Python Find Function: Simple String Searching
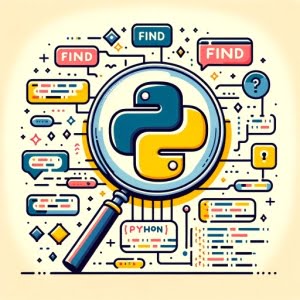
Ever felt like you’re lost in a sea of text, struggling to locate a specific substring within a string in Python? You’re not alone. Many developers find themselves in this predicament. Consider Python’s find function as your trusty compass, guiding you to the exact location of your sought-after substring.
Whether you’re parsing logs, extracting specific data, or simply searching within strings, understanding how to use the find function in Python can significantly enhance your coding efficiency.
In this guide, we’ll navigate through the ins and outs of the Python find function, from its basic usage to advanced techniques. We’ll cover everything from the find()
function’s syntax, its parameters, as well as its return values.
Let’s embark on this journey to master Python’s find function!
TL;DR: How Do I Use the Find Function in Python?
The find function in Python is used to locate the position of a substring within a string, for example with the command
str.find('World')
. If the substring “World” is found in thestr
string,find
returns the lowest index where the substring starts. If not found, it returns -1.
Here’s a simple example:
str = 'Hello, World!'
pos = str.find('World')
print(pos)
# Output:
# 7
In this example, we have a string ‘Hello, World!’ and we’re using the find()
function to locate the substring ‘World’. The function returns 7, which is the starting index of ‘World’ in the string.
This is just a basic illustration of the find function in Python. There’s a lot more to learn about it, including handling different parameters and alternative approaches. Continue reading for a more detailed exploration and advanced usage scenarios.
Table of Contents
Python Find Function: Basic Usage
The find function in Python is primarily used to locate the position of a substring within a string. It’s a built-in function of the string data type, which means you can use it directly on any string object.
The syntax of the find function is as follows:
str.find(sub[, start[, end]])
Here, str
is the string you want to search in, sub
is the substring you’re looking for, and start
and end
are optional parameters to specify the range in which you want to search.
Let’s look at a basic example to understand how the Python find function works:
str = 'Hello, Python learners!'
pos = str.find('Python')
print(pos)
# Output:
# 7
In this example, we’re searching for the substring ‘Python’ in the string ‘Hello, Python learners!’. The find()
function returns 7, which is the starting index of ‘Python’ in the string.
One of the key advantages of the find function is its simplicity and directness. It’s a straightforward way to locate substrings without the need for complex code.
However, it’s important to note that the find function is case-sensitive. This means that ‘Python’ and ‘python’ are considered different substrings. If the exact case doesn’t match, the function will return -1, indicating that the substring was not found.
str = 'Hello, Python learners!'
pos = str.find('python')
print(pos)
# Output:
# -1
In this example, we’re searching for ‘python’ (lowercase) in the string ‘Hello, Python learners!’ (where ‘Python’ is capitalized). The function returns -1, indicating that ‘python’ was not found.
Advanced Use of Python’s Find Function
Beyond its basic usage, the Python find function comes with additional parameters to refine your search. Specifically, these are the start
and end
parameters.
With these parameters, you can specify the range within which you want to search for the substring. This can be particularly useful when you’re dealing with large strings or when you want to limit your search to a specific section of the string.
Here’s how you can use these parameters:
str = 'Hello, Python learners! Welcome to Python programming.'
pos = str.find('Python', 10, 30)
print(pos)
# Output:
# 19
In this example, we’re searching for ‘Python’ in the string ‘Hello, Python learners! Welcome to Python programming.’, but this time we’ve specified a range from index 10 to 30. The find()
function returns 19, which is the starting index of the second occurrence of ‘Python’ in the string within the specified range.
If we hadn’t specified the range, the function would have returned 7, which is the index of the first occurrence of ‘Python’. By using the start
and end
parameters, we were able to skip the first occurrence and find the next one within the given range.
This demonstrates the power and flexibility of the find function in Python. By understanding and leveraging these additional parameters, you can make your substring searches more efficient and targeted.
Alternative Methods for Substring Search in Python
While the find function is a powerful tool for locating substrings in Python, it’s not the only method available. Depending on your specific needs, you might find other methods like the index()
function, regular expressions, or certain third-party libraries more suitable. Let’s explore these alternatives.
The Index Function
The index()
function works similarly to the find()
function, but with a key difference: if the substring is not found, index()
raises an exception, whereas find()
simply returns -1.
Here’s an example of using the index()
function:
str = 'Hello, Python learners!'
try:
pos = str.index('Python')
print(pos)
except ValueError:
print('Substring not found')
# Output:
# 7
In this example, we’re using a try/except block to handle the potential ValueError
exception raised by the index()
function when the substring is not found.
Regular Expressions
For more complex search patterns, regular expressions (regex) can be a powerful tool. Python’s re
module provides functions to work with regex, including the search()
function to locate substrings.
Here’s an example:
import re
str = 'Hello, Python learners!'
match = re.search('Python', str)
if match:
print('Substring found at index:', match.start())
else:
print('Substring not found')
# Output:
# Substring found at index: 7
In this example, we’re using the re.search()
function to locate the substring ‘Python’. If the substring is found, the function returns a match object, from which we can get the starting index of the substring using the start()
method.
Third-Party Libraries
There are also third-party libraries available that provide additional functionality for string searching, like fuzzy string matching. One such library is fuzzywuzzy
.
Here’s a simple example of using fuzzywuzzy
to find a substring:
from fuzzywuzzy import fuzz
str = 'Hello, Python learners!'
match_ratio = fuzz.partial_ratio('Python', str)
print(match_ratio)
# Output:
# 100
In this example, we’re using the fuzzywuzzy.fuzz.partial_ratio()
function to compare the similarity between the substring ‘Python’ and the string ‘Hello, Python learners!’. The function returns a ratio from 0 to 100, with 100 indicating a perfect match.
Each of these methods has its own advantages and disadvantages. The find()
and index()
functions are simple and straightforward, but they might not be suitable for complex search patterns. Regular expressions offer more flexibility, but they can be more complex to use. Third-party libraries like fuzzywuzzy
provide additional features, but they require an external dependency.
It’s important to understand these differences and choose the method that best suits your needs.
Troubleshooting Python’s Find Function
While the find function is a powerful tool, it’s not without its quirks. Here are some common issues you might encounter when using it, along with their solutions.
Handling the Case When the Substring Is Not Found
One of the most common scenarios you’ll encounter is when the substring you’re looking for is not found in the string. In such cases, the find function returns -1. It’s essential to handle this case correctly in your code to avoid confusion with actual index positions.
Here’s an example of how you can handle this:
str = 'Hello, Python learners!'
pos = str.find('Java')
if pos == -1:
print('Substring not found')
else:
print('Substring found at index:', pos)
# Output:
# Substring not found
In this example, we’re searching for ‘Java’ in the string ‘Hello, Python learners!’. Since ‘Java’ is not found in the string, the find()
function returns -1. We check for this value and print a suitable message.
Case-Sensitive Search
The find function is case-sensitive, which means it treats ‘Python’ and ‘python’ as different substrings. If you want to perform a case-insensitive search, you can convert both the string and the substring to the same case (either lower or upper) before performing the search.
Here’s how you can do it:
str = 'Hello, Python learners!'
pos = str.lower().find('python')
if pos == -1:
print('Substring not found')
else:
print('Substring found at index:', pos)
# Output:
# Substring found at index: 7
In this example, we’re converting both the string ‘Hello, Python learners!’ and the substring ‘python’ to lowercase before performing the search. This way, the search becomes case-insensitive, and ‘Python’ in the string matches ‘python’ in the substring.
Understanding these common issues and their solutions can help you use the Python find function more effectively and avoid potential pitfalls.
Understanding Python Strings and Indexing
To fully grasp the workings of Python’s find function, it’s crucial to understand Python’s string data type and how indexing works in it.
Python Strings
In Python, a string is a sequence of characters. It is an immutable data type, which means once a string is created, it cannot be changed. However, you can retrieve parts of the string, concatenate it with other strings, or replicate it.
Here’s a simple example of a string in Python:
str = 'Hello, Python learners!'
print(str)
# Output:
# 'Hello, Python learners!'
In this example, ‘Hello, Python learners!’ is a string. We can perform various operations on this string using Python’s built-in string functions.
String Indexing
Every character in a Python string has a position, known as its index. Python uses zero-based indexing, which means the index of the first character is 0, the second character is 1, and so on.
Here’s an illustration of how indexing works in Python strings:
str = 'Python'
print(str[0]) # Output: 'P'
print(str[3]) # Output: 'h'
In this example, ‘Python’ is a string. We’re retrieving the characters at indices 0 and 3 using the square bracket notation. The output is ‘P’ and ‘h’, respectively.
Understanding Python strings and indexing is fundamental to mastering the find function, as it underpins how the function locates substrings within a string.
Expanding Your Skills Beyond Python’s Find Function
The find function is a fundamental tool in Python’s string manipulation arsenal. Its relevance extends beyond simple searches, playing a crucial role in text processing, data extraction, and more. Mastering it can significantly enhance your proficiency in Python.
Exploring Related Concepts
While the find function is undoubtedly powerful, it’s just the tip of the iceberg when it comes to string manipulation in Python. There are other related concepts and techniques that you might find useful.
For instance, regular expressions, also known as regex, offer a more flexible and powerful way to match patterns in strings. They can be a bit complex to understand at first, but once you get the hang of them, they can be incredibly useful.
Another important concept is string manipulation. Python provides a wealth of built-in functions and methods for manipulating strings, such as replace()
, split()
, join()
, and many more. These functions can help you transform and process strings in various ways.
Further Resources for Mastering Python’s Find Function
To deepen your understanding of Python’s find function and related concepts, here are some external resources that you might find helpful:
- IOFlood’s Python Print Guide explains printing data structures like lists, dictionaries, and tuples with the print() function.
Printing Lists in Python – Master techniques for printing lists in Python to visualize data effectively.
Quick Guide: Printing Without Newline – Master Python print() techniques for precise control over output behavior.
Python’s Official Documentation on String Methods provides a detailed explanation of each string method along with examples.
Real Python’s Guide on Regular Expressions provides an introduction to regular expressions in Python, including the
re
module.PythonForBeginners’ Tutorial on String Manipulation covers the basics of string manipulation in Python.
Remember, mastering any programming concept takes practice. Don’t be afraid to experiment, make mistakes, and learn from them. Happy coding!
Wrapping Up: Mastering Python’s Find Function
In this comprehensive guide, we’ve navigated through the intricacies of Python’s find function. From understanding its basic usage to exploring advanced techniques, we’ve covered the full spectrum of this powerful function.
We began with the basics, learning how to use the find function to locate substrings within a string. We then delved into more advanced territory, exploring how to use the start
and end
parameters to refine our search. We also discussed common issues you might encounter when using the find function, such as handling the case when the substring is not found and performing case-insensitive searches, providing solutions for each issue.
We also explored alternative approaches to substring location, such as the index()
function, regular expressions, and third-party libraries like fuzzywuzzy
. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
find() | Simple, returns -1 if not found | Case-sensitive |
index() | Similar to find(), but raises an exception if not found | Raises exception if not found |
Regular expressions | Flexible, powerful for complex patterns | More complex to use |
Third-party libraries | Additional features, such as fuzzy matching | Requires external dependency |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python’s find function and its capabilities.
With its balance of simplicity, flexibility, and power, the find function is a vital tool for any Python developer. Happy coding!