Mastering NPM Commands | A Project Management Guide
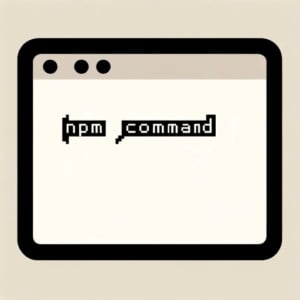
At IOFLOOD, we’ve found that mastering npm commands is essential for efficient project management. Whether it’s installing dependencies, running scripts, or managing packages, understanding npm commands can streamline development workflows. To help simplify the learning process we’ve decided to provide this guide to empower you in navigating npm commands with confidence and ease, just like we do in our daily work at IOFLOOD.
This guide will not only introduce you to the fundamental npm commands but also dive deep into the more sophisticated ones. By the end of this journey, you’ll be equipped with the knowledge to utilize npm commands to their fullest, optimizing your development process and enhancing your project management skills.
Let’s embark on this adventure together, exploring the vast possibilities that npm commands unlock for developers.
TL;DR: What is an npm command?
npm commands are terminal commands used to manage Node.js packages in your projects. They can be used by calling
npm [command] [options]
. Commands can be used such asupdate
,install
, orstart
.
For example, to install a package, you would use:
npm install package-name
# Output:
# + package-name@version
# added 1 package in Xs
In this simple example, we use the npm install
command to add a new package to our project. The output indicates the package name and version that was installed, along with the number of packages added and the time taken for the installation. This command is fundamental for managing dependencies in Node.js projects.
Ready to dive deeper into npm commands? Keep reading to explore more commands and their uses in depth, enhancing your project management and development workflow.
Table of Contents
Getting Started: NPM Commands
Kickstart Your Project with npm init
Every great journey begins with a single step, and in the npm universe, that step is npm init
. This command is your gateway to initializing new Node.js projects. It prompts you to fill in details like the project’s name, version, and entry point, eventually creating a package.json
file that outlines your project’s metadata and dependencies.
npm init
# Output:
# This utility will walk you through creating a package.json file.
# It only covers the most common items, and tries to guess sensible defaults.
# See package.json for more details.
After executing npm init
, you’re presented with a series of prompts. Completing these steps crafts a package.json
file, effectively setting the foundation of your project. This file is crucial as it records your project’s dependencies and scripts, making it a cornerstone for project management with npm.
Installing Dependencies with npm install
One of the first commands you’ll use in any npm-based project is npm install
. This command fetches the Node.js packages your project depends on and installs them into the node_modules
directory. Let’s say you’re building a web application and you need Express, a fast, unopinionated, minimalist web framework for Node.js. Here’s how you’d add it to your project:
npm install express
# Output:
# + [email protected]
# added 1 package from 1 contributor and audited 102 packages in 2.97s
Executing npm install express
not only downloads the Express package but also updates your package.json
and package-lock.json
files to reflect this addition. This ensures that anyone else working on the project or any deployment processes can replicate the environment exactly, thanks to these detailed records.
Launching Your App with npm start
Once your project is set up and dependencies are installed, npm start
serves as the command to breathe life into it. Typically defined in your package.json
file under the scripts section, this command starts your application as specified by the start script.
npm start
# Output:
# > node app.js
# Server running at http://127.0.0.1:3000/
In this example, npm start
executes the start script which is often a simple node command to run your application’s main file, in this case, app.js
. This demonstrates how npm commands not only help in setting up and managing dependencies but also in running and testing your applications, making them an indispensable tool in the developer’s toolkit.
Elevate Your npm Skills
Keeping Packages Up to Date with npm update
As your project grows, keeping dependencies up-to-date becomes crucial for security and functionality. The npm update
command is designed to update all packages listed to the latest version, respecting semver rules found in your package.json
file. Here’s how you can use it:
npm update
# Output:
# + package-name@new-version
# updated 1 package in 3.654s
After running npm update
, npm checks for newer versions of your packages that are compatible with the version ranges specified in your package.json
. If updates are available, it installs them, ensuring your project stays current and secure.
Faster, Reliable Installs with npm ci
When setting up a new instance of your project, be it in development or a CI/CD pipeline, npm ci
is your go-to command for installing dependencies. Unlike npm install
, npm ci
provides faster, more reliable builds by installing directly from package-lock.json
, bypassing the package.json resolution stage.
npm ci
# Output:
# added 123 packages in 5.67s
This command ensures that everyone working on the project, as well as your deployment pipelines, use exactly the same dependencies, significantly reducing “It works on my machine” problems.
Supercharging Workflows with npm Scripts
npm scripts are a powerful feature that allows you to automate repetitive tasks such as testing, building, and deploying your application. You can define scripts in your package.json
file under the “scripts” section. Here’s an example of a custom script to start your application:
"scripts": {
"start": "node server.js"
}
To run this script, you would use the command npm run start
. This approach not only simplifies your workflow but also ensures consistency across environments.
npm run start
# Output:
# > node server.js
# Server listening on port 3000
The beauty of npm scripts lies in their flexibility and the ability to chain multiple commands together, making them a powerful tool in your development arsenal. By mastering these advanced npm commands and techniques, you can significantly optimize your development workflow, making your projects more efficient and your work more productive.
npm vs. Other Package Managers
Choosing Between npm and Yarn
When it comes to JavaScript project management, npm is not the only player in the field. Yarn, another popular package manager, offers some distinct features. The choice between npm and Yarn can influence your project’s efficiency and ease of use. Let’s explore a simple comparison:
# Installing a package using npm
npm install lodash
# Installing a package using Yarn
yarn add lodash
# Output for npm:
# + [email protected]
# added 1 package in 4.528s
# Output for Yarn:
# success Saved 1 new dependency.
# info Direct dependencies
# └─ [email protected]
# Done in 0.89s.
While both commands achieve the same goal—adding a package to your project—the output and the time taken can vary. This example illustrates the efficiency and user-friendly output format that Yarn might offer over npm in some scenarios. However, npm has been catching up with performance improvements and feature additions, making the choice less about technical limitations and more about personal or team preferences.
Custom npm Commands via package.json
One of npm’s powerful features is the ability to create custom commands through scripts in your package.json
file. This allows for a level of automation and customization that can greatly enhance your workflow. For instance, if you frequently run a series of commands for testing, you can simplify this process with a custom npm command.
"scripts": {
"test": "echo 'Running tests...' && mocha"
}
To execute this custom command, you would use npm run test
, which would output something like this:
npm run test
# Output:
# Running tests...
# (output from mocha tests here)
This simple addition to your package.json
can save time and ensure consistency across development environments. By leveraging npm’s flexibility to define custom commands, developers can streamline their workflows, making project management more efficient and effective.
Handling Errors of npm Commands
Resolving EPEERINVALID
Errors
One common issue developers face when using npm is the EPEERINVALID
error. This error occurs when the peer dependency requirements of installed packages conflict. For example, if one package requires a different version of a dependency than what another package requires, npm will throw this error. Here’s how you might encounter it:
npm install
# Output:
# npm ERR! code EPEERINVALID
# npm ERR! peerinvalid The package [email protected] does not satisfy its siblings' peerDependencies requirements!
To resolve this, you need to identify the conflicting packages and update them to compatible versions. Often, this involves checking the package’s documentation or repository for the correct versions that satisfy all peer dependencies.
Handling ENOTFOUND
Errors
Another frequent challenge is the ENOTFOUND
error, which occurs when npm can’t find a package or version specified in package.json
. This might happen if the package is private, misspelled, or has been removed from the npm registry. Here’s an example of encountering this error:
npm install non-existent-package
# Output:
# npm ERR! code ENOTFOUND
# npm ERR! errno ENOTFOUND
# npm ERR! network request to http://registry.npmjs.org/non-existent-package failed
To fix this, verify the package name and version in your package.json
. If the package is private, ensure you’re logged into npm and have access to the package. If the package has been deprecated or removed, you may need to find an alternative or contact the package maintainer.
Avoiding Version Conflicts
Version conflicts can significantly disrupt a project. To avoid these, it’s crucial to understand semantic versioning (semver) and how npm handles package versions. Using the ^
and ~
symbols in your package.json
can help manage minor and patch updates without breaking your project. For instance, ^1.0.0
allows updates to any 1.x.x version, while ~1.0.0
restricts updates to 1.0.x versions.
"dependencies": {
"some-package": "^1.0.0"
}
This approach ensures that you receive necessary updates that don’t introduce breaking changes, keeping your project stable while benefiting from bug fixes and improvements.
Understanding and troubleshooting common npm command issues are essential skills for developers. By effectively resolving EPEERINVALID
and ENOTFOUND
errors and managing version conflicts, you can maintain a smooth and efficient development workflow.
The Heart of npm: package.json
The package.json
file is the cornerstone of any Node.js project managed with npm. It contains metadata about the project, such as the name, version, and dependencies required to run and build the project. Understanding this file is crucial for effective npm command usage.
Here’s a basic example of what a package.json
might look like:
{
"name": "your-project-name",
"version": "1.0.0",
"description": "A brief description of your project",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"dependencies": {
"express": "^4.17.1"
}
}
This file outlines the project’s dependencies, such as Express in this example. The ^
symbol before the version number indicates that the project is compatible with minor updates to Express, ensuring that non-breaking updates are automatically applied when you run npm update
.
Navigating node_modules
The node_modules
directory is where npm stores the packages that your project depends on. When you run npm install
, npm downloads the packages listed in your package.json
and places them in this directory.
Understanding the significance of this directory is key to grasping how npm commands work. It’s also important to note that while this directory is crucial during development, it’s typically not included in version control. Instead, the package.json
and package-lock.json
files ensure that anyone who works on the project can generate an identical node_modules
directory by running npm install
.
package-lock.json: Ensuring Consistency
The package-lock.json
file is automatically generated when you install packages using npm. It locks the versions of the installed packages, ensuring that every install results in the exact same file structure in node_modules
, regardless of when or where npm install
is run.
{
"name": "your-project-name",
"version": "1.0.0",
"lockfileVersion": 1,
"requires": true,
"dependencies": {
"express": {
"version": "4.17.1",
"resolved": "https://registry.npmjs.org/express/-/express-4.17.1.tgz",
"integrity": "sha512-xyzabc123"
}
}
}
This file plays a crucial role in ensuring that your project remains consistent and functional across different environments and installations, mitigating the “It works on my machine” syndrome.
Understanding these fundamental aspects of the npm ecosystem sets the stage for mastering npm commands. These components ensure that your development process is smooth, efficient, and consistent, making npm an indispensable tool for modern JavaScript development.
Advanced Usage of npm Commands
Integrating npm into CI/CD Pipelines
Continuous Integration and Continuous Deployment (CI/CD) pipelines are crucial for modern development practices, ensuring code is automatically tested and deployed. npm commands play a vital role in these processes. For instance, using npm test
in your CI pipeline can automatically run your project’s tests. Here’s an example:
npm test
# Output:
# > [email protected] test
# > echo "Error: no test specified" && exit 1
# This indicates no tests are specified yet in package.json.
This code block demonstrates how npm test
can be integrated into a CI pipeline, executing any tests specified in the package.json
. It’s a simple yet powerful way to ensure your codebase remains stable and reliable with every change.
npm and Automated Testing
Automated testing is another area where npm commands can significantly enhance your workflow. By utilizing npm run test:coverage
, you can not only run your tests but also generate a coverage report, ensuring your code meets quality standards. Here’s how you might set it up in your package.json
:
"scripts": {
"test:coverage": "nyc mocha"
}
Executing this script with npm commands provides a detailed report on which parts of your code are not covered by tests, highlighting areas for improvement.
Further Resources for npm Command Mastery
To deepen your understanding of npm commands and their application in development workflows, here are three invaluable resources:
- Official NPM Documentation – A comprehensive resource that covers all npm commands, their options, and use cases.
The official Node.js Guides offers informative guides on how to utilize npm in Node.js projects effectively.
The NPM Blog – The NPM Blog provides insights, and tips on maximizing npm usage.
These resources offer a wealth of information for both beginners and experienced developers looking to leverage npm commands in their projects. From detailed command references to practical guides on integrating npm into your development practices, these links are a gateway to mastering npm.
Recap: npm Commands Usage Guide
In this comprehensive guide, we’ve navigated the vast universe of npm commands, starting with the basics and advancing through to more complex usage. npm commands serve as the backbone for managing JavaScript projects, providing a powerful set of tools for developers to efficiently manage dependencies, scripts, and more.
We began with an introduction to fundamental npm commands like npm init
, npm install
, and npm start
, demonstrating how these commands lay the groundwork for any Node.js project. We explored how npm init
crafts the essential package.json
file, while npm install
adds necessary packages, and npm start
breathes life into our projects.
Moving to more advanced territory, we delved into commands such as npm update
for keeping packages up-to-date and npm ci
for reliable builds. We also uncovered the power of npm scripts, showcasing how they can automate and streamline development workflows.
Our journey took us even further as we compared npm with other package managers like Yarn, highlighting the considerations for choosing the right tool for your projects. We also explored creating custom npm commands, enhancing flexibility and efficiency in project management.
Feature | npm | Yarn |
---|---|---|
Performance | Fast | Very Fast |
Custom Scripts | Yes | Yes |
Package Management | Comprehensive | Efficient |
As we wrap up, it’s clear that mastering npm commands is crucial for modern JavaScript development. The ability to navigate and utilize npm effectively can significantly streamline project management and enhance productivity. Whether you’re just starting out or looking to refine your npm skills, this guide has provided the foundation and insights needed to leverage npm commands in your development endeavors.
With the knowledge of npm commands at your fingertips, you’re now well-equipped to tackle any JavaScript project with confidence. Happy coding!