Conditions in Java: A Conditional Statements Usage Guide
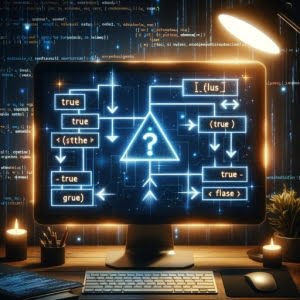
Are you finding it challenging to use conditions in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling conditions in Java, but we’re here to help.
Think of conditions in Java as the traffic lights of your code – they guide the flow of execution based on certain criteria, ensuring your program runs smoothly and efficiently.
In this guide, we’ll walk you through the process of using conditions in Java, from the basics to more advanced techniques. We’ll cover everything from simple ‘if’ statements to the more complex ‘switch’ statements, as well as alternative approaches.
Let’s get started and master conditions in Java!
TL;DR: What are Conditions in Java?
Conditions in Java are used through conditional statements such as
if, else if, else, and switch
. These statements allow your program to make decisions based on certain conditions.
Here’s a simple ‘if’ statement example:
int x = 10;
int y = 5;
if (x > y) {
System.out.println('x is greater than y');
}
// Output:
// 'x is greater than y'
In this example, we have two variables, x
and y
. The ‘if’ statement checks if x
is greater than y
. If the condition is true, it executes the code within the braces, printing ‘x is greater than y’.
This is just a basic way to use conditions in Java, but there’s much more to learn about handling conditions effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Basic Conditions in Java
- Diving Deeper: The ‘Switch’ Statement in Java
- Exploring Alternatives: The Ternary Operator in Java
- Troubleshooting Common Issues with Conditions in Java
- Fundamentals of Conditions in Programming
- Going Beyond: The Relevance of Conditions in Larger Java Projects
- Wrapping Up: Conditions in Java
Understanding Basic Conditions in Java
In Java, conditions are expressed using ‘if’, ‘else if’, and ‘else’ statements. Let’s break down each of these statements and understand how they work.
The ‘if’ Statement
The ‘if’ statement is the most basic form of a conditional statement in Java. It checks if a condition is true, and if so, it executes a block of code.
Here’s an example:
int x = 10;
if (x > 0) {
System.out.println('x is positive');
}
// Output:
// 'x is positive'
In this example, the ‘if’ statement checks if x
is greater than zero. Since x
is 10, the condition is true, so it prints ‘x is positive’.
The ‘else if’ and ‘else’ Statements
The ‘else if’ statement is used to specify a new condition if the first condition is false. The ‘else’ statement is used to specify a block of code to be executed if all conditions are false.
Here’s an example that demonstrates the use of ‘if’, ‘else if’, and ‘else’ together:
int x = 0;
if (x > 0) {
System.out.println('x is positive');
} else if (x < 0) {
System.out.println('x is negative');
} else {
System.out.println('x is zero');
}
// Output:
// 'x is zero'
In this example, the program first checks if x
is greater than zero. Since x
is 0, this condition is false, so it moves to the ‘else if’ statement. This checks if x
is less than zero. Again, this condition is false, so it finally moves to the ‘else’ statement and prints ‘x is zero’.
These statements are the building blocks of handling conditions in Java. They allow your program to make decisions and execute different blocks of code based on various conditions. However, it’s important to note that the order of these conditions matters. The program will execute the first true condition it encounters, so make sure to order your conditions appropriately.
Diving Deeper: The ‘Switch’ Statement in Java
As you progress in your Java journey, you’ll encounter situations where using multiple ‘if’ and ‘else if’ statements can become cumbersome. This is where the ‘switch’ statement comes in handy.
The ‘switch’ statement allows you to select one of many code blocks to be executed. It’s a more efficient and readable way to handle multiple conditions.
Here’s a simple example of a ‘switch’ statement:
int day = 3;
switch (day) {
case 1:
System.out.println('Monday');
break;
case 2:
System.out.println('Tuesday');
break;
case 3:
System.out.println('Wednesday');
break;
// You can add more cases here for other days
default:
System.out.println('Invalid day');
}
// Output:
// 'Wednesday'
In this example, the ‘switch’ statement checks the value of day
. Since day
is 3, it matches with case 3 and prints ‘Wednesday’. The ‘break’ keyword is used to exit the switch statement after a case is matched and executed.
The ‘default’ keyword specifies some code to run if there is no case match. In our example, if day
had a value outside the range 1-7, it would print ‘Invalid day’.
The ‘switch’ statement is particularly useful when you’re dealing with a large number of conditions. It’s cleaner and more efficient than a long series of ‘if’ and ‘else if’ statements. However, keep in mind that ‘switch’ only works with discrete values – you can’t use it with conditions that require relational operators like , or !=.
Exploring Alternatives: The Ternary Operator in Java
As you delve deeper into Java, you’ll discover that there are often multiple ways to accomplish the same task. One such alternative to the traditional ‘if’ and ‘else’ statements is the ternary operator.
The ternary operator is a shorthand way of writing simple ‘if-else’ statements. It’s called ‘ternary’ because it takes three operands: a condition, a result for the true case, and a result for the false case.
Here’s an example of how you can use the ternary operator:
int x = 10;
int y = 5;
String result = (x > y) ? 'x is greater than y' : 'x is not greater than y';
System.out.println(result);
// Output:
// 'x is greater than y'
In this example, (x > y)
is the condition, 'x is greater than y'
is the result if the condition is true, and 'x is not greater than y'
is the result if the condition is false. As x
is indeed greater than y
, the condition is true, so the output is ‘x is greater than y’.
The ternary operator can make your code more concise and easier to read when dealing with simple conditions. However, it can become confusing when dealing with more complex conditions, so use it judiciously. It’s also worth noting that unlike ‘if’ and ‘switch’ statements, the ternary operator can only handle two outcomes – one for true and one for false.
Troubleshooting Common Issues with Conditions in Java
As with any programming concept, using conditions in Java can sometimes lead to unexpected issues. Let’s take a look at some common problems you may encounter and how to solve them.
Syntax Errors
Syntax errors are the most common type of error you’ll encounter when working with conditions. These are typically caused by missing parentheses, braces, or semicolons.
For example, consider the following code:
int x = 10;
if (x > 0)
System.out.println('x is positive')
// Output:
// Error: ';' expected
In this case, the error message indicates that a semicolon is missing at the end of the print statement. Correcting the syntax solves the problem:
if (x > 0) {
System.out.println('x is positive');
}
// Output:
// 'x is positive'
Logical Errors
Logical errors occur when your code runs without crashing but doesn’t produce the expected output. This is often due to an error in the logic of your conditions.
For example, consider the following code:
int x = -10;
if (x > 0) {
System.out.println('x is positive');
} else if (x = 0) {
System.out.println('x is zero');
} else {
System.out.println('x is negative');
}
// Output:
// Error: incompatible types: int cannot be converted to boolean
In this case, the ‘else if’ condition uses a single equals sign (=) instead of a double equals sign (==
), which causes a logical error. The single equals sign is an assignment operator, not a comparison operator. Correcting the operator solves the problem:
if (x > 0) {
System.out.println('x is positive');
} else if (x == 0) {
System.out.println('x is zero');
} else {
System.out.println('x is negative');
}
// Output:
// 'x is negative'
These are just a couple of examples of issues you may encounter when using conditions in Java. Always be sure to carefully review your code for syntax and logical errors, and don’t hesitate to consult Java’s extensive documentation and community resources for help.
Fundamentals of Conditions in Programming
Before we dive deeper into conditions in Java, let’s take a step back and understand the fundamental concept of conditions in programming.
What are Conditions in Programming?
Conditions in programming are like crossroads. They guide the flow of a program, allowing it to make decisions based on certain criteria. Think of them as the ‘brain’ of a program, enabling it to think and make choices.
For example, consider a simple program that calculates the largest of two numbers. The program needs to ‘decide’ which number is larger and then print that number. This decision-making process is made possible by conditions.
The Role of Boolean Expressions
At the heart of every condition is a Boolean expression. These are expressions that evaluate to either true or false. In Java, a Boolean expression could be a simple comparison like x > y
or a more complex condition involving logical operators like &&
(and), ||
(or), and !
(not).
Here’s an example of a Boolean expression in a simple ‘if’ statement:
int x = 10;
int y = 5;
if (x > y) {
System.out.println('x is greater than y');
}
// Output:
// 'x is greater than y'
In this example, (x > y)
is a Boolean expression. It checks if x
is greater than y
. If this expression is true, the program prints ‘x is greater than y’. If it’s false, the program does nothing.
Understanding the concept of conditions and the role of Boolean expressions is crucial for working with any programming language, not just Java. They form the foundation of decision-making in code, allowing programs to behave intelligently and adapt to different situations.
Going Beyond: The Relevance of Conditions in Larger Java Projects
As you advance in your Java programming journey, you’ll find that conditions play a crucial role not just in simple programs, but also in larger, more complex projects. They are fundamental to controlling the flow of execution in your code, making them indispensable in almost every aspect of Java programming.
Conditions in Loops
In loops, conditions are used to determine when the loop should continue and when it should stop. For example, in a ‘while’ loop, the loop continues as long as the condition is true and stops once it becomes false.
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
// Output:
// 0
// 1
// 2
// 3
// 4
In this example, the loop continues to print the value of i
and increment it as long as i
is less than 5.
Conditions in Error Handling
In error handling, conditions are used to check for potential errors and handle them gracefully. This is typically done using ‘try-catch’ blocks, where the ‘try’ block contains code that might cause an error, and the ‘catch’ block contains code to handle the error.
try {
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} catch (Exception e) {
System.out.println('Something went wrong');
}
// Output:
// 'Something went wrong'
In this example, the ‘try’ block attempts to access an array element that doesn’t exist, which causes an error. The ‘catch’ block catches this error and prints ‘Something went wrong’.
Further Resources for Mastering Conditions in Java
To deepen your understanding of conditions in Java, you might find the following resources helpful:
- IOFlood’s Article on Loops in Java can help you understand loop iteration and termination conditions in Java.
Java Sleep Method – Explore the Java sleep method for pausing the execution of a thread.
Using Switch in Java – Master the switch statement for efficient handling of multiple conditions in Java programs.
Java Conditions and If Statements – A comprehensive guide to conditions and ‘if’ statements in Java.
The Java Tutorials: The switch Statement (Oracle) – An in-depth tutorial on the ‘switch’ statement.
JavaGuide on Conditions in Java is an excellent resource for understanding the concept of conditions in Java.
Wrapping Up: Conditions in Java
In this comprehensive guide, we’ve journeyed through the world of conditions in Java, from the basics to more advanced techniques.
We began with the basics, exploring how to use ‘if’, ‘else if’, and ‘else’ statements in Java. We then delved into more advanced territory, discussing the ‘switch’ statement and its advantages over multiple ‘if’ and ‘else if’ statements. We also introduced the ternary operator as an alternative to traditional ‘if’ and ‘else’ statements.
Along the way, we tackled common issues you might encounter when using conditions in Java, such as syntax and logical errors, providing solutions and tips to help you overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘if’, ‘else if’, ‘else’ Statements | Simple, easy to understand | Can become cumbersome with multiple conditions |
‘switch’ Statement | Efficient with multiple conditions, cleaner code | Only works with discrete values |
Ternary Operator | Concise, easy to read for simple conditions | Can be confusing with complex conditions, only handles two outcomes |
Whether you’re just starting out with conditions in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of conditions and their usage in Java.
Understanding and effectively using conditions is a fundamental skill in Java programming. With the knowledge you’ve gained from this guide, you’re well-equipped to handle conditions in your Java programs. Happy coding!