Java String to Int Conversion: Methods and Examples
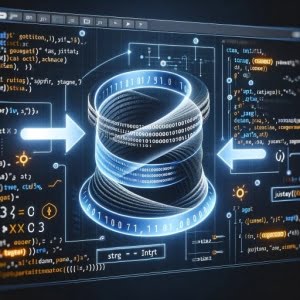
Are you finding it challenging to convert strings to integers in Java? You’re not alone. Many developers find themselves in a bind when it comes to this seemingly simple task. But, like a skilled mathematician, Java has the ability to transform words into numbers with ease.
Java’s string to integer conversion is akin to a translator – adeptly bridging the gap between the realm of text and numbers. This conversion is a fundamental aspect of many programming tasks, especially when dealing with user input or file and network operations.
This guide will walk you through the key methods in Java used for converting strings to integers, from basic use to advanced techniques. We’ll cover everything from the simple Integer.parseInt()
and Integer.valueOf()
methods to handling exceptions and using alternative approaches.
So, let’s dive in and start mastering the art of string to integer conversion in Java!
TL;DR: How Do I Convert a String to an Integer in Java?
To convert a string to an integer in Java, you can use the
Integer.parseInt()
method, with syntaxint num = Integer.parseInt(str);
or theInteger.valueOf()
method, with the syntax,Integer num = Integer.valueOf(str);
. These methods are part of the Integer class in Java and are used to convert a string into its integer equivalent.
Here’s a simple example:
String str = "123";
int num = Integer.parseInt(str);
System.out.println(num);
// Output:
// 123
In this example, we have a string str
with the value “123”. We use the Integer.parseInt()
method to convert this string into an integer. The result is stored in the variable num
. When we print out num
, we get the integer 123.
This is a basic way to convert a string to an integer in Java, but there’s much more to learn about handling different scenarios and exceptions. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Unpacking Integer.parseInt() and Integer.valueOf()
- Handling NumberFormatException in Java
- Exploring Alternative Methods for String to Int Conversion
- Navigating Common Issues in String to Int Conversion
- Understanding Java’s String and Integer Classes
- The Relevance of String to Integer Conversion in Java
- Wrapping Up: String to Integer Conversion in Java
Unpacking Integer.parseInt()
and Integer.valueOf()
Java provides us with two straightforward methods to convert a string to an integer – Integer.parseInt()
and Integer.valueOf()
.
The Integer.parseInt()
Method
The Integer.parseInt()
method is a static method of the Integer class. It takes a string as a parameter and returns its equivalent integer value.
Here’s a simple example:
String str = "456";
int num = Integer.parseInt(str);
System.out.println(num);
// Output:
// 456
In this code block, we are converting the string str
into an integer using Integer.parseInt()
. The result is then printed out, giving us the integer 456.
The Integer.valueOf()
Method
The Integer.valueOf()
method, on the other hand, returns an instance of Integer class. It can be used when you want an Integer object instead of a primitive int.
Here’s how you can use Integer.valueOf()
:
String str = "789";
Integer num = Integer.valueOf(str);
System.out.println(num);
// Output:
// 789
In this example, we’re converting the string str
into an Integer object using Integer.valueOf()
. The result is then printed out, showing us the integer 789.
While both methods can be used to convert a string to an integer, remember that Integer.parseInt()
returns a primitive int, while Integer.valueOf()
returns an Integer object. Your choice between the two will depend on whether you need a primitive type or an object.
Handling NumberFormatException
in Java
In Java, converting a string that doesn’t represent a valid integer (like “123abc” or “hello”) using Integer.parseInt()
or Integer.valueOf()
will throw a NumberFormatException
. To prevent this from crashing your program, you can use a try-catch block to handle this exception.
Using Try-Catch to Handle NumberFormatException
A try-catch
block in Java is used to handle exceptions. It allows us to try a block of code and catch any exceptions that might be thrown from it.
Let’s see how we can use a try-catch block to handle a NumberFormatException
when converting a string to an integer:
String str = "123abc";
try {
int num = Integer.parseInt(str);
System.out.println(num);
} catch (NumberFormatException e) {
System.out.println(str + " cannot be converted to int");
}
// Output:
// 123abc cannot be converted to int
In this code block, we’re trying to convert the string str
to an integer. However, str
contains non-numeric characters, which causes Integer.parseInt()
to throw a NumberFormatException
. The catch block catches this exception and prints out a custom error message.
This is a best practice when converting strings to integers in Java, as it allows your program to continue running even if a string cannot be converted to an integer. It also provides a more user-friendly error message than the default NumberFormatException
.
Exploring Alternative Methods for String to Int Conversion
While Integer.parseInt()
and Integer.valueOf()
are the most common methods for converting strings to integers in Java, there are alternative methods available. Two such alternatives are the DecimalFormat
and NumberFormat
classes.
Using DecimalFormat
for Conversion
The DecimalFormat
class in Java is used to format decimals. However, it can also be used to convert a string to an integer. Here’s how it works:
import java.text.DecimalFormat;
String str = "12345";
DecimalFormat df = new DecimalFormat("#");
try {
Number num = df.parse(str);
System.out.println(num.intValue());
} catch (ParseException e) {
e.printStackTrace();
}
// Output:
// 12345
In this code block, we’re using the DecimalFormat
class to convert the string str
to a number. We then call the intValue()
method on the resulting number to get an integer. This method can be useful when you need to convert a decimal string to an integer.
Leveraging NumberFormat
for Conversion
The NumberFormat
class in Java is another way to convert a string to an integer. It’s a more flexible class that can handle different number formats. Here’s an example:
import java.text.NumberFormat;
import java.text.ParseException;
String str = "67890";
NumberFormat nf = NumberFormat.getInstance();
try {
Number num = nf.parse(str);
System.out.println(num.intValue());
} catch (ParseException e) {
e.printStackTrace();
}
// Output:
// 67890
In this code block, we’re using the NumberFormat
class to convert the string str
to a number. Like with DecimalFormat
, we call intValue()
on the resulting number to get an integer. NumberFormat
is a more flexible option that can handle different number formats and locales.
Comparing the Methods
Method | Returns | Exception Handling | Flexibility |
---|---|---|---|
Integer.parseInt() | Primitive int | Manual | Low |
Integer.valueOf() | Integer object | Manual | Low |
DecimalFormat | Number object | Manual | Medium |
NumberFormat | Number object | Manual | High |
While Integer.parseInt()
and Integer.valueOf()
are the most straightforward methods for converting strings to integers in Java, DecimalFormat
and NumberFormat
provide more flexibility. Your choice will depend on your specific needs and the format of the strings you’re working with.
While converting strings to integers in Java using the methods we’ve discussed, you might encounter some common issues. Let’s explore these problems and discuss their solutions.
Dealing with NumberFormatException
As we’ve mentioned earlier, a NumberFormatException
is thrown when Integer.parseInt()
or Integer.valueOf()
tries to convert a string that doesn’t represent a valid integer. This could be a string containing non-numeric characters or a string representing a number larger than Integer.MAX_VALUE
.
Here’s how to handle this exception:
String str = "123abc";
try {
int num = Integer.parseInt(str);
System.out.println(num);
} catch (NumberFormatException e) {
System.out.println(str + " cannot be converted to int");
}
// Output:
// 123abc cannot be converted to int
In this code block, we’re trying to convert the string str
to an integer. However, str
contains non-numeric characters, which causes Integer.parseInt()
to throw a NumberFormatException
. The catch block catches this exception and prints out a custom error message.
Handling Null or Empty Strings
Another common issue is trying to convert a null or empty string to an integer. This will also result in a NumberFormatException
.
Here’s an example:
String str = "";
try {
int num = Integer.parseInt(str);
System.out.println(num);
} catch (NumberFormatException e) {
System.out.println("Cannot convert empty string to int");
}
// Output:
// Cannot convert empty string to int
In this code block, str
is an empty string. When we try to convert it to an integer using Integer.parseInt()
, a NumberFormatException
is thrown. We catch this exception and print out a custom error message.
By understanding these common issues and how to handle them, you can make your string to integer conversions in Java more robust and error-free.
Understanding Java’s String and Integer Classes
To fully grasp the process of converting a string to an integer in Java, it’s crucial to understand the fundamental concepts of Java’s String and Integer classes.
Java’s String Class
In Java, strings are objects that represent sequences of characters. The Java platform provides the String class to create and manipulate strings.
String str = "Hello, World!";
System.out.println(str);
// Output:
// Hello, World!
In this example, we’re creating a string str
and printing it out. Strings in Java are immutable, meaning once created, their values cannot be changed.
Java’s Integer Class
The Integer class wraps a value of the primitive type int in an object. An object of type Integer contains a single field whose type is int. It provides several methods to convert an int into a String and a String into an int, along with other utilities for dealing with an int.
Integer num = Integer.valueOf(123);
System.out.println(num);
// Output:
// 123
In this example, we’re creating an Integer object num
from the primitive int 123 and printing it out.
Understanding these classes and their methods is crucial for converting strings to integers in Java. The String class provides the string to be converted, while the Integer class provides the methods for conversion.
The Relevance of String to Integer Conversion in Java
Java’s ability to convert strings to integers is not just a programming curiosity, it’s a fundamental skill with practical applications in various areas of software development.
Data Parsing and User Input Handling
One common use case is data parsing. When you’re reading data from a file, network, or user input, the data is often received as a string. If you need to perform mathematical operations on this data, you’ll need to convert it to an integer. Without the ability to convert strings to integers, you’d be unable to use this data effectively.
Exploring Related Concepts
The process of converting strings to integers in Java also introduces several related concepts, such as data type conversion and exception handling. Understanding these concepts is essential for becoming a proficient Java developer.
Data type conversion (or type casting) is a fundamental concept in programming that involves converting an entity of one data type into another. In our case, we’re converting a string (a sequence of characters) into an integer (a numerical value).
Exception handling is another key concept introduced through string to integer conversion. By learning how to handle NumberFormatException
, you’re getting a taste of how Java handles errors and exceptions, which is crucial for writing robust and error-free code.
Further Resources for Java String to Int Conversion
For those who want to delve deeper into the topic of string to integer conversion in Java and related concepts, here are some resources that you might find helpful:
- Use Cases Explored: Java Casting – Explore the benefits and drawbacks of using casting in Java development.
Int to String in Java – Convert integer values to strings effortlessly in Java programming.
ParseInt in Java – Learn how to parse string representations of integers into int values in Java.
Java Tutorials by Oracle cover a wide range of topics, including data types, exception handling, and more.
Baeldung’s Guide on Java Type Casting provides an overview of type conversion in Java.
Java String to int tutorial explains how to convert a string to an integer in Java using different approaches.
By exploring these resources and practicing your skills, you’ll be well on your way to mastering string to integer conversion in Java and other related concepts.
Wrapping Up: String to Integer Conversion in Java
In this comprehensive guide, we’ve delved deep into the process of converting strings to integers in Java. We’ve explored the various methods available in Java for this conversion, their uses, and how to handle common issues that may arise during this process.
We began with the basics, discussing the Integer.parseInt()
and Integer.valueOf()
methods and how to use them. We then moved on to more advanced topics, such as handling NumberFormatException
and using alternative methods like DecimalFormat
and NumberFormat
for conversion.
Along the way, we tackled common challenges you might encounter when converting strings to integers in Java, providing you with solutions and workarounds for each issue. We also looked at the underlying concepts of Java’s String and Integer classes to give you a better understanding of the conversion process.
Here’s a quick comparison of the methods we discussed:
Method | Returns | Exception Handling | Flexibility |
---|---|---|---|
Integer.parseInt() | Primitive int | Manual | Low |
Integer.valueOf() | Integer object | Manual | Low |
DecimalFormat | Number object | Manual | Medium |
NumberFormat | Number object | Manual | High |
Whether you’re just starting out with Java or you’re looking to refine your skills, we hope this guide has helped you master the process of converting strings to integers in Java.
Understanding how to convert strings to integers is a fundamental skill in Java programming, and now you’re well-equipped to handle this task efficiently and effectively. Happy coding!