Int to String Java Conversion: Methods and Examples
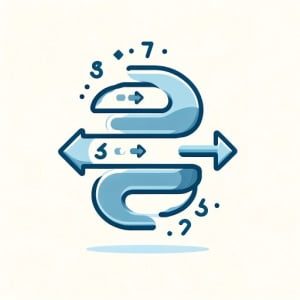
Are you finding it challenging to convert integers to strings in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this task, but there’s a solution.
Think of Java as a skilled interpreter, capable of translating integers into strings. It provides various methods to perform this conversion, each with its own advantages and use cases.
In this guide, we’ll walk you through the process of converting integers to strings in Java, from the basics to more advanced techniques. We’ll cover everything from using the Integer.toString(int)
and String.valueOf(int)
methods, to handling special cases and alternative approaches.
Let’s dive in and start mastering integer to string conversion in Java!
TL;DR: How Do I Convert an Integer to a String in Java?
In Java, you can convert an integer to a string using the
Integer.toString(int)
orString.valueOf(int)
methods. These methods are part of Java’s standard library and are widely used for this type of conversion.
Here’s a simple example:
int num = 123;
String str = Integer.toString(num);
System.out.println(str);
# Output:
# '123'
In this example, we declare an integer num
with a value of 123. We then use the Integer.toString(int)
method to convert this integer into a string. The converted string is stored in the str
variable. Finally, we print out the value of str
, which is ‘123’.
This is a basic way to convert an integer to a string in Java, but there’s much more to learn about this process. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Basic Use: Converting Int to String in Java
- Handling Special Cases: Negative and Large Integers
- Exploring Alternative Methods for Integer to String Conversion
- Troubleshooting Common Conversion Issues
- Understanding Java’s Integer and String Data Types
- Java’s Type System and Type Conversion
- Expanding Your Java Knowledge: Beyond Integer to String Conversion
- Wrapping Up: Integer to String Conversion in Java
Basic Use: Converting Int to String in Java
In Java, two of the most common methods used to convert an integer to a string are Integer.toString(int)
and String.valueOf(int)
. Both methods are straightforward, easy to use, and provide reliable results. Let’s explore each of them.
The Integer.toString(int)
Method
This method is part of the Integer class and is used to convert an integer into a string. Here’s a basic example:
int num = 456;
String str = Integer.toString(num);
System.out.println(str);
# Output:
# '456'
In this example, we’re converting the integer num
into a string using the Integer.toString(int)
method and storing the result in the str
variable. When we print str
, the output is ‘456’.
While this method is simple and effective, it’s important to remember that it can throw a NullPointerException
if the integer is null.
The String.valueOf(int)
Method
The String.valueOf(int)
method is an alternative way to convert an integer to a string. This method is part of the String class and can handle null values without throwing an exception.
Here’s how you can use String.valueOf(int)
:
int num = 789;
String str = String.valueOf(num);
System.out.println(str);
# Output:
# '789'
In this example, we’re using the String.valueOf(int)
method to convert the integer num
into a string. When we print str
, the output is ‘789’.
This method is a safer option if you’re dealing with integers that might be null, as it returns ‘null’ instead of throwing an exception.
Handling Special Cases: Negative and Large Integers
While converting a positive integer to a string in Java is straightforward, dealing with special cases like negative integers and large integers requires some extra consideration.
Converting Negative Integers
Converting a negative integer to a string in Java is as simple as converting a positive integer. Both Integer.toString(int)
and String.valueOf(int)
handle negative integers without any additional steps.
Here’s an example:
int negativeNum = -123;
String str = Integer.toString(negativeNum);
System.out.println(str);
# Output:
# '-123'
In this example, we convert the negative integer -123
to a string using Integer.toString(int)
. The output is ‘-123’, which correctly represents the negative integer.
Handling Large Integers
Large integers can also be converted to strings without any extra steps. However, keep in mind that the maximum value an integer variable can store in Java is 2,147,483,647. Any value beyond this limit will result in an overflow.
Here’s an example of converting a large integer to a string:
int largeNum = 2147483647;
String str = String.valueOf(largeNum);
System.out.println(str);
# Output:
# '2147483647'
In this example, we convert the large integer 2147483647
to a string using String.valueOf(int)
. The output is ‘2147483647’, which correctly represents the large integer.
Remember, if you need to work with numbers larger than Integer.MAX_VALUE
, consider using long
data type or BigInteger
class.
Exploring Alternative Methods for Integer to String Conversion
While Integer.toString(int)
and String.valueOf(int)
are the most commonly used methods for converting integers to strings in Java, there are other alternatives that offer unique advantages. Let’s explore the String.format()
method and the new Integer(int).toString()
approach.
The String.format()
Method
The String.format()
method allows you to convert an integer to a string while also providing options for formatting the output. This can be especially useful when you need to include the integer in a larger string or format the integer in a specific way.
Here’s a simple example:
int num = 101;
String str = String.format("The number is %d", num);
System.out.println(str);
# Output:
# 'The number is 101'
In this example, we use String.format()
to convert the integer num
to a string and include it in a larger string. The %d
in the format string is a placeholder for the integer.
The new Integer(int).toString()
Approach
This approach involves creating a new Integer object and then calling the toString()
method on it. While this method works, it’s generally less efficient than the other methods because it creates a new object.
Here’s how you can use this approach:
int num = 202;
String str = new Integer(num).toString();
System.out.println(str);
# Output:
# '202'
In this example, we create a new Integer object with the value of num
and then call toString()
on it to convert it to a string.
While this method works, it’s generally best to use Integer.toString(int)
or String.valueOf(int)
for converting integers to strings in Java. These methods are more efficient and are more widely used in the Java community.
Method | Advantage | Disadvantage |
---|---|---|
Integer.toString(int) | Simple, efficient | Throws NullPointerException for null values |
String.valueOf(int) | Handles null values | Slightly less efficient than Integer.toString(int) |
String.format() | Offers formatting options | Less efficient for simple conversions |
new Integer(int).toString() | Works, but not commonly used | Less efficient, creates a new object |
Troubleshooting Common Conversion Issues
While converting integers to strings in Java is generally straightforward, there are some common issues you may encounter. Let’s discuss how to handle these problems and ensure your conversions run smoothly.
Dealing with ‘NumberFormatException’
The ‘NumberFormatException’ is a common issue that occurs when trying to convert a string that doesn’t represent a valid integer value. This can be avoided by ensuring the string only contains numerical characters.
Here’s an example of a ‘NumberFormatException’:
String str = "123abc";
int num = Integer.parseInt(str);
# Output:
# Exception in thread "main" java.lang.NumberFormatException: For input string: "123abc"
In this example, we’re trying to convert a string that contains non-numerical characters to an integer, which throws a ‘NumberFormatException’.
Handling Null Values
When converting an integer to a string, a null value can result in a NullPointerException
. To avoid this, you can use the String.valueOf(int)
method, which returns ‘null’ for null integers.
Here’s an example:
Integer num = null;
String str = String.valueOf(num);
System.out.println(str);
# Output:
# 'null'
In this example, we convert a null integer to a string using String.valueOf(int)
. The output is ‘null’, which correctly represents the null integer.
Remember, understanding these common issues and how to handle them can help you avoid errors and ensure your integer to string conversions in Java run smoothly.
Understanding Java’s Integer and String Data Types
Before delving deeper into the process of converting integers to strings in Java, it’s essential to understand the fundamental concepts of Java’s integer and string data types.
Integer Data Type in Java
In Java, the integer data type (int
) is used to store numerical values without a decimal point. It can store values from -2,147,483,648 to 2,147,483,647. It’s one of the most commonly used data types in Java, especially when dealing with numerical operations.
Here’s a simple example of declaring an integer in Java:
int num = 12345;
System.out.println(num);
# Output:
# 12345
In this example, we declare an integer num
with a value of 12345 and print it.
String Data Type in Java
The string data type (String
) in Java is used to store sequences of characters, which can include letters, numbers, and special characters. Strings in Java are objects, not primitive data types.
Here’s a simple example of declaring a string in Java:
String str = "Hello, Java!";
System.out.println(str);
# Output:
# 'Hello, Java!'
In this example, we declare a string str
with a value of ‘Hello, Java!’ and print it.
Java’s Type System and Type Conversion
Java is a statically-typed language, which means the type of every variable must be declared at compile-time. However, Java provides several methods for converting between different data types, known as type conversion.
When it comes to converting an integer to a string in Java, we’re performing a specific type of conversion known as a type casting or type conversion. This process involves taking a value of one type and converting it to another type. In our case, we’re taking a value of type int
and converting it to type String
.
Expanding Your Java Knowledge: Beyond Integer to String Conversion
Converting integers to strings in Java is a fundamental skill, but it’s just the tip of the iceberg. The ability to manipulate and convert data types is crucial in many areas of Java development, from database operations to file I/O, and beyond.
Database Operations
In database operations, you often need to convert integers to strings. For instance, when querying a database, you might need to convert a numeric ID to a string to insert it into a SQL statement.
File I/O
When working with file I/O in Java, you often read data as strings and then convert it to the appropriate data type for processing. Conversely, you might need to convert data to strings before writing it to a file.
Exploring Related Concepts
Once you’re comfortable with converting integers to strings in Java, you might want to explore related concepts like string manipulation and handling numeric data. These skills will further enhance your ability to work with data in Java.
Further Resources for Java
To deepen your understanding of Java and its capabilities, here are some resources that you might find helpful:
- IOFlood’s Java Casting Guide explores how to cast objects and references in Java for polymorphism.
Converting String to Int – Learn techniques to parse and extract integer values from strings.
List to Array in Java – Transform lists into arrays efficiently in Java for flexible data manipulation.
Oracle’s Java Tutorials cover everything from the basics to advanced topics.
Java int to String Conversion – This tutorial on javatpoint explains the various ways to convert an int to a String in Java.
Baeldung’s Guide to Java offers a wealth of tutorials on various aspects of Java, including type conversion.
Wrapping Up: Integer to String Conversion in Java
In this comprehensive guide, we’ve delved into the process of converting integers to strings in Java, a fundamental skill in Java programming. We’ve explored the various methods available for this conversion, their usage, and the potential issues you might encounter.
We began with the basics, learning how to convert integers to strings using the Integer.toString(int)
and String.valueOf(int)
methods. We then moved onto more advanced topics, handling special cases like negative and large integers. We also explored alternative approaches for integer to string conversion, such as using String.format()
and new Integer(int).toString()
methods.
Along the way, we tackled common challenges you might face when converting integers to strings in Java, such as ‘NumberFormatException’ and handling null values, providing you with solutions for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
Integer.toString(int) | Simple, efficient | Throws NullPointerException for null values |
String.valueOf(int) | Handles null values | Slightly less efficient than Integer.toString(int) |
String.format() | Offers formatting options | Less efficient for simple conversions |
new Integer(int).toString() | Works, but not commonly used | Less efficient, creates a new object |
Whether you’re a beginner just starting out with Java, or an experienced developer looking to brush up on your skills, we hope this guide has given you a deeper understanding of how to convert integers to strings in Java.
The ability to manipulate and convert data types is a crucial skill in Java programming. Now, with this guide at your disposal, you’re well equipped to handle integer to string conversion in Java. Happy coding!