Public Static Void Main String Args: Java Entry Points
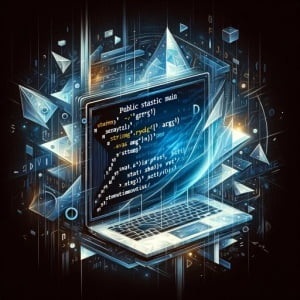
Ever puzzled over the meaning of 'public static void main(String[] args)'
in Java? Consider it the ignition key to a Java program. This line of code is the starting point, the entry point that sets the wheels in motion.
In this guide, we will dissect 'public static void main(String[] args)'
piece by piece, helping you understand its purpose and usage in Java. We’ll delve into the details of each part, discuss how command-line arguments are passed, and even touch on how other programming languages handle the entry point of a program.
So, let’s jump in and start unraveling the mystery of ‘public static void main(String[] args)’ in Java!
TL;DR: What Does ‘public static void main(String[] args)’ Mean in Java?
'public static void main(String[] args)'
is theentry point
for any Java program. It’s where the Java Virtual Machine (JVM) starts executing the program. Here’s a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve created a basic Java program that prints ‘Hello, World!’ to the console. The 'public static void main(String[] args)'
method is where the JVM begins executing the program.
This is just a basic understanding of
'public static void main(String[] args)'
. Continue reading for a more detailed breakdown and advanced usage scenarios.
Table of Contents
- Breaking Down ‘public static void main(String[] args)’
- Command-Line Arguments in Java
- Overloading the Main Method in Java
- Entry Points in Other Programming Languages
- Troubleshooting Java’s Main Method
- Java Programming Language and Syntax
- The Role of Main Method in Java
- Applying ‘public static void main(String[] args)’ in Real-World Java Projects
- Further Resources for Mastering Java’s Main Method
- Wrapping Up: ‘public static void main(String[] args)’ in Java
Breaking Down ‘public static void main(String[] args)’
Let’s dissect ‘public static void main(String[] args)’ to understand what each part means and why it’s necessary for a Java program. Here’s the breakdown:
- public: This keyword means that the method is accessible anywhere, including from outside the class it’s declared in.
static: By using ‘static’, we’re saying that the main method can be run without needing an instance of the class.
void: This keyword indicates that the main method doesn’t return any value.
main: ‘main’ is the name of this method. The JVM looks for a method with this name when it starts running a program.
String[] args: This is an array of ‘String’ objects. It’s used to receive any command-line arguments that were passed when the program was started.
To illustrate, let’s look at a basic Java program:
public class BasicProgram {
public static void main(String[] args) {
System.out.println("This is a basic Java program.");
}
}
# Output:
# This is a basic Java program.
In this program, ‘public static void main(String[] args)’ is the entry point. When the JVM starts running the program, it calls this method. The method then executes the code it contains, which in this case is a statement that prints ‘This is a basic Java program.’ to the console.
Command-Line Arguments in Java
The ‘String[] args’ in ‘public static void main(String[] args)’ is used for passing command-line arguments to your Java program. But what does that mean?
Command-line arguments are inputs that you can pass to your program when you run it. These arguments are stored in the ‘String[] args’ array, and you can use them in your program as needed.
Here’s an example of how you can use command-line arguments in a Java program:
public class CommandLineArgs {
public static void main(String[] args) {
for (String arg : args) {
System.out.println("Argument: " + arg);
}
}
}
# If you run the program with these command-line arguments:
# java CommandLineArgs Hello World
# Output:
# Argument: Hello
# Argument: World
In this program, we’re using a ‘for each’ loop to iterate over the ‘args’ array. For each argument in the array, we print ‘Argument: ‘ followed by the argument.
So, if you run the program with ‘java CommandLineArgs Hello World’, the JVM starts executing the ‘main’ method and passes ‘Hello’ and ‘World’ as command-line arguments. These arguments are stored in the ‘args’ array. The program then prints each argument to the console.
Overloading the Main Method in Java
In Java, it’s possible to overload the main method. Overloading is a feature in Java where a class can have multiple methods with the same name but different parameters.
Here’s an example of overloading the main method:
public class MainOverload {
public static void main(String[] args) {
System.out.println("Main method with String[] args");
}
public static void main(String arg) {
System.out.println("Main method with String arg");
}
}
# If you run the program with this command:
# java MainOverload
# Output:
# Main method with String[] args
In this example, we have two main methods. The first is the standard one that the JVM calls when the program starts. The second is an overloaded version that takes a single ‘String’ argument instead of a ‘String’ array. However, note that the JVM only calls the first main method when the program is run.
Entry Points in Other Programming Languages
Different programming languages have different ways of designating the entry point of a program. For instance, in Python, the entry point is usually a block of code under 'if __name__ == "__main__":'
. In C and C++, the entry point is a function called ‘main’.
Here’s an example of an entry point in Python:
if __name__ == "__main__":
print("This is the entry point of the program.")
# Output:
# This is the entry point of the program.
In this Python program, the code under 'if __name__ == "__main__":'
is the entry point. When the program is run, this code is executed.
Troubleshooting Java’s Main Method
While working with Java, you might encounter a few issues related to the main method. Let’s discuss some of these problems and how to resolve them.
Dealing with ‘Main method not found’
One common error is ‘Main method not found in class’. This typically happens when the JVM can’t find the ‘public static void main(String[] args)’ method in your class. It could be due to a typo, incorrect method signature, or the method being missing altogether.
Here’s an example of a program that will cause this error:
public class MainNotFound {
public static void main(String arg) {
System.out.println("Hello, World!");
}
}
# If you try to run this program, you'll get an error:
# Error: Main method not found in class MainNotFound
In this example, the main method’s signature is incorrect. It should take a ‘String’ array as an argument, not a single ‘String’. To fix the error, you need to correct the main method’s signature:
public class MainNotFound {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Now, if you run the program, you'll get the expected output:
# Hello, World!
Remember, the correct signature for the main method in Java is ‘public static void main(String[] args)’. Any deviation from this can result in the ‘Main method not found’ error.
Java Programming Language and Syntax
Java is a high-level, object-oriented programming language known for its ‘Write Once, Run Anywhere’ capability. This means that compiled Java code can run on all platforms that support Java, without the need for recompilation.
Java syntax is largely influenced by C++, but with a simpler object model and fewer low-level facilities. Java uses .java
files for source code, which are then compiled into .class
files by the Java compiler. These .class
files contain bytecodes that can be interpreted by the Java Virtual Machine (JVM).
Here’s a simple Java program:
public class SimpleProgram {
public static void main(String[] args) {
System.out.println("A Simple Java Program");
}
}
# Output:
# A Simple Java Program
This program consists of a single class named SimpleProgram
with one method: public static void main(String[] args)
. The System.out.println("A Simple Java Program");
line is a simple command to print a string to the console.
The Role of Main Method in Java
The public static void main(String[] args)
method plays a crucial role in Java programming. It serves as the entry point for any Java program and is the first method that gets executed when a Java application starts.
The JVM initiates the program by calling the main method. If the main method is not defined in your program, or if it’s defined with a different signature, the JVM will throw an error, and the program won’t run.
Understanding the public static void main(String[] args)
method is fundamental to mastering Java, as it’s the starting point for learning how to build applications in this versatile language.
Applying ‘public static void main(String[] args)’ in Real-World Java Projects
In larger Java projects, the ‘public static void main(String[] args)’ method still serves as the entry point. However, its role becomes more about directing traffic. It might call methods to initialize application components, load data, or bootstrap application frameworks.
Here’s an example of how the main method might be used in a larger application:
public class LargeApplication {
public static void main(String[] args) {
initializeComponents();
loadData();
startApplication();
}
private static void initializeComponents() {
// Code to initialize application components
}
private static void loadData() {
// Code to load data
}
private static void startApplication() {
// Code to bootstrap the application
}
}
In this example, the main method is calling other methods to perform various startup tasks. This is a common pattern in larger Java applications.
Further Resources for Mastering Java’s Main Method
Want to dive deeper into understanding the ‘public static void main(String[] args)’ method and Java programming in general? Here are some resources that might help:
- Understanding Java Methods: Step-by-Step Tutorial – This tutorial covers essential Java methods for beginners.
Mastering Main Method Usage in Java – Learn how to create and execute Java programs using the main method.
Method Overriding in Java Explained – Delve into method overriding in Java for achieving polymorphic behavior in your classes.
Oracle’s Java Tutorials: Oracle’s official tutorials are a great resource for learning Java from scratch.
Baeldung’s Guide to Java offers a collection of tutorials and articles on the Java main method.
GeeksforGeeks Java Programming Language offers a wide range of Java tutorials and articles on the main method.
Wrapping Up: ‘public static void main(String[] args)’ in Java
In this comprehensive guide, we’ve explored the significance and usage of ‘public static void main(String[] args)’ in Java, the entry point of any Java program.
We began with the basics, breaking down ‘public static void main(String[] args)’ to understand what each part means and why it’s necessary. We then moved on to more advanced topics, such as how command-line arguments are passed through ‘String[] args’ and how they can be used in a program. We also discussed how the main method can be overloaded and how other programming languages handle the entry point of a program.
Along the way, we tackled common issues you might encounter with the main method, such as ‘Main method not found’, and provided solutions to help you overcome these challenges. We also delved into the background of the Java programming language, its syntax, and how the main method fits into the bigger picture.
Method | Accessibility | Returns a Value | Name | Arguments |
---|---|---|---|---|
public | Accessible anywhere | void (No return value) | main | String[] args (Command-line arguments) |
Whether you’re just starting out with Java or you’re looking to deepen your understanding, we hope this guide has given you a clearer picture of ‘public static void main(String[] args)’ and its role in Java programming.
Mastering ‘public static void main(String[] args)’ is a fundamental step in your journey as a Java programmer. With this knowledge, you’re well equipped to start creating your own Java applications. Happy coding!