Hibernate Java: A Basic to Advanced Tutorial
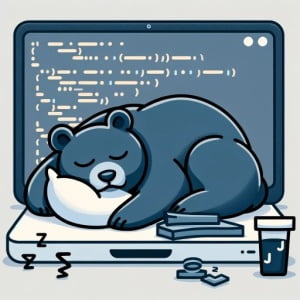
Are you finding it challenging to manage database operations in your Java applications? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled mediator, Hibernate simplifies the interaction between your Java application and the database. It provides an Object-Relational Mapping (ORM) library for Java, which streamlines database operations and reduces the need for repetitive SQL code.
This guide will walk you through the basics to advanced usage of Hibernate in Java. We’ll explore Hibernate’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Hibernate in Java!
TL;DR: What is Hibernate in Java and How Do I Use It?
Hibernate is an Object-Relational Mapping (ORM) library for Java, which simplifies database operations through the use of sessions:
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory()
It provides a framework for mapping an object-oriented domain model to a traditional relational database, making database operations more efficient and less error-prone.
Here’s a simple example of using Hibernate:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class App {
public static void main(String[] args) {
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory();
Session session = sessionFactory.openSession();
session.beginTransaction();
// Save code here
session.getTransaction().commit();
session.close();
}
}
# Output:
# This will initialize a Hibernate session, which can then be used for database operations.
In this example, we first import the necessary Hibernate classes. We then build a SessionFactory and open a Session. The beginTransaction() method starts a new database transaction.
This is a basic way to use Hibernate in Java, but there’s much more to learn about managing database operations efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Hibernate in Java: Basic Usage
- Hibernate in Java: Advanced Usage
- Alternative ORM Libraries for Java
- Troubleshooting Common Hibernate Issues
- Understanding ORM and Its Role in Java Development
- Expanding Hibernate Usage in Larger Java Projects
- Wrapping Up: Mastering Hibernate for Efficient Java Database Operations
Hibernate in Java: Basic Usage
Hibernate is a powerful tool, but it’s also quite user-friendly for beginners. Let’s walk through the process of setting up and using Hibernate in a Java application.
Step 1: Setting Up Hibernate
The first step is to add the Hibernate dependencies to your project. If you’re using Maven, you can add the following lines to your pom.xml
file:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.27.Final</version>
</dependency>
This will automatically download and add the necessary Hibernate libraries to your project.
Step 2: Creating a Hibernate Configuration File
Next, you’ll need to create a Hibernate configuration file (hibernate.cfg.xml
). This file tells Hibernate how to connect to your database and maps your Java classes to database tables.
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydatabase</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">update</property>
<!-- Add your classes here -->
<mapping class="com.example.MyClass" />
</session-factory>
</hibernate-configuration>
This file specifies the database connection details and includes the mapping of your Java classes to database tables.
Step 3: Writing a Simple Hibernate Application
Now, let’s use Hibernate to save a simple object to the database:
import org.hibernate.Session;
import org.hibernate.Transaction;
public class App {
public static void main(String[] args) {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction tx = session.beginTransaction();
MyClass obj = new MyClass();
obj.setName("Hello, Hibernate!");
session.save(obj);
tx.commit();
session.close();
}
}
# Output:
# This will save an instance of MyClass to the database with the name 'Hello, Hibernate!'.
In this example, we create a new instance of our MyClass object, set its name, and then use the session.save()
method to save it to the database. The tx.commit()
line commits the transaction, and session.close()
closes the session. Congratulations, you’ve just used Hibernate to interact with your database!
Hibernate in Java: Advanced Usage
Once you’ve got the hang of basic Hibernate operations, you can move on to more advanced features. Let’s explore Hibernate Query Language (HQL), Criteria API, and Transaction Management.
Hibernate Query Language (HQL)
Hibernate Query Language (HQL) is an object-oriented query language, similar to SQL, but it operates on Java objects rather than database tables. Here’s an example of using HQL to query data:
import org.hibernate.Session;
import org.hibernate.query.Query;
public class App {
public static void main(String[] args) {
Session session = HibernateUtil.getSessionFactory().openSession();
Query<MyClass> query = session.createQuery("FROM MyClass", MyClass.class);
List<MyClass> list = query.list();
for (MyClass obj : list) {
System.out.println(obj.getName());
}
session.close();
}
}
# Output:
# This will print the names of all MyClass objects stored in the database.
In this example, we create a Query
object using the session.createQuery()
method, passing in an HQL query string. The query.list()
method executes the query and returns a list of results.
Criteria API
The Criteria API is another way to retrieve data from the database. It’s a more programmatic and type-safe way to create queries, as you can use it to build complex queries using Java code.
import org.hibernate.Session;
import org.hibernate.Criteria;
import org.hibernate.criterion.Restrictions;
public class App {
public static void main(String[] args) {
Session session = HibernateUtil.getSessionFactory().openSession();
Criteria crit = session.createCriteria(MyClass.class);
crit.add(Restrictions.eq("name", "Hello, Hibernate!"));
List<MyClass> list = crit.list();
for (MyClass obj : list) {
System.out.println(obj.getName());
}
session.close();
}
}
# Output:
# This will print the names of all MyClass objects with the name 'Hello, Hibernate!'.
Here, we create a Criteria
object using the session.createCriteria()
method, and add a restriction using the Restrictions.eq()
method. The crit.list()
method executes the query and returns a list of results.
Transaction Management
In Hibernate, all database operations must be wrapped in a transaction. This ensures that if something goes wrong, all changes can be rolled back, maintaining the integrity of your data.
import org.hibernate.Session;
import org.hibernate.Transaction;
public class App {
public static void main(String[] args) {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
// Perform database operations
tx.commit();
} catch (RuntimeException e) {
if (tx != null) tx.rollback();
throw e;
} finally {
session.close();
}
}
}
# Output:
# This example shows how to handle transactions in Hibernate. If any operation fails, the transaction is rolled back.
In this example, we start a new transaction with session.beginTransaction()
. If any operation throws an exception, we roll back the transaction with tx.rollback()
. If everything goes well, we commit the transaction with tx.commit()
.
Alternative ORM Libraries for Java
While Hibernate is an excellent tool for handling database operations in Java, it’s not the only option. Let’s explore a few other Object-Relational Mapping (ORM) libraries you might consider: JPA, MyBatis, and JOOQ.
Java Persistence API (JPA)
JPA is a specification for ORM in Java. Hibernate is actually an implementation of the JPA specification, but there are other implementations as well, such as EclipseLink and OpenJPA.
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
public class App {
public static void main(String[] args) {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("my-pu");
EntityManager em = emf.createEntityManager();
// Perform database operations
em.close();
emf.close();
}
}
# Output:
# This will initialize a JPA EntityManager, which can then be used for database operations.
In this example, we create an EntityManagerFactory
and an EntityManager
, which are the equivalent of Hibernate’s SessionFactory
and Session
.
MyBatis
MyBatis is a SQL Mapping framework with a focus on simplicity and ease of use. It gives you full control over SQL queries, making it a good choice for complex database operations that are difficult to achieve with ORM libraries.
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
public class App {
public static void main(String[] args) {
SqlSessionFactory sqlSessionFactory = MyBatisUtil.getSqlSessionFactory();
SqlSession session = sqlSessionFactory.openSession();
// Perform database operations
session.close();
}
}
# Output:
# This will initialize a MyBatis SqlSession, which can then be used for database operations.
In this example, we create a SqlSessionFactory
and a SqlSession
, which are similar to Hibernate’s SessionFactory
and Session
.
JOOQ
JOOQ is a simple and modern database interaction library for Java. It generates Java code from your database and lets you build typesafe SQL queries through its fluent API.
import org.jooq.DSLContext;
import org.jooq.SQLDialect;
import org.jooq.impl.DSL;
public class App {
public static void main(String[] args) {
DSLContext create = DSL.using(DB.getConn(), SQLDialect.MYSQL);
// Perform database operations
create.close();
}
}
# Output:
# This will initialize a JOOQ DSLContext, which can then be used for database operations.
In this example, we create a DSLContext
, which is the main interface for database interactions in JOOQ.
These are just a few of the alternatives to Hibernate for handling database operations in Java. Each has its own strengths and weaknesses, and the best choice depends on your specific needs and the nature of your project.
Troubleshooting Common Hibernate Issues
Even with a powerful tool like Hibernate, you might encounter some hurdles. Let’s discuss some common issues and their solutions when using Hibernate in Java.
Issue 1: HibernateException: No CurrentSessionContext configured!
This error occurs when Hibernate can’t find a current session because it hasn’t been configured properly.
// Example of code that causes the error
Session session = sessionFactory.getCurrentSession();
# Output:
# org.hibernate.HibernateException: No CurrentSessionContext configured!
The solution is to make sure you have the following line in your hibernate.cfg.xml
:
<property name="current_session_context_class">thread</property>
This tells Hibernate to use the current thread to manage sessions.
Issue 2: javax.persistence.PersistenceException: org.hibernate.PersistentObjectException: detached entity passed to persist
This error occurs when you try to save an object that has a non-null id. The persist()
method is intended for new entities only, and Hibernate thinks the object is detached because its id is non-null.
// Example of code that causes the error
MyClass obj = new MyClass();
obj.setId(1L);
session.persist(obj);
# Output:
# javax.persistence.PersistenceException: org.hibernate.PersistentObjectException: detached entity passed to persist
The solution is to use the merge()
method instead of persist()
. The merge()
method is intended for updating existing entities.
// Corrected code
MyClass obj = new MyClass();
obj.setId(1L);
session.merge(obj);
Issue 3: org.hibernate.LazyInitializationException: could not initialize proxy – no Session
This error occurs when you try to access a lazily-loaded property of an entity, but there’s no Hibernate Session available to load it.
// Example of code that causes the error
MyClass obj = (MyClass) session.get(MyClass.class, 1L);
session.close();
System.out.println(obj.getSomeProperty());
# Output:
# org.hibernate.LazyInitializationException: could not initialize proxy - no Session
The solution is to make sure you access the property while the Session is still open, or to configure the property for eager loading.
// Corrected code
MyClass obj = (MyClass) session.get(MyClass.class, 1L);
System.out.println(obj.getSomeProperty());
session.close();
These are just a few examples of common issues you might encounter when using Hibernate. Remember, understanding the error message is key to finding a solution. Happy troubleshooting!
Understanding ORM and Its Role in Java Development
The concept of Object-Relational Mapping (ORM) is fundamental to understanding how Hibernate works. But what exactly is ORM, and why is it so important in Java development?
What is Object-Relational Mapping (ORM)?
ORM is a programming technique for converting data between incompatible type systems using object-oriented programming languages. It creates a virtual object database that can be used from within the programming language.
// Example of ORM in action
MyClass obj = new MyClass();
obj.setName("Hello, Hibernate!");
session.save(obj);
# Output:
# This will save an instance of MyClass to the database with the name 'Hello, Hibernate!'.
In this example, we create an instance of MyClass, set its name, and then save it to the database. The session.save()
method is part of the ORM framework (Hibernate), and it knows how to translate this operation into SQL commands that the database can understand.
Why is ORM Important in Java Development?
ORM is important because it lets developers work with databases using their preferred programming language (Java, in this case), rather than writing SQL queries or stored procedures. It abstracts the underlying SQL commands and allows developers to focus more on business logic rather than database operations.
How Does Hibernate Fit Into This Concept?
Hibernate is an implementation of the ORM concept. It provides a framework for mapping an object-oriented domain model to a relational database. Hibernate handles the mapping from Java classes to database tables (and from Java data types to SQL data types), and provides data query and retrieval facilities.
// Example of how Hibernate fits into the ORM concept
MyClass obj = (MyClass) session.get(MyClass.class, 1L);
# Output:
# This will retrieve an instance of MyClass from the database with the id 1.
In this example, we use the session.get()
method to retrieve an object from the database. Hibernate knows how to translate this into a SQL query, execute it, and then build the MyClass object from the result set.
Expanding Hibernate Usage in Larger Java Projects
As your Java projects grow in size and complexity, you’ll likely find that Hibernate continues to be a valuable tool for managing your database operations. However, you’ll also likely find that you need to integrate Hibernate with other technologies to meet your project’s needs.
Hibernate and Spring Framework
The Spring Framework is a comprehensive framework for building Java applications, and it integrates well with Hibernate. Spring provides a layer of abstraction over Hibernate, making it even easier to use.
// Example of using Hibernate with Spring
@Autowired
private SessionFactory sessionFactory;
public void save(MyClass obj) {
sessionFactory.getCurrentSession().save(obj);
}
# Output:
# This will save an instance of MyClass to the database using Spring's integration with Hibernate.
In this example, we use Spring’s @Autowired
annotation to inject a SessionFactory
. We can then use this SessionFactory
to get the current session and save our object to the database.
Hibernate and JDBC
While Hibernate abstracts a lot of the database operations, there might be times when you need to drop down to the lower-level JDBC (Java Database Connectivity) API. Hibernate provides ways to do this when needed.
// Example of using Hibernate with JDBC
Session session = sessionFactory.getCurrentSession();
Connection conn = session.doReturningWork(Connection::unwrap);
# Output:
# This will get a JDBC Connection from the current Hibernate Session.
In this example, we use the session.doReturningWork()
method to get a JDBC Connection
from the current Hibernate Session. This allows us to perform lower-level SQL operations if needed.
Hibernate and Database Design
Good database design is crucial for efficient operations, and Hibernate can help with this as well. Hibernate’s hbm2ddl.auto
configuration property can automatically create, update, or validate your database schema based on your entity classes.
// Example of using Hibernate for database design
<property name="hbm2ddl.auto">update</property>
# Output:
# This will tell Hibernate to automatically update the database schema to match the entity classes.
In this example, we set the hbm2ddl.auto
property to update
. This tells Hibernate to automatically update the database schema to match the entity classes whenever the SessionFactory is created.
Further Resources for Mastering Hibernate in Java
To continue your journey towards mastering Hibernate in Java, here are a few resources that you might find helpful:
- Advanced Techniques for Java Packages – Explore the usage of package-private classes in Java.
Drools Rule Engine – Learn how to use rules and actions in Drools to define and manage business logic.
Maven Central for Dependency Management – Explore the process of using publishing artifacts to Maven Central.
Hibernate Official Documentation is a comprehensive guide to all things Hibernate.
Tutorials Point’s Hibernate Tutorial has a hands-on approach, teaching practical skills to use Hibernate fluently.
Hibernate Tutorial by journaldev is a great source for comprehensive Hibernate knowledge.
Wrapping Up: Mastering Hibernate for Efficient Java Database Operations
This comprehensive guide has covered a wide range of topics related to using Hibernate in Java, a powerful tool for simplifying database operations in your applications. We’ve explored the basics and advanced usage, troubleshooting common issues, and even looked at alternative approaches.
We began with understanding the basics of Hibernate, how to set it up, and how to perform simple database operations. We then delved into more advanced features of Hibernate like Hibernate Query Language (HQL), Criteria API, and Transaction Management. These advanced features enable more complex database operations and provide a more granular control over transactions.
In the process of our exploration, we also tackled some common issues that you might encounter when using Hibernate. We discussed solutions to errors such as No CurrentSessionContext configured!
and could not initialize proxy - no Session
, which can be a great help when you’re stuck with these issues.
We also discussed alternative approaches to Hibernate, comparing it with other ORM libraries for Java like JPA, MyBatis, and JOOQ. Here’s a quick comparison of these libraries:
Library | Flexibility | Complexity | Use Case |
---|---|---|---|
Hibernate | High | Moderate | General-purpose, suitable for most applications |
JPA | Moderate | Low | When compliance with standards is important |
MyBatis | High | High | When full control over SQL queries is required |
JOOQ | High | High | When type-safe SQL querying is required |
Whether you’re just starting out with Hibernate or you’re looking to level up your database handling skills in Java, we hope this guide has given you a deeper understanding of Hibernate and its capabilities. With its balance of flexibility, power, and ease of use, Hibernate is a great tool for managing database operations in your Java applications. Happy coding!