Python CSV Handling: Ultimate Guide
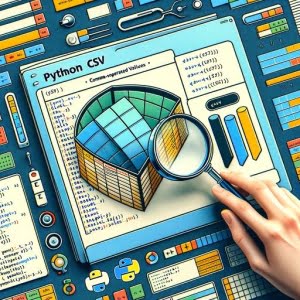
Are you grappling with CSV files in Python? Like a proficient librarian, Python can deftly organize and manipulate CSV data, turning seemingly complex tasks into a breeze.
This guide will walk you through the process of handling CSV files in Python , from reading and writing to advanced manipulation techniques.
Whether you’re a beginner just starting out or an intermediate coder looking to level up your skills, this guide has something for everyone.
TL;DR: How Do I Read a CSV File in Python?
Python’s built-in
csv
module makes it easy to read CSV files. Here’s a basic example:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'Data2', 'Data3']
In this example, we import the csv
module and open a CSV file named ‘file.csv’. We then create a reader object that iterates over lines in the CSV file and print each row. Each row is printed as a list.
This is a simple way to read a CSV file in Python, but there’s so much more to discover about handling CSV files in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Reading and Writing CSV Files in Python
- Handling Large CSV Files and Different Delimiters
- Exploring Alternative Libraries for CSV Handling
- Troubleshooting Common Issues in Python CSV Handling
- Understanding CSV Files and Python’s CSV Module
- The Relevance of CSV Handling in Data Analysis and Machine Learning
- Wrapping Up Python CSV Handling
Reading and Writing CSV Files in Python
Python’s csv
module provides functionality to both read from and write to CSV files. Let’s explore how you can use this in your Python programs.
Reading CSV Files
The csv.reader()
function is used to read data from a CSV file. Here’s a simple example:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'Data2', 'Data3']
In this example, the csv.reader()
function is used to create a reader object. This object iterates over lines in the specified CSV file. Each row from the CSV file is returned as a list and printed out.
Writing to CSV Files
The csv.writer()
function is used to write data into a CSV file. Here’s how you can do it:
import csv
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Column1', 'Column2', 'Column3'])
writer.writerow(['Data1', 'Data2', 'Data3'])
In this example, the csv.writer()
function is used to create a writer object. The writerow()
method writes a row into the CSV file. The row is passed as a list to the writerow()
method.
These are the basics of reading and writing CSV files in Python. However, while these functions are powerful and flexible, they can be tricky to use correctly, especially with complex CSV files. If you’re not careful, you may run into issues with newline characters, different delimiters, or data formatting.
Handling Large CSV Files and Different Delimiters
As you delve deeper into Python CSV handling, you’ll encounter scenarios where you need to deal with large CSV files, different delimiters, or CSV files with headers. Let’s explore these situations.
Reading Large CSV Files
When dealing with large CSV files, it’s not efficient to load the whole file into memory. Instead, you can read the file line by line. Here’s how:
import csv
with open('large_file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
break
# Output:
# ['Column1', 'Column2', 'Column3']
In this example, we only print the first line and then break the loop. This way, we don’t load the entire file into memory, making our program more memory-efficient.
Dealing with Different Delimiters
CSV files can use different delimiters. For instance, some might use semicolons instead of commas. The csv.reader()
function allows you to specify the delimiter. Here’s an example:
import csv
with open('semicolon_delimited.csv', 'r') as file:
reader = csv.reader(file, delimiter=';')
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'Data2', 'Data3']
In this example, we specify the delimiter as a semicolon. The csv.reader()
function will now correctly parse the CSV file.
Working with CSV Files with Headers
CSV files often include a header row. The csv
module provides the csv.DictReader()
function, which treats each row as an ordered dictionary mapped with the header row. Here’s an example:
import csv
with open('file_with_header.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
print(row)
# Output:
# {'Column1': 'Data1', 'Column2': 'Data2', 'Column3': 'Data3'}
In this example, the csv.DictReader()
function reads the CSV file and maps the header row to each data row. Each row is now an ordered dictionary, which you can access with the column names.
Exploring Alternative Libraries for CSV Handling
While Python’s built-in csv
module is powerful, there are alternative libraries like pandas and numpy that offer more advanced features for CSV file handling. Let’s explore these alternatives.
Handling CSV with Pandas
Pandas is a data analysis library that provides high-performance, easy-to-use data structures. It has a function, read_csv()
, for reading CSV files.
import pandas as pd
df = pd.read_csv('file.csv')
print(df)
# Output:
# Column1 Column2 Column3
# 0 Data1 Data2 Data3
In this example, the read_csv()
function reads the CSV file and converts it into a DataFrame, which is a 2-dimensional labeled data structure with columns of potentially different types. You can treat it like a spreadsheet or SQL table, or a dict of Series objects.
Pandas also provides a function, to_csv()
, for writing to CSV files.
import pandas as pd
# Assuming that data is a pandas DataFrame
data.to_csv('file.csv')
In this example, the to_csv()
function writes the DataFrame into a CSV file.
CSV Handling with Numpy
Numpy is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
import numpy as np
data = np.genfromtxt('file.csv', delimiter=',')
print(data)
# Output:
# [[nan nan nan]
# [ 1. 2. 3.]]
In this example, the genfromtxt()
function reads the CSV file and returns an array, which is a powerful N-dimensional array object.
While these libraries offer more advanced features, they also have their own learning curve and may be overkill for simple CSV handling tasks. If you’re dealing with complex or large datasets, these libraries can be a good choice. Otherwise, Python’s built-in csv
module is more than sufficient.
Troubleshooting Common Issues in Python CSV Handling
Working with CSV files in Python isn’t always a smooth ride. You may encounter issues like encoding errors or problems with newline characters. Let’s discuss these common issues and their solutions.
Encoding Errors
When dealing with CSV files, you might come across different encodings. If you try to read a file with an encoding that Python doesn’t recognize, you’ll get an error. Here’s how to handle it:
import csv
try:
with open('file.csv', 'r', encoding='utf-8') as file:
reader = csv.reader(file)
for row in reader:
print(row)
except UnicodeDecodeError:
print('UnicodeDecodeError has occurred. Please check the file encoding.')
# Output:
# UnicodeDecodeError has occurred. Please check the file encoding.
In this example, we try to read the file with ‘utf-8’ encoding. If the file has a different encoding, a UnicodeDecodeError
is raised. We catch this error and print a helpful message.
Handling Newline Characters
When writing CSV files in Python, you might encounter issues with newline characters. Here’s a way to handle it:
import csv
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Column1', 'Column2', 'Column3'])
writer.writerow(['Data1', 'Data2', 'Data3'])
In this example, we pass newline=''
when opening the file for writing. This ensures that the newline characters are handled correctly regardless of your platform.
These are just a few of the issues you might encounter when working with CSV files in Python. The key is to understand the cause of the issue and find the appropriate solution.
Understanding CSV Files and Python’s CSV Module
Before we delve deeper into handling CSV files with Python, let’s take a moment to understand what CSV files are and how the csv
module in Python works.
What are CSV Files?
CSV stands for Comma Separated Values. It’s a simple file format used to store tabular data, such as a spreadsheet or a database. Each line of the file is a data record, and each record consists of one or more fields, separated by commas.
Column1,Column2,Column3
Data1,Data2,Data3
In this example of a CSV file, the first line is the header, and the following lines are data records. The fields in each record are separated by commas.
Python’s CSV Module
Python’s csv
module is a built-in module for reading and writing CSV files. It provides functions like reader()
, writer()
, DictReader()
, and DictWriter()
, allowing you to work with CSV files in various ways.
import csv
# csv.reader example
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'Data2', 'Data3']
In this example, we use the csv.reader()
function to read a CSV file. The reader()
function returns a reader object which iterates over lines in the CSV file.
By understanding the structure of CSV files and the functions provided by Python’s csv
module, you’ll be better equipped to handle CSV files in your Python programs.
The Relevance of CSV Handling in Data Analysis and Machine Learning
Handling CSV files is not just a programming exercise. It’s a vital skill in fields like data analysis and machine learning. Let’s explore why.
CSV Files in Data Analysis
In data analysis, CSV files are often used as a convenient way to store and share large datasets. Python’s ability to read, write, and manipulate CSV files allows data analysts to clean, analyze, and visualize data effectively.
import pandas as pd
df = pd.read_csv('data.csv')
df = df.dropna()
print(df.describe())
# Output:
# count mean std min 25% 50% 75% max
# 10 5.5 3.03 1.0 3.25 5.5 7.75 10
In this example, we read a CSV file into a pandas DataFrame, drop any rows with missing values, and then print the descriptive statistics of the DataFrame. This is a typical data cleaning and preliminary analysis process in data analysis.
CSV Files in Machine Learning
In machine learning, CSV files are often used to store training and testing datasets. Python’s CSV handling capabilities enable machine learning practitioners to preprocess and transform these datasets for machine learning models.
import pandas as pd
from sklearn.model_selection import train_test_split
df = pd.read_csv('data.csv')
X = df.drop('target', axis=1)
y = df['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Output:
# Split the dataset into 80% training data and 20% testing data
In this example, we read a CSV file into a pandas DataFrame, split the DataFrame into features (X) and target (y), and then split the data into training and testing sets. This is a typical process in preparing data for a machine learning model.
Further Resources for Python CSV Mastery
To deepen your understanding of handling CSV files in Python, here are some useful resources:
- This Guide on JSON Usage in Python by IOFlood explores the art of working with JSON arrays, objects, and keys.
Effortless CSV File Reading in Python – Master the art of working with tabular data from CSV files in Python.
JSON Parsing in Python – Techniques and examples on JSON parsing and navigation in Python.
Official Python CSV Module Documentation offers a detailed understanding of the CSV module.
Pandas’ Guide on Reading and Writing CSV Files is the official documentation of pandas library for reading and writing Python CSV files.
Numpy’s Documentation on genfromtxt Function covers the genfromtxt function for loading data from text files.
These resources provide in-depth explanations and more examples on how to handle CSV files in Python. Happy learning!
Wrapping Up Python CSV Handling
In this comprehensive guide, we’ve covered a wide range of topics related to handling CSV files in Python.
We began with understanding the fundamentals of CSV files and how Python’s csv
module can be used to read and write these files. We explored the basic usage of the csv.reader()
and csv.writer()
functions, and also delved into more advanced topics like dealing with large files, different delimiters, and CSV files with headers.
We also discussed common issues you might encounter when working with CSV files in Python, such as encoding errors and newline character issues, and provided solutions to these problems.
Furthermore, we explored alternative approaches for handling CSV files using pandas and numpy libraries, highlighting their advanced features and uses in data analysis and machine learning.
Here’s a quick comparison of the different methods we discussed:
Method | Use Case | Complexity |
---|---|---|
csv module | Basic reading and writing | Low |
csv module (advanced) | Large files, different delimiters, headers | Medium |
pandas | Data analysis, machine learning | High |
numpy | Large arrays and matrices | High |
Each method has its own advantages and use cases, and the best one to use depends on your specific needs and the complexity of your data.
Remember, mastering python csv
handling is not just about knowing the functions and libraries. It’s about understanding the data you’re working with and choosing the right tools and approaches to handle it effectively. Keep practicing and exploring, and you’ll become proficient in no time!