Learn Python: Print to stderr Guide (With Examples)
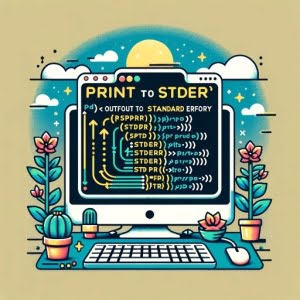
Ever wondered how to handle error messages in Python? Like a traffic cop directing cars, Python can direct your print statements to different outputs, including stderr.
Whether you’re a beginner just starting out or an intermediate looking to level up your skills, this guide will provide you with the knowledge you need to effectively print to stderr in Python.
TL;DR: How Do I Print to stderr in Python?
To print to stderr in Python, you can use the
print()
function with thefile
parameter set tosys.stderr
, like this:print('This is an error message', file=sys.stderr)
.
Here’s a simple example:
import sys
print('This is an error message', file=sys.stderr)
# Output:
# This is an error message
In this example, we import the sys
module and use the print()
function to print an error message. The file=sys.stderr
parameter directs the output to stderr instead of the default stdout.
This is a basic way to print to stderr in Python, but there’s much more to learn about handling error messages in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Use of Python Print to stderr
- Redirecting stderr to a File in Python
- Alternative Methods: Using the Logging Module
- Troubleshooting Common Issues with Python stderr
- Understanding stderr and Its Role in Python
- Exploring stderr in Larger Python Applications
- Further Learning and Related Topics
- Wrapping Up: Python Print to stderr
Basic Use of Python Print to stderr
In Python, the print()
function is typically used to output messages to the console. By default, these messages are sent to stdout (standard output). However, we can redirect these messages to stderr (standard error) by using the file
parameter in the print()
function. Here’s how you can do it:
import sys
print('This is an error message', file=sys.stderr)
# Output:
# This is an error message
In this example, we first import the sys
module, which provides access to some variables used or maintained by the Python interpreter. We then use the print()
function to print an error message. By setting the file
parameter to sys.stderr
, we direct the output to stderr instead of the default stdout.
The advantage of this method is its simplicity and directness. It is straightforward and easy to understand, making it perfect for beginners. However, it’s important to note that this method only works for printing individual error messages. For more complex scenarios, such as redirecting all error messages to stderr, you might need to use more advanced techniques, which we will discuss in the next sections.
Redirecting stderr to a File in Python
As you become more proficient in Python, you might come across scenarios where you need to redirect stderr to a file or another output. This is particularly useful when you have a program that generates a lot of error messages and you want to keep a record of these messages for later analysis. Here’s how you can do it:
import sys
sys.stderr = open('err.txt', 'w')
print('This is an error message', file=sys.stderr)
# No output on console. Error message is written to err.txt
In this example, we first open a file named ‘err.txt’ in write mode. We then assign this file to sys.stderr
. This means that all subsequent error messages will be written to ‘err.txt’ instead of being printed on the console. Note that the file ‘err.txt’ will be created in the same directory as your Python script. If the file already exists, its contents will be overwritten.
This method provides a way to handle large volumes of error messages. However, be aware that once you redirect stderr to a file, you need to reset it back to the console if you want subsequent error messages to be printed on the console. You can do this by calling sys.stderr = sys.__stderr__
.
Alternative Methods: Using the Logging Module
While using the print()
function with sys.stderr
is a straightforward way to print to stderr in Python, it’s not the only method. The Python logging
module offers a more robust solution, particularly for larger applications or scripts.
The logging
module provides a flexible framework for emitting log messages from Python programs. It’s part of the standard Python library, and requires no additional installation. Here’s how you can use it to print to stderr:
import logging
# Create a logger object
logger = logging.getLogger()
# Set the level to print all messages
logger.setLevel(logging.DEBUG)
# Create a stream handler for stderr
handler = logging.StreamHandler(sys.stderr)
# Add the handler to the logger
logger.addHandler(handler)
# Log an error message
logger.error('This is an error message')
# Output:
# This is an error message
In this example, we first import the logging
module and create a logger object. We set the logger’s level to DEBUG
, which means that it will print all messages, including debug, info, warning, error, and critical messages. We then create a stream handler for stderr and add it to the logger. Finally, we use the logger.error()
method to log an error message, which is printed to stderr.
Compared to the print()
function, the logging
module provides more control over message formatting and output routing. It also supports a hierarchy of loggers and multiple handlers per logger, making it a powerful tool for more complex logging needs.
Troubleshooting Common Issues with Python stderr
While printing to stderr in Python is generally straightforward, you may encounter some common issues, particularly when dealing with buffering. Buffering can cause your error messages to appear out of order or not at all, especially when you’re writing to a file. Let’s discuss some of these issues and their solutions.
Dealing with Buffering Issues
By default, Python buffers stdout and stderr. This means that it collects some data before it writes it out as a performance optimization. However, this can cause issues when you’re trying to debug your program in real-time. You can force Python to flush the buffer by using the flush=True
parameter in the print()
function. Here’s an example:
import sys
print('This is an error message', file=sys.stderr, flush=True)
# Output:
# This is an error message
In this example, the flush=True
parameter forces Python to flush the buffer and print the error message immediately. This can be useful when you’re trying to track down a bug and you need to see the error messages in real-time.
Redirecting stderr to stdout
Sometimes, you might want to redirect stderr to stdout, so that all output and error messages are intermixed. This can be useful when you’re logging the output of your program and you want to keep everything in one place. Here’s how you can do it:
import sys
sys.stderr = sys.stdout
print('This is an error message', file=sys.stderr)
# Output:
# This is an error message
In this example, we redirect stderr to stdout by assigning sys.stdout
to sys.stderr
. This means that all subsequent error messages will be printed to stdout instead of stderr.
Remember, while these solutions can help you troubleshoot common issues, they are not without their own pitfalls. For example, forcing Python to flush the buffer can slow down your program, especially if you’re writing a lot of data. Similarly, redirecting stderr to stdout can make it harder to separate out error messages from regular output. Therefore, it’s important to understand your program’s needs and use these solutions judiciously.
Understanding stderr and Its Role in Python
Before diving into how to print to stderr in Python, it’s important to understand what stderr is and why it’s used in programming. Standard error, or stderr, is one of the three standard streams in computer programming. The other two are standard input (stdin) and standard output (stdout).
The Role of stderr
Stderr is specifically designed for error messages. It’s a type of output stream where a program writes its error messages, separate from the standard output stream (stdout) which is used for regular output. This separation is crucial because it allows error messages to be redirected and handled differently from regular output.
# A simple Python program demonstrating stdout and stderr
import sys
print('This is regular output', file=sys.stdout)
print('This is an error message', file=sys.stderr)
# Output:
# This is regular output
# This is an error message
In this example, the first print()
function writes a message to stdout, which is the default output stream. The second print()
function writes an error message to stderr, demonstrating the separation between the two streams.
The Difference Between stdout and stderr
While both stdout and stderr are output streams, they serve different purposes. Stdout is used for regular program output, while stderr is used for error messages. This separation allows programmers to redirect or suppress error messages separately from regular output, which can be particularly useful when debugging or logging program activity.
Exploring stderr in Larger Python Applications
Printing to stderr is not just a tool for simple scripts or basic debugging. In larger Python applications or complex scripts, stderr plays a crucial role in managing and tracking error messages. It’s a fundamental aspect of error handling and logging, two key concepts in Python and programming at large.
Error Handling and Logging in Python
In more complex Python applications, error handling becomes increasingly important. Errors are inevitable in any program, and how you handle them can greatly impact your program’s robustness and reliability. Printing to stderr allows you to separate error messages from regular output, making it easier to spot and address issues.
Logging, on the other hand, is the process of recording program events for later analysis. It’s a crucial tool for debugging, monitoring, and understanding program behavior. By printing to stderr, you can direct all error messages to a specific log file, making it easier to track and review errors.
# Using logging module to log error messages to a file
import logging
# Create a logger
logger = logging.getLogger()
# Set the level to print all messages
logger.setLevel(logging.DEBUG)
# Create a file handler for stderr
handler = logging.FileHandler('error.log')
# Add the handler to the logger
logger.addHandler(handler)
# Log an error message
logger.error('This is an error message')
# No output on console. Error message is written to error.log
In this example, we use the logging
module to log error messages to a file named ‘error.log’. This is particularly useful in larger applications where you might have hundreds or even thousands of error messages. By logging these messages to a file, you can review them at your leisure and have a permanent record of what went wrong.
Further Learning and Related Topics
While this guide has provided you with the basics of printing to stderr in Python, there’s much more to learn. As you continue your Python journey, consider exploring the following guides:
- Python Print Mastery: Step-by-Step – Learn how to print formatted tables and structured data using the print() function.
Python Uppercasing – Explore Python string manipulation techniques for case conversion using the upper() method.
Print List in Python – Learn how to print lists in Python for displaying data structures in a readable format.
Python’s Pretty Print Detailed Documentation – An in-depth exploration of Python’s pprint module.
Python Operator in Print Statement – Get insightful StackOverflow community answers on the use of Python operators in print statements.
Python’s Official Tutorial on Input and Output -Python’s official guide on handling user input and output.
Wrapping Up: Python Print to stderr
In this comprehensive guide, we’ve journeyed through the world of Python’s stderr, exploring its role, how to print to stderr, and how to handle common issues. We started with the basics, using the print()
function with the file
parameter set to sys.stderr
. This simple yet effective method is great for beginners and handles individual error messages well.
As we progressed, we delved into more advanced techniques like redirecting stderr to a file or another output, a useful strategy for handling larger volumes of error messages. We also explored alternative methods such as using the more robust logging
module, offering more control over message formatting and output routing.
We also discussed common issues like dealing with buffering and redirecting stderr to stdout, providing practical solutions for these challenges. Along the way, we emphasized the importance of understanding the role of stderr and its difference from stdout, crucial for effective error handling and logging in Python.
Here’s a brief comparison of the different methods discussed:
Method | Complexity | Use Case |
---|---|---|
print() function | Basic | Printing individual error messages |
Redirecting stderr to a file | Intermediate | Handling large volumes of error messages |
Using the logging module | Advanced | Larger applications or complex scripts |
Remember, mastering python print to stderr
is not just about learning different methods, but understanding when and how to use them. As you continue your Python journey, don’t be afraid to experiment with these techniques and explore beyond what we’ve covered in this guide.