Java Double Keyword: Your Guide to Decimal Precision
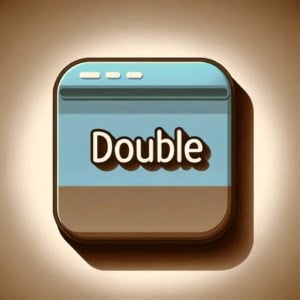
Ever found yourself puzzled about the ‘double’ data type in Java? You’re not alone. Many developers find the concept of ‘double’ a bit tricky, but it’s actually a powerful tool in your programming arsenal.
Think of ‘double’ in Java as a precision instrument. It allows you to work with decimal numbers with a high degree of accuracy, much like a finely-tuned watch. It’s a crucial part of many Java programs, especially those that require precise calculations.
In this guide, we’ll walk you through the ins and outs of using ‘double’ in Java, from the basics to advanced techniques. We’ll cover everything from declaring and initializing ‘double’ variables, performing operations with them, to troubleshooting common issues and considerations.
So, let’s dive in and start mastering the ‘double’ data type in Java!
TL;DR: What is the ‘double’ Keyword in Java?
The
double
keyword is used to identify a data type in Java that is used to store decimal numbers, instantiated with the syntaxdouble var = 3.14;
. It provides a way to work with numbers that have a decimal point and need a high degree of precision.
Here’s a simple example:
double num = 10.5;
System.out.println(num);
# Output:
# 10.5
In this example, we declare a ‘double’ variable named ‘num’ and assign it the value 10.5. When we print the value of ‘num’, we get 10.5 as output. This is a basic use of ‘double’ in Java, but there’s much more to it. ‘double’ is a versatile data type that can be used in a variety of ways in Java programming.
So, if you’re looking to master the use of ‘double’ in Java, from basic to advanced techniques, keep reading. We’ve got a lot of ground to cover!
Table of Contents
- Declaring and Initializing ‘double’ Variables in Java
- Performing Basic Operations with ‘double’ Variables
- Delving Deeper: ‘double’ with Arrays, Methods, and Classes
- Weighing Your Options: ‘double’, ‘float’, and ‘BigDecimal’
- Navigating ‘double’ Pitfalls: Precision Errors and Solutions
- Understanding Java Data Types and the Role of ‘double’
- Extending ‘double’ Use: Real-World Applications and Further Learning
- Wrapping Up: The ‘double’ Data Type in Java
Declaring and Initializing ‘double’ Variables in Java
In Java, you declare a ‘double’ variable by using the keyword ‘double’, followed by the variable name. You can initialize it in the same line or in a separate line. Here’s how you do it:
double num1; // declaration
num1 = 10.5; // initialization
double num2 = 20.5; // declaration and initialization in the same line
In this example, we declare two ‘double’ variables, ‘num1’ and ‘num2’. ‘num1’ is declared first and then initialized with the value 10.5. ‘num2’ is declared and initialized with the value 20.5 in the same line.
Performing Basic Operations with ‘double’ Variables
You can perform basic arithmetic operations with ‘double’ variables, such as addition, subtraction, multiplication, and division. Here’s an example:
double num1 = 10.5;
double num2 = 20.5;
double sum = num1 + num2; // addition
double difference = num2 - num1; // subtraction
double product = num1 * num2; // multiplication
double quotient = num2 / num1; // division
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
# Output:
# Sum: 31.0
# Difference: 10.0
# Product: 215.25
# Quotient: 1.9523809523809523
In this example, we perform basic arithmetic operations with ‘num1’ and ‘num2’ and print the results. The ‘double’ data type allows us to work with decimal numbers and get precise results, as shown by the quotient.
Delving Deeper: ‘double’ with Arrays, Methods, and Classes
The ‘double’ data type in Java isn’t just for simple arithmetic operations. It can also be used in more complex programming constructs such as arrays, methods, and classes.
‘double’ in Arrays
You can create an array of ‘double’ variables to store multiple decimal numbers. Here’s how:
double[] numbers = {10.5, 20.5, 30.5};
for (double number : numbers) {
System.out.println(number);
}
# Output:
# 10.5
# 20.5
# 30.5
In this example, we create an array ‘numbers’ of ‘double’ variables and use a for-each loop to print each number.
‘double’ in Methods
You can also use ‘double’ as the parameter type and return type in methods. Here’s an example:
public static double calculateAverage(double num1, double num2) {
return (num1 + num2) / 2;
}
double average = calculateAverage(10.5, 20.5);
System.out.println("Average: " + average);
# Output:
# Average: 15.5
In this example, we create a method ‘calculateAverage’ that takes two ‘double’ parameters and returns their average, which is also a ‘double’.
‘double’ in Classes
Finally, ‘double’ can be used as the data type for instance variables in classes. Here’s an example:
public class Circle {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double calculateArea() {
return Math.PI * radius * radius;
}
}
Circle circle = new Circle(5.5);
System.out.println("Area: " + circle.calculateArea());
# Output:
# Area: 95.03317777109125
In this example, we create a ‘Circle’ class with a ‘double’ instance variable ‘radius’. The class has a constructor that takes a ‘double’ parameter and a method ‘calculateArea’ that calculates the area of the circle using the ‘double’ instance variable ‘radius’.
Weighing Your Options: ‘double’, ‘float’, and ‘BigDecimal’
While ‘double’ is a powerful tool for working with decimal numbers in Java, it’s not the only one. Other data types, such as ‘float’ and ‘BigDecimal’, can also store decimal numbers, but they each have their own unique characteristics.
‘double’ vs ‘float’
‘float’ is another data type in Java that can store decimal numbers. However, it’s less precise than ‘double’. Here’s a comparison:
double doubleNum = 1.1234567890123456789;
float floatNum = 1.1234567890123456789F;
System.out.println("double: " + doubleNum);
System.out.println("float: " + floatNum);
# Output:
# double: 1.1234567890123457
# float: 1.1234568
In this example, we declare a ‘double’ variable and a ‘float’ variable with the same value. But when we print them, the ‘float’ variable has less precision. So, if you need more precision, ‘double’ is the way to go.
‘double’ vs ‘BigDecimal’
‘BigDecimal’ is a class in Java that provides operations for arbitrary-precision decimal arithmetic. It’s even more precise than ‘double’, but it’s slower. Here’s a comparison:
double doubleNum = 1.1234567890123456789;
BigDecimal bigDecimalNum = new BigDecimal("1.1234567890123456789");
System.out.println("double: " + doubleNum);
System.out.println("BigDecimal: " + bigDecimalNum);
# Output:
# double: 1.1234567890123457
# BigDecimal: 1.1234567890123456789
In this example, we declare a ‘double’ variable and a ‘BigDecimal’ object with the same value. But when we print them, the ‘BigDecimal’ object has more precision. So, if you need the utmost precision and can afford the performance cost, ‘BigDecimal’ is the way to go.
In conclusion, while ‘double’ is a great choice for most cases, ‘float’ and ‘BigDecimal’ can be better in certain situations. It’s all about choosing the right tool for the right job.
While ‘double’ is a powerful tool for handling decimal numbers in Java, it’s not without its quirks. One common issue is precision errors. Let’s delve into this problem and how to avoid it.
Understanding Precision Errors
A precision error occurs when the exact value of a number cannot be represented accurately. This is due to the way decimal numbers are stored in binary format in computers. Here’s an example:
double num = 0.1 + 0.2;
System.out.println(num);
# Output:
# 0.30000000000000004
In this example, you’d expect the output to be 0.3. But due to the way decimal numbers are represented in binary, the result is slightly off. This is a precision error.
Avoiding Precision Errors
One way to avoid precision errors is by using the ‘BigDecimal’ class, which provides operations for arbitrary-precision decimal arithmetic. Here’s how you can do the same operation with ‘BigDecimal’:
BigDecimal num1 = new BigDecimal("0.1");
BigDecimal num2 = new BigDecimal("0.2");
BigDecimal sum = num1.add(num2);
System.out.println(sum);
# Output:
# 0.3
In this example, we use ‘BigDecimal’ to add 0.1 and 0.2. The result is exactly 0.3, as expected. So, if you need to perform operations with decimal numbers and require high precision, consider using ‘BigDecimal’.
Remember, every tool has its quirks. The key is understanding these quirks and knowing how to navigate them. With this knowledge, you can use ‘double’ effectively in your Java programs.
Understanding Java Data Types and the Role of ‘double’
Java has several data types, each with its own purpose and use. They are categorized into two main groups: primitive and reference types. The ‘double’ data type belongs to the primitive group, specifically, it’s a floating-point type.
// Example of declaring a double
double distance = 35.4;
System.out.println(distance);
# Output:
# 35.4
In this example, we declare a ‘double’ variable named ‘distance’ and assign it the value 35.4. When we print the value of ‘distance’, we get 35.4 as output.
The Concept of Precision in Programming
Precision is a critical concept in programming, especially when dealing with floating-point numbers like ‘double’. It refers to the exactness of a number or the amount of detail it can represent. The ‘double’ data type has a precision of about 15 decimal digits, which means it can represent numbers with up to 15 digits accurately.
// Example of precision with double
double preciseNum = 1.123456789012345;
System.out.println(preciseNum);
# Output:
# 1.123456789012345
In this example, we declare a ‘double’ variable named ‘preciseNum’ and assign it a value with 15 decimal digits. When we print the value of ‘preciseNum’, all 15 decimal digits are accurately displayed.
Understanding data types and the concept of precision is fundamental to mastering Java programming. It allows you to choose the right data type for your needs and helps you anticipate and avoid potential issues, such as precision errors.
Extending ‘double’ Use: Real-World Applications and Further Learning
The ‘double’ data type in Java isn’t just for simple arithmetic operations. It has a wide range of applications in larger programs and complex domains.
Mathematical Computations with ‘double’
‘double’ is often used in mathematical computations where precision is crucial. For example, it’s used in algorithms for scientific computing, financial calculations, and statistical analysis.
‘double’ in Graphics and Game Development
In graphics programming and game development, ‘double’ is used to represent coordinates, dimensions, and other values that require decimal points. It’s also used in physics simulations for accurate representation of real-world phenomena.
Exploring ‘BigDecimal’ and ‘BigInteger’
If you’re interested in high-precision arithmetic or handling very large numbers, you might want to explore ‘BigDecimal’ and ‘BigInteger’. These classes provide operations for arbitrary-precision decimal and integer arithmetic, respectively. They’re slower than ‘double’, but they provide more precision and can handle larger numbers.
Further Resources for the ‘double’ data type in Java
To help you delve deeper into the world of ‘double’ in Java, here are some resources you might find useful:
- Getting Started with Java Primitive Data Types – Dive into Java’s boolean type for representing true/false values.
Enum in Java – Explore enums in Java for representing a fixed set of named constants.
Float Data Type in Java – Learn about the range and precision of float variables in Java.
Java Tutorials by Oracle provides a comprehensive overview of data types in Java, including ‘double’.
Java Data Types by W3Schools provides a straightforward breakdown of Java’s numerous data types.
Java ‘double’ Documentation – The official Java documentation for understanding the details of ‘double’ and other data types.
Remember, mastering ‘double’ or any other data type in Java is not just about understanding its syntax and basic operations. It’s also about knowing how to use it effectively in larger programs and different domains. Happy coding!
Wrapping Up: The ‘double’ Data Type in Java
In this comprehensive guide, we’ve explored the ‘double’ data type in Java, a powerful tool for handling decimal numbers with a high degree of precision.
We began with the basics, learning how to declare and initialize ‘double’ variables and perform basic arithmetic operations. We then delved into more advanced usage, exploring how ‘double’ can be used in arrays, methods, and classes. Along the way, we tackled common issues you might encounter when using ‘double’, such as precision errors, and provided solutions to help you navigate these challenges.
We also looked at alternative approaches for handling decimal numbers in Java, comparing ‘double’ with other data types such as ‘float’ and ‘BigDecimal’. Here’s a quick comparison of these data types:
Data Type | Precision | Speed |
---|---|---|
‘double’ | High | Fast |
‘float’ | Moderate | Fast |
‘BigDecimal’ | Very High | Slow |
Whether you’re just starting out with ‘double’ in Java or looking to level up your skills, we hope this guide has given you a deeper understanding of ‘double’ and its capabilities.
With its balance of precision and speed, ‘double’ is a powerful tool for handling decimal numbers in Java. Now, you’re well equipped to use ‘double’ effectively in your Java programs. Happy coding!