‘npm start’ | How-to Start Packages in Node.js Projects
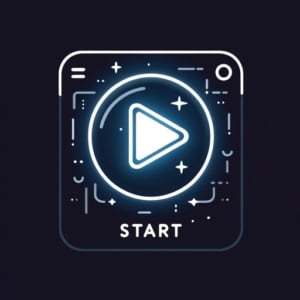
Properly launching web applications on development servers is crucial for testing here at IOFlood. Because of this challenge, I’ve had to learn to utilize ‘npm start’. Today, I have provided this guide to share my insights and step-by-step instructions, and help you initiate and manage your project’s execution.
This guide will navigate you through the essentials of using ‘npm start’, ensuring you’re equipped to launch your Node.js applications smoothly. Whether you’re a beginner just getting your gears in place or an advanced user looking to fine-tune your start scripts, this article aims to provide valuable insights and tips to enhance your development workflow.
Let’s simplify the process together and enhance our development workflow.
TL;DR: How Do I Use ‘npm start’ in My Project?
The
npm start
command is used in Node.js projects to execute the “start” script defined in thepackage.json
file. You can also directly execute the “start” script usingnpm run start
, which is useful if you need to run other scripts defined in your package.json file.
Here’s an example of a “start” script:
"scripts": {
"start": "node app.js"
}
# Output:
# 'Your Node.js application starts and runs the app.js file.'
Then, run npm start
from your terminal. This simple command kicks off your Node.js application by executing the node app.js
command defined under the start
script in your package.json
file.
This guide will delve deeper into customizing and troubleshooting your start script, ensuring you’re well-equipped to handle various scenarios that might arise during development. Whether you’re setting up a basic project or working on a complex application, understanding the nuances of npm start
can significantly streamline your development process.
Ready to dive deeper? Keep reading for more detailed insights and advanced tips on mastering ‘npm start’.
Table of Contents
Getting Started with ‘npm start’
Embarking on your journey with Node.js, the ‘npm start’ command is your first step towards bringing your project to life. It’s simple, yet powerful, providing a straightforward method to execute your application. Let’s break down how to set up a basic ‘start’ script in your package.json
file, followed by a practical example to illustrate its functionality.
Setting Up Your ‘Start’ Script
To utilize ‘npm start’, your project must include a start
script in its package.json
file. This script tells npm what command to run when npm start
is executed. Here’s how you can set it up:
- Open your project’s
package.json
file. - Add a
scripts
section if it doesn’t already exist. - Define a
start
script that specifies the command to run your application.
Here’s an example of a basic package.json
file with a start
script:
{
"name": "my-nodejs-app",
"version": "1.0.0",
"description": "A simple Node.js application.",
"main": "index.js",
"scripts": {
"start": "node index.js"
}
}
Understanding the ‘npm start’ Execution
After setting up your start
script, running npm start
from your terminal will execute the command defined in the script. For our example, it would run node index.js
, initiating your Node.js application.
npm start
# Output:
# Server running at http://localhost:3000
The output indicates that your application has started and is now running on your specified port, in this case, http://localhost:3000
. This simplicity is the beauty of npm start
: with a single command, you’ve transitioned from code to a running application.
Pros and Cons
Pros:
– Simplifies the process of starting your project.
– Provides a consistent command across different projects.
Cons:
– Limited functionality without customization. The basic use of ‘npm start’ is straightforward but doesn’t tap into the full potential of npm scripts.
Understanding the basic use of ‘npm start’ sets a solid foundation for beginners. As you become more comfortable with Node.js and npm, you’ll discover the power of customizing your start scripts to suit your development needs better.
Advanced Customization: ‘npm start’
As you grow more confident in your Node.js journey, customizing the ‘npm start’ command opens up a new realm of possibilities. This section delves into the intermediate-level techniques of passing arguments and setting environment variables, enhancing the flexibility and efficiency of your development and production workflows.
Passing Arguments to Your Script
One powerful feature of npm scripts is the ability to pass arguments through the ‘npm start’ command. This can be particularly useful for specifying different modes (like development or production) or configuring options without altering your script directly.
Consider the following enhanced package.json
example:
{
"scripts": {
"start": "node app.js",
"start:dev": "npm start -- --mode=development",
"start:prod": "npm start -- --mode=production"
}
}
Running npm run start:dev
or npm run start:prod
now passes the --mode
argument to your application, allowing it to adjust its behavior accordingly.
npm run start:dev
# Output:
# Running in development mode...
This code block demonstrates how arguments can dynamically alter the runtime environment of your application, providing a versatile tool for developers to optimize their projects for different scenarios.
Setting Environment Variables
Another layer of customization involves setting environment variables directly in your ‘npm start’ script. Environment variables are a secure and efficient way to manage configuration settings and sensitive information without hardcoding them into your application.
Here’s how you can modify your start
script to include environment variables:
{
"scripts": {
"start": "NODE_ENV=production node app.js"
}
}
By prefixing your command with NODE_ENV=production
, you’re instructing Node.js to run your application in a production environment. This can influence how libraries behave and optimize performance.
npm start
# Output:
# Application running in production mode.
This example illustrates the immediate impact environment variables can have on the behavior of your application, showcasing their importance in creating adaptable and secure Node.js applications.
The Benefits of Script Customization
Customizing your ‘npm start’ script offers significant advantages:
- Flexibility: Tailor your application’s behavior to fit development, testing, or production needs.
- Security: Safely manage sensitive configurations through environment variables.
- Efficiency: Streamline your development process by automating repetitive tasks.
By mastering these intermediate-level customization techniques, you’re well on your way to optimizing your Node.js projects for any environment, ensuring a smoother, more productive development lifecycle.
Beyond ‘npm start’: Expert Alternatives
While ‘npm start’ is a cornerstone for running Node.js applications, the landscape of development tools offers more sophisticated alternatives for managing and executing your projects. This section explores such tools, focusing on nodemon
as a prime example, to provide insights into when and why you might choose these over the traditional ‘npm start’ command.
Nodemon: Automatic Restarting
nodemon
is a utility that monitors for any changes in your source and automatically restarts your server. It’s particularly useful during development, where frequent changes are made to the code.
To use nodemon
, first, install it globally via npm:
npm install -g nodemon
Then, instead of using ‘npm start’, you can run your application with nodemon
:
nodemon app.js
# Output:
# [nodemon] starting `node app.js`
# [nodemon] watching path(s): *.*
# [nodemon] watching extensions: js,mjs,json
# [nodemon] starting `node app.js` on port 3000
The output demonstrates nodemon
initiating your application and actively monitoring for changes. If a file is modified, nodemon
automatically restarts the application, reflecting those changes instantly without the need for manual restarts.
Weighing Your Options
Benefits of using nodemon
:
– Efficiency: Automatically restarts your application upon file changes, saving time during development.
– Convenience: Eliminates the need to manually stop and start your server after each change.
Drawbacks:
– Overhead: Adds an additional tool to your development stack.
– Complexity: Might require configuration for more complex projects.
Choosing Between ‘npm start’ and Alternatives
Deciding whether to use ‘npm start’ or an alternative like nodemon
depends on your project’s needs. For simple applications or when deploying to production, ‘npm start’ is straightforward and efficient. During development, especially in projects with frequent changes, tools like nodemon
enhance productivity by automating the restart process.
Optimizing Start Scripts for Complexity
For complex projects, combining ‘npm start’ with tools like nodemon
can offer the best of both worlds. Configuring your package.json
to use nodemon
for development while sticking with ‘npm start’ for production ensures a smooth transition between environments.
{
"scripts": {
"start": "node app.js",
"dev": "nodemon app.js"
}
}
This setup allows developers to leverage nodemon
for its auto-restarting capabilities during development (npm run dev
) and maintain the simplicity and reliability of ‘npm start’ for production scenarios.
Understanding and utilizing these expert-level alternatives and optimizations empowers developers to tailor their workflow to the specific demands of each project, ensuring efficiency and adaptability throughout the development lifecycle.
Troubleshooting ‘npm start’ Issues
Even with a smooth-running Node.js project, encountering issues with ‘npm start’ can be a common hurdle. This section aims to demystify some of the frequent challenges developers face, offering practical solutions and preventative measures to ensure a seamless development experience.
‘Missing Script: Start’ Error
One of the most common errors when running ‘npm start’ is the ‘missing script: start’ message. This occurs when the package.json
file does not have a start
script defined.
To resolve this, ensure your package.json
includes a start
script like so:
{
"scripts": {
"start": "node yourMainFile.js"
}
}
After adding the start
script, running npm start
should successfully execute your application:
npm start
# Output:
# Server running on http://localhost:3000
The output confirms your application is now up and running, accessible via the specified URL. This simple fix can save you from unnecessary frustration, highlighting the importance of a correctly configured package.json
.
Handling Environment Variable Mishaps
Another common issue involves environment variables not being recognized, leading to unexpected application behavior. This can often be addressed by ensuring environment variables are properly set and accessed within your application.
For instance, setting an environment variable in your start
script:
{
"scripts": {
"start": "NODE_ENV=production node app.js"
}
}
And accessing it in your application:
console.log('Running in', process.env.NODE_ENV, 'mode.');
# Output:
# Running in production mode.
This code block demonstrates setting the NODE_ENV
environment variable to production
before running your application. The expected output confirms the environment variable is successfully recognized and utilized, ensuring your app behaves as intended under different environments.
Best Practices for Avoiding Future Issues
- Validation: Regularly check your
package.json
for accuracy and completeness. - Documentation: Keep a record of environment variables and their purposes within your project documentation.
- Testing: Test your start script in different environments to catch and resolve issues early.
By addressing these common ‘npm start’ issues head-on and adopting best practices, you can minimize disruptions and maintain a productive development workflow.
Core Concepts of npm Scripts
To truly master ‘npm start’, it’s crucial to understand its place within the broader ecosystem of npm (Node Package Manager) and how it enhances Node.js project management. npm scripts are a fundamental part of this ecosystem, serving as a bridge between the developer’s command line and the project’s needs.
Understanding npm Scripts
npm scripts are defined within the package.json
file of a Node.js project. They are shortcuts or aliases to Node.js commands and other scripts, making development tasks like starting, building, and testing your application more streamlined and consistent across different environments.
Consider this example of a package.json
file with multiple script definitions:
{
"name": "example-project",
"version": "1.0.0",
"scripts": {
"start": "node server.js",
"test": "echo \"Error: no test specified\" && exit 1"
}
}
In this example, running npm start
in your terminal would execute the node server.js
command, starting your Node.js server. Similarly, npm test
would run the specified echo command, although it’s merely a placeholder in this case.
The Role of ‘npm start’
The ‘npm start’ command is specifically designed to run the application’s entry point. It’s a convention within the npm ecosystem that enhances readability and ease of use for developers, especially when collaborating on projects. ‘npm start’ abstracts the underlying command needed to run your application, making the process more intuitive.
npm start
# Output:
# Server running at http://localhost:3000
This output indicates that the application is up and running, accessible via the specified URL. The simplicity of ‘npm start’ belies its power, providing a uniform method to kickstart applications across various Node.js projects.
Importance in Project Management
npm scripts, and ‘npm start’ in particular, play a pivotal role in Node.js project management. They offer a standardized approach to executing common tasks, reducing the cognitive load on developers and ensuring that projects can be easily maintained and scaled. By leveraging npm scripts, teams can automate and streamline their development workflows, leading to more efficient and error-free project execution.
Understanding the fundamentals of npm scripts and the specific function of ‘npm start’ within this framework is essential for any Node.js developer. It not only simplifies the development process but also fosters a more collaborative and organized approach to managing Node.js projects.
Best Practices for ‘npm start’
As your Node.js projects grow in complexity, integrating ‘npm start’ seamlessly into larger development workflows becomes crucial. This section explores how ‘npm start’ interacts with testing frameworks and deployment tools, providing a cohesive and efficient development lifecycle.
Testing Framework Integration
A robust testing strategy is essential for maintaining high-quality code. Integrating ‘npm start’ with popular Node.js testing frameworks like Jest or Mocha can streamline your testing process. Here’s how you can modify your package.json
to include a test script that works alongside your start script:
{
"scripts": {
"start": "node app.js",
"test": "jest"
}
}
Running npm test
will now trigger your Jest tests, ensuring your application is thoroughly tested each time you start your development process. This integration highlights the versatility of ‘npm start’, allowing for both application execution and test suite initiation within the same workflow.
Deployment Automation
When it comes to deploying your Node.js application, ‘npm start’ can be part of an automated pipeline that ensures smooth transitions from development to production environments. Tools like Jenkins or GitHub Actions can execute ‘npm start’ as part of their deployment scripts, automating the launch of your application in various environments.
Here’s an example of a GitHub Actions workflow that includes ‘npm start’:
name: Node.js CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v1
with:
node-version: '14.x'
- name: Install dependencies
run: npm install
- name: Start application
run: npm start
This workflow automates the process of testing, building, and starting your Node.js application on every push to the repository, demonstrating ‘npm start’s’ role in continuous integration and deployment processes.
Further Resources for ‘npm start’ Mastery
To deepen your understanding and mastery of ‘npm start’ and its applications, consider exploring the following resources:
- Node.js Documentation – Official Node.js documentation, including detailed guides on npm scripts.
npm Documentation – Comprehensive resource for all things npm, including ‘npm start’ and script customization.
The Net Ninja’s Node.js Tutorial Series – A practical video tutorial series that covers Node.js basics to advanced concepts, including npm script usage.
These resources provide valuable information and practical examples to further enhance your skills and knowledge in managing Node.js projects with ‘npm start’ and beyond.
Recap: ‘npm start’ Guide for Node.js
In this comprehensive guide, we’ve navigated the essential command ‘npm start’, a fundamental tool for launching Node.js applications. From initializing your project to enhancing your development workflow, ‘npm start’ serves as the ignition key to bring your code to life.
We began with the basics, illustrating how to set up a simple ‘start’ script in your package.json
file. This initial step ensures a smooth takeoff for your Node.js projects, making ‘npm start’ an indispensable command for developers.
Moving forward, we explored advanced customization options, such as passing arguments and setting environment variables. These intermediate techniques unlock the full potential of ‘npm start’, allowing for flexible and efficient project management across different environments.
Our journey also covered expert-level alternatives like using nodemon
for automatic restarting, broadening our understanding of the tools available beyond ‘npm start’. This insight equips developers with the knowledge to choose the best tool for their project’s needs, optimizing their development process.
Approach | Flexibility | Efficiency | Use Case |
---|---|---|---|
‘npm start’ | Basic | High | Starting projects |
Passing Arguments | Moderate | High | Custom configurations |
Environment Variables | High | Moderate | Secure configurations |
nodemon | High | High | Development with frequent changes |
As we wrap up, it’s clear that ‘npm start’ and its advanced uses play a pivotal role in streamlining development workflows. Whether you’re just starting out or looking to refine your Node.js project management, this guide has laid the groundwork for efficient and effective use of ‘npm start’.
With the command’s role in simplifying project execution and encouraging further exploration of npm scripts, developers are well-equipped to manage their Node.js projects with confidence. Happy coding!