Python Create Dictionary | Guide (With Examples)
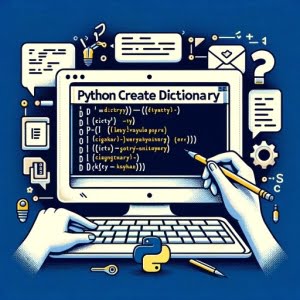
Are you confused about creating dictionaries in Python? Don’t worry, you’re not alone. Many beginners find Python dictionaries a bit challenging at first.
Think of a Python dictionary as a real-life dictionary. In a real-life dictionary, you look up definitions using words. Similarly, in a Python dictionary, you look up values using keys.
This guide will walk you through the process of creating dictionaries in Python, from the basics to more advanced techniques. So, whether you’re a beginner or an intermediate Python programmer, there’s something here for you.
TL;DR: How Do I Create a Dictionary in Python?
You can create a dictionary in Python using curly braces
{}
and separating keys and values with a colon:
. Here’s a simple example:
my_dict = {'apple': 1, 'banana': 2}
print(my_dict)
# Output:
# {'apple': 1, 'banana': 2}
In this example, we created a dictionary named my_dict
with two key-value pairs. The keys are ‘apple’ and ‘banana’, and their corresponding values are 1 and 2. When we print my_dict
, Python displays the entire dictionary.
This is just the tip of the iceberg! Read on for a more detailed explanation and advanced usage of Python dictionaries.
Table of Contents
- Creating a Dictionary in Python
- Exploring Advanced Dictionary Use
- Alternative Ways to Create Dictionaries
- Troubleshooting Common Issues with Python Dictionaries
- Understanding Python’s Dictionary Data Type
- Practical Applications of Python Dictionaries
- Exploring Related Concepts
- Wrapping Up: Python Dictionary Creation
Creating a Dictionary in Python
Creating a dictionary in Python is straightforward. You define a dictionary using curly braces {}
. Inside these braces, you add key-value pairs separated by a colon :
. Let’s create a simple dictionary.
fruit_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
print(fruit_dict)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3}
In this example, fruit_dict
is a dictionary with three key-value pairs. The keys are ‘apple’, ‘banana’, and ‘cherry’, and their corresponding values are 1, 2, and 3, respectively.
Adding, Removing, and Modifying Items in a Dictionary
Once you have a dictionary, you can add, remove, or modify its items. Let’s see how.
Adding Items
To add an item to a dictionary, you use the new key as an index and assign a value to it.
fruit_dict['date'] = 4
print(fruit_dict)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3, 'date': 4}
Removing Items
To remove an item, use the del
statement and specify the key of the item you want to remove.
del fruit_dict['date']
print(fruit_dict)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3}
Modifying Items
To modify an item, use its key as an index and assign a new value to it.
fruit_dict['apple'] = 5
print(fruit_dict)
# Output:
# {'apple': 5, 'banana': 2, 'cherry': 3}
Accessing Values Using Keys
To access a value in a dictionary, you use its corresponding key.
print(fruit_dict['apple'])
# Output:
# 5
In this example, we accessed the value of the key ‘apple’, which is 5. Remember, if you use a key that doesn’t exist in the dictionary, Python will raise a KeyError.
Exploring Advanced Dictionary Use
Python dictionaries offer a lot more than just storing key-value pairs. Let’s dive into some of the advanced uses of dictionaries.
Nested Dictionaries
A dictionary can contain other dictionaries, which are known as nested dictionaries. Let’s create a dictionary that contains other dictionaries.
nested_dict = {
'fruit': {'apple': 1, 'banana': 2},
'vegetable': {'carrot': 1, 'pea': 2}
}
print(nested_dict)
# Output:
# {'fruit': {'apple': 1, 'banana': 2}, 'vegetable': {'carrot': 1, 'pea': 2}}
In this example, nested_dict
is a dictionary that contains two dictionaries. Each of these dictionaries represents a category of food and contains its own key-value pairs.
Dictionary Comprehension
Dictionary comprehension is a concise way to create dictionaries. It’s similar to list comprehension but with an additional requirement of defining a key.
dict_comp = {x: x**2 for x in range(5)}
print(dict_comp)
# Output:
# {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
In this example, dict_comp
is a dictionary that contains numbers from 0 to 4 as keys and their squares as values. We created this dictionary using a single line of code with dictionary comprehension.
Built-In Dictionary Methods
Python provides several built-in methods that you can use with dictionaries. Here are a few examples.
The get()
Method
The get()
method returns the value of a specified key. If the key doesn’t exist, it returns a default value.
print(fruit_dict.get('apple', 'Not found'))
# Output:
# 5
In this example, the get()
method returns the value of the key ‘apple’. If ‘apple’ didn’t exist in the dictionary, it would return ‘Not found’.
The keys()
and values()
Methods
The keys()
method returns a view object that displays a list of all the keys in the dictionary. The values()
method returns a view object that displays a list of all the values in the dictionary.
print(fruit_dict.keys())
print(fruit_dict.values())
# Output:
# dict_keys(['apple', 'banana', 'cherry'])
# dict_values([5, 2, 3])
These are just a few examples of the many built-in methods Python provides for dictionaries. You can find more in the Python documentation.
Alternative Ways to Create Dictionaries
Python offers several alternative approaches to create dictionaries, providing flexibility in different programming scenarios. Let’s explore some of these methods.
Using the dict()
Constructor
Python provides the dict()
constructor that lets you create dictionaries. Here’s how you can use it.
dict_constructor = dict(apple=1, banana=2, cherry=3)
print(dict_constructor)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3}
In this example, we used the dict()
constructor to create a dictionary. We passed arguments to the constructor where each argument is a key-value pair.
Converting Other Data Structures to Dictionaries
You can also convert other data structures to dictionaries. For example, you can convert a list of tuples into a dictionary.
list_of_tuples = [('apple', 1), ('banana', 2), ('cherry', 3)]
dict_from_list = dict(list_of_tuples)
print(dict_from_list)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3}
In this example, list_of_tuples
is a list that contains tuples. Each tuple is a pair of a fruit name and a number. We then used the dict()
constructor to convert this list into a dictionary.
These alternative approaches offer more flexibility and can be more suitable in certain scenarios. For example, the dict()
constructor is a more readable way to create dictionaries, and converting other data structures to dictionaries can be useful when you’re dealing with complex data.
Troubleshooting Common Issues with Python Dictionaries
While Python dictionaries are powerful and flexible, they can also lead to common issues if not used properly. Let’s discuss some of these issues and how to avoid them.
Dealing with KeyError
A KeyError
occurs when you try to access a dictionary key that doesn’t exist. This is one of the most common issues when working with dictionaries.
try:
print(fruit_dict['mango'])
except KeyError:
print('Key not found in dictionary')
# Output:
# Key not found in dictionary
In this example, we tried to access the key ‘mango’ which doesn’t exist in fruit_dict
. Python raises a KeyError
, but we caught it using a try-except block and printed a user-friendly message.
To avoid a KeyError
, you can use the get()
method that we discussed earlier. It returns a default value if the key doesn’t exist.
print(fruit_dict.get('mango', 'Not found'))
# Output:
# Not found
Considerations When Using Mutable Objects as Dictionary Keys
In Python, only immutable objects can be used as dictionary keys. This means you can’t use lists or other dictionaries as keys because they’re mutable. If you try to do so, Python raises a TypeError
.
try:
bad_dict = {[1, 2, 3]: 'numbers'}
except TypeError:
print('Cannot use a mutable object as a dictionary key')
# Output:
# Cannot use a mutable object as a dictionary key
In this example, we tried to use a list [1, 2, 3]
as a dictionary key, which is not allowed in Python. Python raises a TypeError
, but we caught it using a try-except block and printed a user-friendly message.
Remember, when creating dictionaries in Python, always use immutable objects as keys and be cautious when accessing keys that might not exist in the dictionary.
Understanding Python’s Dictionary Data Type
Python’s dictionary is a built-in data type that stores data in key-value pairs. It’s an example of a hash table, which is a data structure that uses a hash function to map keys to their associated values.
Characteristics of Python’s Dictionary
Python’s dictionary has several unique characteristics. Let’s explore some of them.
Unordered
Dictionaries in Python are unordered. This means that the items in a dictionary don’t have a defined order. They’re stored in the way that allows Python to optimize for speed when looking up keys.
Mutable
Dictionaries are mutable. You can add, remove, or modify items after a dictionary is created.
Indexed by Keys
Items in a dictionary are indexed by their keys. You can use a key to access its associated value.
print(fruit_dict['apple'])
# Output:
# 5
In this example, we accessed the value of the key ‘apple’, which is 5.
Advantages Over Other Data Structures
Python’s dictionary offers several advantages over other data structures.
Fast Lookups
Dictionaries use a hash table for storage, which provides fast lookups regardless of the number of items in the dictionary.
Flexible Data Storage
Each value in a dictionary can be of a different data type. You can store a number, a string, a list, or even another dictionary as a value.
Easy to Use
Python provides several built-in methods that make it easy to work with dictionaries. You can add, remove, or modify items, check if a key exists, loop through the keys or values, and more.
In conclusion, Python’s dictionary is a versatile and efficient data structure that can greatly simplify your code and improve performance when used properly.
Practical Applications of Python Dictionaries
Python dictionaries are more than just a way to store and retrieve data. They have many practical applications in Python programming, making them an essential part of a Python programmer’s toolkit.
Data Storage and Organization
Dictionaries are incredibly useful for data storage and organization. They allow you to pair related data together, making it easy to organize and retrieve. For example, you can use a dictionary to store user information, where each key is a user attribute (like ‘name’ or ‘age’), and each value is the specific data for that attribute.
user_info = {'name': 'John Doe', 'age': 30, 'email': '[email protected]'}
print(user_info)
# Output:
# {'name': 'John Doe', 'age': 30, 'email': '[email protected]'}
In this example, user_info
is a dictionary that stores information about a user. We can easily retrieve any piece of information using the appropriate key.
Complex Data Structures
Dictionaries can also be used to build more complex data structures. For example, you can use dictionaries to create graphs, which are a fundamental data structure in computer science. In a graph, each key could represent a node, and its value could be a list or another dictionary representing the adjacent nodes.
graph = {'A': ['B', 'C'], 'B': ['A', 'D'], 'C': ['A', 'D'], 'D': ['B', 'C']}
print(graph)
# Output:
# {'A': ['B', 'C'], 'B': ['A', 'D'], 'C': ['A', 'D'], 'D': ['B', 'C']}
In this example, graph
is a dictionary that represents a graph. Each key is a node, and its value is a list of adjacent nodes.
Exploring Related Concepts
To enhance your Python dictionary skills, you might find the following resources helpful:
- IOFlood’s article “Python Dictionary: Your Comprehensive Guide” explains examples, syntax, and advanced uses. Discover the elegance of Python dictionaries and its role in simplifying complex data structures.
Converting Python Dictionaries to JSON: A Complete Guide – Learn how to convert Python dictionaries to JSON format.
Ensuring Data Integrity: Checking for Keys in Python Dictionaries – Master the art of verifying key presence in Python dictionaries with this IOFlood guide.
Article on How Python Implements Dictionaries – This article breaks down and explains the technical implementation of dictionaries in Python.
Towards Data Science’s Advanced Python Dictionary Techniques – This article shares seven advanced techniques for working with Python dictionaries, which can help readers write more efficient code.
Tutorial on Python Dictionary Comprehension – This tutorial provided by DataCamp explores dictionary comprehension in Python, an efficient technique to create dictionaries from iterables.
Once you’re comfortable with dictionaries, you can explore related concepts in Python, such as sets, lists, and tuples. These data structures have their own unique characteristics and use-cases, and understanding them will make you a more versatile Python programmer.
Wrapping Up: Python Dictionary Creation
In this guide, we’ve explored how to create dictionaries in Python. We started with the basics, learning to create a dictionary using curly braces {}
and separating keys and values with a colon :
. We also learned how to add, remove, and modify dictionary items, and how to access values using keys.
We also delved into more advanced uses of dictionaries, such as nested dictionaries, dictionary comprehension, and using built-in dictionary methods. We learned how to create dictionaries using the dict()
constructor and how to convert other data structures to dictionaries.
We discussed some common issues when working with dictionaries, such as KeyError
, and how to avoid them. We also explored the characteristics of Python’s dictionary data type and its advantages over other data structures.
Finally, we looked at the practical applications of dictionaries in Python programming, such as data storage and organization, and in more complex data structures. We also suggested exploring related concepts like sets, lists, and tuples.
Python dictionaries are a versatile and efficient data structure that can greatly simplify your code and improve performance when used properly. Whether you’re a beginner or an intermediate Python programmer, mastering dictionaries will be a valuable addition to your coding skills.