Learn Python: How to Print a List
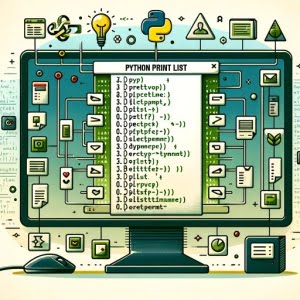
Have you ever found yourself scratching your head over how to print lists in Python? You’re not alone. Lists are a fundamental part of Python, and understanding how to manipulate and display them is a crucial skill for any Pythonista.
But don’t worry! Python is like a skilled printer, ready and waiting to format and print your lists in a multitude of ways.
In this comprehensive guide, we’ll walk you through the process of printing lists in Python. From the basic print()
function to complex formatting, you’ll learn how to display Python lists like a pro. So let’s dive in and start exploring the world of Python list printing!
TL;DR: How Do I Print a List in Python?
You can print a list in Python using the
print()
function. Here’s a simple example:
my_list = [1, 2, 3]
print(my_list)
# Output:
# [1, 2, 3]
This code creates a list called my_list
with the elements 1, 2, and 3. The print()
function then displays the entire list exactly as it was created. It’s a quick and easy way to print a list in Python, but there’s so much more you can do with lists and the print()
function.
So, if you’re interested in mastering the art of printing lists in Python, keep reading for more detailed explanations and advanced usage scenarios.
Table of Contents
Python’s print()
Function: A Beginner’s Guide
The print()
function is Python’s built-in solution for displaying output to the console. It’s simple, versatile, and widely used in Python programming. When it comes to printing lists, print()
is the go-to function for beginners.
Here’s a basic example of how to use the print()
function to display a list:
fruits = ['apple', 'banana', 'cherry']
print(fruits)
# Output:
# ['apple', 'banana', 'cherry']
In this example, we’ve created a list called fruits
containing three string elements. The print()
function then simply prints out the entire list, including the square brackets and the quotes around the string elements.
The print()
function is straightforward and easy to use, making it great for beginners. However, it’s not without its limitations. For example, it doesn’t offer much flexibility in terms of formatting.
As you can see, the output includes the square brackets and quotes, which may not always be desirable. But don’t worry, there are ways around this, which we’ll explore in the intermediate and expert sections.
Advanced List Printing Techniques in Python
Now that you have a handle on the basics, let’s dive into some more advanced techniques for printing lists in Python. We’ll explore methods like using loops, list comprehension, and the join()
function.
Using Loops to Print Lists
A common way to print lists in Python is by using loops. This allows you to iterate over each element in the list and print it individually. Here’s how you can do it:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# apple
# banana
# cherry
In this example, the for
loop iterates over each element in the fruits
list and the print()
function prints each element on a new line. This method provides more control over the output format compared to the basic print()
function.
List Comprehension for List Printing
List comprehension is a powerful feature in Python that allows you to create and manipulate lists in a single line of code. You can also use it to print lists:
fruits = ['apple', 'banana', 'cherry']
[print(fruit) for fruit in fruits]
# Output:
# apple
# banana
# cherry
This does the same thing as the previous example, but in a more concise way. However, it may be less readable for beginners or those unfamiliar with list comprehension.
The join()
Function for List Printing
The join()
function is a string method that allows you to join the elements of a list into a single string with a specified delimiter. Here’s how you can use it to print a list:
fruits = ['apple', 'banana', 'cherry']
print(', '.join(fruits))
# Output:
# apple, banana, cherry
In this example, the join()
function joins the elements of the fruits
list into a single string, with each element separated by a comma and a space. This method offers a great deal of flexibility in terms of output format.
Each of these methods has its pros and cons. Using loops provides more control over the output format, but can be verbose for large lists. List comprehension is concise but may be less readable for beginners. The
join()
function offers great flexibility in output format, but it only works with lists of strings.
Expert-Level List Printing Techniques in Python
As you gain more experience in Python, you’ll discover there are numerous ways to print lists beyond the standard print()
, loops, and join()
methods. In this section, we’ll explore some of these alternative approaches, including list methods and third-party libraries.
List Methods for Advanced List Printing
Python’s list methods provide a variety of ways to manipulate and display lists. For example, the enumerate()
function can be used to print both the index and the value of each element in a list:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(f'{i}: {fruit}')
# Output:
# 0: apple
# 1: banana
# 2: cherry
In this example, the enumerate()
function adds a counter to the fruits
list and returns it as an enumerate object. The for
loop then iterates over this object, and the print()
function prints both the index and the value of each element.
Third-Party Libraries for List Printing
There are also many third-party libraries available in Python that can enhance your list printing capabilities. One such library is pprint
(pretty print), which provides more human-friendly printing of lists, especially for nested lists:
from pprint import pprint
nested_list = [['apple', 'banana'], ['cherry', 'date']]
pprint(nested_list, indent=2)
# Output:
# [ ['apple', 'banana'],
# ['cherry', 'date']]
In this example, the pprint()
function from the pprint
library is used to print a nested list with a specified indentation level. This can make the output much easier to read, especially for large or complex lists.
These alternative methods offer more advanced and flexible ways to print lists in Python. However, they may also be more complex and less intuitive for beginners. It’s important to choose the method that best fits your specific needs and level of experience.
Troubleshooting Common Issues
As with any programming task, printing lists in Python can sometimes lead to unexpected errors or issues. In this section, we’ll discuss some of the most common problems you might encounter, along with their solutions.
Handling ‘TypeError’ When Printing Lists
One common issue is the ‘TypeError’ that occurs when you try to print a list with non-string elements using the join()
method. Remember, join()
only works with lists of strings. Here’s an example:
my_list = [1, 2, 3]
print(', '.join(my_list))
# Output:
# TypeError: sequence item 0: expected str instance, int found
In this case, the join()
method tries to concatenate an integer to a string, which results in a ‘TypeError’. The solution is to convert the integers to strings before using join()
, like this:
my_list = [1, 2, 3]
print(', '.join(map(str, my_list)))
# Output:
# 1, 2, 3
In this corrected example, the map()
function is used to convert each integer in the list to a string before the join()
method is applied.
Dealing with Nested Lists
Another common issue involves printing nested lists. The standard print()
function will simply print the nested list as it is, which might not be the desired output. For instance:
nested_list = [['apple', 'banana'], ['cherry', 'date']]
print(nested_list)
# Output:
# [['apple', 'banana'], ['cherry', 'date']]
To print each sublist on a new line, you can use a for
loop:
nested_list = [['apple', 'banana'], ['cherry', 'date']]
for sublist in nested_list:
print(sublist)
# Output:
# ['apple', 'banana']
# ['cherry', 'date']
In this adjusted example, the for
loop iterates over each sublist in nested_list
, and the print()
function prints each sublist on a new line.
Understanding Python Lists and print()
To truly master the art of printing lists in Python, it’s essential to understand the fundamentals of Python lists and the print()
function. Let’s take a deep dive into these topics.
Python Lists: A Quick Recap
In Python, a list is a data type that holds an ordered collection of items, which can be of any type. Lists are created by placing a comma-separated sequence of items inside square brackets []
.
# Creating a list
fruits = ['apple', 'banana', 'cherry']
In this example, fruits
is a list that contains three strings. Lists are versatile and can contain any mix of data types, including numbers, strings, and even other lists (known as nested lists).
The print()
Function: Python’s Output Maestro
The print()
function in Python sends output to the console. It’s one of the most commonly used functions in Python, especially for beginners learning the language.
# Using the print() function
print('Hello, World!')
# Output:
# Hello, World!
In this example, the print()
function outputs the string ‘Hello, World!’ to the console. When used with a list, the print()
function displays the entire list as it is, including the square brackets and commas.
List Methods and Loops
Python provides a variety of list methods that allow you to manipulate and work with lists. For instance, the append()
method lets you add an item to the end of a list, and the remove()
method lets you remove a specific item from a list.
Loops, on the other hand, are control flow structures that let you repeat a block of code multiple times. In the context of lists, loops are often used to iterate over each element in a list.
Exploring Related Concepts
Mastering list printing is a stepping stone to exploring more advanced concepts in Python. For instance, you might want to delve deeper into list manipulation techniques, such as list slicing and list comprehension. Understanding data structures, such as tuples and dictionaries, is also beneficial.
Further Learning Resources
There’s always more to learn in Python. Here are a few resources that can help you deepen your understanding of list printing and related topics:
- IOFlood’s Python Print Article – Learn how to print formatted text and variables using the print() function in Python.
Find in Python – Discover how to use the find() method in Python for locating substrings within strings.
Python STDERR Printing – Learn Python techniques for directing output to stderr instead of stdout using the print() function.
Medium’s 5 Different Ways to Print in Python – Discover multiple ways to print and format output in Python.
Official Python Documentation – Utilize the official guide to explore Python’s diverse features and capabilities.
Codecademy’s Python Course – Embark on an interactive learning journey with Codecademy’s Python course.
Wrapping Up
We’ve journeyed through the world of printing lists in Python, starting from the basic print()
function to more advanced and alternative techniques. We’ve seen how Python, like a skilled printer, offers numerous ways to format and display lists.
We started with the simple print()
function, which is Python’s built-in solution for displaying output, including lists. We then explored more advanced techniques, such as using loops, list comprehension, and the join()
function to print lists.
We also discussed common issues you might encounter when printing lists in Python, such as ‘TypeError’ and problems with nested lists, along with their solutions. Furthermore, we delved into the fundamentals of Python lists and the print()
function to better understand the concepts underlying the printing of lists.
In conclusion, Python offers a rich set of tools for printing lists, each with its strengths and weaknesses. The key is to understand these tools and choose the one that best fits your specific needs and level of experience. Keep practicing, keep exploring, and happy coding!