?
Java casting is a process of converting one type of data into another. It can be done in two ways: upcasting (casting to a superclass), such as
superClass super = new subClass();
and downcasting (casting to a subclass), such assubClass sub = (subClass)super;
.
Here’s a simple example of upcasting:
Animal myDog = new Dog();
In this example, we’re creating a new Dog
object and treating it as an Animal
. This is known as upcasting, where we’re casting an object to its superclass.
And here’s an example of downcasting:
Dog myDog = (Dog) myAnimal;
Here, we’re doing the opposite. We have an Animal
object, but we’re certain that it’s actually a Dog
, so we’re casting it down to a Dog
. This is known as downcasting, where we’re casting an object to its subclass.
These are the basics of Java casting, but there’s much more to it. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Java Casting: Upcasting and Downcasting for Beginners
- Navigating Complex Casting Scenarios in Java
- Alternative Techniques for Type Conversion in Java
- Troubleshooting Java Casting: Avoiding ‘ClassCastException’
- Java Fundamentals: Type System, Inheritance, and Polymorphism
- The Relevance of Casting in Object-Oriented Programming and Design Patterns
- Wrapping Up: Java Casting
Java Casting: Upcasting and Downcasting for Beginners
In Java, casting is a fundamental concept that every developer needs to grasp. It allows us to convert an object of one type into another, and it’s used in a variety of different scenarios. Let’s delve into the two main types of casting: upcasting and downcasting.
Upcasting in Java
Upcasting is when we convert a subclass object into a superclass object. It’s also known as ‘widening’ because we’re moving up the inheritance hierarchy, which is generally wider at the top.
Here’s an example of upcasting:
Dog myDog = new Dog();
Animal myAnimal = myDog; // Upcasting
System.out.println(myAnimal.getClass().getSimpleName());
// Output:
// Dog
In this example, we’re creating a new Dog
object and treating it as an Animal
. Even though myAnimal
is of type Animal
, it still retains the original Dog
type underneath.
Upcasting is safe and doesn’t require an explicit cast operator. It’s useful when we want to use only the superclass’s methods and fields on an object, regardless of its actual subclass type.
Downcasting in Java
Downcasting, on the other hand, is when we convert a superclass object into a subclass object. It’s also known as ‘narrowing’ because we’re moving down the inheritance hierarchy, which is generally narrower at the bottom.
Here’s an example of downcasting:
Animal myAnimal = new Dog();
Dog myDog = (Dog) myAnimal; // Downcasting
System.out.println(myDog.getClass().getSimpleName());
// Output:
// Dog
In this example, we have an Animal
object, but we’re certain that it’s actually a Dog
, so we’re casting it down to a Dog
. Downcasting requires an explicit cast operator, and it’s risky because if myAnimal
wasn’t actually a Dog
, we would get a ClassCastException
at runtime.
Downcasting is useful when we know that a superclass object is actually a specific subclass object, and we want to use the subclass’s methods and fields on it.
In summary, upcasting and downcasting are powerful tools in Java, but they should be used with care. Upcasting is always safe, while downcasting can lead to runtime errors if not used correctly.
As you become more comfortable with Java casting, you’ll encounter more complex scenarios that go beyond simple upcasting and downcasting. Two such scenarios include casting in inheritance hierarchies and casting with interfaces. Let’s dive into each of these.
Casting in Inheritance Hierarchies
In an inheritance hierarchy, you might need to cast an object to a specific subclass or superclass. This can be tricky and requires a good understanding of the hierarchy structure.
Consider the following code:
class Animal {}
class Mammal extends Animal {}
class Dog extends Mammal {}
Animal animal = new Dog();
Mammal mammal = (Mammal) animal; // Casting to Mammal
System.out.println(mammal.getClass().getSimpleName());
// Output:
// Dog
In this example, we have a Dog
object stored in an Animal
reference. We then cast it to a Mammal
. Even though we cast to Mammal
, the underlying object is still a Dog
.
Casting with Interfaces
Casting isn’t limited to classes. It can also be used with interfaces. If a class implements an interface, you can cast an object of the class to the interface type.
Here’s an example:
interface Barkable {
void bark();
}
class Dog implements Barkable {
public void bark() {
System.out.println("Woof!");
}
}
Barkable barkable = new Dog(); // Upcasting to interface
Dog dog = (Dog) barkable; // Downcasting back to Dog
dog.bark();
// Output:
// Woof!
In this example, Dog
implements the Barkable
interface. We create a Dog
object and upcast it to Barkable
. Then we downcast it back to Dog
and call the bark
method.
These examples illustrate that Java casting can get complex, especially in larger codebases with multiple inheritance hierarchies and interfaces. However, with practice and understanding, you’ll be able to navigate these scenarios with ease.
Alternative Techniques for Type Conversion in Java
While casting is a powerful tool for type conversion in Java, it’s not the only option. There are other ways to convert between types, particularly when dealing with primitive types and their corresponding wrapper classes. Let’s explore two of these methods: using wrapper classes and the toString()
method.
Converting with Wrapper Classes
Java provides wrapper classes for each of the primitive data types. These classes offer methods to convert between primitive types and strings. Here’s an example:
int number = 123;
String numberString = Integer.toString(number);
System.out.println(numberString);
// Output:
// 123
In this example, we’re using the Integer
wrapper class’s toString()
method to convert an int
to a String
. This method is safe and doesn’t risk throwing a ClassCastException
.
Converting with the toString() Method
Every object in Java has a toString()
method, as it’s defined in the Object
class from which all classes inherit. This method returns a string representation of the object, which can often be used for type conversion. Here’s an example:
Dog myDog = new Dog();
String dogString = myDog.toString();
System.out.println(dogString);
// Output:
// Dog@4554617c
In this example, we’re using the toString()
method to convert a Dog
object to a String
. The output is the class name, followed by the ‘@’ symbol, followed by the hashcode of the object in hexadecimal.
Both of these methods offer alternatives to casting for type conversion. However, they’re not perfect substitutes. They can only convert to and from String
types, and they don’t offer the same level of control as casting. But in certain scenarios, they can be useful tools to have in your Java toolbox.
Troubleshooting Java Casting: Avoiding ‘ClassCastException’
As you delve deeper into Java casting, you’ll inevitably run into some common issues. The most notorious of these is the ClassCastException
. This exception is thrown when an attempt is made to cast an object to a type with which it is not compatible.
Understanding ‘ClassCastException’
The ‘ClassCastException’ often occurs during downcasting, when we try to cast an object to a subclass it doesn’t belong to. Here’s an example:
Animal myAnimal = new Animal();
Dog myDog = (Dog) myAnimal; // Throws ClassCastException
In this example, we’re trying to cast an Animal
object to a Dog
. But myAnimal
is not a Dog
, so a ClassCastException
is thrown.
Preventing ‘ClassCastException’
One way to prevent ‘ClassCastException’ is to use the instanceof
operator before casting. The instanceof
operator checks if an object is an instance of a particular class or implements a particular interface.
Here’s how you can use it:
if (myAnimal instanceof Dog) {
Dog myDog = (Dog) myAnimal;
} else {
System.out.println("Cannot cast to Dog");
}
// Output:
// Cannot cast to Dog
In this example, we’re checking if myAnimal
is an instance of Dog
before attempting to cast. If it’s not, we print a message instead of throwing a ClassCastException
.
Other Considerations
While the instanceof
operator can prevent ‘ClassCastException’, it’s not always the best solution. Overuse of instanceof
can make your code harder to read and maintain. It’s often better to design your classes and interfaces in a way that minimizes the need for explicit type checking and casting.
In conclusion, while Java casting is a powerful tool, it’s not without its pitfalls. Understanding these issues and knowing how to avoid them is a crucial part of mastering Java casting.
Java Fundamentals: Type System, Inheritance, and Polymorphism
To fully grasp the concept of Java casting, it’s essential to understand the fundamentals that underpin it: Java’s type system, inheritance, and polymorphism.
Java’s Type System
In Java, every variable and every expression has a type. The type system is robust and strictly enforced, which helps prevent bugs and makes code easier to read and understand.
Java’s type system is divided into two categories: primitive types and reference types. Primitives are the basic types like int
, char
, and boolean
. Reference types are any instantiable class as well as arrays.
Inheritance in Java
Inheritance is a fundamental concept in object-oriented programming, and Java is no exception. It allows classes to inherit fields and methods from other classes.
In Java, each class is allowed to inherit from one superclass. The superclass (parent) features can be used in the subclass (child), and if the subclass needs to modify a behavior, it can override the superclass’s methods.
Here’s a simple example of inheritance:
class Animal {
void eat() {
System.out.println("Animal is eating...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Dog is barking...");
}
}
Dog myDog = new Dog();
myDog.eat();
myDog.bark();
// Output:
// Animal is eating...
// Dog is barking...
In this example, Dog
is a subclass of Animal
and inherits the eat
method from Animal
. Dog
also defines its own method bark
.
Polymorphism in Java
Polymorphism is another fundamental concept in object-oriented programming. It allows a subclass to be treated as its superclass. This is a powerful feature that allows the same code to work with different types.
Here’s a simple example of polymorphism:
Animal myAnimal = new Dog();
myAnimal.eat();
// Output:
// Animal is eating...
In this example, even though myAnimal
is of type Animal
, it can be assigned a Dog
object. This is polymorphism in action.
Understanding these fundamentals is crucial to mastering Java casting. Casting is essentially a way to manipulate Java’s type system, allowing a variable of one type to be treated as another type. It’s a powerful tool, but with great power comes great responsibility. Use it wisely!
The Relevance of Casting in Object-Oriented Programming and Design Patterns
Java casting is not an isolated concept. It plays a significant role in object-oriented programming and design patterns. Understanding casting can open up a world of possibilities in your Java programming journey.
Casting in Object-Oriented Programming
In an object-oriented paradigm, casting is a vital tool. It allows us to leverage polymorphism, one of the key principles of object-oriented programming. With casting, we can treat an object of a subclass as an instance of its superclass, or vice versa. This flexibility enables us to write more generic and reusable code.
Casting in Design Patterns
Many design patterns, such as the Factory and Visitor patterns, rely on casting. These patterns often involve a superclass reference being cast to a subclass reference, allowing for dynamic method binding and increased flexibility.
Exploring Related Concepts: Generics in Java
Java casting is just the tip of the iceberg. If you’re interested in diving deeper, another related concept to explore is generics. Generics provide a way for you to generalize over types, making your code more flexible and reusable.
Here’s a simple example of generics:
List<String> list = new ArrayList<>();
list.add("Hello");
String str = list.get(0);
System.out.println(str);
// Output:
// Hello
In this example, we’re creating a list that can only contain String
objects. Generics ensure type safety, reducing the need for casting and the risk of ClassCastException
.
Further Resources for Mastering Java Casting
If you’re interested in learning more about Java casting and related concepts, here are some resources that you might find helpful:
- Java Data Types – Understand Java’s data types for storing various kinds of values efficiently.
Converting Char to String – Learn convenient methods to transform char data to string format.
String to Int in Java – Easily convert string representations of numbers to integers in Java.
Java Tutorials by Oracle covers a wide range of topics, including casting and generics.
Java Programming Masterclass for Software Developers – This course covers the core concepts of Java programming.
Java Generics and Collections – This book provides an in-depth look at generics and collections in Java.
By mastering Java casting and diving into related concepts, you’ll be well on your way to becoming a proficient Java programmer.
Wrapping Up: Java Casting
In this comprehensive guide, we’ve journeyed through the world of Java casting, a fundamental concept in Java programming that allows us to convert one type of data into another.
We began with the basics, learning how to perform upcasting and downcasting in Java. We then ventured into more advanced territory, exploring complex casting scenarios in inheritance hierarchies and casting with interfaces. Along the way, we tackled common challenges you might face when using Java casting, such as the dreaded ‘ClassCastException’, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to type conversion in Java, such as using wrapper classes and the toString()
method. These methods offer alternatives to casting for type conversion, particularly when dealing with primitive types and their corresponding wrapper classes.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Casting | Powerful, allows for precise control | Risk of ‘ClassCastException’ |
Wrapper Classes | Safe, no risk of exception | Limited to converting to and from String |
toString() Method | Safe, no risk of exception | Limited to converting to String |
Whether you’re just starting out with Java casting or you’re looking to level up your Java programming skills, we hope this guide has given you a deeper understanding of Java casting and its alternatives.
With its balance of power and flexibility, Java casting is a crucial tool for any Java programmer. Remember to use it wisely and enjoy the benefits it brings to your programming toolkit. Happy coding!