Python Update Dictionary: Methods and Usage Guide
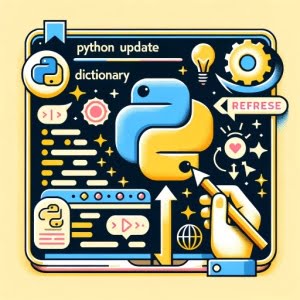
Are you finding it challenging to update dictionaries in Python? You’re not alone. Many developers find themselves puzzled when it comes to handling this task, but we’re here to help.
Think of Python’s dictionaries as a well-organized bookshelf – allowing us to store and manage data in an efficient and accessible manner. Python dictionaries, like a well-organized bookshelf, can be easily updated and managed.
In this guide, we’ll walk you through the process of updating dictionaries in Python, from the basics to more advanced techniques. We’ll cover everything from the simple update()
method to more complex techniques like dictionary comprehension and merging dictionaries, as well as alternative approaches.
Let’s dive in and start mastering Python dictionaries!
TL;DR: How Do I Update a Dictionary in Python?
To update a dictionary in Python, you can use the
update()
method, likedict1.update({'b': 3, 'c': 4})
. This method allows you to add new items or change the value of existing items in a Python dictionary.
Here’s a simple example:
dict1 = {'a': 1, 'b': 2}
dict1.update({'b': 3, 'c': 4})
print(dict1)
# Output:
# {'a': 1, 'b': 3, 'c': 4}
In this example, we have a dictionary dict1
with keys ‘a’ and ‘b’. We use the update()
method to change the value of ‘b’ and add a new key-value pair ‘c’: 4. The updated dictionary now includes ‘a’: 1, ‘b’: 3, and ‘c’: 4.
This is a basic way to update a dictionary in Python, but there’s much more to learn about handling dictionaries. Continue reading for a more detailed explanation and additional methods.
Table of Contents
- Understanding Python’s update() Method
- Advanced Techniques for Updating Dictionaries in Python
- Exploring Alternative Methods for Updating Dictionaries in Python
- Troubleshooting Common Issues in Python Dictionary Updates
- Understanding Python’s Dictionary Data Type
- Exploring the Impact of Dictionary Updates in Python
- Wrapping Up: Mastering Python Dictionary Updates
Understanding Python’s update()
Method
Python’s update()
function is a simple yet powerful tool for updating dictionaries. This method allows you to add new items or modify existing ones, making it a go-to solution for many developers.
Let’s take a look at a basic example:
dict1 = {'a': 1, 'b': 2}
dict1.update({'b': 3, 'c': 4})
print(dict1)
# Output:
# {'a': 1, 'b': 3, 'c': 4}
In this example, dict1
is our original dictionary with keys ‘a’ and ‘b’. We then use the update()
method to change the value of ‘b’ from 2 to 3 and add a new key-value pair ‘c’: 4 to the dictionary.
Advantages of the update()
Method
The update()
method is straightforward and easy to use, making it a great way to update dictionaries in Python for beginners. It’s also very flexible, as it can accept dictionaries, iterables with key-value pairs, and keyword arguments.
Potential Pitfalls of the update()
Method
While the update()
method is powerful, it’s important to use it correctly. One common pitfall is trying to update a dictionary with a key that doesn’t exist. In this case, the update()
method will add a new key-value pair to the dictionary, which might not be the desired outcome.
Another potential issue is trying to update a dictionary with a non-iterable object. The update()
method requires an iterable, so trying to use a non-iterable will result in a TypeError.
Advanced Techniques for Updating Dictionaries in Python
As you become more comfortable with Python and its dictionaries, you may want to explore more advanced techniques for updating dictionaries. These methods include using dictionary comprehension, merging dictionaries, and using the **
operator.
Dictionary Comprehension
Dictionary comprehension is a concise and readable way to create and update dictionaries. Here’s how you can use it to update a dictionary:
original_dict = {'a': 1, 'b': 2, 'c': 3}
update_dict = {'b': 3, 'c': 4, 'd': 5}
new_dict = {**original_dict, **update_dict}
print(new_dict)
# Output:
# {'a': 1, 'b': 3, 'c': 4, 'd': 5}
In this example, we have two dictionaries: original_dict
and update_dict
. We use dictionary comprehension to merge these two dictionaries into new_dict
. The values from update_dict
overwrite the values from original_dict
for any common keys.
Merging Dictionaries
Python also provides the |
operator to merge two dictionaries. It’s similar to using the update()
method or dictionary comprehension:
original_dict = {'a': 1, 'b': 2, 'c': 3}
update_dict = {'b': 3, 'c': 4, 'd': 5}
new_dict = original_dict | update_dict
print(new_dict)
# Output:
# {'a': 1, 'b': 3, 'c': 4, 'd': 5}
Again, the values from update_dict
overwrite the values from original_dict
for any common keys.
The **
Operator
The **
operator is another way to merge dictionaries in Python. It works similarly to the |
operator and dictionary comprehension:
original_dict = {'a': 1, 'b': 2, 'c': 3}
update_dict = {'b': 3, 'c': 4, 'd': 5}
new_dict = {**original_dict, **update_dict}
print(new_dict)
# Output:
# {'a': 1, 'b': 3, 'c': 4, 'd': 5}
This code creates a new dictionary new_dict
by merging original_dict
and update_dict
. The **
operator unpacks the dictionaries and overwrites the values of original_dict
with the values of update_dict
for any common keys.
These advanced techniques provide more flexibility and control when updating dictionaries in Python. However, they also require a deeper understanding of Python’s syntax and concepts. Always remember to choose the method that best fits your needs and the specific task at hand.
Exploring Alternative Methods for Updating Dictionaries in Python
As we delve deeper into Python’s capabilities, we discover that there are alternative ways to update dictionaries. These methods include using the setdefault()
method and leveraging third-party libraries.
Using the setdefault()
Method
The setdefault()
method in Python is a less common, but still useful, way to update dictionaries. This method returns the value of a key if it exists. However, if the key does not exist, it inserts the key with a specified value.
Here’s an example:
dict1 = {'a': 1, 'b': 2}
dict1.setdefault('c', 3)
print(dict1)
# Output:
# {'a': 1, 'b': 2, 'c': 3}
In this case, the key ‘c’ did not exist in the dictionary dict1
, so the setdefault()
method added ‘c’: 3 to the dictionary.
Leveraging Third-Party Libraries
Third-party libraries can also provide alternative ways to update dictionaries. For example, the collections
library’s defaultdict
function can be used to automatically assign a default value to non-existent keys when they’re accessed, effectively updating the dictionary.
from collections import defaultdict
dict1 = defaultdict(lambda: 0, {'a': 1, 'b': 2})
print(dict1['c'])
# Output:
# 0
In this example, we create a defaultdict
where non-existent keys return a default value of 0. When we access ‘c’, which doesn’t exist in dict1
, it returns 0.
These alternative methods offer more flexibility and functionality, but they may also add complexity to your code. It’s essential to understand the trade-offs and choose the method that best suits your specific needs.
Troubleshooting Common Issues in Python Dictionary Updates
As with any programming task, updating dictionaries in Python can sometimes lead to unexpected issues. In this section, we’ll discuss some common problems you might encounter and provide solutions and tips to help you navigate these challenges.
Dealing with Key Errors
One common issue when updating dictionaries is encountering a KeyError
. This error occurs when you try to access or update a key that doesn’t exist in the dictionary.
dict1 = {'a': 1, 'b': 2}
print(dict1['c'])
# Output:
# KeyError: 'c'
In this example, we’re trying to print the value of ‘c’, which doesn’t exist in dict1
, resulting in a KeyError
.
To avoid this, you can use the get()
method, which returns None
if the key doesn’t exist, or a default value that you can specify.
dict1 = {'a': 1, 'b': 2}
print(dict1.get('c', 0))
# Output:
# 0
Handling Type Errors
Another common issue is a TypeError
, which occurs when you try to use an unhashable type, like a list, as a dictionary key.
dict1 = {['a']: 1, 'b': 2}
# Output:
# TypeError: unhashable type: 'list'
In this example, we’re trying to use a list ['a']
as a key, which is not allowed in Python dictionaries. To solve this, you can convert the list to a tuple, which is hashable and can be used as a dictionary key.
dict1 = {('a',): 1, 'b': 2}
print(dict1)
# Output:
# {('a',): 1, 'b': 2}
These are just a few examples of the issues you might encounter when updating dictionaries in Python. Understanding these common problems and their solutions can help you write more robust and error-free code.
Understanding Python’s Dictionary Data Type
Before we delve deeper into updating dictionaries, it’s essential to understand what a dictionary in Python is and why it’s a powerful data structure.
A dictionary in Python is an unordered collection of data values used to store data values like a map. It’s a mutable data type that stores data in key:value
pairs. Unlike other data types that hold only a single value as an element, dictionaries hold key:value
pairs.
# An example of a Python dictionary
my_dict = {
'name': 'John',
'age': 27,
'profession': 'Engineer'
}
print(my_dict)
# Output:
# {'name': 'John', 'age': 27, 'profession': 'Engineer'}
In this example, my_dict
is a dictionary with three key-value pairs. The keys are ‘name’, ‘age’, and ‘profession’, and the corresponding values are ‘John’, 27, and ‘Engineer’.
One of the main features of dictionaries that makes them unique is the fact that they are mutable, i.e., we can change, add or remove items after the dictionary is created. This is where the update()
method and other techniques for updating dictionaries come into play.
Understanding the fundamental nature of Python’s dictionary data type is crucial for grasping the concepts underlying dictionary updates. With this knowledge, you’ll be better equipped to handle dictionary updates and tackle any related challenges.
Exploring the Impact of Dictionary Updates in Python
Python’s dictionary updates are not just a stand-alone operation. In fact, they play a significant role in various areas of programming and data manipulation. Let’s explore how they impact different domains.
Dictionary Updates in Data Manipulation
In data manipulation, Python dictionaries are often used to store and manipulate data. Updating dictionaries allows us to modify this data dynamically, which is crucial in data analysis.
Python Dictionary Updates in Web Scraping
Web scraping often involves handling large amounts of data. Python dictionaries serve as an efficient way to store and manage this data. Updating dictionaries allows us to add, modify, or delete data as we scrape websites.
Exploring Related Concepts
While updating dictionaries is a fundamental task, there are several related concepts that are worth exploring. These include nested dictionaries, where a dictionary contains other dictionaries as values, and other dictionary methods like pop()
, clear()
, and copy()
.
# An example of a nested dictionary
nested_dict = {
'dict1': {'name': 'John', 'age': 27},
'dict2': {'name': 'Jane', 'age': 25}
}
# Updating a nested dictionary
nested_dict['dict1']['age'] = 28
print(nested_dict)
# Output:
# {'dict1': {'name': 'John', 'age': 28}, 'dict2': {'name': 'Jane', 'age': 25}}
In this example, we have a nested dictionary nested_dict
. We update the age of the person in dict1
from 27 to 28 using dictionary update techniques.
Further Resources for Python Dictionary Mastery
To dive deeper into Python dictionaries and their manipulation, you might find the following resources helpful:
- Python Dictionary Examples and Syntax – Learn the ins and outs of Python dictionaries, from basic usage to advanced techniques, with this complete IOFlood guide.
Adding Entries to Python Dictionaries: A How-To Guide by IOFlood – Discover methods to add new entries to Python dictionaries.
Python Nested Dictionary Tutorial: Creating and Accessing – Master the art of creating and manipulating nested dictionaries.
Python Documentation: Dictionaries – A comprehensive guide to dictionaries straight from Python’s official documentation.
Real Python’s Dictionaries in Python – An in-depth tutorial on Python dictionaries, including how to update, iterate over, and manipulate them.
Dictionary Manipulation in Python by PythonForBeginners – A beginner-friendly guide to dictionary manipulation in Python, including updating dictionaries.
Wrapping Up: Mastering Python Dictionary Updates
In this comprehensive guide, we’ve delved into the process of updating dictionaries in Python. We’ve explored how a simple data structure like a dictionary can be efficiently manipulated using Python’s built-in methods and some advanced techniques.
We began with the basics, introducing the concept of Python dictionaries and how to update them using the update()
method. We then moved onto more advanced techniques, covering dictionary comprehension, merging dictionaries, and the use of the **
operator. We also explored alternative methods such as the setdefault()
method and leveraging third-party libraries.
Along the way, we tackled common issues you might encounter when updating dictionaries, such as KeyError
and TypeError
. We provided solutions and workarounds for these challenges, equipping you to handle any roadblocks in your Python dictionary journey.
Here’s a quick comparison to the options we discussed:
Method | Ease of Use | Flexibility | Use Case |
---|---|---|---|
update() method | High | Moderate | Basic dictionary updates |
Dictionary comprehension | High | High | Advanced dictionary updates |
Merging dictionaries | Moderate | High | Merging two or more dictionaries |
setdefault() method | Moderate | Moderate | Adding new keys or updating existing ones |
Third-party libraries | Low | High | Specialized tasks |
Whether you’re just starting out with Python dictionaries or looking to enhance your data manipulation skills, we hope this guide has provided you with a deeper understanding of how to update dictionaries in Python.
Understanding how to update dictionaries in Python is a crucial skill for any Python programmer. With this knowledge, you’re now equipped to handle and manipulate dictionary data more effectively. Happy coding!