Java Import Statements: Managing Classes and Packages
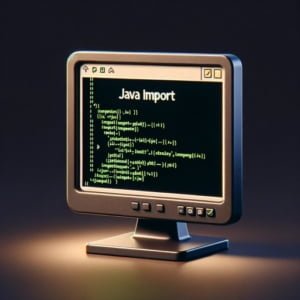
Are you finding it challenging to manage your Java classes and packages? You’re not alone. Many developers find themselves puzzled when it comes to handling Java imports, but we’re here to help.
Think of Java’s import statement as a skilled librarian – it helps organize your code by bringing in the classes and packages you need, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using import in Java, from the basics to more advanced techniques. We’ll cover everything from importing single classes to entire packages, as well as alternative approaches.
Let’s get started and master Java imports!
TL;DR: How Do I Use Import in Java?
To use import in Java, you use the
import
statement at the beginning of your file, for exampleimport java.util.*;
. This statement allows you to use classes and packages without having to write their full names every time you need them.
Here’s a simple example:
// Import ArrayList from java.util package
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
// Create an ArrayList object
ArrayList<String> animals = new ArrayList<String>();
// Add elements to ArrayList
animals.add("Lion");
animals.add("Tiger");
animals.add("Elephant");
System.out.println(animals);
// Output:
// [Lion, Tiger, Elephant]
}
}
In this example, the ArrayList
is a resizable array, which can be found in the java.util
package. It provides us dynamic arrays in Java, allowing us to add or remove the elements as per the requirement. The elements in the ArrayList
are maintained insertion order.
This is just a basic way to use the
import
statement in Java, but there’s much more to learn about managing your classes and packages efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Using the Java Import Statement: A Beginner’s Guide
The import
statement in Java is a powerful tool that allows you to access classes and packages from other parts of your program or from Java’s extensive libraries. Let’s delve into the basics of using the import
statement in Java.
Importing Single Classes
To import a single class in Java, you use the import
statement followed by the fully qualified name of the class, which includes its package.
Here’s an example:
import java.util.Scanner;
Scanner myScanner = new Scanner(System.in);
String input = myScanner.nextLine();
// Output:
// (Assuming 'Hello, World!' was entered)
// 'Hello, World!'
In this example, we import the Scanner class from the java.util package. This allows us to create a Scanner object to read user input from the console. By using the import
statement, we can refer to the Scanner class by its simple name, making our code cleaner and easier to read.
Importing Entire Packages
If you need to use several classes from the same package, you can import the entire package using the *
wildcard. Here’s how:
import java.util.*;
List<String> myList = new ArrayList<>();
myList.add("Hello, World!");
System.out.println(myList.get(0));
// Output:
// 'Hello, World!'
In this example, we import all classes from the java.util package. This allows us to use any class from this package, such as List and ArrayList, without having to import each class individually.
While this method can make your code shorter, it can also lead to confusion if you have classes with the same name in different packages. Therefore, it’s generally better to import only the specific classes you need.
Advantages and Potential Pitfalls
The main advantage of using the import
statement in Java is that it makes your code more readable by allowing you to refer to classes by their simple names. However, overuse of the *
wildcard can lead to confusion and potential naming conflicts. Therefore, it’s best to use it sparingly and only when you need to use many classes from the same package.
Best Practices for Java Import Usage
As you become more comfortable with Java imports, it’s essential to understand the best practices. These will help you write code that is not only efficient but also easy to read and maintain.
Single Class vs. Wildcard Imports
One of the key decisions you’ll make when using imports is whether to import single classes or entire packages. While wildcard imports can make your code shorter, they can also lead to confusion and potential naming conflicts. Therefore, it’s generally recommended to import only the specific classes you need.
Here’s an example of how this can make your code clearer:
import java.util.List;
import java.util.ArrayList;
List<String> myList = new ArrayList<>();
myList.add("Hello, World!");
System.out.println(myList.get(0));
// Output:
// 'Hello, World!'
By importing only the List and ArrayList classes, we make it clear which classes we’re using. This can make our code easier to understand for other developers and help prevent naming conflicts.
Organizing Your Imports
Another best practice is to organize your imports in a logical way. This can make your code easier to read and understand. One common approach is to group imports by package, with a blank line between each group.
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.ArrayList;
import com.mycompany.myapp.MyClass;
In this example, we group together imports from the same package and separate different packages with a blank line. This makes it easy to see at a glance which packages we’re using in our code.
By following these best practices, you can use Java imports to make your code more efficient, readable, and maintainable.
Exploring Alternative Approaches to Java Imports
While using the import
statement is the most common way to manage dependencies in Java, it’s not the only way. Let’s explore some alternative approaches you can use, particularly when working with larger projects.
IDE’s Auto-Import Feature
Most Integrated Development Environments (IDEs) like IntelliJ IDEA and Eclipse have an auto-import feature. This feature automatically adds import statements for you as you write your code. It can save you time and reduce the risk of errors.
Here’s an example of how this might work in IntelliJ IDEA:
// You start typing the name of a class
List<String> myList = new ArrayList<>();
// IntelliJ IDEA recognizes that you're using the List and ArrayList classes
// It automatically adds the appropriate import statements at the top of your file
import java.util.List;
import java.util.ArrayList;
While the auto-import feature can be a great time-saver, it’s important to review the imports it adds to ensure they’re the ones you intended to use. This is especially true when working with classes that have the same name but come from different packages.
Using Build Tools: Maven and Gradle
When working with larger projects, you might find it useful to use a build tool like Maven or Gradle. These tools can manage your project’s dependencies for you, ensuring that all the necessary libraries are included in your project.
Here’s an example of how you might specify a dependency in a Maven pom.xml
file:
<dependencies>
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
</dependency>
</dependencies>
In this example, we specify that our project depends on the Guava library. Maven will automatically download this library and make it available to our project, saving us the trouble of manually managing this dependency.
While build tools can be very powerful, they also come with a learning curve. If you’re new to Java, you might want to start by mastering the import
statement before moving on to these tools.
In conclusion, while the import
statement is a fundamental part of Java, it’s not the only way to manage your dependencies. Depending on your needs, you might find it useful to use an IDE’s auto-import feature or a build tool like Maven or Gradle.
Troubleshooting Java Import Issues
While Java imports are a powerful tool, they can sometimes lead to issues and conflicts. Let’s explore some of the common problems you might encounter and how to resolve them.
Resolving Import Conflicts
One common issue is import conflicts, which can occur when you have two classes with the same name from different packages. Java won’t know which one you’re referring to, leading to a compile error.
Here’s an example:
import java.util.Date;
import java.sql.Date;
Date date = new Date();
// Output:
// Error: reference to Date is ambiguous
In this example, we’re trying to import two different Date classes from the java.util and java.sql packages. Java doesn’t know which one we’re referring to when we try to create a new Date object, leading to an error.
To resolve this issue, you can use the fully qualified name of the class when you declare your object:
java.util.Date date = new java.util.Date();
// Output:
// No error
In this example, we specify that we want to use the Date class from the java.util package, resolving the conflict.
Handling Classpath Issues
Another common issue is classpath issues. The classpath is the list of directories and JAR files where Java looks for your classes. If a class isn’t on the classpath, Java won’t be able to find it, leading to a ClassNotFoundException
.
Here’s an example:
import com.mycompany.myapp.MyClass;
MyClass myObject = new MyClass();
// Output:
// Error: java.lang.ClassNotFoundException: com.mycompany.myapp.MyClass
In this example, we’re trying to import a class that isn’t on the classpath, leading to an error.
To resolve this issue, you need to add the directory or JAR file containing your class to the classpath. You can do this when you run your program using the -cp
or -classpath
option:
java -cp /path/to/your/classes com.mycompany.myapp.MyApp
In this example, we add the directory containing our classes to the classpath, allowing Java to find our class.
By understanding these common issues and how to resolve them, you can use Java imports more effectively and avoid common pitfalls.
Understanding Java Classpath and Packages
To fully comprehend Java imports, it’s essential to understand two fundamental concepts: the Java classpath and packages.
The Role of Java Classpath
The Java classpath is a parameter—set either on the command-line or through an environment variable—that tells the Java Virtual Machine or the Java compiler where to look for user-defined classes and packages. In simpler terms, it’s like a map guiding Java to locate the classes you’ve imported.
For instance, when you run a Java program from the command line like this:
java -cp /path/to/your/classes com.mycompany.myapp.MyApp
The -cp
or -classpath
option followed by /path/to/your/classes
sets the classpath. This way, Java knows where to find the com.mycompany.myapp.MyApp
class.
The Concept of Packages
Packages in Java are a way of grouping related classes and interfaces together. They serve as a namespace that manages the classes in a project. When you use the import
statement, you’re essentially telling Java to bring in a specific class or an entire package of classes into your namespace.
For instance, when you write:
import java.util.List;
You’re importing the List
class from the java.util
package into your namespace. Now, you can simply use List
in your code instead of the fully qualified name java.util.List
.
Packages help organize code, prevent naming conflicts, and control access with the help of visibility modifiers. They are a crucial aspect of Java programming that works hand in hand with the import statement for efficient coding.
The Role of Java Import in Larger Projects
Java imports play a critical role in larger projects. They help manage dependencies and interact with build tools and Integrated Development Environments (IDEs) to maintain a clean and efficient codebase.
Interactions with Build Tools
In larger Java projects, build tools like Maven and Gradle are often used to manage dependencies. These tools can automatically download and manage libraries, relieving you from manual work. However, it’s crucial to understand that these tools don’t replace the need for Java imports. Even when using Maven or Gradle, you still need to import the classes in your code.
Interactions with IDEs
IDEs like IntelliJ IDEA and Eclipse often have features that interact with Java imports. They can automatically add necessary imports as you type, organize and optimize imports, and highlight unused imports. These features can significantly improve your productivity and the cleanliness of your code.
Understanding the Java Classloader
The Java Classloader is a part of the Java Runtime Environment (JRE) that dynamically loads Java classes into the Java Virtual Machine (JVM). It plays a crucial role in the functioning of Java imports. When you import a class, the Classloader is responsible for locating it and loading it into the JVM. Understanding how the Classloader works can give you deeper insights into the functioning of Java imports.
Further Resources for Mastering Java Imports
If you’re interested in learning more about Java imports, here are a few resources that can help:
- Exploring Java’s Versatility: What Is It Used For? – Understand Java’s contribution to cloud computing.
Methods Usage in Java – Master defining and calling methods to modularize and reuse code in Java applications.
Understanding Java Syntax – Learn about keywords, operators, control flow statements, and other language constructs
Oracle’s Java Tutorials cover many topics, including packages and the
import
statement.GeekforGeek’s Guide to Java Imports provides a deep dive into the workings of Java imports.
Java Code Geeks’ Understanding the Java Import Statement article provides a good understanding of the Java import statement.
These resources should provide a good starting point for deepening your understanding of Java imports and how they interact with other components of the Java ecosystem.
Wrapping Up: Mastering Java Imports
In this comprehensive guide, we’ve delved into the world of Java imports, an essential tool for managing dependencies in your Java programs.
We started with the basics, learning how to import single classes and entire packages in Java. We then explored best practices for using imports, such as when to import single classes vs. entire packages, and how to organize your imports for maximum readability.
We ventured into more advanced territory, discussing alternative approaches such as using an IDE’s auto-import feature or build tools like Maven and Gradle. We also tackled common issues you might encounter when using imports, such as import conflicts and classpath issues, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Java Import | Direct control, readability | Manual management |
IDE Auto-Import | Time-saving, reduces errors | Requires review |
Build Tools | Handles large projects, automatic management | Learning curve |
Whether you’re just starting out with Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Java imports and their role in effective coding.
Understanding and effectively using Java imports is a crucial skill for any Java developer. With this guide, you’re well equipped to use Java imports to manage your dependencies efficiently and write clean, readable code. Happy coding!