Python Dictionary Guide | Examples, Syntax, and Usage
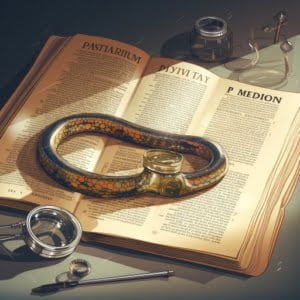
Are Python dictionaries making your head spin? Do you find yourself wondering how they stack up against lists, or how to put them to work in your code? If you’re nodding in agreement, you’ve landed at the right spot! Python dictionaries, much like lists, are mutable and dynamic data structures that can nest to accommodate complex data forms. However, they boast a unique set of features and applications.
In this comprehensive guide, we aim to decode the enigma of Python dictionaries for you. We’ll traverse through everything from their basic attributes to the effective ways to access and manage dictionary data. So, are you ready for a deep dive into the realm of Python dictionaries? Let’s embark on this journey!
TL;DR: What are Python Dictionaries?
Python dictionaries are a mutable, dynamic data structure in Python that can store complex data types. They work on a unique key-value pair system, making them different from lists. This guide covers the essentials of Python dictionaries, their comparison with lists, and their effective usage in Python programming.
Table of Contents
Python Dictionary Basics
As you embark on your Python journey, you’ll encounter various data types. Among the most flexible and frequently used are lists and dictionaries. At a glance, they might appear quite similar. Both are mutable, which means their content can be altered without changing their identity. They’re dynamic, enabling you to add or remove items spontaneously. Moreover, they can both be nested, leading to lists of lists, dictionaries of dictionaries, or even dictionaries of lists!
However, a key distinction sets them apart: the method of accessing their elements. For lists, an index is used, a numerical value signifying an item’s position in the list. In contrast, dictionaries operate differently. Here, a unique key is utilized to access a corresponding value. This key-value pair system is what lends dictionaries their uniqueness and strength.
So, how do you define a dictionary in Python? There are a couple of ways. The most direct approach is to use curly braces {}
and separate keys from values with a colon :
. For example, my_dict = {'name': 'John', 'age': 30}
. Alternatively, you can use the dict()
constructor, like so: my_dict = dict(name='John', age=30)
.
my_dict = {'name': 'John', 'age': 30}
my_dict = dict(name='John', age=30)
These key-value pairs form the crux of Python dictionaries. They facilitate data storage and organization in a manner that’s easy to comprehend and access. But here’s the twist: keys and values in dictionaries can be of different data types. Your keys could be strings, and your values could be integers, lists, or even other dictionaries. This versatility is one of the reasons why Python dictionaries are so widely used in data manipulation and organization.
Example of a dictionary with different data types as keys and values:
my_dict = {'name': 'John', 'age': 30, 3.14: 'pi', ('x', 'y'): 'coordinates'}
Accessing and Modifying Dictionary Values
With the basics of Python dictionaries under our belt, it’s time to delve into interacting with them. One of the most routine operations you’ll perform with a dictionary is fetching values. This is achieved using keys. For instance, if we have a dictionary my_dict = {'name': 'John', 'age': 30}
, we can access the value ‘John’ by using the key ‘name’ like this: print(my_dict['name'])
.
my_dict = {'name': 'John', 'age': 30}
print(my_dict['name'])
Incorporating entries into a dictionary is equally straightforward. You define a new key-value pair within the dictionary. For example, my_dict['job'] = 'Engineer'
adds a new entry with ‘job’ as the key and ‘Engineer’ as the value. Updating an entry adheres to the same syntax. If we want to update ‘age’ to 31, we’d write my_dict['age'] = 31
.
my_dict['job'] = 'Engineer'
my_dict['age'] = 31
To delete entries, the del
keyword is used, followed by the dictionary key. For example, del my_dict['job']
would eliminate the ‘job’ entry from the dictionary.
del my_dict['job']
Lists indices vs Dictionary keys
While these operations are simple, a crucial difference between list indices and dictionary keys is worth noting. List indices are always integers and signify a position in the list. Dictionary keys, on the other hand, can be of diverse data types and represent a specific value rather than a position.
This leads us to an essential requirement for dictionary keys: they must be immutable, meaning they can’t be altered. This is why you can use integers, strings, and tuples as dictionary keys but not lists or other dictionaries. However, dictionary values have no such limitation and can be of any type.
Lastly, what transpires when you attempt to access a key that doesn’t exist in the dictionary? Python raises a KeyError
. To handle this, Python offers several mechanisms, one of which is the get()
method. This method returns the value for a key if it exists in the dictionary; otherwise, it returns a default value. For example, print(my_dict.get('salary', 'Not Found'))
would print ‘Not Found’ since ‘salary’ is not a key in our dictionary.
Example of handling a KeyError
when trying to access a key that doesn’t exist in the dictionary:
try:
print(my_dict['salary'])
except KeyError:
print('Not Found')
print(my_dict.get('salary', 'Not Found'))
Incremental Building and Modification of Dictionaries
One of the major strengths of Python dictionaries is their flexibility, which allows you to build and modify them incrementally. You can kick off with an empty dictionary and add entries as you proceed. For instance, my_dict = {}
initiates an empty dictionary, and my_dict['name'] = 'John'
contributes a new entry to it.
Example of adding entries to a nested dictionary:
my_dict = {'person': {}}
my_dict['person']['name'] = 'John'
my_dict['person']['age'] = 30
my_dict = {}
my_dict['name'] = 'John'
What if you have a dictionary within a dictionary? This is where the concept of nested dictionaries comes into play. Let’s assume we have a dictionary like my_dict = {'person': {'name': 'John', 'age': 30}}
. To access ‘John’, you would first need to access the ‘person’ dictionary and then the ‘name’ key, as shown: print(my_dict['person']['name'])
.
my_dict = {'person': {'name': 'John', 'age': 30}}
print(my_dict['person']['name'])
This example underscores the versatility of dictionaries in handling a diverse range of data types. From integers and strings to lists and other dictionaries, a Python dictionary can store a broad spectrum of data types. This versatility makes them an indispensable tool for managing complex data structures.
Dictionary Key Immutability
Despite their versatility, dictionary keys come with a restriction: they must be immutable. This is why you can use data types like integers, strings, and tuples as dictionary keys but not lists or other dictionaries. If you attempt to use a mutable data type as a dictionary key, Python will raise a TypeError
.
Example of what happens when you try to use a mutable data type as a dictionary key:
try:
my_dict = {['x', 'y']: 'coordinates'}
except TypeError:
print('Cannot use a mutable data type as a dictionary key.')
Hashable Objects in Python
The immutability restriction on dictionary keys introduces the concept of hashable objects in Python. A hashable object possesses a hash value that remains constant throughout its lifetime. It can be compared to other objects and can serve as a dictionary key. All of Python’s immutable built-in objects are hashable, while no mutable containers (such as lists or dictionaries) are. This is why, for instance, you can use a tuple as a dictionary key (since it’s immutable and therefore hashable) but not a list.
Example of using a tuple as a dictionary key:
my_dict = {('x', 'y'): 'coordinates'}
Operators, Built-in Functions, and Methods for Dictionaries
Python equips you with a variety of operators, built-in functions, and methods that enhance the efficiency of working with dictionaries.
Operators
One of the most fundamental operations you can perform on a dictionary is verifying if a key exists. This is done using the ‘in’ and ‘not in’ operators. For example, if 'name' in my_dict:
checks if the key ‘name’ exists in the dictionary my_dict
.
if 'name' in my_dict:
Built-in Functions
Another simple yet handy function is len()
, which returns the number of key-value pairs in a dictionary. For instance, len(my_dict)
would return the number of entries in my_dict
.
len(my_dict)
Methods for Dictionaries
Python dictionaries also come with a plethora of built-in methods that allow you to manipulate and access dictionary data efficiently:
.items()
returns a view object that displays a list of a dictionary’s key-value tuple pairs..keys()
returns a view object that displays a list of all the keys..values()
returns a view object that displays a list of all the values..get(key[, default])
returns the value for a key if it exists in the dictionary; otherwise, it returns a default value..pop(key[, default])
removes a key from a dictionary, if it is present, and returns its value..popitem()
removes and returns a (key, value) pair from the dictionary. Pairs are returned in LIFO (Last In, First Out) order..update([other])
updates the dictionary with the key-value pairs from other, overwriting existing keys.
my_dict.items()
my_dict.keys()
my_dict.values()
my_dict.get(key[, default])
my_dict.pop(key[, default])
my_dict.popitem()
my_dict.update([other])
The returned views from the .items()
, .keys()
, and .values()
methods are dynamic and reflect any changes to the dictionary.
It’s important to note that dictionaries’ behavior has somewhat changed in recent Python versions. As of Python 3.7, dictionaries maintain the insertion order of their elements, meaning that if you add new key-value pairs to a dictionary, they will be added to the end of the dictionary.
Example of using the update()
method to merge two dictionaries:
my_dict = {'name': 'John', 'age': 30}
other_dict = {'job': 'Engineer', 'city': 'New York'}
my_dict.update(other_dict)
This feature can be particularly useful when you need to rely on the order of items in a dictionary.
Further Resources for Python Dictionaries
- Understanding Python Data Types – A comprehensive guide on the fundamental Python data types and their applications, including Dictionaries and more.
Python Dictionary Methods | Unlocking the Power of Dictionaries – Learn essential Python dictionary methods for effective data manipulation.
Creating Python Dictionaries: A Step-by-Step Tutorial – Explore techniques for creating Python dictionaries from scratch.
Python Cheat Sheet: Dictionaries – This cheat sheet is a helpful quick reference for common operations with Python dictionaries.
Working with Lists, Dictionaries in Python – Analytics Vidhya provides a tutorial on how to work with lists and dictionaries in Python.
What is a Key in Python Dictionaries? – A discussion on SoloLearn forum explaining the concept of keys in Python dictionaries.
Wrapping Up Python Dictionaries
We’ve taken a deep dive into the world of Python dictionaries in this guide. We’ve examined their similarities and differences with lists, their unique characteristics, and their wide range of applications in Python programming. Dictionaries, with their key-value pair system, provide a dynamic and flexible method to manage and manipulate data. Whether you’re dealing with a few entries or managing complex, nested data structures, Python dictionaries prove to be an invaluable tool.
As we wrap up, consider broadening your Python knowledge with this handy reference guide.
In essence, Python dictionaries are a robust, flexible, and efficient way to store and manipulate paired data in Python. They stand as a testament to Python’s commitment to readability and ease of use, and are a fundamental part of any Python programmer’s toolkit. So, the next time you find yourself grappling with complex data structures, remember: Python dictionaries might just be the solution you’re seeking!