npm ci vs npm install | Key Differences Explained
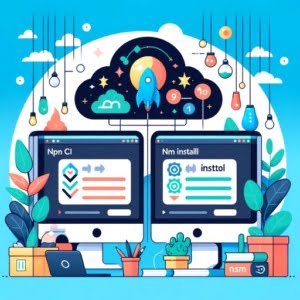
Ever wondered about the difference between npm ci and npm install? At IOFLOOD, we grappled with this question while striving for efficient package management. That’s why we’ve created a comparison guide to help you navigate the nuances between these commands. By understanding when to use each command, you’ll streamline your development process and ensure consistent dependency management.
This guide will explore the nuances of npm ci
versus npm install
, aiming to provide developers with the knowledge to choose the right tool for their development journey. Whether you’re a seasoned developer or just starting out, understanding these commands can significantly impact the success and efficiency of your projects.
Let’s dive in and demystify npm package installation!
TL;DR: What’s the Difference Between npm ci and npm install?
npm ci
installs dependencies directly from thepackage-lock.json
file, providing a faster and more reliable installation for continuous integration environments.npm install
, on the other hand, updates thepackage-lock.json
with the latest versions of dependencies.
Here’s a quick example of using npm ci
:
$ npm ci
This command will install all the dependencies specified in your package-lock.json
file without modifying it, ensuring that your project dependencies are consistent across all installations.
Dive deeper into the nuances of each command, their ideal usage scenarios, and gather advanced tips by continuing to read.
Table of Contents
Basic Use of npm Commands
npm install: The Foundation
npm install
is the basic command used by developers to add new dependencies to their projects or update existing ones. When you run npm install
, npm looks up the package you specified and installs the latest version that matches the version range in your package.json
file. If you don’t specify a package, npm install
will install all dependencies listed in your package.json
.
$ npm install lodash
# Output:
# + [email protected]
# added 1 package in 0.567s
This command installs the lodash
library, a modern JavaScript utility library delivering modularity, performance, & extras. The output indicates that the specific version of lodash
was added to the project. This demonstrates how npm install
updates your project dependencies to the latest versions within the limits of the version ranges specified in your package.json
file.
npm ci: Consistency Across Environments
npm ci
, short for Continuous Integration, is used primarily in automated environments, like CI/CD pipelines. It offers a more reliable and faster installation process by bypassing the package.json’s version ranges and installing directly from package-lock.json
. This ensures that all installations are identical, preventing discrepancies between development and production environments.
$ npm ci
# Output:
# added 1234 packages in 10.123s
After running npm ci
, you’ll notice the output indicates a significant number of packages added in a relatively short time. This highlights npm ci
‘s efficiency and its role in ensuring that the exact versions of dependencies are installed, as recorded in package-lock.json
, thereby enhancing project consistency and reliability.
Deciding Between npm install and npm ci
For beginners, understanding when to use npm install
versus npm ci
can streamline project setup and development. Use npm install
when adding new packages or updating existing ones in your development environment. Opt for npm ci
in continuous integration pipelines or when you need to ensure a clean, consistent installation of your project’s dependencies.
Advanced npm Command Uses
npm install in Complex Scenarios
When working on larger projects with multiple dependencies, npm install
can be used to manage updates in a more granular manner. For instance, updating a single package without affecting others can be crucial for maintaining project stability. Here’s how you can update a specific package using npm install
:
$ npm install express@latest
# Output:
# + [email protected]
# updated 1 package in 1.234s
This command updates the express
package to its latest version. The output confirms the update and shows how quickly the process completes. This capability is essential when you need to ensure that the rest of your dependencies remain unchanged, thus avoiding unintended updates that could introduce bugs into your project.
npm ci for Continuous Integration
npm ci
plays a pivotal role in continuous integration (CI) environments by ensuring that the exact versions of dependencies are installed every time. This consistency is crucial for testing and deployment processes. Here’s an example of npm ci
being used in a CI workflow:
$ npm ci --silent
# Output:
# (no output due to --silent flag)
The --silent
flag suppresses the npm output, which is often preferred in CI environments to keep the logs clean. While the output is not visible, npm ci
performs its task efficiently, installing all dependencies exactly as specified in package-lock.json
, ensuring a stable and consistent build environment.
Handling Private Registries with npm
Working with private registries often requires additional configuration. Both npm ci
and npm install
can be adapted for use with private packages. When configuring npm for a private registry, it’s important to include authentication details either in your .npmrc
file or as part of your CI environment variables. This ensures secure access to private packages while maintaining the efficiency and reliability of your dependency management workflow.
Understanding these advanced use cases of npm ci
and npm install
not only enhances your capability to manage dependencies more effectively but also ensures that your development and deployment processes are as smooth and error-free as possible.
Alternative Package Managers
Beyond npm: Yarn and pnpm
While npm ci
and npm install
are staples in the Node.js and JavaScript ecosystems, there are alternative tools that offer unique advantages. Two notable alternatives are Yarn and pnpm. Each of these package managers introduces different approaches to handling dependencies, which can be beneficial depending on your project’s needs.
Yarn Example
$ yarn install
# Output:
# Done in 0.56s.
Yarn’s install
command is akin to npm install
, but it’s known for better performance and more reliable dependency resolution. The output demonstrates Yarn’s efficiency, completing installations in a fraction of the time. This speed can significantly impact development workflows, especially in large projects.
pnpm Example
$ pnpm install
# Output:
# Packages: +1124
#++++++++++++++++++++++++++++++++++++++
# Progress: resolved 1124, reused 1124, downloaded 0, added 1124, done
pnpm stands out for its unique approach to node_modules, using a content-addressable filesystem to share packages across projects. This not only reduces disk space usage but also increases installation speed. The output shows how pnpm handles package installations, emphasizing the efficiency and disk space savings.
When to Use npm update
npm update
serves a different purpose compared to npm ci
and npm install
. It’s used to update existing project dependencies to their latest versions within the constraints of the package.json
file. This command is particularly useful for routine maintenance of your project, ensuring you have the latest and most secure versions of dependencies.
$ npm update lodash
# Output:
# + [email protected]
# updated 1 package in 0.567s
This command updates the lodash
library within the version constraints specified in your package.json
, showcasing npm update
‘s role in project upkeep. Understanding when to use npm update
is crucial for maintaining project health without introducing breaking changes.
Third-Party Tools and Their Place
In addition to Yarn and pnpm, there are other third-party tools and libraries that can enhance your npm workflows. Tools like npx
allow you to run npm package binaries without installing them globally, and dependency management utilities can help visualize and manage your project’s dependencies more effectively. While npm ci
and npm install
are foundational, exploring these alternatives can offer tailored solutions that better fit specific project requirements.
Resolving package-lock.json
Conflicts
Conflicts in package-lock.json
can arise when different versions of dependencies are installed than what’s expected. This often occurs in teams where different members may inadvertently update dependencies without syncing changes. Here’s how you can reset your package-lock.json
to match the project repository:
$ git checkout -- package-lock.json
$ npm install
# Output:
# up to date in 0.845s
# fixed 0 of 0 vulnerabilities
This command sequence first resets package-lock.json
to its state in the git repository, then npm install
runs to ensure all dependencies are correctly installed based on the reset file. This process helps in aligning team members with the correct versions of dependencies, reducing conflicts and ensuring consistency.
Tackling Slow Installation Times
Slow installation times with npm install
can be frustrating, especially in larger projects. Utilizing npm ci
can often provide a faster alternative due to its more deterministic nature and bypassing the dependency resolution process. However, to further optimize installation times, consider leveraging a caching mechanism:
$ npm install --cache /path/to/cache
# Output:
# added 204 packages in 11.123s
Specifying a cache directory with npm install
can significantly reduce installation times by reusing previously downloaded packages. This approach is beneficial in environments where dependencies do not change frequently, offering a balance between performance and flexibility.
Dependency Resolution Issues
Encountering dependency resolution problems can halt project progress. These issues often stem from incompatible version requirements among dependencies. To diagnose and resolve these issues, use the npm ls
command to identify conflicting dependencies:
$ npm ls <conflicting-package>
# Output:
# project-name@version
# └─┬ dependency-tree
# └── conflicting-package@version
The npm ls
command provides a detailed view of where the conflict arises in the dependency tree, allowing you to pinpoint the exact cause and address it appropriately. Understanding the dependency structure is crucial for resolving conflicts and ensuring that your project remains stable and functional.
Best Practices for npm Command Use
Adopting best practices can mitigate many common issues with npm ci
and npm install
. Regularly updating the package-lock.json
file, utilizing version control effectively, and maintaining clear communication within development teams are foundational strategies. Additionally, exploring npm’s documentation and community resources can provide further insights and solutions tailored to specific challenges.
npm Ecosystem Fundamentals
The Role of package-lock.json
The package-lock.json
file is a cornerstone of the npm ecosystem, ensuring that projects are reproducible, and dependencies remain consistent across installations. It locks down the versions of each package and its dependencies, which npm installed in your project. This file is automatically generated and should be committed to your version control system. Here’s an example of what happens when you first create a package-lock.json
file:
$ npm install
# Output:
# created a package-lock.json file
This simple command initializes your project with a package-lock.json
file, capturing the exact dependency tree at that moment. The creation of this file is crucial for maintaining project consistency, especially when collaborating with others. It ensures that everyone working on the project has the same versions of dependencies, reducing “it works on my machine” problems.
npm Dependency Management
npm manages project dependencies through the package.json
and package-lock.json
files. When you add a new package to your project using npm install
, npm updates both files. The package.json
file records the desired range of versions for your dependencies, allowing for updates within those ranges. In contrast, package-lock.json
records the exact version installed, ensuring that future installations match the development environment.
$ npm install express
# Output:
# + [email protected]
# added 1 package from 1 contributor and audited 101 packages in 2.547s
This command adds the express
framework to your project, demonstrating npm’s dependency management in action. The output shows the specific version of express
added, along with a summary of the audit report. This process highlights the balance npm strikes between flexibility in versioning and the need for consistency.
Understanding the npm ecosystem’s fundamentals, including the pivotal role of package-lock.json
and how npm manages dependencies, equips developers with the knowledge to navigate the complexities of package management. This foundational understanding is essential for making informed decisions about using npm commands effectively in various development scenarios.
Practical Usage of npm Commands
Automated Testing with npm ci
Incorporating npm ci
into your automated testing workflow can significantly enhance consistency and reliability. By using npm ci
, you ensure that every test run installs dependencies precisely as defined in package-lock.json
, mirroring the exact environment in which your application will run. Here’s an example of integrating npm ci
into a CI/CD pipeline script:
$ npm ci
# Output:
# added 1234 packages in 10.123s
In this script, npm ci
efficiently installs all required packages, as indicated by the output. This step is crucial for preventing discrepancies between development, testing, and production environments, thereby increasing confidence in the test results.
Deployment Pipelines and npm install
Using npm install
in deployment pipelines can be beneficial when you aim to incorporate the latest updates of dependencies that comply with the version ranges specified in your package.json
. This approach can be particularly useful for projects where staying up-to-date with dependencies is critical. However, it’s essential to balance this with the need for stability and reliability in your production environment.
Package Publishing with npm
Publishing packages is a vital part of the npm ecosystem. Whether you’re sharing a library within your organization or with the global npm community, understanding the nuances of package versioning and dependencies is crucial. Here’s how you can publish a package using npm:
$ npm publish
# Output:
# + [email protected]
This command publishes your package to the npm registry, making it available for others to install. The output confirms the successful publication of your package, including its version. This process is a key part of contributing to the vast npm ecosystem, enabling code reuse and collaboration.
Further Resources for npm Command Mastery
To deepen your understanding of npm commands and their applications in development workflows, the following resources are invaluable:
- npm Documentation – An official comprehensive guide on all npm commands, their options, and use cases.
Node.js Guides – These guides cover in application development, package management and publishing with Node.js.
The npm Blog – For the latest news, updates, and insights into the npm ecosystem, the npm blog is a great resource.
Exploring these resources can significantly enhance your proficiency with npm commands, contributing to more efficient and effective development practices.
Wrapping Up: npm ci vs npm install
In this comprehensive guide, we’ve navigated the nuanced differences between npm ci
and npm install
, two cornerstone commands in the npm ecosystem. Understanding these commands enhances your ability to manage project dependencies with precision and efficiency.
We began with the basics, illustrating the primary functions and immediate benefits of each command. Through practical examples, we demonstrated how npm install
is the go-to for adding new packages and updating existing ones, while npm ci
shines in continuous integration environments by ensuring consistent installations.
We then explored advanced scenarios, highlighting how these commands adapt to complex project structures and continuous integration workflows. We delved into the significance of package-lock.json
in achieving deterministic builds with npm ci
and discussed how npm install
facilitates package updates within specified version ranges.
Lastly, we examined alternative package managers like Yarn and pnpm, offering insights into the broader landscape of dependency management tools. Each alternative brings unique advantages to the table, from performance enhancements to innovative approaches to node_modules.
Command | Ideal Use Case | Speed | Reliability |
---|---|---|---|
npm install | Adding/updating packages | Moderate | High (with caveats) |
npm ci | CI/CD pipelines | Fast | Very High |
Whether you’re just starting out or looking to refine your npm command skills, we hope this guide has equipped you with the knowledge to make informed decisions. The choice between npm ci
and npm install
depends on your specific needs, but understanding their differences is key to optimizing your development workflow.
With the ability to navigate these commands, you’re better prepared to tackle project dependencies, ensuring your projects are both efficient and consistent. Happy coding!