Java Record Keyword: Simplify Immutable Data Carriers
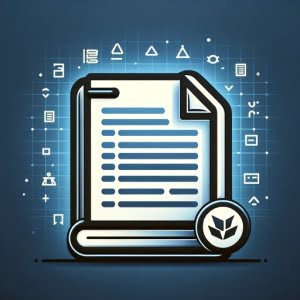
Are you finding it challenging to manage boilerplate code in your Java classes? You’re not alone. Many developers grapple with this task, but there’s a feature that can make this process a breeze.
Like a skilled carpenter, Java’s ‘record’ feature can help you craft clean, concise data carriers. These data carriers can simplify your code, making it more readable and maintainable.
This guide will walk you through the ins and outs of using ‘record’ in Java. We’ll explore ‘record’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java Record!
TL;DR: What is ‘record’ in Java?
‘Record’ is a keyword introduced in Java 14 that helps you create simple, immutable data carrier classes without boilerplate code. Instantiated with the syntax,
record className(dataType x, dataType y) {}
It’s a quick and efficient way to encapsulate a group of related data.
Here’s a simple example:
record Point(int x, int y) {}
Point p = new Point(5, 10);
System.out.println(p.x());
// Output:
// 5
In this example, we’ve declared a record
named Point
with two fields, x
and y
. We then created a new Point
instance p
with x
as 5 and y
as 10. Finally, we printed the value of x
using the automatically generated x()
method, which resulted in the output ‘5’.
This is just a basic way to use ‘record’ in Java, but there’s much more to learn about this powerful feature. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Declaring and Using Java Record: A Beginner’s Guide
- Advanced Usage of Java Record
- Exploring Alternatives to Java Record
- Troubleshooting Java Record: Common Issues and Solutions
- Understanding the Need for ‘record’ in Java
- Exploring ‘record’ in Larger Projects and Real-World Scenarios
- Wrapping Up: Record Keyword in Java
Declaring and Using Java Record: A Beginner’s Guide
Java Record is a special kind of class that helps you encapsulate related data without the need for boilerplate code. Let’s dive into how to declare and use a ‘record’ in Java.
To declare a record, you use the record
keyword, followed by the record name and the state description enclosed in parentheses. Here’s an example:
record Employee(String name, int age) {}
In this code, we’ve declared a record named Employee
with two fields, name
and age
.
To use this record, you can create a new instance of Employee
like this:
Employee e = new Employee("John", 25);
System.out.println(e.name());
System.out.println(e.age());
// Output:
// John
// 25
As you can see, we’ve created a new Employee
instance e
with name
as ‘John’ and age
as 25. We then printed the values of name
and age
using the automatically generated methods name()
and age()
, respectively.
The beauty of Java Record is its simplicity. It eliminates the need for boilerplate code, such as getters and setters, which are automatically provided by the record
keyword. However, it’s worth noting that records are immutable – once a record is created, you can’t change its state. This can be a potential pitfall if you need mutable data carriers.
Advanced Usage of Java Record
Java Record isn’t just for simplifying data carriers; it can also be used in more complex scenarios, such as with generics, inheritance, and interfaces. Let’s explore these advanced uses of ‘record’.
Working with Generics
Records can be used with generics to create type-safe data carriers. Here’s an example:
record Pair<K, V>(K key, V value) {}
Pair<String, Integer> p = new Pair<>("Alice", 25);
System.out.println(p.key());
System.out.println(p.value());
// Output:
// Alice
// 25
In this code, we’ve created a generic Pair
record that can hold a pair of any two types. We then created a Pair
instance p
with a String
key ‘Alice’ and an Integer
value 25.
Inheritance and Interfaces
While records cannot extend other classes, they can implement interfaces. Here’s an example of a record implementing an interface:
interface Named {
String name();
}
record Employee(String name, int age) implements Named {}
Employee e = new Employee("John", 30);
System.out.println(e.name());
// Output:
// John
In this code, we’ve created a Named
interface with a name()
method. Our Employee
record implements this interface, so it must provide a name()
method. However, because name
is a field in our record, Java automatically generates this method for us.
Using records can greatly simplify your code, but they also have their limitations. Records can’t extend other classes, and they’re immutable, meaning their state can’t be changed after they’re created. However, their simplicity and the automatic generation of methods like equals()
, hashCode()
, and toString()
make them a powerful tool for many Java developers.
Exploring Alternatives to Java Record
While Java Record is a powerful feature, it’s not always the best tool for the job. There are other ways to handle data carriers in Java, such as traditional classes, abstract classes, and interfaces. Let’s explore these alternatives and see how they compare to ‘record’.
Traditional Classes
Before the introduction of ‘record’, developers often used traditional classes to create data carriers. Here’s an example:
public class Employee {
private final String name;
private final int age;
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Employee e = new Employee("John", 30);
System.out.println(e.getName());
// Output:
// John
In this code, we’ve created a traditional class Employee
with two fields, name
and age
. We’ve also included a constructor and getter methods. While this approach offers more flexibility than ‘record’ (for example, you can make your class mutable), it also comes with more boilerplate code.
Abstract Classes and Interfaces
Abstract classes and interfaces can also be used to define data carriers. However, these are more abstract constructs and are typically used when you need to define a contract for classes, not when you need to encapsulate data.
Choosing between ‘record’, traditional classes, abstract classes, and interfaces depends on your specific needs. If you need a simple, immutable data carrier, ‘record’ is a great choice. If you need more flexibility, a traditional class might be a better fit. And if you’re defining a contract for classes, consider using an abstract class or interface.
Troubleshooting Java Record: Common Issues and Solutions
Like any feature, using ‘record’ in Java can sometimes lead to unexpected issues. Let’s explore some common problems you might encounter and how to solve them.
Immutable Fields
One of the main characteristics of ‘record’ is that it’s immutable. This means once a record is created, its state can’t be changed. If you try to modify a field of a record, you’ll get a compile-time error. Here’s an example:
record Point(int x, int y) {}
Point p = new Point(5, 10);
p.x() = 15; // This will cause a compile-time error
To avoid this issue, always remember that records are designed to be immutable data carriers. If you need a mutable data carrier, consider using a traditional class instead.
Inheritance Limitations
Records cannot extend other classes, except for java.lang.Record
. If you try to make a record extend another class, you’ll get a compile-time error. Here’s an example:
class MySuperClass {}
record MyRecord(int x, int y) extends MySuperClass {} // This will cause a compile-time error
To get around this limitation, consider using interfaces or abstract classes. Records can implement any number of interfaces, providing some of the benefits of inheritance.
Best Practices and Optimization
When using ‘record’, it’s important to follow best practices for optimal performance and readability. Here are a few tips:
- Use ‘record’ when you need a simple, immutable data carrier.
- Avoid using ‘record’ for mutable data carriers or complex class hierarchies.
- Use descriptive names for your records and their fields to increase code readability.
By understanding these common issues and following best practices, you can make the most of the ‘record’ feature in Java.
Understanding the Need for ‘record’ in Java
Before the introduction of ‘record’ in Java 14, developers had to write a lot of boilerplate code to create data carrier classes. This not only made the code longer and harder to read, but also introduced potential sources of error.
Java Record was introduced to address these issues. It offers a compact syntax to declare classes that are intended to be simple data carriers. The ‘record’ keyword automatically infers a lot of the boilerplate code, such as constructors, getter methods, equals()
, hashCode()
, and toString()
methods. This leads to cleaner, more maintainable code.
A Brief History of ‘record’
Java Record was first introduced as a preview feature in Java 14 and finalized in Java 16. It was part of a series of features aimed at making Java more practical and efficient for everyday use.
‘record’ in the Larger Java Ecosystem
The introduction of ‘record’ fits into the larger context of Java’s evolution. Java has always valued readability and simplicity of code. By reducing boilerplate code and making data carrier classes more straightforward to declare, ‘record’ contributes to these goals.
Furthermore, ‘record’ complements other features in Java, such as ‘sealed classes’ and ‘pattern matching’. For example, ‘record’ and ‘sealed classes’ together can create algebraic data types, a powerful concept in functional programming.
In conclusion, while ‘record’ might seem like a small addition to the language, it plays a significant role in making Java code more concise, readable, and expressive.
Exploring ‘record’ in Larger Projects and Real-World Scenarios
The ‘record’ feature in Java is not just a tool for simplifying data carrier classes in small projects. It also has significant applications in larger, real-world scenarios.
Practical Applications of ‘record’
In large-scale projects, ‘record’ can be used to create clean, concise data models. This can be especially useful in applications that deal with large amounts of data, such as data analysis tools, databases, or web applications.
For example, consider a web application that retrieves user data from a database. The user data can be represented as a ‘record’, with fields for the user’s id, name, email, etc. This makes the data easy to handle and reduces the risk of errors.
Complementary Features to ‘record’
While ‘record’ is a powerful feature on its own, it often works best when used in conjunction with other features. For example, ‘record’ and ‘sealed classes’ can be used together to create algebraic data types, a concept from functional programming that can greatly enhance the expressiveness of your code.
Further Resources for Mastering Java Record
To deepen your understanding of ‘record’ and its applications, here are some additional resources:
- Java Keywords: A Comprehensive Guide – Explore the essential keywords of the Java programming language.
Java ‘extends’ Keyword – Explore the ‘extends’ keyword in Java for creating subclasses and implementing inheritance.
Java ‘volatile’ Keyword explores the ‘volatile’ Java keyword, for ensuring visibility and ordering variable updates.
Project Amber: The Origin of Java Record – The origin and features of Java Record from OpenJDK’s Project Amber.
Oracle’s Official Java Record Documentation provides full coverage of the Java Record feature.
Baeldung’s Guide to Java Record – An in-depth exploration of the Java Record keyword through Baeldung’s expert guide.
These resources provide a wealth of information about ‘record’ and related features, helping you to fully leverage the power of ‘record’ in your Java projects.
Wrapping Up: Record Keyword in Java
In this comprehensive guide, we’ve explored the ins and outs of Java Record, a feature introduced in Java 14 to simplify the creation of data carrier classes.
We began with the basics, learning how to declare and use a ‘record’ in Java. We then dove into more advanced usage, such as working with generics, inheritance, and interfaces. Along the way, we tackled common issues you might encounter when using ‘record’, such as immutable fields and inheritance limitations, providing solutions and workarounds for each issue.
We also looked at alternative approaches to handle data carriers in Java, comparing ‘record’ with traditional classes and abstract classes. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Java Record | Simple, concise, automatic generation of methods | Immutable, cannot extend other classes |
Traditional Class | Flexible, can be mutable | Requires more boilerplate code |
Abstract Class | Can define a contract for classes | More abstract, not ideal for encapsulating data |
Whether you’re just starting out with Java Record or looking to deepen your understanding, we hope this guide has given you a comprehensive view of ‘record’ and its applications.
With its simplicity and the automatic generation of methods, Java Record is a powerful tool for creating data carrier classes. Now, you’re well equipped to leverage the power of ‘record’ in your Java projects. Happy coding!