Java Methods: Your Ultimate Guide to Mastery
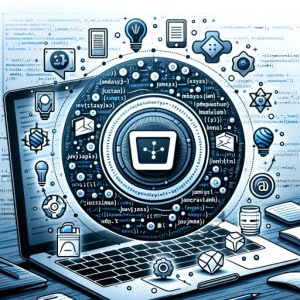
Are Java methods causing you confusion? You’re not alone. Many developers find themselves puzzled when it comes to understanding and using Java methods. Think of Java methods as the building blocks of a Java program, each performing a specific task, much like the gears in a well-oiled machine.
Java methods are a fundamental part of Java programming, and mastering them can significantly enhance your coding skills, making your programs more efficient and your code more readable.
In this guide, we’ll walk you through the process of understanding and using Java methods effectively, from basic usage to advanced techniques. We’ll cover everything from defining and calling methods, to more complex uses such as method overloading and overriding, and even alternative approaches like using lambda expressions or streams in Java.
So, let’s dive in and start mastering Java methods!
TL;DR: What is a Method in Java?
A method in Java is a block of code that performs a specific task. It is defined with the name of the method, followed by parentheses (), for example:
public void greet()
creates a method used to greet the user. Java provides several types of methods, including instance methods, static methods, and abstract methods.
Here’s a simple example:
public void introduce(String name) {
System.out.println("Hello, my name is " + name);
}
// Usage:
// introduce("John");
// Output:
// 'Hello, my name is John'
In this example, we’ve defined a method called introduce()
. This method accepts one parameter (name)
and prints a personalized greeting when called. The public keyword means this method can be accessed from anywhere, while the void keyword means it doesn’t return any value.
This is just a basic way to define and use a method in Java, but there’s much more to learn about Java methods. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Defining and Calling Java Methods: A Beginner’s Guide
- Delving Deeper: Method Overloading and Overriding
- Advanced Techniques: Lambda Expressions and Streams
- Troubleshooting Java Methods: Common Issues and Solutions
- Java Fundamentals: Object-Oriented Programming and Encapsulation
- Java Methods in Larger Projects: A Wider Perspective
- Wrapping Up: Mastering Java Methods
Defining and Calling Java Methods: A Beginner’s Guide
In Java, a method is a block of code that performs a specific task. Defining a method means creating a new method, and calling a method means using a method that has already been defined.
Defining a Method in Java
Let’s start with a basic example of defining a method in Java:
public void greet() {
System.out.println('Hello, World!');
}
// Output:
// 'Hello, World!'
In this example, public
is the access modifier, which means this method can be accessed from anywhere. void
is the return type, which means this method doesn’t return any value. greet()
is the method name, and the code inside the curly braces {}
is the method body.
Calling a Method in Java
Now that we have defined our method, let’s see how to call it:
greet();
// Output:
// 'Hello, World!'
In this example, we simply use the method name followed by parentheses ()
to call the method. When this line of code is executed, it calls the greet()
method, which prints ‘Hello, World!’.
Advantages and Potential Pitfalls
One of the main advantages of using methods in Java is reusability. Once a method is defined, it can be reused multiple times. This can make your code more organized and easier to understand.
However, one potential pitfall to be aware of is the scope of the method. The scope of a method refers to where it can be called from. For example, a method defined inside a class can only be called within that class unless it’s declared as public.
Understanding how to define and call methods in Java is a fundamental skill for any Java programmer. As you continue to learn more about Java methods, you’ll find them to be a powerful tool for organizing and reusing your code.
Delving Deeper: Method Overloading and Overriding
As you progress with Java, you’ll encounter more complex uses of methods. Two such concepts are method overloading and method overriding. Both are fundamental to Java’s object-oriented programming.
Method Overloading in Java
Method overloading in Java is a technique where a class has more than one method of the same name, but with different parameters. It’s a way of increasing the readability of the program.
Here’s an example:
void demo (int a) {
System.out.println ('a: ' + a);
}
void demo (int a, int b) {
System.out.println ('a and b: ' + a + ',' + b);
}
double demo(double a) {
System.out.println('double a: ' + a);
return a*a;
}
// Output:
// 'a: 10'
// 'a and b: 10,20'
// 'double a: 5.5'
In this example, we have three methods named demo()
, but they all have different parameters. The first method takes one integer, the second method takes two integers, and the third method takes one double. When calling the demo()
method, Java will use the version that matches the parameters.
Method Overriding in Java
Method overriding in Java occurs when a subclass provides a specific implementation of a method that is already provided by its parent class. It’s used for runtime polymorphism and to provide the specific implementation of the method.
Here’s an example:
class Animal {
void move() {
System.out.println('Animals can move');
}
}
class Dog extends Animal {
void move() {
System.out.println('Dogs can walk and run');
}
}
// Output:
// 'Dogs can walk and run'
In this example, the Dog
class overrides the move()
method of the Animal
class. So, when we call the move()
method on a Dog
object, the version in the Dog
class is executed.
Best Practices
While both method overloading and overriding are powerful tools in Java, it’s important to use them appropriately. Overloading can make your code more readable and flexible. However, it should be used sparingly, as having too many overloaded methods can make your code more complex and harder to debug.
On the other hand, overriding is essential for achieving runtime polymorphism in Java. It allows a subclass to provide a specific implementation of a method that is already provided by its parent class. However, when overriding methods, you should always adhere to the contract of the superclass method.
Advanced Techniques: Lambda Expressions and Streams
Java is a versatile language that offers multiple ways to accomplish tasks. Two alternative approaches to using traditional Java methods are lambda expressions and streams. These techniques can provide more efficient, readable, and concise code.
Lambda Expressions in Java
Lambda expressions are a new and important feature of Java which was introduced in Java 8. It provides a clear and concise way to represent one method interface using an expression.
Here’s an example of a lambda expression in Java:
interface Drawable{
public void draw();
}
public class LambdaExpressionExample {
public static void main(String[] args) {
int width=10;
//without lambda, Drawable implementation using anonymous class
Drawable d=new Drawable(){
public void draw(){System.out.println('Drawing '+width);}
};
d.draw();
}
}
// Output:
// 'Drawing 10'
In this example, we are using a lambda expression to define the draw method of the Drawable interface. This provides a more concise and readable way to define methods in Java.
Streams in Java
Streams in Java are a sequence of elements supporting sequential and parallel aggregate operations. They can be used to perform complex data processing tasks on sequences of elements, such as filtering, mapping, or matching.
Here’s an example of using a stream in Java:
List<String> list = Arrays.asList('Java', 'Python', 'C++', 'JavaScript');
list.stream()
.filter(s -> s.startsWith('J'))
.forEach(System.out::println);
// Output:
// 'Java'
// 'JavaScript'
In this example, we’re using a stream to filter a list of programming languages and print only the ones that start with ‘J’. This shows how streams can be used to perform complex tasks with a simple and readable syntax.
Advantages and Disadvantages
While lambda expressions and streams can provide more efficient and readable code, they also have their disadvantages. Lambda expressions can be hard to understand for beginners, and they can make debugging more difficult. Streams can be slower than traditional loops and are not suitable for all types of data processing tasks.
However, in the right situations, these techniques can be powerful tools for writing efficient and readable Java code. As always, the best approach depends on the specific requirements of your project.
Troubleshooting Java Methods: Common Issues and Solutions
While Java methods are a powerful tool, they can sometimes lead to unexpected issues. Let’s discuss some of the common problems you might encounter when using Java methods and how to solve them.
Dealing with NullPointerException
A NullPointerException is a runtime exception thrown by the JVM when your code attempts to use a null reference in a situation where an object is required.
For example, consider the following code:
String str = null;
int length = str.length();
// Output:
// java.lang.NullPointerException
In this example, we’re trying to find the length of a string that is null. This results in a NullPointerException.
A good practice to avoid NullPointerException is to perform a null check before using an object reference:
String str = null;
if (str != null) {
int length = str.length();
}
Understanding Method Visibility Problems
Method visibility refers to where a method can be accessed from. It’s determined by the access modifier used when defining the method (public, private, protected, or default).
For example, if a method is declared as private, it can only be accessed within the same class. Attempting to access it from another class will result in a compile-time error.
public class MyClass {
private void myMethod() {
System.out.println('Hello, World!');
}
}
public class Test {
public static void main(String[] args) {
MyClass obj = new MyClass();
obj.myMethod(); // Compile-time error
}
}
// Output:
// error: myMethod() has private access in MyClass
In this example, we’re trying to call a private method from another class, which results in a compile-time error. To fix this issue, we could change the access modifier of the method to public, or provide a public method in the same class that calls the private method.
Java methods are a powerful tool, but they can sometimes lead to unexpected issues. Understanding these issues and how to solve them can help you write more robust and reliable code.
Java Fundamentals: Object-Oriented Programming and Encapsulation
To fully grasp the concept of Java methods, it’s essential to understand the principles of object-oriented programming (OOP) and encapsulation in Java.
Object-Oriented Programming in Java
Java is fundamentally an object-oriented programming language. In OOP, we design our software using objects and classes. An object is a real-world entity that has state and behavior, and a class is a blueprint or template from which objects are created.
Methods in Java are a key part of this object-oriented approach. They define the behavior of an object, and each method performs a specific task.
Here’s a simple example of a class and an object in Java:
public class Car {
// State of the Car
String color;
String model;
// Behavior of the Car, defined by methods
void accelerate() {
System.out.println('The car is accelerating.');
}
void brake() {
System.out.println('The car is braking.');
}
}
Car myCar = new Car();
myCar.accelerate();
myCar.brake();
// Output:
// 'The car is accelerating.'
// 'The car is braking.'
In this example, Car
is a class that has two methods: accelerate()
and brake()
. When we create a Car
object myCar
, we can call these methods on the object.
Encapsulation in Java
Encapsulation is one of the four fundamental principles of object-oriented programming. It refers to the bundling of data (fields) and methods together into a single unit (class).
Encapsulation provides control over the data by making the fields private and providing public methods to access and change them. This is also known as data hiding.
Here’s an example of encapsulation in Java:
public class Employee {
// private field
private String name;
// public method to access the private field
public String getName() {
return name;
}
public void setName(String newName) {
name = newName;
}
}
Employee emp = new Employee();
emp.setName('John');
System.out.println(emp.getName());
// Output:
// 'John'
In this example, name
is a private field, and getName()
and setName()
are public methods that provide access to the private field. This is a fundamental concept that underlies the use of methods in Java.
Understanding these fundamental concepts is key to mastering the use of methods in Java. They provide the foundation upon which more advanced concepts, such as inheritance and polymorphism, are built.
Java Methods in Larger Projects: A Wider Perspective
Java methods are not just useful for small programs or tasks; they’re a fundamental part of larger software projects as well. In large-scale software development, methods play a crucial role in keeping the code organized, maintainable, and reusable.
Exploring Inheritance and Polymorphism
Once you’re comfortable with Java methods, it’s beneficial to explore related concepts like inheritance and polymorphism. These are key principles of object-oriented programming that work closely with methods.
- Inheritance allows one class to inherit the fields and methods of another class. This can help reduce code duplication and make the code more organized.
public class Animal {
void eat() {
System.out.println('The animal eats');
}
}
public class Dog extends Animal {
void bark() {
System.out.println('The dog barks');
}
}
Dog dog = new Dog();
dog.eat();
dog.bark();
// Output:
// 'The animal eats'
// 'The dog barks'
In this example, Dog
class inherits the eat()
method from the Animal
class. So, we can call the eat()
method on a Dog
object.
- Polymorphism allows one interface to be used for a general class of actions. This can make the code more flexible and extensible.
public class Animal {
void sound() {
System.out.println('The animal makes a sound');
}
}
public class Dog extends Animal {
void sound() {
System.out.println('The dog barks');
}
}
Animal myDog = new Dog();
myDog.sound();
// Output:
// 'The dog barks'
In this example, Dog
class overrides the sound()
method of the Animal
class. So, when we call the sound()
method on a Dog
object stored in an Animal
reference, the version in the Dog
class is executed.
Further Resources for Mastering Java Methods
To deepen your understanding of Java methods and related concepts, here are some valuable resources:
- What Can You Do with Java? A Comprehensive Overview – Explore real-world examples of Java applications.
Importing Packages in Java – Understand best practices for managing dependencies and organizing import statements.
Exploring Comments in Java – Learn about single-line and multi-line comments, and their usage conventions.
W3Schools’ Java Methods offers concise and clear explanations about Java methods and their application.
Baeldung’s Guide to Java Methods provides a detailed explanation of Java methods, along with examples.
GeeksforGeeks’ Java Programming Language covers everything you need to know about Java, from basic to advanced concepts.
By exploring these resources and practicing your skills, you can become proficient in using Java methods and apply them effectively in your projects.
Wrapping Up: Mastering Java Methods
In this comprehensive guide, we’ve delved deep into the world of Java methods, exploring their usage, advantages, and potential pitfalls. We’ve shed light on the fundamental building blocks of Java programming, equipping you with the knowledge to write efficient, maintainable, and robust code.
We started off with the basics, understanding how to define and call Java methods. We then explored advanced concepts like method overloading and overriding, providing you with a solid foundation in Java’s object-oriented programming. We also introduced alternative approaches like lambda expressions and streams, showcasing Java’s versatility and adaptability.
Along the way, we addressed common issues you might encounter when working with Java methods, such as NullPointerExceptions and method visibility problems, providing you with practical solutions and workarounds. We further delved into the underlying principles of object-oriented programming and encapsulation, facilitating a deeper understanding of Java methods.
Here’s a quick comparison of the methods and techniques we’ve discussed:
Technique | Pros | Cons |
---|---|---|
Basic Java Methods | Simple, Reusable | Limited Functionality |
Method Overloading/Overriding | Increased Flexibility, Code Readability | Complexity in Debugging |
Lambda Expressions | Concise, Readable Code | Difficult for Beginners |
Streams | Efficient Data Processing | Not Suitable for All Tasks |
Whether you’re a beginner just starting out with Java or an intermediate developer looking to level up your skills, we hope this guide has equipped you with a deeper understanding of Java methods.
Mastering Java methods is a significant step towards becoming a proficient Java programmer. With the knowledge you’ve gained from this guide, you’re well on your way to writing efficient and effective Java code. Happy coding!