Java .compareTo() Method: Ordering Strings by the Letter
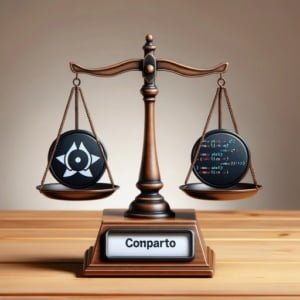
Ever felt like you’re wrestling with the compareTo method in Java? You’re not alone. Many developers find the compareTo method a bit challenging. Think of Java’s compareTo as a race referee – it determines who comes first, second, or third.
The compareTo method is a powerful tool to compare strings in Java, making it extremely popular for sorting and comparing data.
In this guide, we’ll walk you through the process of using compareTo in Java, from the basics to more advanced techniques. We’ll cover everything from making simple comparisons using compareTo, handling different types of data, to dealing with special cases and even troubleshooting common issues.
Let’s kick things off and learn to master the compareTo method in Java!
TL;DR: How Do I Use compareTo in Java?
The compareTo method in Java is used for comparing two strings lexicographically with the syntax,
str1.compareTo(str2);
. The method returns a negative integer, zero, or a positive integer as the first string is less than, equal to, or greater than the second.
Here’s a simple example:
String str1 = "apple";
String str2 = "banana";
int result = str1.compareTo(str2);
// Output:
// The output will be a negative number as 'apple' comes before 'banana' in lexicographical order.
In this example, we have two strings: ‘apple’ and ‘banana’. We use the compareTo method to compare these two strings. The method returns a negative number because ‘apple’ comes before ‘banana’ in lexicographical order.
This is just a basic way to use the compareTo method in Java, but there’s much more to learn about comparing strings and handling special cases. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- A Beginner’s Guide to Using compareTo in Java
- Intermediate-Level Uses of compareTo in Java
- Exploring Alternatives to compareTo in Java
- Troubleshooting Common compareTo Issues in Java
- The Mechanics of String Comparison in Java
- Leveraging compareTo in Larger Java Projects
- Wrapping Up: Java .compareTo() Method
A Beginner’s Guide to Using compareTo in Java
The compareTo method is a fundamental part of Java, especially when you need to sort or compare strings. Let’s dive into how you can use it in your code.
Step-by-Step Usage of compareTo
Here’s a simple example of how you can use the compareTo method in Java:
String str1 = "apple";
String str2 = "banana";
int result = str2.compareTo(str1);
// Output:
// The output will be a positive number as 'banana' comes after 'apple' in lexicographical order.
In this example, we’re comparing ‘apple’ to ‘banana’. The compareTo method returns a negative number because ‘apple’ comes before ‘banana’ in lexicographical order.
Understanding compareTo Results
The compareTo method returns three types of results:
- A negative integer if the first string comes before the second string in lexicographical order.
- Zero if the two strings are equal.
- A positive integer if the first string comes after the second string in lexicographical order.
Advantages and Pitfalls of compareTo
The compareTo method is a powerful tool for comparing strings in Java. It’s simple, straightforward, and works well for most scenarios. However, it has its pitfalls. It’s case-sensitive and treats uppercase and lowercase letters differently, which can lead to unexpected results. Moreover, it doesn’t handle null values and will throw a NullPointerException if you try to compare a string with null.
In the following sections, we’ll delve into more complex uses of the compareTo method and how to handle these potential pitfalls.
Intermediate-Level Uses of compareTo in Java
As you become more familiar with Java’s compareTo method, you’ll find it can handle more complex scenarios beyond simple string comparisons. Let’s explore some of these advanced uses.
Comparing Objects with compareTo
Java’s compareTo method isn’t limited to comparing strings. It can also compare custom objects if they implement the Comparable interface. Let’s consider an example:
public class Fruit implements Comparable<Fruit> {
private String name;
public Fruit(String name) {
this.name = name;
}
@Override
public int compareTo(Fruit otherFruit) {
return this.name.compareTo(otherFruit.name);
}
}
// Usage:
Fruit apple = new Fruit("apple");
Fruit banana = new Fruit("banana");
int result = apple.compareTo(banana);
// Output:
// The output will be a negative number as 'apple' comes before 'banana' in lexicographical order.
In this example, we have a Fruit class that implements the Comparable interface. The compareTo method is overridden to compare the names of the fruit. When we compare an ‘apple’ to a ‘banana’, we get a negative number because ‘apple’ comes before ‘banana’ in lexicographical order.
Dealing with Null Values
By default, the compareTo method will throw a NullPointerException if you try to compare a string with null. However, you can handle this situation gracefully by adding a null check before calling compareTo. Here’s an example:
String str1 = null;
String str2 = "banana";
int result = (str1 == null) ? -1 : str1.compareTo(str2);
// Output:
// The output will be -1 as we return -1 when str1 is null.
In this code block, we check if str1 is null before calling compareTo. If str1 is null, we return -1. Otherwise, we proceed with the comparison.
These advanced uses of compareTo can help you handle more complex scenarios in your Java code. In the next section, we’ll explore alternative approaches to comparing strings in Java.
Exploring Alternatives to compareTo in Java
The compareTo method is a powerful tool for comparing strings in Java, but it’s not the only one. There are alternatives such as using equals() or ==
operator. Let’s take a deeper look at these alternatives and their implications.
Using equals() Method
The equals() method checks if two strings are identical. Here’s how you can use it:
String str1 = "apple";
String str2 = "apple";
boolean result = str1.equals(str2);
// Output:
// The output will be true as both strings are identical.
In this example, equals() returns true because both strings are identical. Unlike compareTo, equals() only checks for equality, not lexicographical order.
Using ==
Operator
The ==
operator checks if two string references point to the same object. Let’s see it in action:
String str1 = new String("apple");
String str2 = new String("apple");
boolean result = (str1 == str2);
// Output:
// The output will be false as str1 and str2 point to different objects.
In this case, even though the strings are identical, the ==
operator returns false because str1 and str2 point to different objects.
Making the Right Choice
Choosing between compareTo, equals(), and ==
depends on your specific use case. If you need to sort strings or determine their lexicographical order, compareTo is your best bet. For checking string equality, equals() is a better option. If you need to check if two string references point to the same object, the ==
operator is the way to go.
Keep these alternatives in mind as you work with strings in Java. In the next section, we’ll delve into common issues and considerations when using compareTo.
Troubleshooting Common compareTo Issues in Java
While the compareTo method is a powerful tool in Java, it’s not without its quirks. Developers often encounter issues related to case sensitivity and special characters. Let’s delve into these common issues and their solutions.
Dealing with Case Sensitivity
The compareTo method is case-sensitive, which means it treats uppercase and lowercase letters differently. Here’s an example:
String str1 = "Apple";
String str2 = "apple";
int result = str1.compareTo(str2);
// Output:
// The output will be a positive number as 'Apple' comes after 'apple' in lexicographical order.
In this example, even though the two strings look similar, compareTo returns a positive number because uppercase ‘A’ comes after lowercase ‘a’ in the Unicode table.
To ignore case sensitivity, you can use the compareToIgnoreCase method. Here’s how:
String str1 = "Apple";
String str2 = "apple";
int result = str1.compareToIgnoreCase(str2);
// Output:
// The output will be 0 as 'Apple' and 'apple' are considered equal when ignoring case.
This time, compareToIgnoreCase returns 0, considering ‘Apple’ and ‘apple’ equal.
Handling Special Characters
Special characters can also lead to unexpected results with compareTo. Here’s an example:
String str1 = "apple!";
String str2 = "apple";
int result = str1.compareTo(str2);
// Output:
// The output will be a positive number as 'apple!' comes after 'apple' in lexicographical order.
In this case, ‘apple!’ comes after ‘apple’ in lexicographical order because the exclamation mark has a higher Unicode value than the end of a string.
Understanding these common issues and their solutions can help you use compareTo effectively in your Java code. In the next section, we’ll explore the fundamentals of string comparison in Java.
The Mechanics of String Comparison in Java
Understanding the fundamentals of how string comparison works in Java is crucial to effectively using methods like compareTo. Let’s dive into the concept of lexicographical order and the role of Unicode values.
Lexicographical Order Explained
Lexicographical order, also known as dictionary order or alphabetical order, is a system used to arrange words based on the alphabetical order of their component letters. It’s the method Java uses when comparing strings.
Here’s a simple example of lexicographical comparison:
String str1 = "cat";
String str2 = "dog";
int result = str1.compareTo(str2);
// Output:
// The output will be a negative number as 'cat' comes before 'dog' in lexicographical order.
In this example, ‘cat’ comes before ‘dog’ in lexicographical order, so compareTo returns a negative number.
The Role of Unicode Values
Java uses Unicode values to determine the lexicographical order. Each character has a unique Unicode value, and these values are used when comparing strings. For example, the Unicode value of ‘a’ is 97, while ‘b’ is 98. Therefore, ‘a’ comes before ‘b’ in lexicographical order.
Here’s an example that illustrates this:
String str1 = "a";
String str2 = "b";
int result = str1.compareTo(str2);
// Output:
// The output will be a negative number as 'a' comes before 'b' in lexicographical order.
In this code, ‘a’ comes before ‘b’ in lexicographical order because its Unicode value is less than that of ‘b’.
Understanding these fundamentals will help you better grasp how Java’s compareTo method works and how strings are compared in Java.
Leveraging compareTo in Larger Java Projects
The compareTo method is not just for comparing strings or custom objects. It can also play an integral role in larger projects, such as sorting lists or filtering data. Understanding how to use compareTo in these contexts can greatly enhance your Java programming skills.
Sorting Lists with compareTo
In Java, you can use the compareTo method to sort lists of strings. Here’s an example:
import java.util.Arrays;
import java.util.List;
List<String> fruits = Arrays.asList("banana", "apple", "cherry");
Collections.sort(fruits);
// Output:
// The list will be sorted in lexicographical order: ['apple', 'banana', 'cherry']
In this example, we have a list of fruits that we sort using Collections.sort. The sort method uses compareTo under the hood to arrange the strings in lexicographical order.
Filtering Data with compareTo
You can also use compareTo to filter data. For example, you might want to filter a list of names to find those that come before ‘m’ in lexicographical order:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Dave");
List<String> filteredNames = names.stream()
.filter(name -> name.compareTo("m") < 0)
.collect(Collectors.toList());
// Output:
// The filtered list will be: ['Alice', 'Bob']
In this code, we use a stream to filter the list of names. The filter method uses compareTo to keep only those names that come before ‘m’ in lexicographical order.
Further Resources for Java .compareTo() Method
Want to dive deeper into Java’s compareTo method and related topics? Here are some resources that can help:
- Java String Tutorial: Getting Started – Explore string concatenation and formatting in Java.
Handling Escape Characters in Java – Master using escape characters to include special characters in string literals.
CharAt in Java – Explore the charAt() method in Java for retrieving characters from a string by index.
Oracle’s official Java documentation – A comprehensive guide on ordering objects in Java, including the use of compareTo.
Baeldung’s guide on compareTo covers how to use the Comparable interface and compareTo method in Java.
GeeksforGeeks’ on Java String compareTo() explains the compareTo method with examples.
Wrapping Up: Java .compareTo() Method
In this comprehensive guide, we’ve delved into the details of the compareTo method in Java, a powerful tool for comparing strings and objects. We’ve explored the basic use, advanced techniques, and alternative approaches to string comparison in Java.
We started with the basics of compareTo, learning how to use it to compare strings in a simple, straightforward manner. We then moved onto more complex scenarios, such as comparing custom objects and handling null values. We also discussed common issues you might encounter when using compareTo, such as case sensitivity and special characters, and provided solutions for each.
We didn’t stop there. We also explored alternative approaches to comparing strings in Java, such as using equals() and the ==
operator. Each method has its pros and cons, and the right choice depends on your specific use case.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
compareTo | Can compare lexicographical order, works with custom objects | Case sensitive, doesn’t handle null values |
equals() | Checks if two strings are identical | Doesn’t compare lexicographical order |
== operator | Checks if two string references point to the same object | Doesn’t check string content |
Whether you’re a beginner just starting out with compareTo or an experienced developer looking to brush up your skills, we hope this guide has provided you with a deeper understanding of how to use compareTo in Java effectively.
Mastering the compareTo method is a valuable skill in Java programming, allowing you to compare strings and objects efficiently. Now, you’re well-equipped to navigate the world of string comparison in Java. Happy coding!