Convert a Python Dict to JSON | json.dumps() User Guide
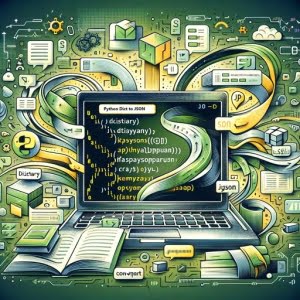
Ever found yourself tangled in the task of converting Python dictionaries to JSON? Fear not, Python, like a skilled linguist, can effortlessly translate dictionaries into the universally recognized language of JSON.
This comprehensive guide will walk you through the process, from the elementary steps to the more advanced techniques. We’ll delve into Python’s json
module, explore its functions, and see how we can leverage them to convert Python dictionaries into JSON.
So whether you’re a beginner or an intermediate programmer, there’s something for you here. Let’s dive in and demystify the process of converting Python dictionaries to JSON.
TL;DR: How Do I Convert a Python Dictionary to JSON?
Quite simply, you use the
json.dumps()
function provided by Python. Here’s a quick example to illustrate this:
import json
# Here's our Python dictionary
dict_data = {'name': 'John', 'age': 30}
# We use json.dumps() to convert it to JSON
json_data = json.dumps(dict_data)
print(json_data)
# Output:
# '{"name": "John", "age": 30}'
In the code above, we first import the json
module. We then define a Python dictionary dict_data
. Using the json.dumps()
function, we convert this dictionary into a JSON object, json_data
, which we then print. The output is a JSON-formatted string.
Intrigued? Keep reading for a more detailed walkthrough and exploration of advanced scenarios in the conversion of Python dictionaries to JSON.
Table of Contents
Basic Conversion: Python Dictionary to JSON
Python provides a built-in module json
that comes with a method json.dumps()
. This method converts Python data types into their JSON equivalents. Let’s focus on how we can use it to convert a Python dictionary to a JSON object.
import json
# Define a Python dictionary
dict_data = {'name': 'John', 'age': 30, 'city': 'New York'}
# Use json.dumps() to convert the dictionary to JSON
json_data = json.dumps(dict_data)
print(json_data)
# Output: '{"name": "John", "age": 30, "city": "New York"}'
In the example above, we first import the json
module. We then create a Python dictionary dict_data
. Using the json.dumps()
function, we convert this dictionary into a JSON object, json_data
, which we then print.
The output is a JSON-formatted string. This is the most basic use of json.dumps()
to convert a Python dictionary into JSON.
Advantages and Pitfalls
The json.dumps()
function is straightforward and easy to use. It’s part of Python’s built-in json
module, so there’s no need for additional installations.
However, it’s important to remember that not all Python data types can be converted into JSON. For example, Python sets and custom classes will raise a TypeError
when you try to convert them to JSON using json.dumps()
.
We’ll cover more about this in the ‘Troubleshooting and Considerations’ section.
Handling Complex Dictionaries and Non-Serializable Objects
As we dive deeper into the world of Python dictionaries and JSON, we encounter more complex scenarios. Let’s explore how to handle complex dictionaries, nested structures, and non-serializable objects.
Dealing with Nested Structures
Python dictionaries can contain other dictionaries, creating nested structures. The json.dumps()
function can handle these nested structures without a problem. Let’s see this in action:
import json
# Define a nested Python dictionary
dict_data = {
'name': 'John',
'age': 30,
'city': 'New York',
'children': {
'child1': {
'name': 'Sam',
'age': 5
},
'child2': {
'name': 'Alex',
'age': 3
}
}
}
# Use json.dumps() to convert the dictionary to JSON
json_data = json.dumps(dict_data)
print(json_data)
# Output: '{"name": "John", "age": 30, "city": "New York", "children": {"child1": {"name": "Sam", "age": 5}, "child2": {"name": "Alex", "age": 3}}}'
In this example, our Python dictionary dict_data
has another dictionary as a value. When we use json.dumps()
, it processes the nested dictionary just like it would a simple one, converting the entire structure into a JSON object.
Non-Serializable Objects
However, there are certain Python objects that json.dumps()
cannot serialize into JSON. For example, Python’s set data type. If you attempt to convert a set to JSON, you’ll encounter a TypeError
. Let’s illustrate this:
import json
# Define a Python dictionary containing a set
dict_data = {'name': 'John', 'age': 30, 'cities_visited': {'New York', 'London', 'Paris'}}
# Attempt to use json.dumps() to convert the dictionary to JSON
try:
json_data = json.dumps(dict_data)
except TypeError as e:
print(f'Error: {e}')
# Output: Error: Object of type set is not JSON serializable
In the above code, our dictionary dict_data
contains a set as a value. When we try to convert this dictionary to JSON using json.dumps()
, we get a TypeError
.
This is because the json.dumps()
function does not know how to convert a set into a JSON-compatible format. We’ll explore how to handle these non-serializable objects in the ‘Troubleshooting and Considerations’ section.
Exploring Alternative Methods for Conversion
While Python’s built-in json
module is a powerful tool for converting dictionaries to JSON, it’s not the only game in town. For those seeking alternative methods, the simplejson
library offers a viable option.
Using Simplejson for Conversion
The simplejson
library works similarly to the json
module, but it’s more flexible when dealing with non-serializable objects. Let’s see it in action:
import simplejson
# Define a Python dictionary containing a set
dict_data = {'name': 'John', 'age': 30, 'cities_visited': {'New York', 'London', 'Paris'}}
# Use simplejson.dumps() to convert the dictionary to JSON
json_data = simplejson.dumps(dict_data, ignore_nan=True)
print(json_data)
# Output: '{"name": "John", "age": 30, "cities_visited": ["Paris", "New York", "London"]}'
In the example above, we import the simplejson
library and define a Python dictionary that contains a set. Using simplejson.dumps()
, we’re able to convert the set into a JSON array, which is not possible with json.dumps()
.
Advantages and Disadvantages
The simplejson
library can handle more data types than the json
module and offers more control over how your data is serialized.
However, it’s not a built-in Python module, so it requires an additional installation. Also, it may behave differently from json.dumps()
, which could lead to unexpected results.
Here’s a comparison table to help you choose the best method for your needs:
Method | Handles Complex Structures | Handles Non-Serializable Objects | Requires Additional Installation |
---|---|---|---|
json.dumps() | Yes | No | No |
simplejson.dumps() | Yes | Yes (with more flexibility) | Yes |
While json.dumps()
is a great go-to method for most scenarios, simplejson.dumps()
can be a powerful alternative when dealing with non-serializable objects or when you need more control over the serialization process.
Troubleshooting Common Conversion Issues
While converting Python dictionaries to JSON is generally straightforward, you may encounter some issues. Let’s discuss common problems and their solutions.
Handling ‘TypeError: Object of type ‘set’ is not JSON serializable’
As we’ve seen earlier, certain Python data types like sets are not JSON serializable. Here’s how this error looks like:
import json
dict_data = {'name': 'John', 'age': 30, 'cities_visited': {'New York', 'London', 'Paris'}}
try:
json_data = json.dumps(dict_data)
except TypeError as e:
print(f'Error: {e}')
# Output: Error: Object of type set is not JSON serializable
In this code, we try to convert a Python dictionary containing a set to JSON using json.dumps()
. However, since sets are not JSON serializable, we encounter a TypeError
.
One way to handle this issue is to convert the set to a list before serializing it. Lists, unlike sets, are JSON serializable. Here’s how you can do it:
import json
dict_data = {'name': 'John', 'age': 30, 'cities_visited': {'New York', 'London', 'Paris'}}
# Convert set to list
dict_data['cities_visited'] = list(dict_data['cities_visited'])
try:
json_data = json.dumps(dict_data)
except TypeError as e:
print(f'Error: {e}')
else:
print(json_data)
# Output: '{"name": "John", "age": 30, "cities_visited": ["Paris", "New York", "London"]}'
In the code above, we first convert the set to a list using the list()
function. We then try to serialize the dictionary again. This time, json.dumps()
successfully converts the dictionary, including the list, to JSON.
Remember, understanding the data types that are not JSON serializable and pre-processing your data accordingly can save you from many headaches down the line.
Understanding Python Dictionaries and JSON
Before we dive deeper into the conversion process, it’s essential to understand the key players: Python dictionaries and JSON objects.
Python Dictionaries: Key-Value Storage
A Python dictionary is a built-in data type that stores data in key-value pairs. Each key is unique and the values can be just about anything, from numbers and strings to complex objects like lists and other dictionaries. Here’s an example of a Python dictionary:
# A Python dictionary
dict_data = {'name': 'John', 'age': 30, 'city': 'New York'}
print(dict_data)
# Output: {'name': 'John', 'age': 30, 'city': 'New York'}
In the above code, name
, age
, and city
are keys, and John
, 30
, and New York
are their respective values.
JSON Objects: The Universal Data Format
JSON (JavaScript Object Notation) is a popular data format with a diverse range of applications, from data storage to server-client communication.
A JSON object is similar to a Python dictionary, storing data in key-value pairs. However, JSON keys must be strings, and values can only be a finite number of types (string, number, array, boolean, null, object).
Serialization and Deserialization: The Conversion Process
The process of converting a Python dictionary to a JSON object is known as serialization.
In this process, the dictionary’s data is converted into a format (a string) that can be written to a file or transmitted over a network. The reverse process, converting a JSON object back into a Python dictionary, is known as deserialization.
Understanding these fundamental concepts is crucial for effectively converting Python dictionaries to JSON and vice versa.
Exploring Related Concepts
If you’re interested in delving deeper into this subject, there are plenty of related concepts to explore.
Working with JSON in Python, for instance, includes not only converting dictionaries to JSON but also parsing JSON data and converting it back to dictionaries. You might also want to look into REST APIs, which often use JSON for data exchange.
Further Learning Resources
There’s always more to learn in the vast world of Python and JSON. Here are a few resources to help you continue your journey:
- This article is a tutorial for Python Dictionary manipulation and advanced uses. Explore real-world use cases of Python dictionaries and learn how they can optimize your code.
Python Dictionary Key Lookup – Using the Get Function – Master the art of using the get() method to access dictionary values in Python.
Python Dictionary Initialization – Building Data Structures – Dive into Python dictionary creation and learn to initialize dictionaries.
Python official documentation on the json module – This is the official documentation for the json module, offering details on methods for parsing and handling JSON data.
Guide on working with JSON data – Mozilla provides a comprehensive guide on processing and understanding JSON data, though the guide is Javascript-focused, it provides solid foundational knowledge.
Tutorial on building a REST API with Python – This tutorial from Dataquest teaches the reader how to build a RESTful API from scratch by using Python.
Remember, the journey of mastering Python’s interaction with JSON is a marathon, not a sprint. Take your time, practice regularly, and don’t hesitate to experiment and ask questions.
Python Dictionary to JSON: A Recap
Throughout this comprehensive guide, we’ve explored how to convert Python dictionaries to JSON using the json.dumps()
function.
- We’ve seen how this function can handle simple and nested dictionaries, and we’ve also learned about its limitations with non-serializable objects like sets.
We’ve also discussed alternative methods for conversion, such as the
simplejson
library, which offers more flexibility with non-serializable objects.Finally, we’ve addressed common issues you might encounter during conversion and suggested ways to troubleshoot them, such as converting non-serializable sets to lists before serialization.
Whether you’re a beginner just starting out or an intermediate programmer looking to handle more complex scenarios, understanding how to convert Python dictionaries to JSON is a valuable skill in your coding repertoire. Keep practicing, keep exploring, and happy coding!