How to Echo Newline in Bash: A Linux Shell Scripting Guide
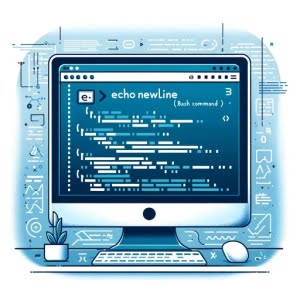
Are you finding it challenging to print newlines in Bash using the echo command? You’re not alone. Many developers find themselves puzzled when it comes to handling newlines in Bash, but we’re here to help.
Think of the echo command in Bash as a versatile printer – capable of printing not just text, but also special characters like newlines. It’s a powerful tool that can greatly simplify your scripting tasks.
In this guide, we’ll walk you through the process of using echo to print newlines in Bash, from the basics to more advanced techniques. We’ll cover everything from the fundamental use of the echo command, handling escape sequences, to dealing with common issues and their solutions.
So, let’s get started and master newline printing in Bash with echo!
TL;DR: How Do I Print a Newline in Bash Using Echo?
To print a newline in bash using the echo command, you can use the
'\n'
character in combination with the'-e'
option. For instance,echo -e 'Hello\nWorld'
would print ‘Hello’ and ‘World’ on separate lines.
Here’s a simple example:
echo -e 'Hello\nWorld'
# Output:
# Hello
# World
In this example, we use the '-e'
option with the echo command to enable interpretation of backslash escapes. The '\n'
is a special character that represents a newline. Therefore, ‘Hello\nWorld’ prints ‘Hello’ and ‘World’ on separate lines.
This is a basic way to print a newline in bash using echo, but there’s much more to learn about using echo and other commands in bash. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding Echo and Newline Basics in Bash
- Advanced Echo Techniques: Multiple Newlines and Variables
- Exploring Alternatives: The Printf Command
- Troubleshooting Echo and Newline Issues in Bash
- Understanding Echo and Escape Sequences in Bash
- The Bigger Picture: Newlines in Larger Bash Scripts
- Wrapping Up: Mastering Newlines in Bash with Echo
Understanding Echo and Newline Basics in Bash
The echo
command in Bash is a built-in function that outputs its arguments, followed by a newline. It’s a common tool used in scripting and programming for displaying messages or other data. Let’s take a closer look at how to use echo
to print newlines.
Using the ‘-e’ Option with Echo
The ‘-e’ option with the echo
command enables the interpretation of backslash escapes. Backslash escapes are sequences of characters that are interpreted to represent special characters. For instance, ‘\n’ represents a newline.
Let’s see this in action with a different example:
echo -e 'Bash\nEcho\nNewline'
# Output:
# Bash
# Echo
# Newline
In this example, we have three words: ‘Bash’, ‘Echo’, and ‘Newline’, each separated by the ‘\n’ character. When we run this command, each word is printed on a new line.
This basic use of the echo
command and the ‘-e’ option is simple, yet powerful. However, it’s important to be aware of potential pitfalls. For instance, not all versions of echo
support the ‘-e’ option. In such cases, you might need to use alternative methods to print newlines, which we’ll discuss later in this guide.
Now that you’ve got a handle on the basics, let’s dive into some more advanced uses of the echo
command in the next section.
Advanced Echo Techniques: Multiple Newlines and Variables
As you become more comfortable with the echo
command and newline character, you can start to explore more complex uses. Let’s delve into some advanced techniques, such as printing multiple newlines, combining text and newlines, and using variables.
Printing Multiple Newlines
First, let’s look at how to print multiple newlines. This can be useful in scripts where you want to create more space between lines of output for readability. To print multiple newlines, you simply include multiple ‘\n’ characters in your echo
statement.
Here’s an example:
echo -e 'Bash\n\nEcho\n\nNewline'
# Output:
# Bash
#
# Echo
#
# Newline
In this example, each word ‘Bash’, ‘Echo’, and ‘Newline’ is separated by two ‘\n’ characters, resulting in an extra line of space between each word in the output.
Combining Text and Newlines
You can also combine text and newlines in a single echo
statement. This can be useful when you want to format your output in a specific way.
Here’s how you can do it:
echo -e 'Bash Echo\nNewline'
# Output:
# Bash Echo
# Newline
In this example, ‘Bash Echo’ is printed on the same line, followed by a newline, and then ‘Newline’ is printed.
Using Variables
Lastly, you can use variables in your echo
statements. This can be useful when you have dynamic data that you want to print.
Here’s an example:
var='Bash Echo'
echo -e "$var\nNewline"
# Output:
# Bash Echo
# Newline
In this example, we first assign the string ‘Bash Echo’ to the variable var
. We then include var
in our echo
statement, followed by a newline, and then ‘Newline’. The variable var
is replaced with its value when the statement is executed, resulting in ‘Bash Echo’ being printed on the first line, followed by ‘Newline’ on the second line.
These advanced techniques open up a world of possibilities for formatting your output in Bash scripts. However, there are also alternative methods for printing newlines in Bash, which we will explore in the next section.
Exploring Alternatives: The Printf Command
While the echo
command is a powerful tool for printing newlines in Bash, it’s not the only method available. Another popular alternative is the printf
command. This command offers more control over the output format, making it a viable choice in certain situations.
Printf: An Alternative to Echo
The printf
command in Bash is similar to its counterpart in the C programming language. It’s used to format and print data to the standard output. Unlike echo
, printf
does not automatically add a newline at the end of the output, giving you more control over the format of the output.
Here’s an example of how to use printf
to print newlines:
printf 'Bash\nEcho\nNewline'
# Output:
# Bash
# Echo
# Newline
In this example, we use printf
instead of echo
to print ‘Bash’, ‘Echo’, and ‘Newline’ on separate lines. Notice that we don’t need to use the ‘-e’ option with printf
as we do with echo
. This is because printf
automatically interprets backslash escapes.
Benefits and Drawbacks of Printf
The printf
command offers several benefits over echo
. It provides more control over the output format and automatically interprets backslash escapes. This can make your scripts more readable and maintainable, especially when dealing with complex output formats.
However, printf
also has its drawbacks. It’s more complex and less intuitive than echo
, especially for beginners. Moreover, because it doesn’t automatically add a newline at the end of the output, you need to manually add ‘\n’ at the end of each string that you want to print on a separate line.
Making the Right Choice
Choosing between echo
and printf
for printing newlines in Bash depends on your specific needs. If you’re dealing with simple output formats and prefer simplicity and ease of use, echo
might be the better choice. However, if you need more control over the output format and don’t mind a bit of complexity, printf
could be the way to go.
In the next section, we’ll discuss some common issues you might encounter when using echo
to print newlines in Bash, along with their solutions.
Troubleshooting Echo and Newline Issues in Bash
While using the echo
command to print newlines in Bash is generally straightforward, you might encounter some issues along the way. Let’s look at some common problems and their solutions.
Echo Command Not Recognizing ‘\n’
One common issue is that the echo
command doesn’t recognize ‘\n’ as a newline character. This usually happens because the ‘-e’ option is not used with the echo
command.
For instance, consider the following command:
echo 'Hello\nWorld'
# Output:
# Hello\nWorld
In this example, ‘Hello\nWorld’ is printed as is, without interpreting ‘\n’ as a newline. To solve this issue, you simply need to add the ‘-e’ option to the echo
command:
echo -e 'Hello\nWorld'
# Output:
# Hello
# World
Inconsistent Behavior Across Different Systems
Another issue you might encounter is inconsistent behavior of the echo
command across different systems. This is because the behavior of echo
can vary depending on the system and the shell. For instance, some versions of echo
support the ‘-e’ option, while others don’t.
If you’re writing a script that will be run on different systems, it might be better to use printf
instead of echo
, as printf
has more consistent behavior.
Special Characters Inside Double Quotes
Finally, be aware that special characters like ‘\n’ are not interpreted inside single quotes, but are interpreted inside double quotes. For instance, consider the following command:
echo -e "Hello\nWorld"
# Output:
# Hello
# World
In this example, ‘Hello\nWorld’ is printed with a newline because the string is enclosed in double quotes.
These are some of the common issues you might encounter when using echo
to print newlines in Bash. Understanding these issues and their solutions can help you write more robust and reliable Bash scripts.
Understanding Echo and Escape Sequences in Bash
To fully grasp the concept of printing newlines in Bash using the echo
command, it’s important to understand some fundamental concepts. Let’s delve into the basics of the echo
command, escape sequences, and the difference between single and double quotes in Bash.
The Echo Command: A Closer Look
The echo
command in Bash is a powerful tool for printing text or data to the terminal. It’s commonly used in scripts and programming for outputting messages or variables. Here’s a simple example of using echo
to print a string:
echo 'Hello, World'
# Output:
# Hello, World
In this example, echo
simply prints the string ‘Hello, World’ to the terminal.
Escape Sequences: What Are They?
Escape sequences are special sequences of characters used to represent certain special characters, like newline (\n
), tab (\t
), and more. These sequences are interpreted by Bash and replaced with the special characters they represent.
Here’s an example of using echo
with an escape sequence:
echo -e 'Hello\nWorld'
# Output:
# Hello
# World
In this example, the ‘\n’ escape sequence is interpreted as a newline, so ‘Hello’ and ‘World’ are printed on separate lines.
Single vs. Double Quotes in Bash
In Bash, there’s a significant difference between single and double quotes. Inside single quotes, all special characters are preserved exactly as they are. Inside double quotes, however, certain special characters (like escape sequences and variable references) are interpreted.
For instance, consider the following command:
var='World'
echo 'Hello, $var'
echo "Hello, $var"
# Output:
# Hello, $var
# Hello, World
In this example, the variable var
is not interpreted inside single quotes, but is interpreted inside double quotes.
Understanding these fundamental concepts can help you better utilize the echo
command to print newlines and other special characters in Bash.
The Bigger Picture: Newlines in Larger Bash Scripts
Printing newlines in Bash using the echo
command might seem like a small detail, but it plays a crucial role when you’re working on larger scripts or projects. Proper use of newlines can make your scripts more readable and easier to debug, which is essential for efficient development.
String Manipulation in Bash
Beyond printing newlines, there’s a whole world of string manipulation in Bash that you can explore. For instance, you can use the echo
command along with other tools and techniques in Bash to concatenate strings, extract substrings, replace substrings, and more. Mastering these skills can greatly enhance your scripting capabilities.
Here’s an example of string manipulation in Bash, where we concatenate two strings with a newline in between:
str1='Hello'
str2='World'
echo -e "$str1\n$str2"
# Output:
# Hello
# World
In this example, we first assign the strings ‘Hello’ and ‘World’ to the variables str1
and str2
, respectively. We then use echo
with the ‘-e’ option and the ‘\n’ character to print str1
and str2
on separate lines.
Further Resources for Bash Scripting Mastery
To deepen your understanding of Bash scripting, here are some additional resources you might find helpful:
- GNU Bash Manual: This is the official manual for Bash, providing comprehensive documentation on all aspects of Bash scripting.
Advanced Bash-Scripting Guide: This guide provides in-depth coverage of advanced Bash scripting topics, including string manipulation, regular expressions, and more.
How to Echo New Line in Bash: This Linux Handbook guide explores different methods for echoing a new line in Bash scripting.
Remember, mastering Bash scripting is not an overnight process. It requires practice and patience. But with the right resources and a persistent mindset, you can become proficient in Bash scripting and take your development skills to the next level.
Wrapping Up: Mastering Newlines in Bash with Echo
In this comprehensive guide, we’ve explored the ins and outs of printing newlines in Bash using the echo
command. We’ve seen how a simple command like echo -e 'Hello\nWorld'
can print text on separate lines, making your scripts more readable and organized.
We began with the basic use of the echo
command and the ‘-e’ option, which enables the interpretation of backslash escapes like ‘\n’. We then delved into more advanced techniques, such as printing multiple newlines, combining text and newlines, and using variables in your echo
statements.
We also tackled common issues you might encounter when using echo
to print newlines, such as the echo
command not recognizing ‘\n’, inconsistent behavior across different systems, and special characters not being interpreted inside single quotes. We provided solutions to help you overcome these challenges.
We even looked at alternative approaches to printing newlines in Bash, such as using the printf
command. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Echo with ‘-e’ | Simple and easy to use | Behavior may vary across systems |
Printf | More control over output format, consistent behavior | More complex, does not automatically add newline |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to print newlines in Bash using the echo
command.
The ability to control the formatting of your output is a powerful tool in Bash scripting. Now, you’re well equipped to use the echo
command to print newlines and other special characters in your scripts. Happy scripting!