How to Compare Dates in JavaScript
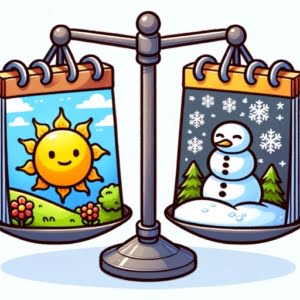
Ever felt like you’re wrestling with JavaScript dates? You’re not alone. Many developers find themselves puzzled when it comes to handling dates in JavaScript, but we’re here to help.
Think of JavaScript’s date handling as a time machine – it allows us to navigate through time and compare different dates, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of comparing dates in JavaScript, from their creation, manipulation, and usage. We’ll cover everything from the basics of date comparison to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Compare Dates in JavaScript?
To compare dates in JavaScript, you can convert them to milliseconds since the Unix Epoch using the
getTime()
method. This allows you to easily compare two dates regardless of their format.
Here’s a simple example:
var date1 = new Date('2021-01-01');
var date2 = new Date('2021-12-31');
console.log(date1.getTime() < date2.getTime());
// Output:
// true
In this example, we create two Date objects, date1
and date2
, representing the dates ‘2021-01-01’ and ‘2021-12-31’ respectively. We then use the getTime()
method to convert these dates to milliseconds since the Unix Epoch, and compare them using the less than operator (` This is a basic way to compare dates in JavaScript, but there’s much more to learn about handling and comparing dates in JavaScript. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Date Comparison in JavaScript
- Handling Time Zones and Formats
- Exploring Third-Party Libraries for Date Comparison
- Troubleshooting Common Issues in JavaScript Date Comparison
- Understanding JavaScript’s Date Object
- The Relevance and Applications of Date Comparison
- Further Resources for Mastering JavaScript Date and Time
- Wrapping Up: Date Comparison in JavaScript
Basic Date Comparison in JavaScript
In JavaScript, the most fundamental way to compare dates is by using the Date
object and the getTime()
method. The Date
object is used to work with dates and times, while the getTime()
method returns the number of milliseconds since the Unix Epoch (January 1, 1970 00:00:00 UTC).
Here’s how you can use these to compare dates:
var date1 = new Date('2022-01-01');
var date2 = new Date('2022-12-31');
if (date1.getTime() < date2.getTime()) {
console.log('Date1 is earlier than Date2');
} else {
console.log('Date1 is later than Date2');
}
// Output:
// 'Date1 is earlier than Date2'
In this example, we’re creating two Date
objects, date1
and date2
. We then use the getTime()
method to convert these dates to milliseconds since the Unix Epoch. As date1
is earlier than date2
, the getTime()
method returns a smaller number for date1
, and our comparison date1.getTime() < date2.getTime()
returns true
. Hence, the output is ‘Date1 is earlier than Date2’.
Advantages and Potential Pitfalls
This method is straightforward and works well for simple comparisons. However, it doesn’t consider time zones or different date formats, which can lead to unexpected results. In the next section, we’ll explore how to handle these complexities.
Handling Time Zones and Formats
When comparing dates in JavaScript, it’s crucial to consider time zones and different date formats. This can be done using methods such as toISOString()
and toLocaleDateString()
.
Dealing with Time Zones
The toISOString()
method returns a string in simplified extended ISO format (ISO 8601), which is always 24 or 27 characters long (YYYY-MM-DDTHH:mm:ss.sssZ or ±YYYYYY-MM-DDTHH:mm:ss.sssZ). The timezone is always zero UTC offset, as denoted by the suffix ‘Z’.
Let’s see how this works:
var date1 = new Date('2022-01-01T00:00:00Z'); // UTC time
var date2 = new Date('2022-01-01T00:00:00'); // Local time
console.log(date1.toISOString());
console.log(date2.toISOString());
// Output:
// '2022-01-01T00:00:00.000Z'
// '2022-01-01T05:30:00.000Z' (assuming IST timezone)
In this example, date1
is created with a UTC time string, while date2
is created with a local time string. When we print the ISO string, we can see the difference in time due to the timezone.
Dealing with Different Formats
The toLocaleDateString()
method returns a string representing the date portion of a Date object, formatted to the language and options specified. If no locale is specified, the default is the user’s locale.
Here’s an example:
var date = new Date('2022-01-01T00:00:00Z'); // UTC time
console.log(date.toLocaleDateString('en-US')); // US format
console.log(date.toLocaleDateString('de-DE')); // German format
// Output:
// '12/31/2021'
// '31.12.2021'
In this example, the same date is formatted in different ways according to the specified locale.
Best Practices
When comparing dates, it’s important to be aware of these differences and handle them appropriately. Always consider time zones, especially when dealing with servers located in different geographical locations. Also, when displaying dates, consider the locale of your users to provide a familiar format.
Exploring Third-Party Libraries for Date Comparison
Beyond the built-in JavaScript Date object and its methods, there are additional ways to compare dates using third-party libraries. Two popular choices are Moment.js and date-fns.
Using Moment.js for Date Comparison
Moment.js is a comprehensive, yet lightweight, JavaScript date library that allows for easy parsing, validating, manipulating, and formatting of dates.
Here’s an example of how you can compare dates using Moment.js:
var moment = require('moment');
var date1 = moment('2022-01-01');
var date2 = moment('2022-12-31');
console.log(date1.isBefore(date2));
// Output:
// true
In this example, we’re using the isBefore()
method from Moment.js to compare two dates. This method returns true
if date1
is before date2
.
Using date-fns for Date Comparison
date-fns is a modern JavaScript date utility library, which provides a set of consistent, clear, and simple functions for manipulating JavaScript dates.
Here’s how you can compare dates using date-fns:
var { isBefore, parseISO } = require('date-fns');
var date1 = parseISO('2022-01-01');
var date2 = parseISO('2022-12-31');
console.log(isBefore(date1, date2));
// Output:
// true
In this example, we’re using the isBefore()
and parseISO()
functions from date-fns to compare two dates. The isBefore()
function returns true
if date1
is before date2
.
Advantages and Disadvantages
Both Moment.js and date-fns offer a range of features for date manipulation and comparison, and can handle complex scenarios with ease. However, they add an extra dependency to your project and may be overkill for simple tasks. Therefore, consider your project’s requirements and the complexity of the date operations you need to perform when deciding whether to use these libraries.
Troubleshooting Common Issues in JavaScript Date Comparison
When comparing dates in JavaScript, you may encounter a few common issues. Let’s discuss these problems and provide solutions and workarounds.
Dealing with Time Zones
One of the most common issues is handling time zones. JavaScript’s Date object uses the local time zone by default, which can lead to unexpected results when comparing dates from different time zones.
Here’s an example:
var date1 = new Date('2022-01-01T00:00:00Z'); // UTC time
var date2 = new Date('2022-01-01T00:00:00'); // Local time
console.log(date1.getTime() === date2.getTime());
// Output:
// false (assuming your local time zone is not UTC)
In this example, date1
is created with a UTC time string, while date2
is created with a local time string. Although the strings look identical, the getTime()
method returns different values because of the time zone difference.
To avoid this problem, always be explicit about the time zone when creating Date objects.
Handling Daylight Saving Time
Another issue is dealing with daylight saving time (DST). In regions that observe DST, the clock is set forward by one hour in the spring (‘spring forward’) and set back by one hour in the fall (‘fall back’) to extend evening daylight.
This can affect date comparison if the dates you’re comparing fall in different DST periods. To avoid this issue, use UTC times for date comparison.
Using the Right Comparison Operator
When comparing dates, ensure you’re using the correct comparison operator. For example, to check if two dates are the same, don’t use the ==
or ===
operators, as they check if the Date objects are the same, not if the dates they represent are the same. Instead, use the getTime()
method for comparison.
Here’s an example:
var date1 = new Date('2022-01-01');
var date2 = new Date('2022-01-01');
console.log(date1 === date2);
console.log(date1.getTime() === date2.getTime());
// Output:
// false
// true
In this example, date1 === date2
returns false
because it’s checking if date1
and date2
are the same object, which they’re not. However, date1.getTime() === date2.getTime()
returns true
because it’s checking if date1
and date2
represent the same time, which they do.
Understanding JavaScript’s Date Object
Before diving deeper into date comparison, it’s crucial to understand JavaScript’s Date object and its methods. The Date object is a built-in object in JavaScript that allows us to create, read, update, and delete dates.
Here’s how you create a Date object:
var date = new Date();
console.log(date);
// Output:
// Current date and time
In this example, we’re creating a new Date object without any arguments, which gives us the current date and time.
JavaScript Date Methods
The Date object comes with a variety of methods for manipulating and retrieving dates. Here are a few key ones:
getTime()
: Returns the number of milliseconds since the Unix Epoch.getFullYear()
: Returns the year of the specified date according to local time.getMonth()
: Returns the month of the specified date according to local time, where 0 indicates January, 1 indicates February, and so on.getDate()
: Returns the day of the month of the specified date according to local time.getHours()
,getMinutes()
,getSeconds()
,getMilliseconds()
: Return the hours, minutes, seconds, and milliseconds of the specified date according to local time, respectively.
The Unix Epoch and Time Zones
The Unix Epoch is the point where time begins, and is universally recognized as 00:00:00 UTC Thursday, 1 January 1970. All time-related computations in JavaScript are based on this epoch.
Time zones are regions of the earth that have the same standard time. JavaScript’s Date object uses the local time zone by default, but we can also work with UTC (Coordinated Universal Time) or any other time zone.
Understanding these fundamentals about JavaScript’s Date object, its methods, the Unix Epoch, and time zones will help you better grasp the concepts underlying the comparison of dates.
The Relevance and Applications of Date Comparison
Comparing dates in JavaScript has a wide range of applications. It’s not just about comparing two dates and determining which one is earlier or later. It’s about the ability to handle and manipulate time, which is a crucial aspect of many applications.
Scheduling Tasks
One common use case is scheduling tasks. For example, you might want to run a certain task every day at a specific time. By comparing the current date and time with the scheduled date and time, you can determine whether to run the task.
Creating Calendars
Another application is in creating calendars. Calendars involve a lot of date comparisons, such as determining the number of days in a month, the day of the week a month starts on, and so on.
Exploring Related Concepts
Beyond date comparison, there are other related concepts in JavaScript that are worth exploring. These include date manipulation (adding or subtracting days, months, or years from a date) and date formatting (displaying a date in a specific format).
Further Resources for Mastering JavaScript Date and Time
To deepen your understanding of JavaScript dates, here are some resources that you might find useful:
- MDN Web Docs: Date: This is a comprehensive guide to the Date object in JavaScript, including its methods and usage.
JavaScript.info: Date and time: This tutorial covers the basics of dates in JavaScript, as well as more advanced topics like date comparison and formatting.
You Don’t Know JS: Dates & Time: This chapter from the popular ‘You Don’t Know JS’ book series provides an in-depth look at dates and time in JavaScript.
Wrapping Up: Date Comparison in JavaScript
In this comprehensive guide, we’ve journeyed through the intricate world of JavaScript dates, focusing on how to compare them effectively.
We started with the basics, learning how to compare dates using the JavaScript Date object and its getTime()
method. We then ventured into more complex territory, exploring how to handle time zones and different date formats using methods like toISOString()
and toLocaleDateString()
.
Next, we tackled common challenges you might encounter when comparing dates, such as dealing with time zones, daylight saving time, and using the correct comparison operator. We provided solutions and workarounds for each issue, equipping you with the knowledge to overcome these hurdles.
We also explored alternative approaches to date comparison, diving into third-party libraries like Moment.js and date-fns. These libraries offer powerful tools for date manipulation and comparison, but they also add extra dependencies to your project.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Dependency |
---|---|---|---|
JavaScript Date and getTime() | Moderate | Low | No |
Moment.js | High | Moderate | Yes |
date-fns | High | Moderate | Yes |
Whether you’re just starting out with JavaScript or you’re looking to level up your date handling skills, we hope this guide has given you a deeper understanding of how to compare dates in JavaScript.
With its balance of flexibility and complexity, JavaScript provides a range of options for date comparison. Now, you’re well equipped to tackle any date-related challenges. Happy coding!