NPM Redis Integration | Node.js Application Guide
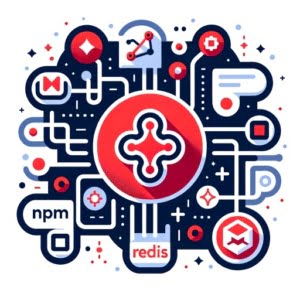
At IOFLOOD, we’ve encountered the need to optimize data storage and retrieval in Node.js applications. When exploring Redis as a solution we found that, when integrated with npm, it offers powerful caching and data management capabilities. We wanted to share this info with others, to help enhance the performance and scalability of applications, and the best way to do so was with this step-by-step guide.
This guide will walk you through the process of integrating Redis into your Node.js projects using npm, from installation to advanced usage. We’ll cover everything from the ground up, ensuring you have a solid foundation to start from. Whether you’re new to Redis or looking to deepen your understanding, this guide is designed to provide you with the knowledge and tools needed to supercharge your Node.js applications with Redis.
Let’s simplify data management together and unlock the full potential of Redis for your Node.js applications!
TL;DR: How Do I Use Redis with npm for My Node.js Application?
To integrate Redis with npm in your Node.js application, start by installing the Redis client package using
npm install redis
. Next, require the Redis client in your Node.js code withconst redis = require('redis')
. Then, initialize a Redis client by creating an instance withconst client = redis.createClient()
.
npm install redis
After installation, incorporate Redis into your Node.js application by requiring and configuring the Redis client. This sets the stage for utilizing Redis’s capabilities to enhance your application’s performance.
Here’s a quick snippet to get you started:
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis...');
});
// Output:
// 'Connected to Redis...'
In this example, we demonstrate how to require the Redis package and establish a basic connection. Notice the console log that confirms the successful connection to Redis. This is just the beginning of what you can achieve with Redis and Node.js.
Eager to dive deeper? Keep reading for a comprehensive guide on setting up, using examples, and exploring advanced configurations with Redis in your Node.js applications.
Table of Contents
- Basic Redis Integration Steps
- Advanced Features with Redis Node.js
- Redis with Node.js Frameworks
- Scaling Redis Operations
- Native vs. Third-Party Libraries
- Common Integration Pitfalls of Redis
- Core Concepts of Redis
- Package Management Role in Node.js
- Practical Usage Cases of Redis
- Recap: Redis and Node.js Integration
Basic Redis Integration Steps
Integrating Redis into your Node.js application is a straightforward process that can significantly enhance your app’s performance. After installing the Redis package via npm, as shown in the TL;DR section, the next step is to require the Redis client in your application and establish a basic connection. Here, we’ll delve into how to perform a simple Redis operation: setting and getting a value. This operation is fundamental but showcases the efficiency and speed of Redis as a data store.
Requiring Redis Client and Connecting
First, ensure you have Redis installed on your system and it’s running. Then, in your Node.js application, require the Redis client and connect to your Redis server with the following code snippet:
const redis = require('redis');
const client = redis.createClient({
host: 'localhost',
port: 6379
});
client.on('error', function(error) {
console.error(`Connection Error: ${error}`);
});
Setting and Getting a Value with Redis
Once connected, you can start utilizing Redis to manage data. Let’s try setting a value and then retrieving it, which is a common operation in many applications:
client.set('key', 'value', redis.print);
client.get('key', function(err, reply) {
if (err) throw err;
console.log('The key value is:', reply);
});
# Output:
# Reply: OK
# The key value is: value
In the code above, client.set
is used to store a value (‘value’) with a corresponding key (‘key’). The redis.print
function is a helpful callback that prints the result of the Redis command to the console. Following this, client.get
retrieves the value associated with the given key. The expected output confirms the successful setting and retrieval of the value, demonstrating the simplicity and efficiency of performing basic operations in Redis.
Understanding these basic operations is crucial as they form the foundation for more complex data management and manipulation tasks in Redis. This simple example illustrates how Redis can be used to quickly store and access data, making it an invaluable tool for enhancing the performance and scalability of your Node.js applications.
Advanced Features with Redis Node.js
As you become more comfortable with basic Redis operations within your Node.js applications, it’s time to explore some of Redis’s more sophisticated capabilities. These advanced features can significantly enhance the functionality and performance of your applications. Let’s dive into pub/sub messaging, working with hashes and lists, and implementing session storage—each with practical code examples.
Pub/Sub Messaging with Redis
Redis’s publish/subscribe (pub/sub) messaging system allows messages to be sent between different parts of an application. This is particularly useful for real-time features like chat applications or live updates.
const subscriber = redis.createClient();
const publisher = redis.createClient();
subscriber.subscribe('channel');
subscriber.on('message', (channel, message) => {
console.log(`Received message: ${message} on channel: ${channel}`);
});
publisher.publish('channel', 'Hello, Redis pub/sub!');
# Output:
# Received message: Hello, Redis pub/sub! on channel: channel
In this example, we create both a publisher and a subscriber client. The subscriber listens to messages on a specific channel, while the publisher sends a message to that channel. This demonstrates how Redis can facilitate communication between different parts of an application in real time.
Working with Hashes and Lists
Redis supports various data structures, including hashes and lists, which are essential for storing collections of data.
Hashes Example
client.hmset('user:100', 'firstName', 'John', 'lastName', 'Doe', redis.print);
client.hgetall('user:100', (err, obj) => {
console.log(obj);
});
# Output:
# { firstName: 'John', lastName: 'Doe' }
This code snippet demonstrates how to store and retrieve user information using a hash. hmset
is used to set multiple fields in a hash, and hgetall
retrieves all fields and values in the hash, making it perfect for objects like user profiles.
Lists Example
client.rpush(['tasks', 'Task 1', 'Task 2'], redis.print);
client.lrange('tasks', 0, -1, (err, tasks) => {
console.log(tasks);
});
# Output:
# ['Task 1', 'Task 2']
Here, rpush
adds elements to the end of a list named ‘tasks’, and lrange
retrieves elements from the list. Lists are ideal for queues, timelines, or simply storing ordered data.
Implementing Session Storage
Storing session data in Redis can significantly improve the performance of your Node.js applications by ensuring quick access to session information.
// Assuming express-session and connect-redis are used
const session = require('express-session');
const RedisStore = require('connect-redis')(session);
app.use(session({
store: new RedisStore({ client: redisClient }),
secret: 'keyboard cat',
resave: false,
saveUninitialized: true
}));
This snippet shows how to configure the session middleware for Express.js applications to use Redis for session storage. It leverages connect-redis
as the session store, which automatically manages sessions in Redis, providing fast and reliable access to session data.
By harnessing these advanced Redis features, you can build more sophisticated, efficient, and scalable Node.js applications. Each feature offers unique benefits, from real-time communication to efficient data storage and retrieval, making Redis an invaluable tool in your development arsenal.
Redis with Node.js Frameworks
When integrating Redis into Node.js applications, especially those built with advanced frameworks like Express.js, developers have the opportunity to leverage Redis for a variety of purposes, from session storage to caching. Express.js, being one of the most popular Node.js frameworks, offers a seamless way to integrate Redis, enhancing application performance and user experience.
Express.js and Redis for Session Storage
const express = require('express');
const redis = require('redis');
const session = require('express-session');
let RedisStore = require('connect-redis')(session);
let redisClient = redis.createClient();
const app = express();
app.use(session({
store: new RedisStore({ client: redisClient }),
secret: 'secret',
resave: false,
saveUninitialized: false
}));
In this example, connect-redis
is used with Express.js to manage session data in Redis. This setup ensures session data is stored in a fast, efficient, and scalable manner. By storing session information in Redis, applications can handle larger volumes of traffic without a decline in performance.
Scaling Redis Operations
Scaling Redis operations is crucial for applications requiring high availability and performance. Redis clusters offer a way to achieve this by distributing data across multiple Redis nodes, ensuring data persistence and load balancing.
Implementing Redis Clusters
const cluster = require('redis-cluster').createCluster({
servers: [
{ host: '127.0.0.1', port: 7000 },
{ host: '127.0.0.1', port: 7001 }
]
});
This code snippet demonstrates the initialization of a Redis cluster with two nodes. Redis clusters enhance application performance by distributing operations across several nodes, thus handling more requests and maintaining high availability.
Native vs. Third-Party Libraries
When working with Redis in Node.js applications, developers must decide between using native Redis commands or third-party libraries. While native commands offer more control and direct interaction with Redis, third-party libraries like ioredis
provide additional functionalities, such as built-in support for clusters, promises, and more.
Example Using ioredis
const Redis = require('ioredis');
const redis = new Redis();
redis.set('hello', 'world');
redis.get('hello', (err, result) => {
console.log(result);
});
# Output:
# world
This example illustrates the simplicity of using ioredis
for basic Redis operations, such as setting and getting values. The library simplifies interaction with Redis, making it accessible for developers to incorporate advanced Redis features into their Node.js applications.
Choosing between native Redis commands and third-party libraries depends on the specific requirements of your project and your familiarity with Redis. Both approaches offer unique advantages, and understanding the capabilities and limitations of each can help you make an informed decision that best suits your application’s needs.
Common Integration Pitfalls of Redis
Integrating Redis with your Node.js application using npm can significantly enhance your app’s performance and scalability. However, like any technology integration, it’s not without its challenges. Understanding common pitfalls and how to troubleshoot them can save you time and frustration.
Connection Issues
One of the first hurdles you might encounter is connection issues. Ensuring your Redis server is running and accessible from your Node.js application is crucial.
const redis = require('redis');
const client = redis.createClient({
host: 'localhost',
port: 6379
});
client.on('error', function(err) {
console.log('Error ' + err);
});
In this code snippet, we’re setting up a basic Redis client connection. If there’s an issue connecting to the Redis server, an error event will be triggered, and the error will be logged to the console. This simple setup helps in identifying connection issues early in the development process.
Handling Redis Errors
Properly handling errors in your Redis operations is vital for maintaining a robust application. Redis errors can occur for various reasons, including command syntax errors, attempting operations on the wrong data type, or exceeding memory limits.
client.set('key', 'value', function(err, reply) {
if (err) {
console.error('Redis set error:', err);
return;
}
console.log('Set operation successful:', reply);
});
# Output:
# Set operation successful: OK
This example demonstrates handling errors during a set
operation. If an error occurs, it’s logged to the console, otherwise, the successful operation message is displayed. Error handling ensures that your application can gracefully manage issues and continue operating.
Debugging Tips
Debugging issues with Redis in Node.js applications can be tricky, especially when dealing with asynchronous operations. Using console.log
to print out values at different stages of your Redis operations can help pinpoint where things are going wrong. Additionally, the Redis CLI can be an invaluable tool for directly interacting with your Redis instance to test commands and inspect data.
Best Practices for Secure and Efficient Redis Usage
- Use Redis passwords: Securing your Redis instance with a password helps protect against unauthorized access.
- Implement SSL/TLS: If your application communicates with Redis over a network, using SSL/TLS encryption can safeguard your data in transit.
- Optimize data structures: Choosing the right Redis data structures and using them efficiently can significantly impact your application’s performance.
- Monitor memory usage: Keeping an eye on Redis memory usage and setting appropriate limits can prevent issues related to memory exhaustion.
By being aware of these common pitfalls and adhering to best practices, you can ensure a smooth and successful integration of Redis with your Node.js application using npm. Remember, the key to effectively using Redis is not just in its implementation but also in how you handle the challenges that come with it.
Core Concepts of Redis
Redis stands out in the world of database management systems for its speed and efficiency. At its core, Redis is an in-memory data structure store, capable of serving as a database, cache, and message broker. Redis supports various data structures such as strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs, and geospatial indexes with radius queries. This versatility allows developers to use Redis for a wide range of scenarios, from caching web pages for rapid loading times to managing real-time analytics and leaderboards.
Key Features of Redis
- Speed: Being an in-memory store, Redis delivers unparalleled speed, making it ideal for scenarios where rapid access to data is critical.
- Versatility: Redis supports a variety of data structures, allowing for flexible data management strategies.
- Scalability: Redis can be easily scaled to accommodate growing data needs, ensuring your application remains efficient as it grows.
Package Management Role in Node.js
npm, standing for Node Package Manager, plays a crucial role in the Node.js ecosystem. It is the largest software registry in the world, allowing developers to share and borrow packages. npm makes it easy to manage dependencies in Node.js projects, ensuring that you have the right tools and libraries at your disposal.
npm install redis
This command installs the Redis client library for Node.js, enabling your application to interact with a Redis server. It’s a simple yet powerful step towards integrating Redis into your project.
The Synergy Between Redis and Node.js
The combination of Redis and Node.js can significantly boost the performance and scalability of applications. Node.js’s non-blocking I/O model pairs excellently with Redis’s speed, allowing for high-throughput, low-latency applications that can handle large volumes of requests simultaneously. Whether you’re building a social media platform, an e-commerce site, or a real-time analytics dashboard, integrating Redis with your Node.js application can provide a substantial performance advantage.
By understanding the fundamentals of Redis and npm, developers can leverage these powerful tools to build more efficient, scalable, and high-performing applications. The synergy between Redis and Node.js is a game-changer for many projects, offering a combination of speed, efficiency, and scalability that is hard to beat.
Practical Usage Cases of Redis
Redis, while renowned for its speed as an in-memory data store, also offers capabilities for persistent storage, ensuring that your data isn’t lost even after a system restart. This feature is crucial for applications requiring data durability alongside high performance.
Redis Persistence Options
Redis provides two main mechanisms for data persistence: RDB (Redis Database) snapshots and AOF (Append Only File) logging.
# Example of forcing an RDB snapshot manually
redis-cli bgsave
# Expected output:
# Background saving started
In this example, bgsave
triggers a background save process, creating a snapshot of the database. This operation allows Redis to recover its state after a restart, ensuring data durability.
Integrating Redis into microservices architectures enhances scalability and performance. Redis can act as a stateful component within a stateless microservices ecosystem, facilitating quick data access and message passing between services.
Leveraging Redis for Real-Time Analytics
Real-time analytics is another area where Redis excels, thanks to its high throughput and low latency. Applications can process and analyze data in real-time, providing insights and responses without delay.
// Incrementing a page view counter
client.incr('page_views');
client.get('page_views', (err, reply) => {
console.log(`Total page views: ${reply}`);
});
# Output:
# Total page views: 1
This simple code snippet demonstrates using Redis to increment and retrieve a page view counter. Such real-time analytics can be crucial for applications requiring immediate data insights, like dashboards or monitoring systems.
Further Resources for Redis Mastery
To deepen your understanding and mastery of Redis and its integration with Node.js, consider exploring the following resources:
- Redis Official Documentation – A comprehensive guide to all things Redis, from basic commands to advanced configuration.
Node.js Redis Client Library – The official GitHub repository for the Node.js Redis client library, offering insights into its usage and examples.
Building Scalable Node.js Applications with Redis – An insightful article by Redis Labs on leveraging Redis for building scalable Node.js applications.
By exploring these resources, you can expand your knowledge on persistent storage, microservices architectures, and real-time analytics with Redis. The journey towards Redis mastery is continuous, and these resources will provide valuable guidance along the way.
Recap: Redis and Node.js Integration
In this comprehensive guide, we’ve explored the powerful combination of Redis and Node.js to enhance application performance and scalability. Starting with the basics, we demonstrated how to integrate Redis into your Node.js projects using npm, ensuring a solid foundation for developers new to Redis.
We began with the fundamental steps of installing the Redis package via npm and establishing a basic connection. This initial setup is crucial for developers to understand the ease with which Redis can be incorporated into Node.js applications, offering immediate performance gains.
Next, we ventured into more advanced Redis functionalities, such as pub/sub messaging, working with hashes and lists, and implementing session storage. These intermediate-level concepts showcased Redis’s versatility and how it can be utilized for more than just caching—transforming it into a dynamic tool for real-time applications and complex data management.
Finally, we discussed alternative approaches for expert-level developers, including integrating Redis with advanced Node.js frameworks like Express.js, scaling Redis operations through clustering, and the choice between using native Redis commands versus third-party libraries. These insights are aimed at helping developers make informed decisions based on their application requirements.
Feature | Benefit |
---|---|
Basic Integration | Quick setup and immediate performance improvement |
Advanced Functionalities | Enhanced versatility for complex applications |
Scalability Options | High availability and performance even under load |
Whether you’re just starting out with Redis or looking to deepen your expertise, we hope this guide has provided you with a clear roadmap for integrating Redis into your Node.js applications using npm. The journey from basic setup to advanced configurations and troubleshooting has highlighted the benefits of using Redis for enhancing application performance and scalability.
Redis, with its high speed and efficiency, combined with the non-blocking I/O model of Node.js, offers a compelling solution for building scalable, high-performance applications. As you continue to explore and implement Redis in your projects, remember the key takeaways from this guide to ensure a smooth and successful integration. Happy coding!