Maven Central | Guide to Java’s Maven Library Repo
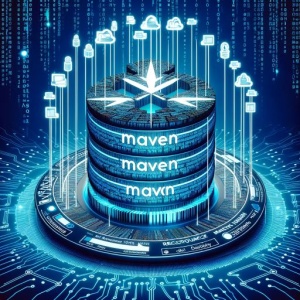
Ever felt overwhelmed while trying to use Maven Central for your Java projects? You’re not alone. Many developers find themselves in a maze when it comes to navigating Maven Central. Think of Maven Central as a vast library, stocked with all the resources you need for your Java projects.
Like a well-stocked library, Maven Central can provide you with all the resources you need. From basic usage to advanced techniques, Maven Central is a treasure trove of Java libraries and resources waiting to be explored.
This guide will walk you through everything you need to know about Maven Central. We’ll cover everything from searching for libraries, adding dependencies to your project, updating dependencies, to more advanced features such as using the API, uploading your own libraries, and managing versions.
So, let’s dive in and start mastering Maven Central!
TL;DR: What is Maven Central and How Do I Use It?
Maven Central
is a public repository that hosts Java libraries and resources, which can be used in your Maven projects. Dependencies can be added to your project by using identifiers such asgroupId
,artifactId
, andversion
. Here’s a simple example of how to add a dependency from Maven Central to your project:
<dependency>
<groupId>com.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
</dependency>
In this example, we’ve added a dependency to our Maven project. The groupId
, artifactId
, and version
are the unique identifiers for the library we want to include in our project. Maven Central will then handle downloading and including this library in our project.
But Maven Central’s capabilities go far beyond this. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Maven Central for Beginners: Basic Use
- Leveraging Advanced Features of Maven Central
- Exploring Alternatives to Maven Central
- Troubleshooting Common Issues in Maven Central
- Understanding Maven: The Build Tool Behind Maven Central
- Maven Central in the Larger Java Ecosystem
- Wrapping Up: Maven Central for Java Projects
Maven Central for Beginners: Basic Use
Searching for Libraries
Maven Central is a treasure trove of Java libraries. But how do you find the right one for your project? It’s simple. You can use the search function on the Maven Central website. Just type in the name or the functionality you’re looking for, and Maven Central will list all the libraries that match your search criteria.
Adding Dependencies to Your Project
Once you’ve found the right library, you can add it as a dependency to your Maven project. Here’s how you do it:
<dependency>
<groupId>com.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
</dependency>
In the above example, we’ve added a dependency to our project. The groupId
, artifactId
, and version
are the unique identifiers for the library we want to include in our project. Maven Central will then handle downloading and including this library in our project.
Updating Dependencies
What happens when a library you’re using gets updated? You’ll want to update the version in your project too. To do this, simply change the version
in your dependency declaration to the new version number. Maven Central will take care of the rest.
<dependency>
<groupId>com.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.1.0</version>
</dependency>
In the updated code block, we’ve changed the version number from 1.0.0
to 1.1.0
. This tells Maven Central to download and include the updated version of the library in our project.
Leveraging Advanced Features of Maven Central
Using the Maven Central API
Maven Central also provides an API that you can use to automate tasks or integrate Maven Central with other tools. For instance, you can use the API to search for libraries, get the latest version of a library, or even upload your own libraries. Here’s an example of how to use the API to search for a library:
curl 'https://search.maven.org/solrsearch/select?q=a:example-artifact&rows=20&wt=json'
In the above example, we are using the curl
command to send a GET request to the Maven Central API. The q
parameter in the URL specifies the query, in this case, we are searching for the artifact with the id ‘example-artifact’. The rows
parameter limits the number of results returned, and the wt
parameter specifies the response format (in this case, JSON).
Uploading Your Libraries
Maven Central isn’t just a place to download libraries, you can also upload your own. This allows other developers to use your libraries in their projects. To upload a library, you’ll need to create a JIRA account, create a new project, and then upload your library using Maven’s deploy
command.
Managing Versions
Maven Central also makes it easy to manage versions of libraries. When you upload a new version of your library, Maven Central automatically archives the old version. This means that other developers can still download and use the old version if they need to. To specify a version, you simply include it in the version
field of your dependency declaration.
<dependency>
<groupId>com.example</groupId>
<artifactId>example-artifact</artifactId>
<version>2.0.0</version>
</dependency>
In the updated code block, we’ve changed the version number to 2.0.0
. This tells Maven Central to download and include this specific version of the library in our project.
Exploring Alternatives to Maven Central
Considering JCenter
While Maven Central is a popular choice for hosting Java libraries, it’s not the only game in town. JCenter is another public repository that you might consider. It’s often praised for its speed and user-friendly interface. Here’s how you can add a dependency from JCenter to your project:
repositories {
jcenter()
}
dependencies {
implementation 'com.example:example-artifact:1.0.0'
}
In this example, we first declare jcenter()
as a repository, and then we add a dependency just like we would in Maven. However, keep in mind that as of February 2021, JCenter has announced that it will be winding down its services, so it’s recommended to migrate to other repositories.
The Power of Private Repositories
For more control over your libraries, you might consider setting up a private repository. A private repository gives you full control over who can access your libraries. You can set up a private repository using tools like Nexus or Artifactory. Here’s an example of how you can add a dependency from a private repository to your project:
repositories {
maven {
url "http://myprivaterepo.com/maven2"
}
}
dependencies {
implementation 'com.example:example-artifact:1.0.0'
}
In this example, we’re adding a dependency from a private repository hosted at http://myprivaterepo.com/maven2
. We declare the URL of the private repository in the repositories
block, and then we add the dependency as usual.
Remember, while private repositories give you more control, they also come with additional maintenance responsibilities. You’ll need to manage access, handle backups, and ensure uptime. So, weigh the benefits and drawbacks carefully before deciding.
Troubleshooting Common Issues in Maven Central
Resolving Dependency Conflicts
One of the common issues you might encounter when using Maven Central is dependency conflicts. This happens when two or more of your dependencies rely on different versions of the same library. Maven has a built-in mechanism for resolving these conflicts, but sometimes you might need to intervene manually. Here’s an example of how to exclude a conflicting dependency:
<dependency>
<groupId>com.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>com.conflict</groupId>
<artifactId>conflict-artifact</artifactId>
</exclusion>
</exclusions>
</dependency>
In the above example, we’re excluding the conflict-artifact
from com.conflict
that was being brought in by the example-artifact
dependency.
Dealing with Missing Artifacts
Another issue you might run into is missing artifacts. This can happen if a library has been removed from Maven Central or if there’s a network issue preventing Maven from downloading the library. In such cases, you can try searching for the library in other repositories or check your network connection.
Improving Download Speeds
If you find that downloading libraries from Maven Central is slow, there are a couple of things you can try. First, you can set up a local mirror of Maven Central. This will store copies of the libraries you download on your local machine, reducing the need to download them from Maven Central each time. Second, you can use a repository manager like Nexus or Artifactory, which can cache libraries and improve download speeds.
Understanding Maven: The Build Tool Behind Maven Central
Maven is a powerful tool for building and managing any Java-based project. It simplifies the build process by managing dependencies, compiling source code, packaging the code into a JAR file, and installing the package into a local repository or server.
mvn install
In the above example, we’re using the install
command, which is one of many lifecycle commands provided by Maven. This command compiles the source code, packages it into a JAR file, and installs the package into your local repository.
The Role of Dependencies in Java Projects
Dependencies are external Java libraries that your project needs to function properly. For example, if your project uses a JSON processing library like Jackson, then Jackson is a dependency of your project.
In Maven, dependencies are defined in a Project Object Model (POM) file, which is an XML file that contains information about the project and configuration details used by Maven to build the project.
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.10.3</version>
</dependency>
In the code block above, we’ve added a dependency to our Maven project. The groupId
, artifactId
, and version
elements specify the library that we want to include in our project. Maven will automatically download this library from Maven Central and include it in our project.
Understanding these fundamentals of Maven and dependencies is crucial to effectively navigate and utilize Maven Central, as it’s the primary repository from which Maven retrieves these dependencies.
Maven Central in the Larger Java Ecosystem
Maven Central and Continuous Integration
Continuous Integration (CI) is a development practice where developers integrate code into a shared repository frequently. Maven Central plays a crucial role in CI pipelines. When setting up a CI pipeline, Maven Central is often the source from which your build tools pull dependencies. This ensures that your application always has the latest, most secure versions of its dependencies.
Automated Testing and Maven Central
Automated testing is another area where Maven Central shines. Many Java testing libraries, like JUnit and Mockito, are hosted on Maven Central. This means you can easily add these libraries as dependencies in your project and start writing automated tests.
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
In the above example, we’ve added JUnit as a dependency to our project. The scope
element is set to test
, which tells Maven that this dependency is only required for testing.
Artifact Management with Maven Central
Maven Central is also an effective tool for artifact management. An artifact could be a JAR file, a WAR file, or any other file produced by your project. By hosting your artifacts on Maven Central, you can ensure they are accessible to other developers and CI/CD pipelines.
Further Resources for Mastering Maven Central
For those interested in delving deeper into Maven Central and its applications, here are some resources that provide further reading:
- Java Package Mastery Step-by-Step – Understand the role of the default package in Java and its limitations.
Hibernate in Java Overview – Explore Hibernate’s object-relational mapping (ORM) framework in Java.
Understanding Maven Concepts – Learn managing project dependencies and compiling source code in Maven.
Maven: The Complete Reference Guidebook delves into all aspects of using Maven for project management.
Official Apache Maven Project Guides covers various topics for effective project builds.
Maven by Example – Interactive PDF tutorial that uses practical examples to teach Maven.
Wrapping Up: Maven Central for Java Projects
In this comprehensive guide, we’ve delved into the world of Maven Central, a key repository for Java libraries and resources.
We began with the basics, learning how to search for libraries, add dependencies to your project, and update dependencies. We then ventured into more advanced territory, exploring the Maven Central API, uploading your own libraries, and managing versions. Along the way, we tackled common challenges you might encounter when using Maven Central, such as dependency conflicts, missing artifacts, and slow download speeds, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to Maven Central, comparing it with other repositories like JCenter and private repositories. Here’s a quick comparison of these repositories:
Repository | Pros | Cons |
---|---|---|
Maven Central | Comprehensive, reliable | Can be slow at times |
JCenter | Fast, user-friendly | Winding down its services |
Private Repositories | Full control, privacy | Additional maintenance responsibilities |
Whether you’re just starting out with Maven Central or you’re looking to level up your Java project management skills, we hope this guide has given you a deeper understanding of Maven Central and its capabilities.
With its vast collection of Java libraries and resources, Maven Central is a powerful tool for any Java developer. Happy coding!