JList Usage: A Detailed Guide for Java Swing
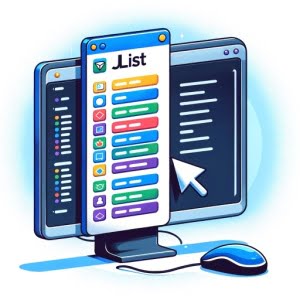
Are you finding it challenging to work with JList in Java Swing? You’re not alone. Many developers find the task of managing a collection of items for user selection a bit daunting, but there’s a tool that can make this process a breeze.
Think of JList as a versatile bookshelf – it can neatly display a collection of items for user selection, making it an essential tool for various tasks in Java Swing applications.
This guide will walk you through the ins and outs of using JList in your Java Swing applications. We’ll explore JList’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering JList!
TL;DR: How Do I Use JList in Java Swing?
To use
JList
in Java Swing, you create an instance of JList, with the syntaxJList<String> list = new JList<>(data);
, add items to it, and add it to a container, such asJScrollPane
. This can be done using a few lines of code.
Here’s a basic example:
String[] data = {"Item 1", "Item 2", "Item 3"};
JList<String> list = new JList<>(data);
JScrollPane scrollPane = new JScrollPane(list);
frame.add(scrollPane);
// Output:
// A window with a list containing 'Item 1', 'Item 2', and 'Item 3'
In this example, we create a string array data
with three items. We then create a JList
instance list
and pass data
to it. To make the list scrollable, we wrap it in a JScrollPane
and add it to the frame. The result is a window displaying a scrollable list of items.
This is a basic way to use JList in Java Swing, but there’s much more to learn about creating and manipulating lists in Java Swing. Continue reading for more detailed instructions and advanced usage scenarios.
Table of Contents
- JList Basics: Creating and Adding Items
- Enhancing JList: Advanced Features and Customization
- Exploring Alternatives: JComboBox and JTable
- Navigating JList Pitfalls: Troubleshooting and Best Practices
- Understanding the Basics: Java Swing, GUI Components, and Event Handling
- JList Applications: Beyond the Basics
- Wrapping Up: JList
JList Basics: Creating and Adding Items
In Java Swing, creating a JList and adding items to it is a straightforward process. Here’s a step-by-step guide to help you get started.
First, you need to create an instance of JList. This can be done by using the JList constructor, which takes an array or vector of objects. For example, let’s create a JList of Strings:
String[] data = {"Item 1", "Item 2", "Item 3"};
JList<String> list = new JList<>(data);
In this code block, we first declare a string array data
with three items. We then create a JList
instance list
and pass data
to it.
Next, to make our list scrollable and visible, we need to add it to a container, such as a JScrollPane, and then add that to a JFrame:
JScrollPane scrollPane = new JScrollPane(list);
frame.add(scrollPane);
// Output:
// A window with a list containing 'Item 1', 'Item 2', and 'Item 3'
In this step, we create a JScrollPane
and pass our list
to it. This makes our list scrollable. We then add scrollPane
to our frame
, making the list visible in our window.
While the process of creating a JList and adding items to it is simple, there are a few things to keep in mind. For instance, remember that the JList constructor doesn’t create a copy of the array you pass to it. This means that any changes you make to the original array after creating the JList won’t be reflected in the list.
Stay tuned for more advanced usage scenarios and learn how to make the most out of JList in Java Swing.
Enhancing JList: Advanced Features and Customization
As you get more comfortable with JList, you might want to explore its more complex features, such as adding a ListSelectionListener, customizing the list’s appearance, and using a custom ListModel.
Adding a ListSelectionListener
A ListSelectionListener allows you to respond to user selection events. Here’s an example of how to add a ListSelectionListener to a JList:
list.addListSelectionListener(new ListSelectionListener() {
public void valueChanged(ListSelectionEvent e) {
if (!e.getValueIsAdjusting()) {
System.out.println(list.getSelectedValue());
}
}
});
// Output:
// Prints the selected item to the console when a selection is made
In this code block, we add a ListSelectionListener
to our list
. When the user makes a selection, the valueChanged
method is called, and the selected item is printed to the console.
Customizing List Appearance
You can also customize the appearance of your JList. For instance, you can change the selection mode to allow for multiple interval selections:
list.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
// Output:
// The list now allows multiple items to be selected
In this example, we change the selection mode of our list
to MULTIPLE_INTERVAL_SELECTION
, which allows multiple items to be selected.
Using a Custom ListModel
A ListModel provides the data for the JList. You can create a custom ListModel to control how data is added, removed, or changed. Here’s an example of a custom ListModel:
DefaultListModel<String> model = new DefaultListModel<>();
model.addElement("Item 1");
model.addElement("Item 2");
model.addElement("Item 3");
JList<String> list = new JList<>(model);
// Output:
// A list with 'Item 1', 'Item 2', and 'Item 3'
In this code block, we create a DefaultListModel
and add elements to it. We then create a JList
and pass our model
to it.
These advanced features can significantly enhance the functionality and appearance of your JList. Stay tuned for alternative approaches and troubleshooting tips.
Exploring Alternatives: JComboBox and JTable
While JList is a powerful tool for displaying a collection of items in Java Swing, it’s not the only option. Depending on your specific needs, you might find that a JComboBox or a JTable is a better fit.
Using JComboBox for Drop-Down Lists
A JComboBox is a component that combines a button or editable field and a drop-down list. It’s an excellent choice when you want to display a list of items in a compact format.
String[] data = {"Item 1", "Item 2", "Item 3"};
JComboBox<String> comboBox = new JComboBox<>(data);
frame.add(comboBox);
// Output:
// A window with a drop-down list containing 'Item 1', 'Item 2', and 'Item 3'
In this example, we create a JComboBox and add it to our frame. The JComboBox displays a drop-down list of items when clicked.
Using JTable for Tabular Display
A JTable is a user-interface component that displays data in a two-dimensional table. It’s a great option when you need to display complex data structures.
String[] columnNames = {"Column 1", "Column 2", "Column 3"};
Object[][] data = { {"Item 1", "Item 2", "Item 3"}, {"Item 4", "Item 5", "Item 6"} };
JTable table = new JTable(data, columnNames);
frame.add(new JScrollPane(table));
// Output:
// A window with a table containing six items distributed across three columns
In this code block, we create a JTable with two rows and three columns, and add it to our frame. The JTable displays a table of items, organized into rows and columns.
Both JComboBox and JTable offer unique benefits, but they also come with their own drawbacks. A JComboBox can be less intuitive for users who are not familiar with drop-down lists, and a JTable can be overkill for simple lists of items. It’s important to consider these factors when deciding which component to use.
Like any tool, JList comes with its own set of challenges and considerations. Let’s explore some common issues you might encounter while using JList and discuss their solutions.
Issue: JList Not Displaying Items
One common issue is that the JList is not displaying any items, even though you’ve added items to it. This could be due to forgetting to add the JList to a JScrollPane or a JFrame.
String[] data = {"Item 1", "Item 2", "Item 3"};
JList<String> list = new JList<>(data);
// Output:
// Nothing is displayed
In this example, we create a JList but forget to add it to a JScrollPane or a JFrame. As a result, nothing is displayed.
The solution is to add the JList to a JScrollPane and then add that to a JFrame:
JScrollPane scrollPane = new JScrollPane(list);
frame.add(scrollPane);
// Output:
// A window with a list containing 'Item 1', 'Item 2', and 'Item 3'
Issue: JList Not Updating When Data Changes
Another common issue is that the JList is not updating when the data changes. This is because JList does not create a copy of the array you pass to it, so changes to the original array won’t be reflected in the JList.
String[] data = {"Item 1", "Item 2", "Item 3"};
JList<String> list = new JList<>(data);
data[0] = "Item 4";
// Output:
// The list still contains 'Item 1', 'Item 2', and 'Item 3'
In this code block, we change the first item in our data
array after creating the JList. However, the change is not reflected in the list.
The solution is to use a ListModel and update the data through the model:
DefaultListModel<String> model = new DefaultListModel<>();
model.addElement("Item 1");
model.addElement("Item 2");
model.addElement("Item 3");
JList<String> list = new JList<>(model);
model.set(0, "Item 4");
// Output:
// The list now contains 'Item 4', 'Item 2', and 'Item 3'
In this example, we create a DefaultListModel, add elements to it, and then create a JList with this model. When we change an element in the model, the change is reflected in the list.
Best Practices and Optimization
To optimize your use of JList, here are a few best practices:
- Always add your JList to a JScrollPane to ensure that all items can be viewed, even if there are more items than can fit in the visible area.
- Update your data through the ListModel to ensure that changes are reflected in the JList.
- Use a custom ListModel if you need more control over how data is added, removed, or changed.
By understanding these common issues and best practices, you can avoid pitfalls and make the most out of JList in Java Swing.
Understanding the Basics: Java Swing, GUI Components, and Event Handling
Before we dive deeper into JList, it’s important to understand some fundamental concepts: Java Swing, GUI components, and event handling in Java Swing.
Java Swing: A Quick Overview
Java Swing is a set of Java libraries used for creating graphical user interfaces (GUIs). It provides a rich set of widgets and packages to create sophisticated desktop applications.
import javax.swing.*;
public class HelloWorldSwing {
private static void createAndShowGUI() {
JFrame frame = new JFrame("HelloWorldSwing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JLabel label = new JLabel("Hello World");
frame.getContentPane().add(label);
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
// Output:
// A window titled 'HelloWorldSwing' with a label that reads 'Hello World'
In this example, we create a basic Swing application that displays a window with the text ‘Hello World’.
GUI Components: The Building Blocks
GUI components are the building blocks of any GUI application. They include things like buttons, checkboxes, and, of course, lists. These components are instances of classes like JButton, JCheckbox, and JList.
JButton button = new JButton("Click me");
JCheckBox checkbox = new JCheckBox("Check me");
JList<String> list = new JList<>(new String[]{"Item 1", "Item 2", "Item 3"});
// Output:
// Creates a button, a checkbox, and a list
In this code block, we create a JButton, a JCheckBox, and a JList. These are some of the basic components you can use in a Swing application.
Event Handling in Java Swing
Event handling is a fundamental aspect of interactive applications. In Java Swing, you handle events by adding event listeners to your components. These listeners respond to user interactions, such as mouse clicks or key presses.
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked");
}
});
// Output:
// Prints 'Button clicked' to the console when the button is clicked
In this example, we add an ActionListener to our button. When the button is clicked, the actionPerformed
method is called, and ‘Button clicked’ is printed to the console.
Understanding these fundamental concepts is crucial for mastering JList and other advanced features in Java Swing. Stay tuned for more in-depth discussion about the application of JList in larger projects.
JList Applications: Beyond the Basics
As you become more proficient with JList, you’ll find it’s not just a tool for creating simple lists. It’s a versatile component that can significantly enhance the functionality of your Java Swing applications.
JList in Larger Projects
In larger projects, JList can be used to create complex user interfaces, such as file explorers, email clients, or chat applications. For instance, you might use a JList to display a list of files in a directory, a list of emails in an inbox, or a list of messages in a chat.
DefaultListModel<String> model = new DefaultListModel<>();
JList<String> list = new JList<>(model);
// Add items to the model dynamically
for (int i = 0; i < 100; i++) {
model.addElement("Item " + i);
}
// Output:
// A list with 100 items
In this example, we create a JList with a DefaultListModel and add 100 items to it dynamically. This could represent a list of files, emails, or messages in a larger application.
Complementary Components and Concepts
JList often works in tandem with other components and concepts. For instance, you might use a JScrollPane to make your JList scrollable, a ListModel to manage your list data, or a ListSelectionListener to respond to user selection events.
Understanding these related components and concepts can help you make the most out of JList in your applications.
Further Resources for JList Mastery
If you are interested in learning more about other Java Classes, we have written a Complete Guide on Java Classes. To read it, Click Here!
To continue your journey towards JList mastery, consider exploring these resources:
- Understanding JFrame Basics – Explore JFrame in Java for building windows-based applications with rich user interfaces.
Mastering Time Duration Handling in Java – Explore Java’s Duration class for time-based calculations and operations.
Java Swing Tutorial – Oracle’s official tutorial on Java Swing, including a section on JList.
JList Official API Documentation provides detailed information about its methods and usage.
Java Swing (GUI) Programming: From Beginner to Expert – A course on Udemy that covers Java Swing, and JList, in depth.
With these resources and the knowledge you’ve gained from this guide, you’re well on your way to mastering JList in Java Swing.
Wrapping Up: JList
In this comprehensive guide, we’ve delved into the world of JList, a versatile tool for managing collections of items in Java Swing applications. We’ve covered the basics of creating a JList, adding items to it, and making it visible in a window. We’ve also explored advanced features, such as adding a ListSelectionListener, customizing the list’s appearance, and using a custom ListModel.
We began with the basics, offering a step-by-step guide on how to create a JList and add items to it. We then delved into more advanced topics, exploring how to add a ListSelectionListener, customize the list’s appearance, and use a custom ListModel. Along the way, we tackled common issues such as JList not displaying items or not updating when data changes, providing solutions and best practices to navigate these challenges.
We also explored alternative approaches to managing collections of items in Java Swing, such as using a JComboBox for a drop-down list or a JTable for a tabular display. Here’s a quick comparison of these components:
Component | Use Case | Complexity |
---|---|---|
JList | Displaying a list of items | Moderate |
JComboBox | Creating a drop-down list | Moderate |
JTable | Displaying data in a table format | High |
Whether you’re just starting out with JList or looking to level up your Java Swing skills, we hope this guide has given you a deeper understanding of JList and its capabilities. With its balance of versatility and simplicity, JList is a powerful tool for creating interactive user interfaces in Java Swing. Happy coding!