Java Escape Characters: Your Ultimate Guide
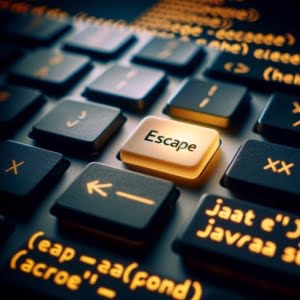
Are you finding it challenging to use escape characters in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling escape characters in Java, but we’re here to help.
Think of Java’s escape characters as a secret code – allowing us to represent special characters in our strings, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using escape characters in Java, from their basic usage, to more advanced techniques, as well as alternative approaches.
Let’s get started and start mastering Java escape characters!
TL;DR: How Do I Use Escape Characters in Java?
To use escape characters in Java, you use a backslash (\) followed by the character you want to escape. For instance, to include a quotation mark within a string, you would write it as \”, such as:
String example = "This is a \"quote\" inside a string.";
.
Here’s a simple example:
String example = "This is a \"quote\" inside a string.";
System.out.println(example);
// Output:
// This is a "quote" inside a string.
In this example, we’ve used the escape character (\) to include quotation marks within a string. The backslash tells Java to treat the following character (in this case, the quotation mark) as a literal character, not a special one.
But Java’s escape characters capabilities go far beyond this. Continue reading for more detailed examples and advanced escape characters techniques.
Table of Contents
Getting Started with Escape Characters in Java
Escape characters in Java are special characters used to perform specific tasks. They’re denoted by a backslash (\) followed by the character you want to escape. Let’s dive into the basic usage of escape characters in Java.
Java Escape Characters: The Basics
Consider a scenario where you want to include a quotation mark in your string. In Java, strings are enclosed in quotation marks, so including a quotation mark in your string could confuse the compiler. Here’s where escape characters come to the rescue.
String quote = "Mark Twain once said, \"The secret of getting ahead is getting started.\"";
System.out.println(quote);
// Output:
// Mark Twain once said, "The secret of getting ahead is getting started."
In the above code snippet, we’ve used escape characters (\”) to include quotation marks in the string. This tells Java to treat the following character as a literal character, not a special one.
Advantages of Using Escape Characters
Escape characters give us the ability to include special characters in our strings, which can be extremely useful in various scenarios. They help us to maintain the integrity of our data and prevent syntax errors.
Potential Pitfalls
While escape characters are handy, they can also lead to confusion if not used correctly. For example, forgetting to use an escape character when needed or using an incorrect escape character can lead to syntax errors or unexpected output. Therefore, it’s crucial to understand how and when to use them.
Advanced Escape Characters in Java
As you become more comfortable with Java’s escape characters, you can start to explore their more advanced uses. Let’s discuss two important ones: escaping Unicode characters and creating escape sequences.
Escaping Unicode Characters
Java supports Unicode, and you can use escape characters to represent Unicode characters in your string. The syntax for this is \\u
followed by the four-digit Unicode value of the character.
String unicodeString = "The unicode for Omega is: \\u03A9";
System.out.println(unicodeString);
// Output:
// The unicode for Omega is: Ω
In the above example, we’ve used the escape sequence \\u03A9
to represent the Greek letter Omega (Ω) in our string.
Creating Escape Sequences
Escape sequences are combinations of escape characters and other characters that represent special characters. For example, \n
represents a newline, and \t
represents a tab.
String escapeSequences = "This is line 1\nThis is line 2\n\tThis is indented text.";
System.out.println(escapeSequences);
// Output:
// This is line 1
// This is line 2
// This is indented text.
In this code snippet, we’ve used the escape sequences \n
and \t
to create a new line and a tab space, respectively.
Using escape characters in these advanced ways allows for more complex string manipulation and formatting in Java.
Exploring Alternatives: Beyond Java Escape Characters
While Java escape characters are a powerful tool, there are alternative methods to represent special characters in Java. Let’s explore two of these: using character codes and leveraging third-party libraries.
Using Character Codes
Java allows you to use character codes to represent special characters. This can be particularly useful when working with non-standard characters or symbols.
char quoteChar = 34; // ASCII value of "
String quote = quoteChar + "To be or not to be, that is the question." + quoteChar;
System.out.println(quote);
// Output:
// "To be or not to be, that is the question."
In this example, we’ve used the ASCII value for the quotation mark (34) to include quotes in our string. This method can be useful, but it requires knowledge of the ASCII or Unicode tables, which can be a drawback for some.
Leveraging Third-Party Libraries
Third-party libraries, like Apache Commons Lang, provide utilities to handle escape characters. These libraries can offer more functionality and ease of use compared to the native Java approach.
// Assuming Apache Commons Lang is added to the project
import org.apache.commons.lang3.StringEscapeUtils;
String quote = StringEscapeUtils.escapeJava("To be or not to be, that is the question.");
System.out.println(quote);
// Output:
// \"To be or not to be, that is the question.\"
In the above example, we’ve used the escapeJava
method from the StringEscapeUtils
class to handle escape characters. While this method offers convenience, it does add an external dependency to your project, which might not be ideal in all situations.
Choosing the right method to handle special characters in Java depends on your specific use case, the complexity of the strings you’re working with, and whether you are willing to add external dependencies to your project.
Troubleshooting Java Escape Characters
While Java escape characters can be invaluable, they can also lead to common issues, such as syntax errors or unexpected output. Let’s discuss some of these problems and their solutions.
Syntax Errors
One of the most common problems when using escape characters is syntax errors. These usually occur when the escape character is not used correctly.
String brokenString = "This is a broken string;
System.out.println(brokenString);
// Output:
// Error: unclosed string literal
In the above example, we’ve forgotten to close the string with a quotation mark, leading to a syntax error. The solution is to ensure that all strings are properly closed and that escape characters are used when necessary.
Unexpected Output
Another common issue is unexpected output. This typically happens when an escape character is used incorrectly or not used when needed.
String unexpectedOutput = "This is a \\"quote\\".";
System.out.println(unexpectedOutput);
// Output:
// This is a "quote".
In this example, we’ve used the escape character \\"
to include a quote in our string. However, if we intended for the output to be This is a \"quote\".
, we would need to escape the backslashes as well, like so: This is a \\\"quote\\\".
.
Remember, practice makes perfect. The more you work with Java escape characters, the more familiar you’ll become with their nuances and potential pitfalls.
Understanding Java Strings and Escape Characters
To fully grasp the concept of escape characters, we first need to understand Java’s string data type and how escape characters function within it.
Java Strings: A Brief Overview
In Java, a string is a sequence of characters. It’s not a primitive data type, but rather a class in the Java standard library. Strings are immutable, meaning once created, they cannot be changed. Any operation that appears to modify a string instead creates a new string.
String str = "Hello, World!";
str = str.replace("World", "Java");
System.out.println(str);
// Output:
// Hello, Java!
In this example, we didn’t actually change the original string. Instead, we created a new string with the replaced text.
The Role of Escape Characters in Strings
Escape characters play a crucial role in Java strings. They allow us to include special characters in our strings and help us to format our strings accurately.
For example, the escape sequence \n
creates a new line, and \t
creates a tab space. This helps in formatting the output of the string.
String formattedString = "Hello,\n\tWorld!";
System.out.println(formattedString);
// Output:
// Hello,
// World!
In this example, we’ve used the escape sequences \n
and \t
to format our string with a new line and a tab space. As you can see, escape characters are a powerful tool for representing special characters and formatting strings in Java.
Exploring Escape Characters: Beyond Strings
Escape characters in Java aren’t just limited to strings. They play a significant role in other aspects of Java programming, such as file handling and user input. Let’s explore these areas and see how escape characters come into play.
File Handling and Escape Characters
When working with file paths in Java, escape characters become vital. File paths often contain backslashes (\), which need to be escaped in Java strings.
String filePath = "C:\\Users\\User\\Documents\\file.txt";
File file = new File(filePath);
// The file path is correctly recognized
In this example, we’ve used escape characters to correctly represent the file path in our string. Without them, Java would interpret the backslashes as escape characters, leading to an incorrect file path.
User Input and Escape Characters
Escape characters are also important when dealing with user input. For instance, if a user enters a string that includes a quotation mark, we need to escape that character to prevent syntax errors.
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a string:");
String userInput = scanner.nextLine();
userInput = userInput.replace("\"", "\\\"");
// The user input is correctly handled, even if it includes quotation marks
In this example, we’ve replaced any quotation marks in the user input with their escaped counterpart, ensuring that the input is correctly handled.
Further Resources for Java Escape Characters
If you’re interested in delving deeper into the world of Java escape characters, here are some resources that can help:
- IOFlood’s Java String Article – Learn about string indexing and substring extraction in Java.
CompareTo in Java – Learn about the compareTo() method in Java for comparing strings lexicographically.
Java Format Basics – Learn about formatting patterns and placeholders for customizing output in Java.
Oracle’s Java Documentation – A comprehensive resource for understanding Java’s data types, including strings.
Escaping Characters in Java by CodeGym provides explanations and examples on how to use escape characters in Java.
GeeksforGeeks’ Java String tutorial provides a deep dive into Java strings, including the use of escape characters.
Wrapping Up: Java Escape Characters
In this comprehensive guide, we’ve delved deep into the world of Java escape characters. We’ve explored their usage, from basic to advanced, and even looked at alternative approaches for handling special characters in Java.
We began with the basics, discussing how to use escape characters in Java and their role in string manipulation. We then moved onto more advanced techniques, such as escaping Unicode characters and creating escape sequences. We also explored alternative methods to represent special characters, including using character codes and third-party libraries.
Throughout our journey, we addressed common issues that you might encounter when using escape characters, such as syntax errors and unexpected output, and provided solutions to help you overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Java Escape Characters | Versatile, no external dependencies | Requires understanding of escape sequences |
Character Codes | Useful for non-standard characters | Requires knowledge of ASCII or Unicode tables |
Third-Party Libraries | Offers more functionality, ease of use | Adds external dependencies |
Whether you’re a beginner just starting out with Java, or an experienced developer looking to enhance your skills, we hope this guide has provided you with a deeper understanding of Java escape characters and their usage.
Understanding and using Java escape characters effectively is a valuable skill in your Java programming toolkit. Now, you’re well-equipped to handle special characters in Java with ease. Happy coding!