Understanding Package.json Files | NPM Setup Guide
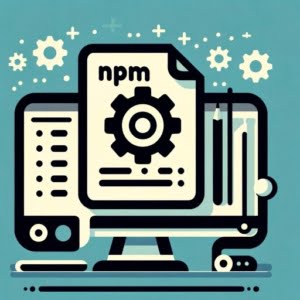
Embarking on a Node.js project without understanding the package.json file is like setting sail without a map. This essential file acts as the heart of your project, guiding npm on how to handle your project’s dependencies and scripts. Today, our goal is to help you learn npm package.json configuration easily!
When administrating Node.js servers for IOFLOOD, we often go over the npm package.json configuration to ensure optimized server performance and resource management. To help our customers with their bare metal cloud servers, and other developers, we have gathered our tips and tricks into this step-by-step guide.
This guide is your comprehensive walkthrough on npm’s package.json. From its basic structure to advanced configurations, we’ll cover everything you need to know to make the most out of this powerful tool.
Let’s dive in and unlock the full potential of package.json in your Node.js projects.
TL;DR: What is npm’s package.json?
The
package.json
file in a Node.js project specifies the project’s metadata, dependencies, scripts, and other functional parts required before publishing.
Here’s a basic example:
{
"name": "my-project",
"version": "1.0.0",
"description": "A Node.js project",
"scripts": {
"start": "node app.js"
},
"dependencies": {
"express": "^4.17.1"
}
}
# Output:
# This configuration tells npm to start your app using 'node app.js' and includes 'express' as a project dependency.
In this example, we’ve created a simple package.json
file for a hypothetical Node.js project named ‘my-project’. It defines a single script to start the application and includes Express, a popular web framework, as a dependency. This file acts as a blueprint for npm, detailing how to assemble and run your project.
Curious about how to leverage
package.json
for more complex projects? Keep reading for a deeper dive into its capabilities and best practices for Node.js development.
Table of Contents
Understanding package.json Basics
When you’re starting with Node.js, one of the first files you’ll encounter in any project is package.json
. This file is more than just a piece of JSON; it’s the backbone of your project’s structure, telling npm (Node Package Manager) everything it needs to know about your project. Let’s break down some of the essential fields you’ll find in a package.json
file:
name
: The unique identifier for your project.version
: Follows semantic versioning (semver), indicating the current version of your project.scripts
: Defines scripts to run various tasks, such as starting your server or running tests.dependencies
: Lists other npm packages your project needs to run.
Creating Your First package.json
Creating a package.json
file is straightforward. You can manually create one or use the npm init
command to generate it interactively. Let’s explore the latter:
npm init -y
# Output:
# This command creates a basic package.json file with default values for your project.
This command generates a package.json
with default values for fields like name
, version
, and description
. It’s a quick way to get started, but you’ll likely want to customize it to fit your project’s needs.
Modifying package.json
Once you have your package.json
, modifying it is as simple as opening the file in your favorite text editor and making changes. For example, adding a start script to run your application might look like this:
{
"scripts": {
"start": "node server.js"
}
}
# Output:
# This addition tells npm that running `npm start` will execute `node server.js`, starting your application.
This example shows how to add a start
script, which is crucial for defining how to kick off your application. By specifying node server.js
, you’re telling npm to start your Node.js server when you run npm start
from the command line. It’s a simple yet powerful way to control your project’s behavior through npm.
Advanced package.json Features
As you dive deeper into Node.js development, understanding the nuanced capabilities of package.json
becomes crucial. This file doesn’t just hold your project’s metadata and dependencies; it’s a powerful tool for fine-tuning your development workflow. Let’s explore some of the advanced fields and functionalities that can take your project management to the next level.
Leveraging devDependencies
While dependencies
are essential for your application to run, devDependencies
play a crucial role during the development phase. These include packages needed for testing, building, and local development but are not required in production.
{
"devDependencies": {
"jest": "^26.6.0",
"eslint": "^7.12.1"
}
}
# Output:
# This configuration adds Jest and ESLint as development dependencies, indicating they are only needed during development, not in production.
By specifying packages like Jest and ESLint in devDependencies
, you ensure that your production environment remains lean and focused on what’s necessary for running the application, thereby optimizing performance.
Script Customization for Efficiency
package.json
allows you to define custom scripts for various development tasks. This not only streamlines your workflow but also ensures consistency across development environments.
{
"scripts": {
"test": "jest",
"lint": "eslint ."
}
}
# Output:
# Running `npm run test` will execute Jest, and `npm run lint` will run ESLint on your project, demonstrating the power of script customization in package.json.
Custom scripts like these allow you to automate repetitive tasks with a single command, enhancing productivity and ensuring that your project adheres to best practices.
Mastering peerDependencies
peerDependencies
are particularly useful when developing libraries or plugins that work alongside other packages. They specify versions of packages that your project is compatible with, without forcing those packages to be installed.
{
"peerDependencies": {
"react": "^16.8.0"
}
}
# Output:
# This configuration indicates that your project is compatible with React version 16.8.0 and above, but does not require React to be installed as a direct dependency.
Including peerDependencies
in your package.json
ensures that your project can seamlessly integrate with other software, maintaining compatibility without dictating the dependencies of other projects.
Through the strategic use of devDependencies
, custom scripts, and peerDependencies
, your package.json
becomes a central hub for managing your project’s lifecycle, dependencies, and compatibility. As you master these advanced features, you’ll unlock more efficient and effective project management strategies, tailored to the complex needs of intermediate-level Node.js development.
More Techniques with package.json
As you evolve from an intermediate to an expert Node.js developer, your projects grow in complexity. This growth demands a more sophisticated approach to managing your package.json
files, especially when working with large codebases or multiple projects. Let’s delve into some advanced techniques and tools that can significantly enhance your package management strategy.
NPM Workspaces: Managing Monorepos
One of the challenges of working with monorepos is managing dependencies across multiple packages within the same repository. NPM introduced workspaces to address this challenge, enabling you to install dependencies from a single place and link them across your workspace.
{
"workspaces": [
"packages/*"
]
}
# Output:
# This configuration tells npm to treat each directory inside `packages/` as a separate package, sharing and linking dependencies where applicable.
The workspaces
feature not only simplifies dependency management but also facilitates a more organized and efficient development workflow. By leveraging npm workspaces, you can ensure that all your packages stay in sync, reducing the overhead of managing multiple package.json
files.
Auto-generating package.json with npm init
For new projects, npm init
provides a quick way to generate a package.json
file. Taking this a step further, npm allows for customization and automation of this process with npm init
, which can scaffold a project based on predefined templates.
npm init express-generator
# Output:
# This command uses the express-generator package to create a new Express app, automatically generating a tailored package.json file.
This approach not only speeds up the project setup process but also ensures that your package.json
adheres to best practices right from the start. It’s an invaluable tool for experts who frequently initiate new projects and seek efficiency and consistency.
NPM vs. Yarn: A Comparative Look
While npm is the default package manager for Node.js, Yarn emerged as a popular alternative, offering some differences in how package.json
is handled. For instance, Yarn introduced yarn.lock
to ensure that the same versions of packages are installed across different environments, similar to package-lock.json
in npm.
Yarn’s workspaces feature also provides a slightly different approach to managing monorepos, emphasizing performance and lockfile management. Choosing between npm and Yarn often comes down to personal preference and specific project requirements, but understanding the nuances of each can help you make an informed decision.
Mastering these advanced techniques and tools not only enhances your package.json
management skills but also prepares you for the complexities of modern Node.js development. By exploring npm workspaces, utilizing npm init
for automation, and comparing npm with alternatives like Yarn, you can adopt a more strategic approach to package management, tailored to the needs of expert-level projects.
Solving Common package.json Issues
While package.json
is a powerful tool for managing your Node.js projects, it’s not without its challenges. Dependency conflicts, versioning issues, and script errors are common hurdles that developers face. Let’s explore how to troubleshoot these issues effectively and maintain a clean, efficient package.json
file.
Resolving Dependency Conflicts
Dependency conflicts occur when different parts of your project require incompatible versions of the same package. This can lead to unexpected behavior or build failures. A common solution is to use the npm ls
command to identify dependencies and resolve conflicts manually.
npm ls conflicting-package
# Output:
# This command lists all installed versions of `conflicting-package`, helping you identify where the conflict arises.
Understanding the output of npm ls
is crucial for resolving conflicts. It shows the dependency tree of the conflicting package, allowing you to trace back to the source of the conflict and make informed decisions on how to resolve it.
Tackling Versioning Problems
Semantic versioning (semver) is a blessing and a curse. While it helps manage dependencies efficiently, it can also lead to versioning problems if not used carefully. The npm outdated
command can identify outdated dependencies, and updating the package.json
file with compatible versions can resolve these issues.
npm outdated
# Output:
# This command displays a list of outdated packages, their current version, and the latest available version.
The output of npm outdated
serves as a guide for updating your dependencies. By carefully selecting compatible versions and updating your package.json
accordingly, you can avoid versioning problems that might break your application.
Debugging Script Errors
Script errors in package.json
can halt your development workflow. These errors often result from typos or incorrect script paths. Running scripts with the npm run
command provides detailed error logs that can help identify the issue.
npm run broken-script
# Output:
# This command executes `broken-script`, and if it fails, npm provides a detailed error log.
The error log from npm run
is invaluable for debugging script errors. It pinpoints the source of the error, whether it’s a missing file, a typo, or a misconfiguration, allowing you to quickly address the issue and get back on track.
Maintaining a clean and efficient package.json
is crucial for the success of your Node.js projects. By understanding how to troubleshoot common issues like dependency conflicts, versioning problems, and script errors, you can ensure that your project management remains smooth and your development workflow uninterrupted. These best practices and solutions not only help you resolve immediate problems but also contribute to the overall health and maintainability of your project.
NPM and package.json Fundamentals
To truly master npm package.json
, it’s essential to grasp the foundational concepts that underpin npm (Node Package Manager) and the pivotal role of package.json
in Node.js and JavaScript projects. Npm is more than just a tool for installing packages; it’s a comprehensive package management system designed to facilitate package installation, version management, and dependency control.
The Heart of Node.js Projects
package.json
serves as the blueprint for your Node.js application or package. It meticulously catalogs project metadata, dependencies, scripts, and more, enabling npm to manage your project’s needs efficiently.
{
"name": "hello-world",
"version": "0.1.0",
"description": "A sample Node.js app",
"main": "index.js",
"scripts": {
"start": "node index.js"
}
}
# Output:
# This `package.json` example outlines a basic Node.js project setup, including a start script to run the application.
This simple package.json
example highlights how the file specifies the project’s entry point (main
), a brief description, and a script to start the application. Understanding this structure is crucial for effective project management and development within the npm ecosystem.
Semantic Versioning (SemVer)
Semantic versioning, or SemVer, is a critical component of package management. It’s a versioning system that ensures compatibility and stability across package releases. SemVer uses a three-part version number: major, minor, and patch (e.g., 1.4.2), where each segment signifies the level of changes made.
npm update my-package
# Output:
# Assuming my-package was at version 1.4.1, this command updates it to the latest compatible version, following SemVer rules.
In this example, running npm update my-package
would update my-package
to the latest version that is compatible with the current major version, adhering to SemVer guidelines. This ensures that updates do not break existing functionality, highlighting the importance of understanding versioning in package management.
Grasping these fundamental concepts of npm and package.json
is vital for any developer working in the Node.js and JavaScript ecosystems. It lays the groundwork for efficient project management, from initial setup to ongoing maintenance and updates. By mastering these basics, you’re well on your way to becoming proficient with npm package.json
, enabling you to leverage the full power of npm in your projects.
Best Practices for package.json
The package.json
file is not just a cornerstone for managing project dependencies in Node.js; it plays a pivotal role in streamlining advanced development practices such as Continuous Integration/Continuous Deployment (CI/CD) pipelines and automated testing. Understanding how to leverage package.json
in these contexts can significantly enhance your development workflow and project quality.
CI/CD Pipelines and package.json
Continuous Integration and Continuous Deployment pipelines benefit greatly from a well-configured package.json
. For instance, specifying scripts for test and build processes in package.json
can automate these steps in your CI/CD pipeline.
{
"scripts": {
"test": "jest",
"build": "webpack"
}
}
# Output:
# This configuration enables automated testing with Jest and build processes with Webpack in CI/CD pipelines.
In this example, the test
script runs your tests using Jest, and the build
script compiles your project with Webpack. Integrating these scripts into your CI/CD pipeline ensures that every commit is tested and built automatically, promoting a more reliable and efficient development process.
Automated Testing with package.json
Automated testing is another area where package.json
proves invaluable. By defining test scripts and specifying test-related packages in devDependencies
, you can ensure that your testing environment is consistent and easily reproducible.
{
"scripts": {
"test": "mocha"
},
"devDependencies": {
"mocha": "^8.2.1",
"chai": "^4.2.0"
}
}
# Output:
# Running `npm test` executes the Mocha test suite, utilizing Chai for assertions, streamlining the testing process.
This setup facilitates running your test suite with a single command (npm test
), making it straightforward to integrate automated testing into your development and CI/CD workflows. It exemplifies the role of package.json
in establishing a robust testing strategy.
Further Resources for Mastering package.json
To deepen your understanding of package.json
and its applications in modern development practices, here are three invaluable resources:
- Offical npm Documentation – Comprehensive insights into
package.json
, its fields, and best practices. Node.js Guides – Practical advice on using
package.json
in your Node.js projects, from setup to advanced configurations.Understanding npm – An in-depth exploration of npm as a tool and its ecosystem, meant to help developers make the most of
package.json
.
Leveraging package.json
in advanced development practices not only streamlines your workflow but also enhances the quality and reliability of your projects. By exploring the resources above, you can further refine your skills and understanding, positioning yourself as an expert in Node.js development.
Recap: npm package.json Guide
In this comprehensive guide, we’ve traversed the landscape of npm’s package.json, uncovering its pivotal role in Node.js project management. From its basic structure and purpose to advanced techniques for optimizing your development workflow, package.json stands as the cornerstone of efficient project management.
We began with the essentials, understanding the structure of package.json and its significance in defining project metadata, dependencies, and scripts. We then moved on to explore how to leverage this file for more complex project requirements, including managing development dependencies, customizing scripts, and utilizing peerDependencies for better version control.
Our journey took us further into the realms of npm workspaces for managing monorepos and the nuances of generating package.json files through npm init. We also compared npm with other package managers like Yarn, shedding light on the diverse ecosystem of package management.
Feature | npm | Yarn |
---|---|---|
Dependency Management | Comprehensive | Efficient |
Workspaces | Supported | Enhanced support |
Performance | Fast | Faster with caching |
Custom Scripts | Extensive | Extensive |
Whether you’re just starting out with npm and Node.js or looking to deepen your understanding of package.json, we hope this guide has provided you with valuable insights and tools to enhance your projects. package.json is more than just a file; it’s a blueprint for your project’s success, guiding npm in managing dependencies, scripts, and more.
Understanding and mastering package.json is crucial for any Node.js developer aiming for efficient project management and development. We encourage you to experiment with the advanced features and best practices discussed, as they hold the key to unlocking the full potential of your Node.js projects. Happy coding!