Thread.sleep(): Your Guide to Pausing Execution in Java
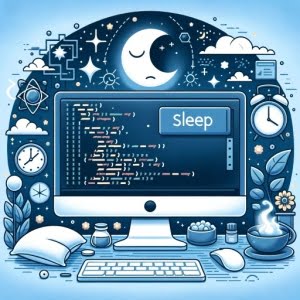
Are you finding it difficult to pause your Java program? You’re not alone. Many developers find themselves in a bind when it comes to managing the flow of their Java programs, but there’s a solution.
Like a well-timed conductor, Java’s sleep method can halt the execution of your program, providing a crucial pause. This pause can be instrumental in managing the flow of your program, especially in complex multi-threaded applications.
This guide will walk you through the ins and outs of using Java’s sleep method, from basic usage to advanced techniques. We’ll cover everything from the basics of the sleep method, its practical applications, to dealing with common issues and their solutions.
So, let’s dive in and start mastering the Java sleep method!
TL;DR: How Do I Pause or Delay Execution in Java?
To pause execution in Java, you use the
Thread.sleep()
method. This method can halt the execution of your program for a specified period of time.
Here’s a simple example:
public class Main {
public static void main(String[] args) {
try {
System.out.println("Sleeping...");
Thread.sleep(1000);
System.out.println("Awake!");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# Sleeping...
# (waits for 1 second)
# Awake!
In this example, we use Thread.sleep(1000);
to pause the program for 1000 milliseconds (or 1 second). The program first prints ‘Sleeping…’, then waits for 1 second, and finally prints ‘Awake!’.
This is a basic way to use
Thread.sleep()
in Java, but there’s much more to learn about pausing execution and managing the flow of your programs. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Exploring the Basics of Java Sleep
The Thread.sleep()
method in Java is used to pause the execution of the current thread for a specified period of time. It’s a static method of the Thread class and it takes a single argument: the length of time to sleep in milliseconds.
Here’s a simple example of how to use Thread.sleep()
:
public class BasicSleep {
public static void main(String[] args) {
try {
System.out.println("Sleep starts");
Thread.sleep(2000);
System.out.println("Sleep ends");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# Sleep starts
# (waits for 2 seconds)
# Sleep ends
In this example, the current thread sleeps for 2000 milliseconds, or 2 seconds, between printing ‘Sleep starts’ and ‘Sleep ends’.
The Thread.sleep()
method can be incredibly useful in a variety of situations. For instance, it can be used to simulate network latency in a testing environment, or to create a delay in an animation.
However, it’s important to note that while Thread.sleep()
is a simple and straightforward method to implement, it’s not always the best choice for managing the timing of your program. One of the main drawbacks of Thread.sleep()
is that it can lead to less responsive applications, especially if used excessively or with very long sleep durations. This is because while a thread is sleeping, it’s not able to do anything else, which can make your program seem unresponsive or slow.
Advanced Techniques with Java Sleep
As your Java programming skills advance, you’ll find more complex and nuanced uses for Thread.sleep()
. One such use is within loops, where you can control the execution flow of iterative operations. Another is in conjunction with other methods to manage more complex scenarios.
Let’s explore these scenarios in more detail.
Using Java Sleep within Loops
You can use Thread.sleep()
within a loop to pause the execution of each iteration. This can be useful in scenarios where you want to space out the operations being performed in the loop.
Here’s a simple example:
public class SleepInLoop {
public static void main(String[] args) {
try {
for (int i = 0; i < 5; i++) {
System.out.println("Iteration: " + i);
Thread.sleep(1000);
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# Iteration: 0
# (waits for 1 second)
# Iteration: 1
# (waits for 1 second)
# Iteration: 2
# (waits for 1 second)
# Iteration: 3
# (waits for 1 second)
# Iteration: 4
In this example, the loop iterates five times, printing the iteration number each time. After each print statement, the thread sleeps for 1 second. This creates a one-second pause between each iteration of the loop.
Java Sleep in Conjunction with Other Methods
Thread.sleep()
can also be used in conjunction with other methods to manage more complex scenarios. For instance, you might use Thread.sleep()
with System.currentTimeMillis()
to measure the actual time that your thread sleeps. This can be useful for understanding how long your thread is actually sleeping, as the sleep time can sometimes be longer than you requested due to factors like system load or thread scheduling.
Here’s an example:
public class SleepWithTimeCheck {
public static void main(String[] args) {
try {
long start = System.currentTimeMillis();
System.out.println("Sleeping...");
Thread.sleep(2000);
long end = System.currentTimeMillis();
System.out.println("Awake! Slept for " + (end - start) + " milliseconds.");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# Sleeping...
# (waits for 2 seconds)
# Awake! Slept for 2001 milliseconds.
In this example, we first record the current time in milliseconds before the sleep. After the sleep, we record the time again and subtract the start time from it to get the actual sleep time. This allows us to see the actual duration of the sleep, which in this case was 2001 milliseconds, slightly more than the 2000 milliseconds we requested.
These advanced uses of Thread.sleep()
provide more control over your Java program’s execution flow and can be incredibly powerful tools in your Java programming toolkit.
Exploring Alternatives to Java Sleep
While Thread.sleep()
is a handy tool, it’s not the only way to pause execution in Java. Other methods, like the wait()
method and the ScheduledExecutorService
, offer alternative approaches. These can be more suitable for certain scenarios and can provide more control over your program’s execution.
Using the wait()
Method
The wait()
method is part of Java’s object class and is used in multi-threaded environments to pause the execution of a thread until another thread invokes the notify()
or notifyAll()
method for the same object.
Here’s a simple example of how wait()
can be used:
public class WaitExample {
public static void main(String[] args) {
synchronized (args) {
try {
System.out.println("Waiting...");
args.wait(2000);
System.out.println("Resumed!");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
# Output:
# Waiting...
# (waits for 2 seconds)
# Resumed!
In this example, the wait(2000)
method causes the current thread to wait for 2000 milliseconds, similar to Thread.sleep(2000)
. However, unlike Thread.sleep()
, wait()
also allows other threads to interrupt the wait by calling notify()
or notifyAll()
on the same object.
Using the ScheduledExecutorService
The ScheduledExecutorService
is a more sophisticated service for scheduling tasks to run after a delay, or to execute periodically. It offers more flexibility and control compared to Thread.sleep()
.
Here’s a simple example of how to use ScheduledExecutorService
to create a delay:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ScheduledExecutorServiceExample {
public static void main(String[] args) {
ScheduledExecutorService executorService = Executors.newSingleThreadScheduledExecutor();
Runnable task = () -> {
System.out.println("Task executed");
};
System.out.println("Scheduling task to be executed after 2 seconds");
executorService.schedule(task, 2, TimeUnit.SECONDS);
executorService.shutdown();
}
}
# Output:
# Scheduling task to be executed after 2 seconds
# (waits for 2 seconds)
# Task executed
In this example, a task is scheduled to run after a delay of 2 seconds. The ScheduledExecutorService
provides more control over task scheduling and is generally more flexible than Thread.sleep()
, but it’s also more complex to use.
These alternative methods to Thread.sleep()
each have their own benefits and drawbacks. The wait()
method can be useful in multi-threaded environments, while the ScheduledExecutorService
offers more control over task scheduling. However, they’re also more complex to use than Thread.sleep()
. When choosing between these methods, consider your specific needs and the complexity of your program.
Troubleshooting Java Sleep Issues
While Thread.sleep()
is a powerful tool, it’s not without its potential pitfalls. One of the most common issues you might encounter when using Thread.sleep()
is InterruptedException
. This exception is thrown when another thread interrupts the current thread while it’s sleeping or waiting.
Handling InterruptedException
When a thread is interrupted during a sleep, it receives an InterruptedException
. This is a checked exception, meaning you need to handle it in your code. Most often, you’ll either throw the exception or handle it with a try-catch block.
Here’s an example of how to handle an InterruptedException
:
public class InterruptedExceptionExample {
public static void main(String[] args) {
try {
System.out.println("Going to sleep...");
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println("Was interrupted during sleep");
}
System.out.println("Woke up!");
}
}
# Output:
# Going to sleep...
# (if interrupted, prints: Was interrupted during sleep)
# Woke up!
In this example, if the thread is interrupted during the sleep, it catches the InterruptedException
and prints ‘Was interrupted during sleep’. If it’s not interrupted, it simply goes to sleep, wakes up after 1 second, and prints ‘Woke up!’.
Best Practices
When using Thread.sleep()
, there are a few best practices to keep in mind:
- Don’t rely on
Thread.sleep()
for precise timing. Due to factors like system load and thread scheduling,Thread.sleep()
isn’t always precisely accurate. If you need more precise timing, consider using other tools likeScheduledExecutorService
. Avoid long sleep times. Long sleep times can make your application seem unresponsive or slow. If you need to delay operations, consider using other techniques like scheduling tasks or using wait/notify.
Always handle
InterruptedException
. When a thread is interrupted during a sleep, it receives anInterruptedException
. Always handle this exception to ensure your program can deal with interruptions gracefully.
By understanding the potential issues and following these best practices, you can use Thread.sleep()
effectively and avoid common pitfalls.
Understanding Java’s Threading Model
Before diving deeper into the methods to pause execution in Java, it’s crucial to understand the underlying concept – Java’s threading model. The threading model is the foundation of how Java handles multiple threads of execution within a single program.
In Java, a thread is a lightweight process or, in other words, a unit of execution. Each Java application can have multiple threads, all executing concurrently. This concurrency is managed by the Java Virtual Machine (JVM), which schedules threads using an internal algorithm.
Pausing Execution: Why and When?
Pausing execution, as we do with Thread.sleep()
, is a way to control the flow of these concurrent threads. By pausing a thread, we can simulate delays, control the timing of certain operations, or even allow other threads to complete their tasks.
Thread Scheduling and Synchronization
Thread scheduling is the process by which the JVM determines which thread to execute at any given time. It’s a complex process that involves factors like thread priority and thread state.
Synchronization, on the other hand, is a mechanism that controls the access of multiple threads to shared resources. Without synchronization, it’s possible for one thread to modify a shared variable while another thread is in the process of using or updating that same variable. This leads to significant errors.
Here’s a simple example of synchronization in Java:
public class SynchronizedCounter {
private int c = 0;
public synchronized void increment() {
c++;
}
public synchronized void decrement() {
c--;
}
public synchronized int value() {
return c;
}
}
In this example, the increment()
, decrement()
, and value()
methods are all declared as synchronized
. This means that only one thread can access these methods at a time, preventing issues with concurrent access to the shared c
variable.
Understanding these fundamental concepts is key to mastering the use of Thread.sleep()
and other methods for pausing execution in Java.
Practical Applications of Pausing Execution in Java
Pausing execution in Java has numerous practical applications. It’s not just a theoretical concept, but a practical tool that you can use to solve real-world problems in your Java programs.
Creating Animations
One of the most common uses of pausing execution is in creating animations. By controlling the timing of your animation frames, you can create smooth and visually appealing animations. Here’s a simple example:
public class AnimationExample {
public static void main(String[] args) {
try {
for (int i = 0; i < 10; i++) {
System.out.println("Frame " + i);
Thread.sleep(500);
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# Frame 0
# (waits for 0.5 seconds)
# Frame 1
# (waits for 0.5 seconds)
# Frame 2
# ...
# Frame 9
In this example, we simulate an animation with 10 frames. Each frame is displayed for 0.5 seconds, creating a smooth animation effect.
Simulating Time-Consuming Tasks
Another practical application of pausing execution is to simulate time-consuming tasks. This can be especially useful in testing environments, where you might need to simulate a network delay or a long-running computation.
public class SimulationExample {
public static void main(String[] args) {
try {
System.out.println("Starting long-running task...");
Thread.sleep(5000);
System.out.println("Task completed!");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# Starting long-running task...
# (waits for 5 seconds)
# Task completed!
In this example, we simulate a long-running task by sleeping for 5 seconds. This can be useful for testing how your program handles delays or long-running operations.
Further Resources for Mastering Java Sleep
To continue your journey in mastering Java’s sleep method and other concurrency utilities, check out these resources:
- Java Loops Tutorial: Getting Started – Learn about the do-while loop and its application in Java.
Java Recursion Basics – Learn about base cases, recursive cases, and termination conditions.
Using Conditions in Java – Master handling various conditions effectively in Java programming.
Oracle’s Java Tutorials: Concurrency – Delve into Oracle’s guide on managing simultaneous operations in Java.
Guide to the Java ExecutorService – Uncover how to optimize thread execution in Java with Baeldung’s guide.
Understanding Java Threads – Master the operation and application of threads in Java with this tutorial from JavaWorld.
These resources provide in-depth discussions and tutorials on Java’s concurrency utilities, including Thread.sleep()
, and can help you deepen your understanding and improve your skills.
Wrapping Up: Mastering Java Sleep for Pausing Execution
In this comprehensive guide, we’ve explored the nuances of Java’s sleep method, a powerful tool for pausing the execution of your Java programs.
We began with the basics, showing you how to use Thread.sleep()
to create a simple pause in your program’s execution. We then delved into more complex uses, such as using Thread.sleep()
within loops and in conjunction with other methods to manage more intricate scenarios.
Along the way, we tackled common challenges you might face when using Thread.sleep()
, like handling InterruptedException
, and provided solutions to help you navigate these issues. We also ventured into alternatives to Thread.sleep()
, such as the wait()
method and the ScheduledExecutorService
, and discussed when these might be more appropriate tools for pausing execution.
Method | Use Case | Complexity |
---|---|---|
Thread.sleep() | Simple delays, testing environments | Low |
wait() | Multi-threaded environments, waiting for specific conditions | Moderate |
ScheduledExecutorService | Precise scheduling, recurring tasks | High |
Whether you’re just starting out with Java or you’re a seasoned developer looking to better manage the flow of your programs, we hope this guide has given you a deeper understanding of how to pause execution in Java using Thread.sleep()
and its alternatives.
With the ability to control the timing and flow of your program, you’re well equipped to handle a wide variety of programming scenarios. Happy coding!